iOS/iPhone NSURLConnection POST Method and Send Parameter (Objective-C) |
iOS/iPhone NSURLConnection POST Method and Send Parameter (Objective-C) การ POST Method เป็นการส่งค่า POST จาก iOS ไปยัง URL ปลายทาง โดยปลายทางสามารถรับค่า POST ได้เหมือน ๆ กับการเปขียนโปรแกรมทั่ว ๆ ไป เช่น PHP จะใช้ $_POST หรือ ASP/ASP.NET จะใช้ Request.Form() ในการใช้ POST บน iOS เราสามารถ Apply เขียนโปรแกรมได้หลากหลายมาก เช่น การเขียนระบบลงทะเบียนผ่าน iPhone ที่ทำหน้าที่เป็น Client โดยเราจะออกแบบ Form บนหน้าจอ View และส่งข้อมูล POST ไปยังปลายทางโดยจะส่งผ่าน URL ของ Server พร้อม ๆ กับ ที่ Server จะส่งค่ากลับมายัง Client
iOS/iPhone NSURLConnection POST Method and Send Parameter
ในการส่งผ่าน POST เราจะใช้ Class ของ NSMutableURLRequest และ NSURLConnection ที่จะใช้กำหนด URL ปลายทาง พร้อม ๆ กับการเชื่อมต่อไปยัง Web Server ซึ่งเราอาจจะใช้ PHP / ASP / ASP.NET หรือภาษาอื่น ๆ ก็แล้วแต่
Method POST /NSMutableURLRequest/NSURLConnection
//Name=Weerachai&Surname=Nukitram"
NSMutableString *post = [NSString stringWithFormat:@"Name=%@&Surname=%@",[txtName text],[txtSurname text]];
NSData *postData = [post dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%d", [postData length]];
NSURL *url = [NSURL URLWithString:@"https://www.thaicreate.com/url/post.php"];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-Length"];
[request setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
[request setHTTPBody:postData];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:request delegate:self];
ในการส่งค่า POST เราสามารถกำหนดรุปแบบได้ตามตัวอย่าง
NSMutableString *post = [NSString stringWithFormat:@"Name=%@",[txtName text]];
หรือ
NSMutableString *post = [NSString stringWithFormat:@"Name=%@&Surname=%@",[txtName text],[txtSurname text]];
อ่านเพิ่มเติม iOS/iPhone NSURLConnection (Objective-C)
Example การใช้งาน Method POST ส่งค่าไปยัง PHP และการรับค่าที่ Server ส่งกลับ
post.php
<?php
echo "Sawatdee Khun ".$_POST["Name"]." ".$_POST["Surname"];
?>
ไฟล์ php ที่จะทำหน้าที่อ่านค่า POST และส่งกลับไปยัง Client
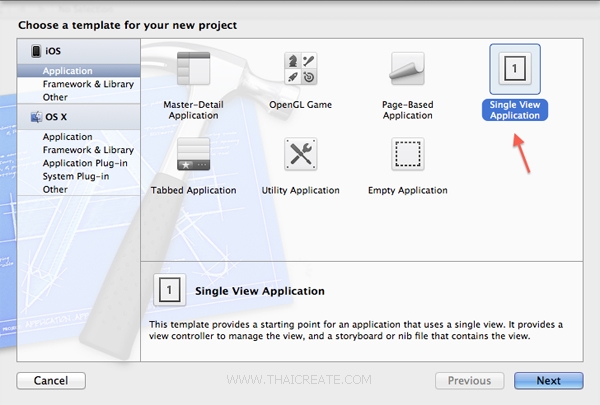
เริ่มต้นด้วยการสร้าง Application แบบ Single View Application
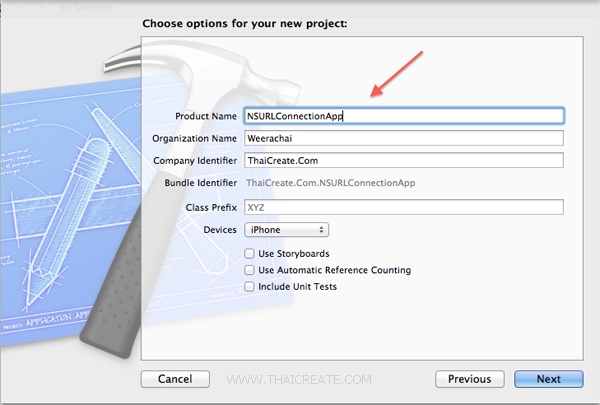
เลือกและไม่เลือกรายการดังรูป
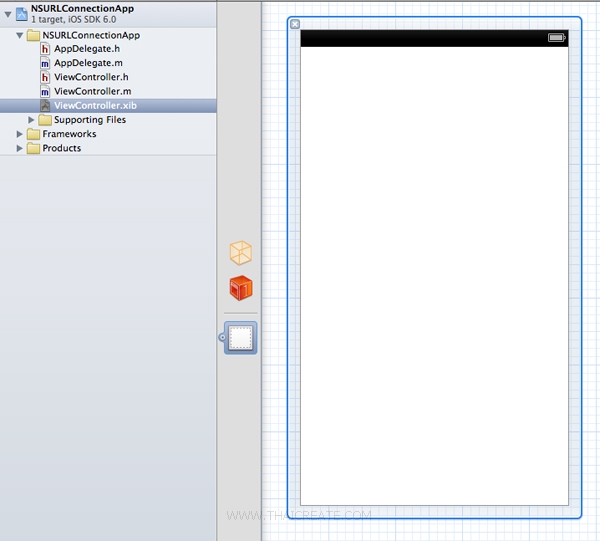
ตอนนี้หน้าจอของเราจะยังว่าง ๆ
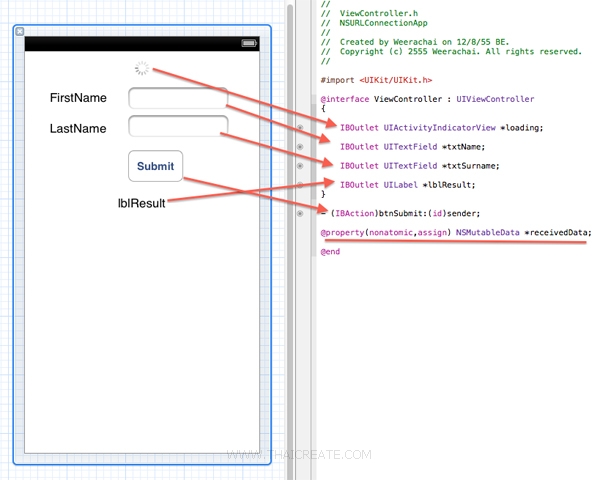
ออกแบบหน้าจอด้วย Object ต่าง ๆ ดังรูป ซึ่งประกอบด้วย Label , Text Fields, Button และ Activity Indicator View จากนั้นให้เชื่อม IBOutlet และ IBAction ดังรูป และเขียน Code ต่าง ๆ ดังนี้
ViewController.h
//
// ViewController.h
// NSURLConnectionApp
//
// Created by Weerachai on 12/8/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UIActivityIndicatorView *loading;
IBOutlet UITextField *txtName;
IBOutlet UITextField *txtSurname;
IBOutlet UILabel *lblResult;
}
- (IBAction)btnSubmit:(id)sender;
@property(nonatomic,assign) NSMutableData *receivedData;
@end
ViewController.m
//
// ViewController.m
// NSURLConnectionApp
//
// Created by Weerachai on 12/8/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
@end
@implementation ViewController
@synthesize receivedData;
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
[loading setHidden:TRUE];
}
- (IBAction)btnSubmit:(id)sender {
[loading setHidden:FALSE];
//Name=Weerachai&Surname=Nukitram"
NSMutableString *post = [NSString stringWithFormat:@"Name=%@&Surname=%@",[txtName text],[txtSurname text]];
NSData *postData = [post dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%d", [postData length]];
NSURL *url = [NSURL URLWithString:@"https://www.thaicreate.com/url/post.php"];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-Length"];
[request setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
[request setHTTPBody:postData];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:request delegate:self];
[loading startAnimating];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
sleep(5);
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
[connection release];
[receivedData release];
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
[didFailWithErrorMessage release];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
if(receivedData)
{
NSLog(@"%@",receivedData);
NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
NSLog(@"%@",dataString);
lblResult.text = dataString;
[loading stopAnimating];
[loading setHidden:TRUE];
}
// release the connection, and the data object
[connection release];
[receivedData release];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[loading release];
[txtName release];
[txtSurname release];
[lblResult release];
[super dealloc];
}
@end
Screenshot

กรอกข้อมูลบน Text Fields ตามหน้าจอ
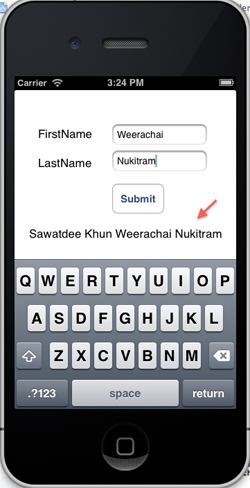
คลิกที่ Button ก็จะมีการส่งค่า POST ไปยัง URL ของ php และ php ก็จะส่งค่ากลับมา
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-12-13 09:49:04 /
2017-03-26 08:58:02 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|