iOS/iPhone Display Image on Table View from JSON URL (Web Site) |
iOS/iPhone Display Image on Table View from JSON URL (Web Site) ถ้าเคยใช้ App บน iPhone ที่เกี่ยวกับพวกข่าว News เช่น Thairath Lite , Post Today หรือ อื่น ๆ จะเห็นว่าในรายการที่แสดงข่าว จะมีการใช้ Table View ที่แสดงหัวข้อข่าว และภาพ Screenshot เล็ก ๆ อยู่ข้างหน้าในแต่ล่ะ Cell เราอาจจะสงสัยว่ามันทำยังไง ที่ข้อมูลเหล่านี้เมื่อมีข่าวใหม่ ๆ จะแสดง Update จาก Server ได้ตลอดเวลา และผมจะบอกว่าสิ่งที่เราเห็นนั้นมันสามารถทำได้ง่าย ๆ โดยไม่ยากเลย สำหรับ App เหล่านั้นเราอาจจะไม่รู้ว่าเค้าใช้วิธีหรือเทคโนโลยี่แบบไหนในการที่จะอ่านข้อมูลจาก Web Server แต่ผมจะมาแนะนำวิธีที่ง่ายสุด ๆ สามารถเขียนได้ง่าย ๆ ด้วยสิ่งที่เราสามารถทำได้จริง ๆ
iOS/iPhone Display Image on Table View from JSON URL (Web Site)
ตัวอย่าง App ของ Thairath คาดว่าน่าจะใช้ Table View แสดง Image และ Text
สำหรับวิธีนั้นก็คือเราจะออกแบบ Application ที่ทำหน้าที่กระจ่ายข้อมูลซึ่งจะทำงานอยู่บน Web Server ด้วย PHP กับ MySQL Database โดยที่ Web Server จะสร้าง URL ของ php ขึ้นมา 1 ตัว ที่จะทำหน้าที่อ่านข้อมูลจาก MySQL มาเข้ารหัสด้วยเทคโนโลยี่ของ JSON และเมื่อ iOS ทำการ Request ผ่าน URL นั้น ๆ ก็จะส่งค่า JSON ไปยัง iOS ที่ทำหน้าที่เป็น Client และอีกอย่างหนึ่งที่เรา Focus คือ ข้อมูลที่เป็น Image รูปภาพนั้น เราจะไม่สามารถส่งไปกับ JSON ได้ แต่เราจะส่งไปแค่ URL ที่เป็น Path ของ Image โดยที่ iOS จะสามารถอ่าน URL ของ Image นั้น ๆ และเรียกรูปภาพเพื่อแสดงบน Table View ของแต่ล่ะ Cell
อ่านเพิ่มเติมเพื่อความเข้าใจ
iOS/iPhone PHP/MySQL and JSON Parsing (Objective-C)
iOS/iPhone Table View Image Column Multiple Column (Objective-C,iPhone)
Web Server ทำหน้าที่อ่านข้อมูลจาก MySQL Database ด้วย PHP
MySQL Database
CREATE TABLE `gallery` (
`GalleryID` int(3) NOT NULL auto_increment,
`Name` varchar(50) NOT NULL,
`TitleName` varchar(150) NOT NULL,
`Thumbnail` varchar(100) NOT NULL,
PRIMARY KEY (`GalleryID`)
) ENGINE=MyISAM AUTO_INCREMENT=5 ;
--
-- Dumping data for table `gallery`
--
INSERT INTO `gallery` VALUES (1, 'Name 1', 'The my gallery title 1', 'https://www.thaicreate.com/url/girl1.jpg');
INSERT INTO `gallery` VALUES (2, 'Name 2', 'The my gallery title 2', 'https://www.thaicreate.com/url/girl2.jpg');
INSERT INTO `gallery` VALUES (3, 'Name 3', 'The my gallery title 3', 'https://www.thaicreate.com/url/girl3.jpg');
INSERT INTO `gallery` VALUES (4, 'Name 4', 'The my gallery title 4', 'https://www.thaicreate.com/url/girl4.jpg');
โครงสร้างของ MySQL Database
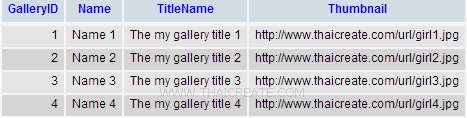
getGallery.php ไฟล์ PHP สำหรับอ่านข้อมูลจาก MySQL Database
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM gallery WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
รายละเอียดของ PHP ไฟล์ เข้ารหัสแปลงข้อมูลเป็น JSON คำสั่งง่าย ๆ ด้วย json_encode()
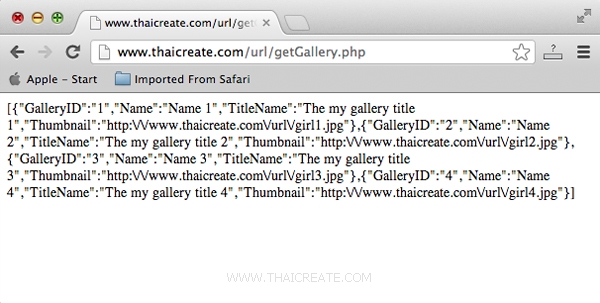
เมื่อทดสอบบน Web Browser จะได้ JSON ดังรูป
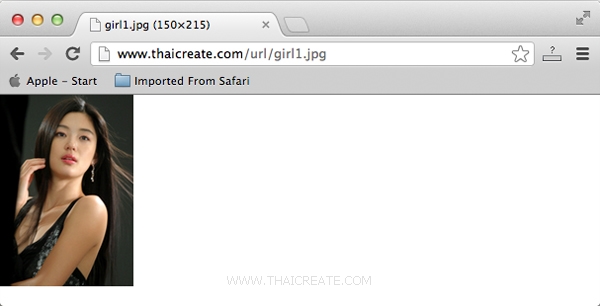
เป็นไฟล์รูปภาพเมื่อเรียกจาก Web Browser ตาม Path ที่เก็บใน MySQL
และจาก PHP กับ MySQL Database ที่อยู่บน Web Server เราสามารถเขียน Code ของ Objective-C สำหรับการอ่านข้อมูลและแปลงให้อยู่ในรุปแบบของ Array สำหรับการแสดงผลบน Table View (UITableView) ได้ดังนี้
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *galleryid;
NSString *name;
NSString *titlename;
NSString *thumbnail;
// Define keys
galleryid = @"GalleryID";
name = @"Name";
titlename = @"TitleName";
thumbnail = @"Thumbnail";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSData *jsonData = [NSData dataWithContentsOfURL:
[NSURL URLWithString:@"https://www.thaicreate.com/url/getGallery.php"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strGalleryID = [dataDict objectForKey:@"GalleryID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTitleName = [dataDict objectForKey:@"TitleName"];
NSString *strThumbnail = [dataDict objectForKey:@"Thumbnail"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strGalleryID, galleryid,
strName, name,
strTitleName, titlename,
strThumbnail, thumbnail,
nil];
[myObject addObject:dict];
}
Example ทดสอบ Image Column บน Table View ด้วย JSON จาก PHP และ MySQL
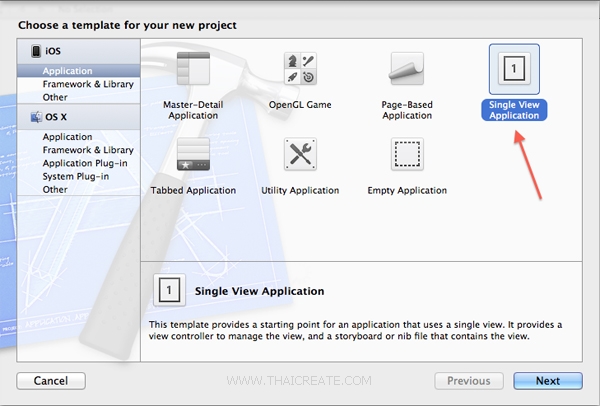
เริ่มต้นด้วยการสร้าง Application บน Xcode แบบ Single View Application
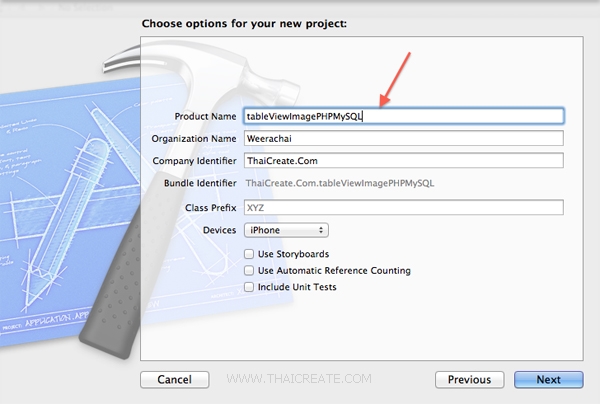
เลือกและไม่เลือกรายการดังรูป
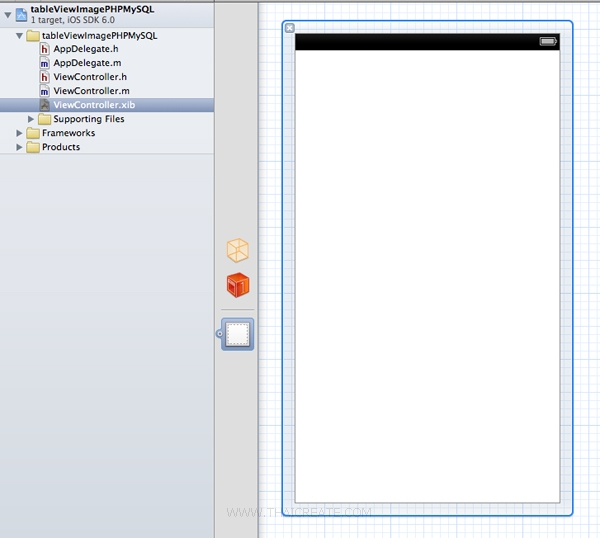
ตอนนี้หน้าจอ View ยังว่าง ๆ
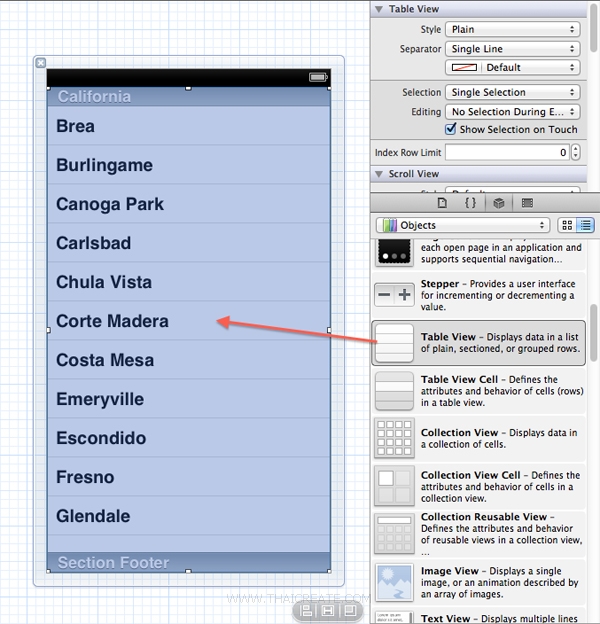
จาก Table View มาไว้ที่หน้าจอของ View
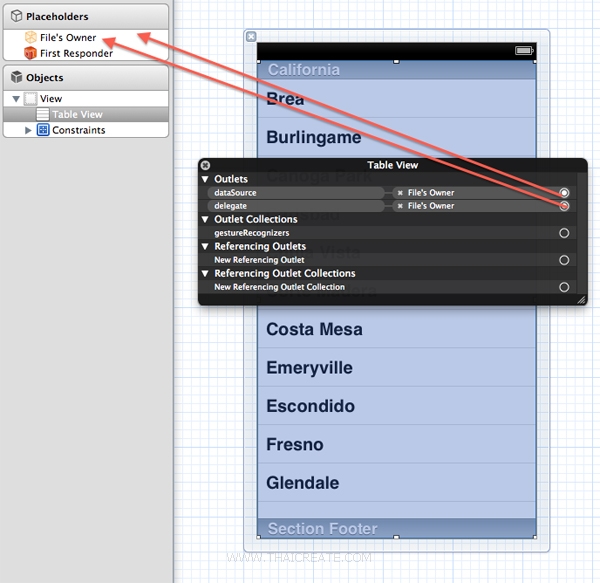
คลิกขวาที่ Table View ให้ทำการเชื่อม dataSoruce กับ delegate กับ File's Owner
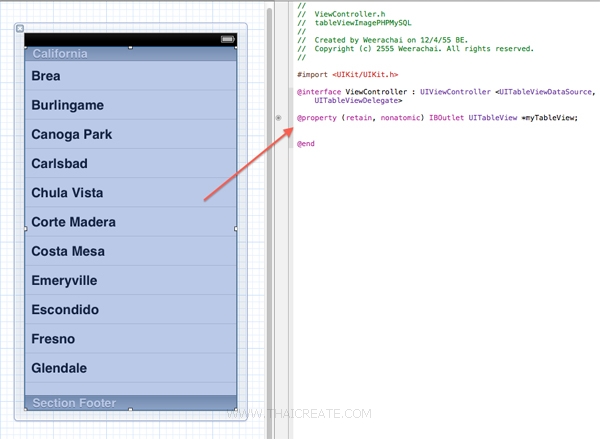
ใน Class ของ .h ให้ทำการเชื่อม IBOutlet ให้เรียบร้อย และก็ใส่ extend คลาสของ <UITableViewDataSource,UITableViewDelegate>
อันนี้เป็น Code ทั้งหมดของ .h และ .m
ViewController.h
//
// ViewController.h
// tableViewImagePHPMySQL
//
// Created by Weerachai on 12/4/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController <UITableViewDataSource,UITableViewDelegate>
@property (retain, nonatomic) IBOutlet UITableView *myTableView;
@end
ViewController.m
//
// ViewController.m
// tableViewImagePHPMySQL
//
// Created by Weerachai on 12/4/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
{
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *galleryid;
NSString *name;
NSString *titlename;
NSString *thumbnail;
}
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Define keys
galleryid = @"GalleryID";
name = @"Name";
titlename = @"TitleName";
thumbnail = @"Thumbnail";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSData *jsonData = [NSData dataWithContentsOfURL:
[NSURL URLWithString:@"https://www.thaicreate.com/url/getGallery.php"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strGalleryID = [dataDict objectForKey:@"GalleryID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTitleName = [dataDict objectForKey:@"TitleName"];
NSString *strThumbnail = [dataDict objectForKey:@"Thumbnail"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strGalleryID, galleryid,
strName, name,
strTitleName, titlename,
strThumbnail, thumbnail,
nil];
[myObject addObject:dict];
}
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return myObject.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
NSURL *url = [NSURL URLWithString:[tmpDict objectForKey:thumbnail]];
NSData *data = [NSData dataWithContentsOfURL:url];
UIImage *img = [[UIImage alloc] initWithData:data];
cell.imageView.image = img;
cell.textLabel.text = [tmpDict objectForKey:name];
cell.detailTextLabel.text= [tmpDict objectForKey:titlename];
//[tmpDict objectForKey:memberid]
//[tmpDict objectForKey:name]
//[tmpDict objectForKey:titlename]
//[tmpDict objectForKey:thumbnail]
return cell;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[_myTableView release];
[super dealloc];
}
@end
สำหรับ Code ไม่มีอะไรมากมาย สามารถอ่านแล้วเข้าใจ หรือไม่ก็กลับไปอ่านพื้นฐานเกี่ยวกับ Table View และ JSON ตามบทความที่ได้แนะนำไว้ก่อนหน้านี้
Screenshot
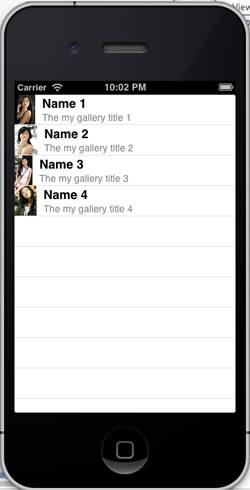
แสดงข้อมูล Image บน Table View ที่มาจาก JSON ด้วย PHP กับ MySQL Database
เพิ่มเติม
จากตัวอย่างนี้เป็นการแสดงข้อมูลบน Table View แบบง่าย ๆ และไม่มีอะไรซับซ้อน และสำหรับคำอธิบายสามารถอ่านได้จากพื้นฐานของบทความ Table View
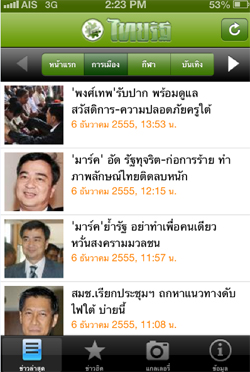
และในกรณีที่ข้อมูลมีความซับซ้อน หรือ การจัดรูปแบบ Cell ตามต้องการ เหมือนกับ App ของ Thairath LITE ก็สามารถอ่านได้จากบทความของ Table View Custom Cell ตามลิงค์นี้
iOS/iPhone TableView and UITableView (Objective-C, iPhone, iPad)
iOS/iPhone Table View and Table View Cell - Custom Cell Column (iPhone, iPad)
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-12-06 12:09:39 /
2017-03-26 09:04:02 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|