iOS/iPhone and JSON (Create JSON and JSON Parsing, Objective-C) |
iOS/iPhone and JSON (Create JSON and JSON Parsing , Objective-C) เข้าสู่โลกของ iOS และ JSON ในการเขียน Application บน iPhone หรือ iPad หรือ App อื่น ๆ ทำงานระหว่าง Web Server กับ Client หรือ Web Server ต่อ Web Server นั้น ในการแลกเปลี่ยนข้อมูลระหว่างกันสิ่งที่ขาดไม่ได้ก็คือ JSON เพราะมันเป็นมาตรฐานที่ได้ถูกใช้กันอย่างหลากหลาย ช่วยให้การรับส่งข้อมูลนั้นง่าย และ เกิดประสิทธิภาพ ถูกต้องและแม่นยำ สามารถส่งข้อมลที่เป็นชุดของข้อมูลที่อยู่ในรูปแบบของ Array ที่ประกอบด้วยหลาย Index และหลายมิติ และฝั่งที่เป็นฝั่งรับข้อมูลก็สามารถที่จะทำการ DeCode หรือ Parsing ข้อมูลในรูปแบบของ JSON ได้อย่างถูกต้อง
iOS/iPhone and JSON (Create JSON and JSON Parsing)
ตัวอย่าง JSON แบบ Array มิติเดียว
{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }
ตัวอย่าง JSON แบบ Array หลาย Index และหลายมิติ
[{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
ในบทความนี้ผมจะรวบรวมการใช้งาน JSON บน iOS (Objective-C) ที่เป็นพื้นฐานที่จะนำไปใช้งานเกือบทั้งหมด เช่น การอ่าน JSON ในรูปแบบของ Array มิติเดียว หรือ Array หลายมิติ และหลาย Index รวมทั้งการสร้างเข้ารหัส JSON การอ่าน JSON จาก URL ที่อยู่บน Web Server โดยทั้งหมดนี้เราจะใช้ Class ของ NSJSONSerialization ที่อยู่ในภาษา Objecttive-C ที่สามารถเรียกใช้งานได้แบบง่าย ๆ
Ex 1 : การอ่าน JSON แบบ Array มิติเดียว
ข้อความ JSON
{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }
// { "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }
NSString *stringData = @"{ \"MemberID\":\"1\", \"Name\":\"Weerachai\", \"Tel\":\"0819876107\" }";
NSData *jsonData = [stringData dataUsingEncoding:NSUTF8StringEncoding];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
NSArray *keys = [jsonObjects allKeys];
// value in key name
NSString *strMemberID = [jsonObjects objectForKey:@"MemberID"];
NSString *strName = [jsonObjects objectForKey:@"Name"];
NSString *strTel = [jsonObjects objectForKey:@"Tel"];
NSLog(@"MemberID = %@",strMemberID);
NSLog(@"Name = %@",strName);
NSLog(@"Tel = %@",strTel);
NSLog(@"====================");
// values in foreach loop
for (NSString *key in keys) {
NSLog(@"%@ is %@",key, [jsonObjects objectForKey:key]);
}
Screenshot
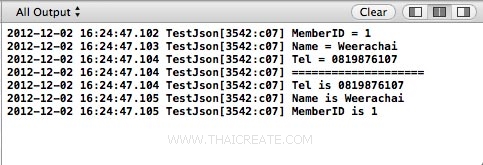
แสดงค่า JSON ที่ได้
Ex 2 : การอ่าน JSON จาก URL ของเว็บ URL (Website)
https://www.thaicreate.com/json/
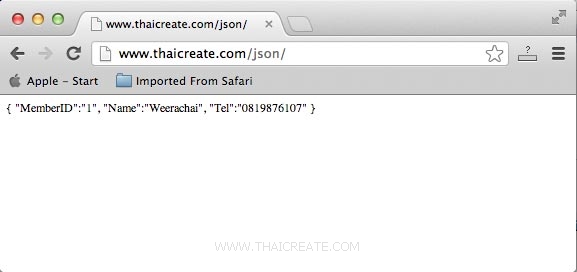
ไฟล์ JSON และ URL ที่อยู่บนเว็บไซต์
// { "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }
NSData *jsonData = [NSData dataWithContentsOfURL:[NSURL URLWithString:@"https://www.thaicreate.com/json/"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
NSArray *keys = [jsonObjects allKeys];
// value in key name
NSString *strMemberID = [jsonObjects objectForKey:@"MemberID"];
NSString *strName = [jsonObjects objectForKey:@"Name"];
NSString *strTel = [jsonObjects objectForKey:@"Tel"];
NSLog(@"MemberID = %@",strMemberID);
NSLog(@"Name = %@",strName);
NSLog(@"Tel = %@",strTel);
NSLog(@"====================");
// values in foreach loop
for (NSString *key in keys) {
NSLog(@"%@ is %@",key, [jsonObjects objectForKey:key]);
}
Screenshot
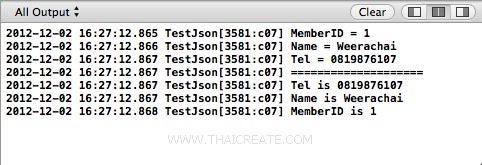
แสดงค่า JSON ที่ได้
Ex 3 : การอ่าน JSON แบบ Array หลายมิติ และ หลาย Index
https://www.thaicreate.com/json/
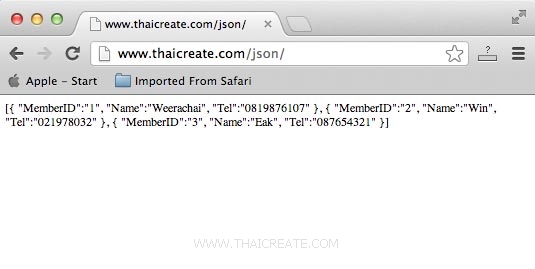
ไฟล์ JSON และ URL ที่อยู่บนเว็บไซต์
ข้อความ JSON
[{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
// [{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
NSData *jsonData = [NSData dataWithContentsOfURL:[NSURL URLWithString:@"https://www.thaicreate.com/json/"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
NSLog(@"MemberID = %@",strMemberID);
NSLog(@"Name = %@",strName);
NSLog(@"Tel = %@",strTel);
NSLog(@"====================");
}
Screenshot
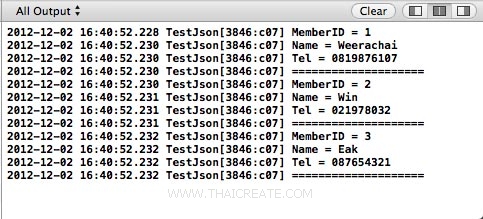
แสดงค่า JSON ที่ได้
Ex 4 : การสร้าง Create ข้อมูลให้อยู่ในรูปแบบ JSON แบบ Array มิติเดียว
ข้อความ JSON ที่ต้องการ
{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }
/*** Create JSON ***/
NSMutableDictionary *nameElements = [NSMutableDictionary dictionary];
[nameElements setObject:@"1" forKey:@"MemberID"];
[nameElements setObject:@"Weerachai" forKey:@"Name"];
[nameElements setObject:@"0819876107" forKey:@"Tel"];
NSData *jsonData = [NSJSONSerialization dataWithJSONObject:nameElements
options:NSJSONWritingPrettyPrinted
error:nil];
// Result
// { "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }
/*** Read JSON ***/
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
NSArray *keys = [jsonObjects allKeys];
// values in foreach loop
for (NSString *key in keys) {
NSLog(@"%@ is %@",key, [jsonObjects objectForKey:key]);
}
Screenshot
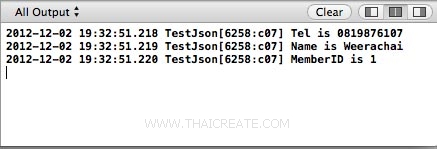
แสดงค่า JSON ที่ได้ จากการสร้าง JSON และ อ่านค่า JSON
Ex 5 : การสร้าง Create ข้อมูลให้อยู่ในรูปแบบ JSON แบบ Array หลายมิติ และ หลาย Index
ข้อความ JSON ที่ต้องการ
[{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
/*** Create JSON ***/
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *memberid;
NSString *name;
NSString *tel;
// Define keys
memberid = @"MemberID";
name = @"Name";
tel = @"Tel";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"1", memberid,
@"Weerachai", name,
@"0819876107", tel,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"2", memberid,
@"Win", name,
@"021978032", tel,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"3", memberid,
@"Eak", name,
@"087654321", tel,
nil];
[myObject addObject:dict];
NSData *jsonData = [NSJSONSerialization dataWithJSONObject:myObject
options:NSJSONWritingPrettyPrinted
error:nil];
// Resutl
// [{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
/*** Read JSON ***/
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
NSLog(@"MemberID = %@",strMemberID);
NSLog(@"Name = %@",strName);
NSLog(@"Tel = %@",strTel);
NSLog(@"====================");
}
Screenshot
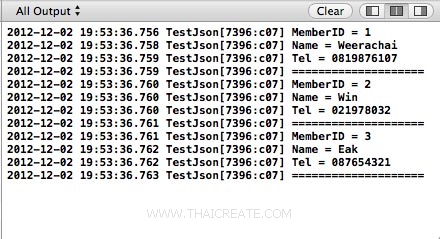
แสดงค่า JSON ที่ได้ จากการสร้าง JSON และ อ่านค่า JSON
จากตัวอย่าง 5 ตอนถือได้ว่าเกือบครบทั้งหมดสำหรับการใช้งาน JSON เบื้องต้น เช่นการแปลง JSON และอ่าน JSON จาก URL (Website) และการสร้าง String ให้อยู่ในรูปแบบของ JSON ซึ่งอาจจะนำไปใช้ในการส่งค่าผ่าน View ต่าง ๆ ซึ่งบทความทั้งหมดเหล่านี้ถือได้ว่าผมได้รวบรวมไว้ให้ได้ใช้งานและเข้าใจง่ายที่สุด ที่เหลือคือการนำไปประยุกต์ใช้กับส่วนต่าง ๆ ของโปรแกรมได้ ลองมาดูการใช้ JSON กับ Table View ตามตัวอย่างนี้กันครับ
iOS/iPhone Table View and JSON (UITableView from JSON Parser)
.
|