iOS/iPhone Retrieve Read List Show Data from Web Server (URL,Website,PHP,MySQL) |
iOS/iPhone Retrieve List Show Data from Web Server (URL,Website) หลังจากที่ Insert ข้อมูลลงใน Database ของ MySQL ที่อยู่บน Web Server แล้ว ในบทความนี้เราจะมาอ่านข้อมูลต่าง ๆ ที่อยู่บน Web Server โดยใช้ PHP อ่านข้อมูล และใช้ JSON แสดงข้อมูลจาก Web Server เช่นเดิม จะทำการแสดงผลบน Table View (UITableView) โดยบทความนี้เป็นเพียงการทบทวนความรู้และ Apply ใช้งานจากบทความก่อน ๆ เพื่อให้เข้าใจการทำงานอีกครั้ง
iOS/iPhone Retrieve List Show Data from Web Server (URL,Website)
ผมจะไม่ขอธิบายการทำงานของ PHP กับ MySQL เพระาคาดว่าทุกคนที่มาเขียน iOS คงจะได้ผ่านการเขียน PHP กับ MySQL กันมาบ้างพอแล้ว หรือไม่ก็สามารถ Apply ใช้กับ Application ได้เช่นเดียวกัน เช่น ASP / ASP.NET หรือ JSP กับฐานข้อมูลต่าง ๆ ที่ต้องการ
iOS/iPhone Add Insert Data to Web Server (URL,Website)
Example การอ่าข้อมูลจาก Web Server มาแสดงบน Table View (UITableView)
MySQL Database
CREATE TABLE `member` (
`MemberID` int(11) NOT NULL auto_increment,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
PRIMARY KEY (`MemberID`)
) ENGINE=MyISAM AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'Weerachai', '0819876107');
INSERT INTO `member` VALUES (2, 'Win', '021978032');
INSERT INTO `member` VALUES (3, 'Eak', '0876543210');
โครงสร้างของฐานข้อมูล MySQL Database
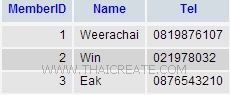
getData.php ไฟล์ php สำหรับอ่านข้อมูลจาก MySQL Database
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
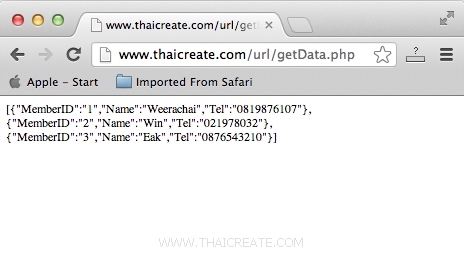
ทดสอบเรียกไฟล์ PHP จะได้ JSON Code ดังรูป
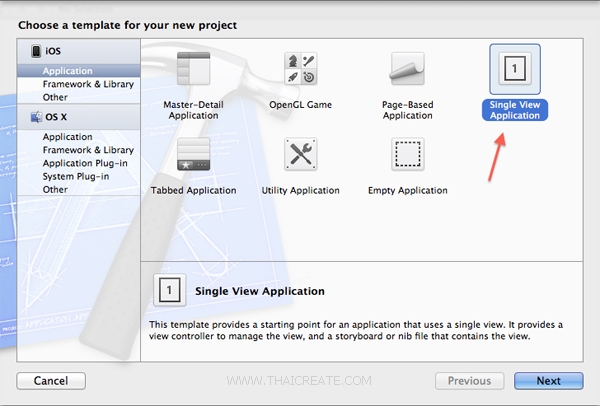
เริ่มต้นด้วยการสร้าง Application บน Xcode แบบ Single View Application
เลือกและไม่เลือกรายการดังรูป
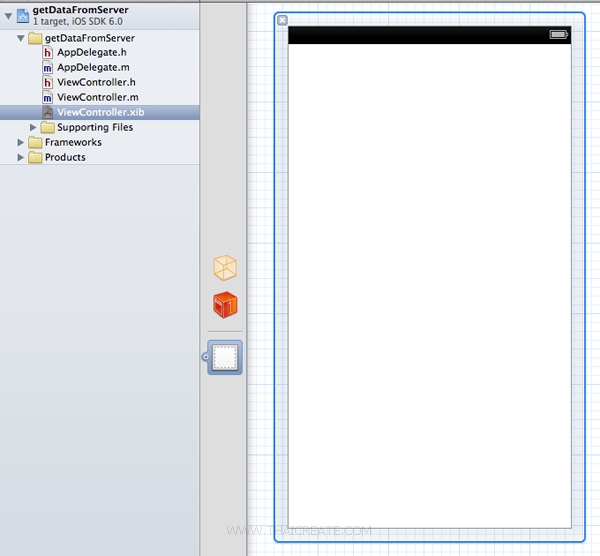
ตอนนี้หน้าจอ View ของเรายังว่าง ๆ
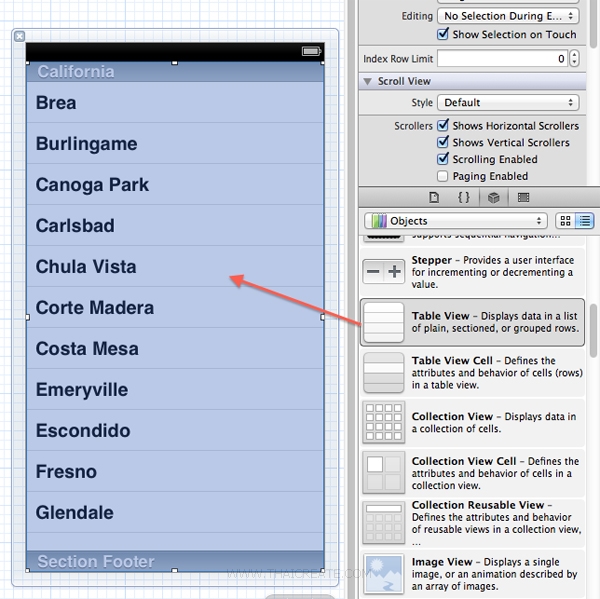
ให้ลาก Table View มาวางไว้ที่หน้าจอของ View
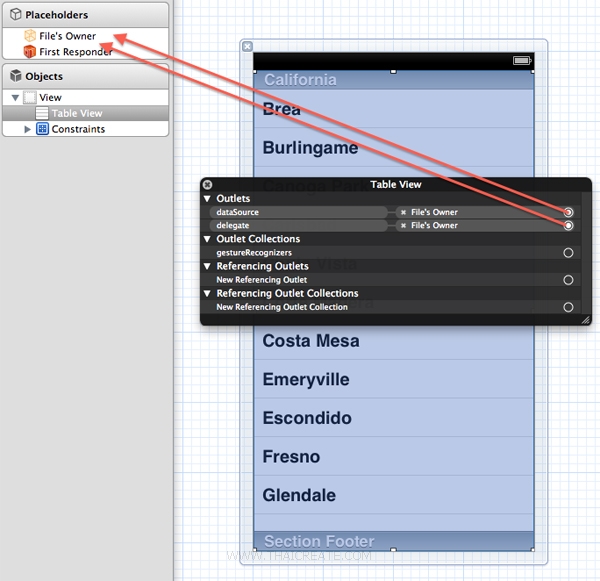
คลิกวาที่ Table View ให้ทำการเชื่อม dataSource และ delegate กับ File's Owner
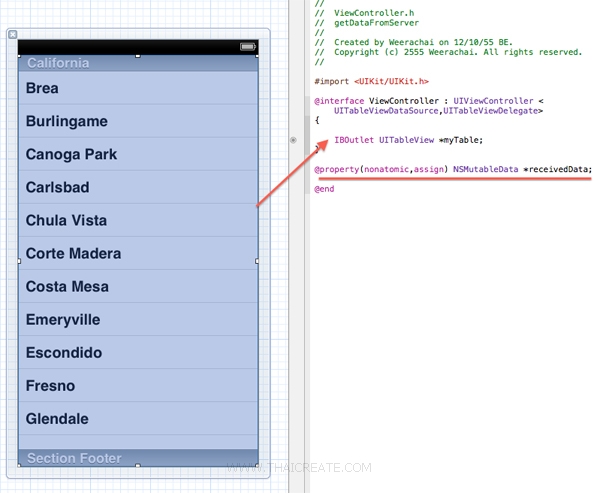
ใน Class ของ .h ให้เชื่อม IBOutlet ดังรูป จากนั้นเขียน Code ต่าง ๆ ดังนี้
ViewController.h
//
// ViewController.h
// getDataFromServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController <UITableViewDataSource,UITableViewDelegate>
{
IBOutlet UITableView *myTable;
}
@property(nonatomic,assign) NSMutableData *receivedData;
@end
ViewController.m
//
// ViewController.m
// getDataFromServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
{
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *memberid;
NSString *name;
NSString *tel;
UIAlertView *loading;
}
@end
@implementation ViewController
@synthesize receivedData;
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Define keys
memberid = @"MemberID";
name = @"Name";
tel = @"Tel";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSURLRequest *theRequest =
[NSURLRequest requestWithURL:[NSURL URLWithString:@"https://www.thaicreate.com/url/getData.php"]
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:theRequest delegate:self];
// Loading...
[UIApplication sharedApplication].networkActivityIndicatorVisible = YES;
loading = [[UIAlertView alloc] initWithTitle:@"" message:@"Please Wait..." delegate:nil cancelButtonTitle:nil otherButtonTitles:nil];
UIActivityIndicatorView *progress= [[UIActivityIndicatorView alloc] initWithFrame:CGRectMake(125, 50, 30, 30)];
progress.activityIndicatorViewStyle = UIActivityIndicatorViewStyleWhiteLarge;
[loading addSubview:progress];
[progress startAnimating];
[progress release];
[loading show];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
sleep(2);
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
[connection release];
[receivedData release];
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
[didFailWithErrorMessage release];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
[UIApplication sharedApplication].networkActivityIndicatorVisible = NO;
[loading dismissWithClickedButtonIndex:0 animated:YES];
if(receivedData)
{
//NSLog(@"%@",receivedData);
//NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
//NSLog(@" abc = %@",dataString);
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strMemberID, memberid,
strName, name,
strTel, tel,
nil];
[myObject addObject:dict];
}
[myTable reloadData];
}
// release the connection, and the data object
[connection release];
[receivedData release];
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
int nbCount = [myObject count];
if (nbCount == 0)
return 1;
else
return [myObject count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
int nbCount = [myObject count];
if (nbCount ==0)
cell.textLabel.text = @"Loading Data";
else
{
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
// MemberID
NSMutableString *text;
text = [NSString stringWithFormat:@"MemberID : %@",[tmpDict objectForKey:memberid]];
// Name & Tel
NSMutableString *detail;
detail = [NSString stringWithFormat:@"Name : %@ , Tel : %@"
,[tmpDict objectForKey:name]
,[tmpDict objectForKey:tel]];
cell.textLabel.text = text;
cell.detailTextLabel.text= detail;
//[tmpDict objectForKey:memberid]
//[tmpDict objectForKey:name]
//[tmpDict objectForKey:tel]
}
return cell;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[myTable release];
[super dealloc];
}
@end
Screenshot
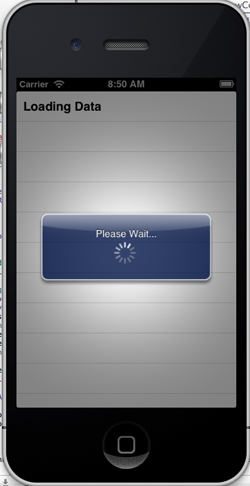
แสดงสถานะการทำทำงานด้วย Progress แบบ Activity Indicator View และ AlertView (UIAlertView / UIActivityIndicatorView)
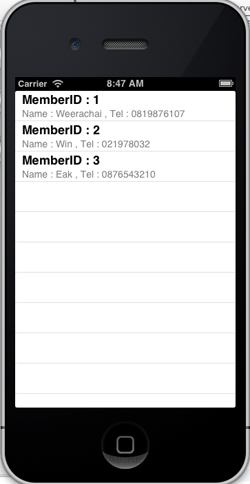
แสดงข้อมูลบน Table View
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-12-13 12:26:45 /
2017-03-26 08:55:18 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|