iOS/iPhone Search Data from Web Server (URL,Website) |
iOS/iPhone Search Data from Web Server (URL,Website) บทความนี้จะเรียนรู้วิธีการ Search หรือค้นหาข้อมูลที่อยู่บน Web Server โดยใช้ NSURLConnection ส่ง Keyword ที่ต้องการค้นหา ผ่าน POST ไปยัง URL ของ Web Server ที่ทำหน้าที่โดย PHP กับ MySQL และหลังจากที่ PHP รับค่า POST แล้วทำการค้นหาข้อมูลออกมาได้แล้ว ก็จะส่งค่ากลับมายัง Client ในรูปแบบของ JSON Data
iOS/iPhone Search Data from Web Server (URL,Website)
วิธีการแบบนี้จะดีกว่าการโหลดข้อมูลมาทั้งหมดแล้วค่อยใช้ Search Bar เพราะเมื่อข้อมูลมีปริมาณมาก หลายพันหรือหมื่น Record การที่จะโหลดข้อมูลมาทั้งหมดแล้วใช้ Search Bar ในการ Filter นั้จะทำให้มีการใช้ Memory ในการจัดเก็บตัวแปรในรุปแบบของ Object เช่น Array นั้นสูงมาก แต่วิธีนี้จะใช้การส่งเฉพาะ Result ที่ค้นหาได้จริง ๆ เท่านั้น
iOS/iPhone Search Bar (UISearchBar) and Table View (UITableView)
iOS/iPhone Search Bar (UISearchBar) Data from Web Server Using JSON
Example การทำระบบ Search ค้นหาข้อมูลจาก Web Server (URL)
MySQL Database
CREATE TABLE `member` (
`MemberID` int(11) NOT NULL auto_increment,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
PRIMARY KEY (`MemberID`)
) ENGINE=MyISAM AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'Weerachai', '0819876107');
INSERT INTO `member` VALUES (2, 'Win', '021978032');
INSERT INTO `member` VALUES (3, 'Eak', '0876543210');
โครงสร้างของ MySQL Database
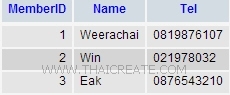
searchData.php
<?php
$strKeyword = $_POST["keyword"];
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE Name LIKE '%".$strKeyword."%' ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
ไฟล์ php ที่รับค่า POST แล้วค้นหาใน MySQL Database
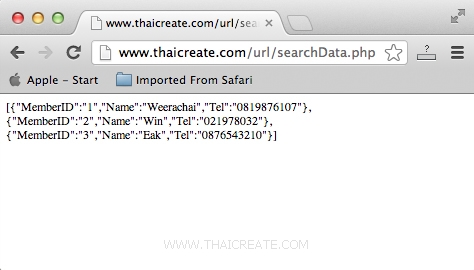
ทดสอบเรียก php ผ่าน URL โดยไม่ได้ทำการ POST ก็จะแสดงข้อมูลทั้งหมด
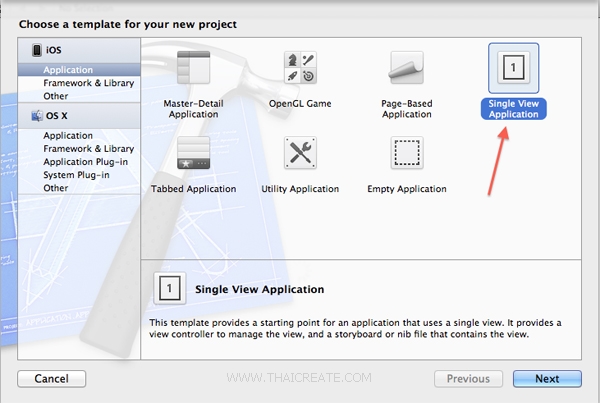
สร้าง Project บน Xcode เลือกแบบ Single View Application
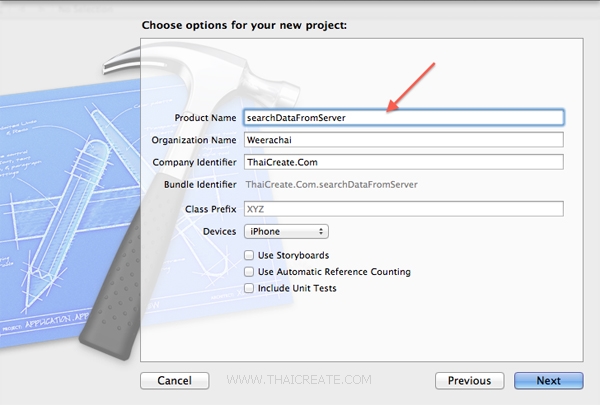
เลือกและไม่เลือกรายการดังรูป
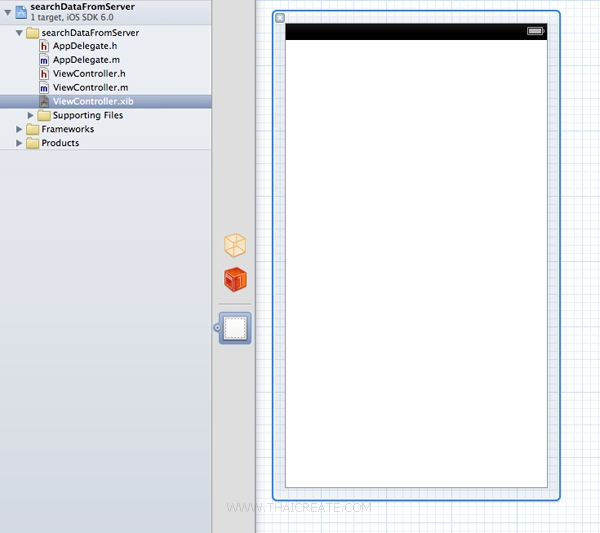
ตอนนี้หน้าจอจะยังว่าง ๆ
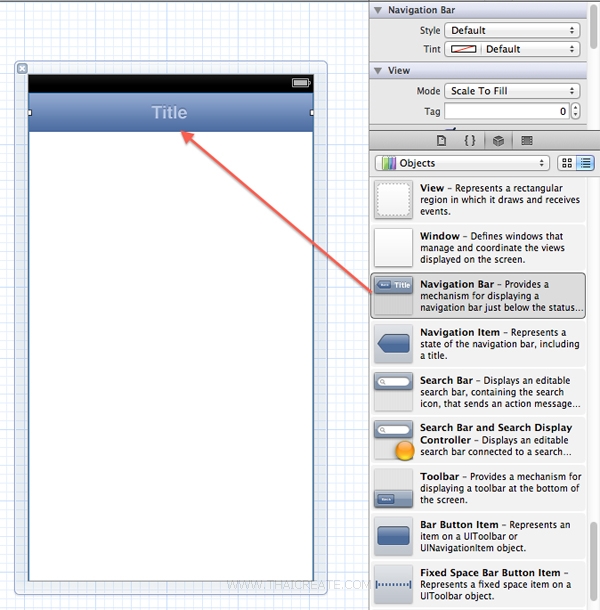
ลาก Navigation Bar มาวางไว้ในหน้าจอ View
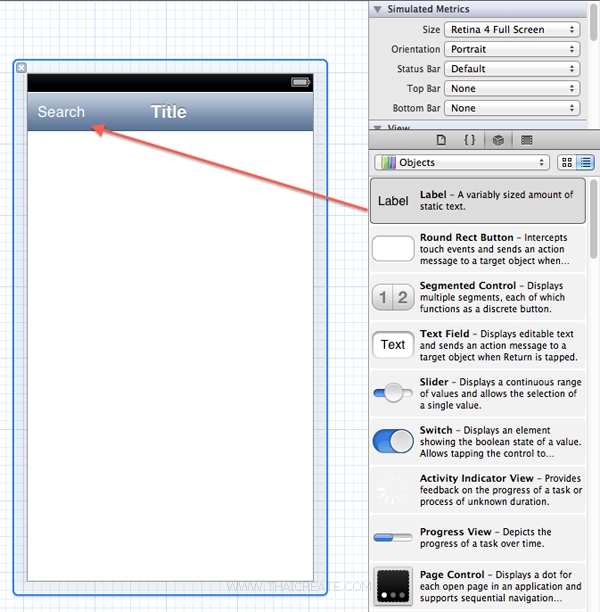
ใส่ Label บน Navigation Bar
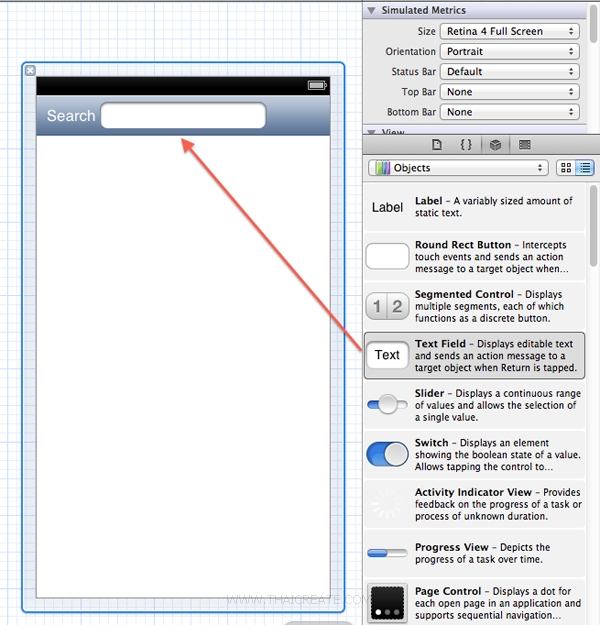
ลาก Text Fields มาวางไว้บน Navigation Bar
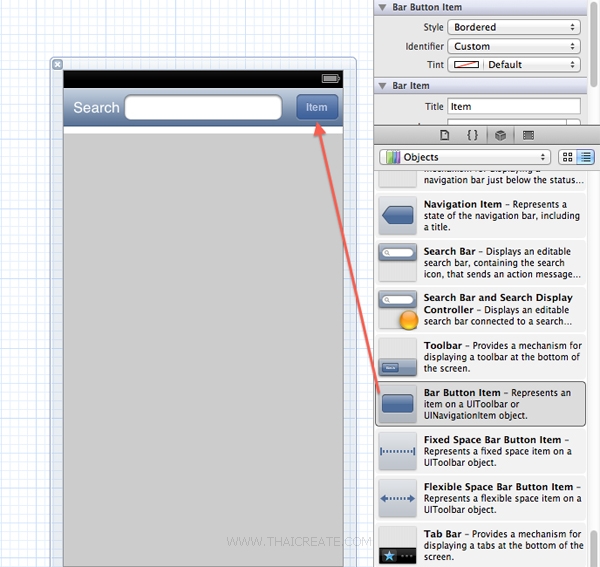
ตามด้วย Bar Button Item
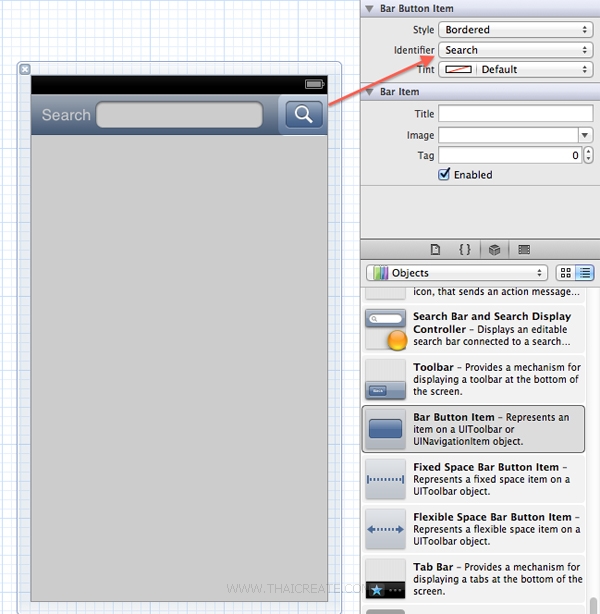
เปลี่ยนให้เป็น Icons ของการ Search
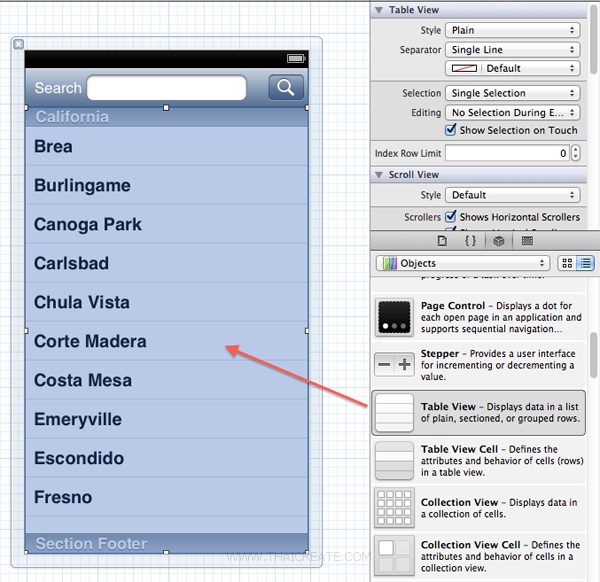
ลาก Table View มาวางไว้ในหน้าจอ View
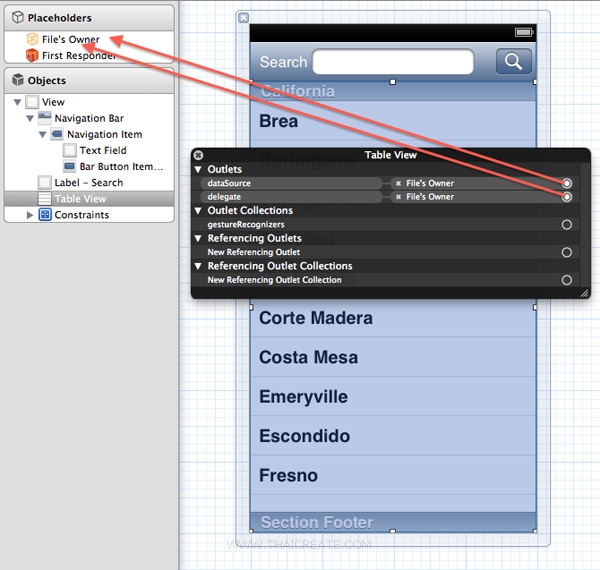
คลิกขวาที่ Table View ให้เชื่อม dataSource และ delegate กับ File's Owner
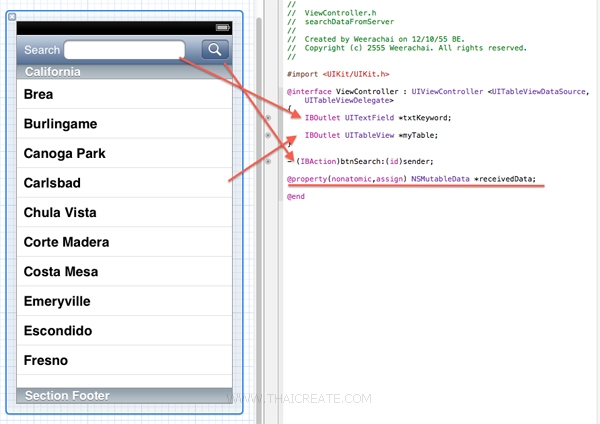
ใน Class ของ .h ให้เชื่อม IBOutlet และ IBAction ดังรูป จากนั้นเขียน Code ทั้งหมดดังนี้
ViewController.h
//
// ViewController.h
// searchDataFromServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController <UITableViewDataSource,UITableViewDelegate>
{
IBOutlet UITextField *txtKeyword;
IBOutlet UITableView *myTable;
}
- (IBAction)btnSearch:(id)sender;
@property(nonatomic,assign) NSMutableData *receivedData;
@end
ViewController.m
//
// ViewController.m
// searchDataFromServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
{
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *memberid;
NSString *name;
NSString *tel;
UIAlertView *loading;
}
@end
@implementation ViewController
@synthesize receivedData;
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Define keys
memberid = @"MemberID";
name = @"Name";
tel = @"Tel";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
}
- (IBAction)btnSearch:(id)sender {
//Keyword=abc
NSMutableString *post = [NSString stringWithFormat:@"keyword=%@",[txtKeyword text]];
NSData *postData = [post dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%d", [postData length]];
NSURL *url = [NSURL URLWithString:@"https://www.thaicreate.com/url/searchData.php"];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-Length"];
[request setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
[request setHTTPBody:postData];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:request delegate:self];
// Show Progress Loading...
[UIApplication sharedApplication].networkActivityIndicatorVisible = YES;
loading = [[UIAlertView alloc] initWithTitle:@"" message:@"Please Wait..." delegate:nil cancelButtonTitle:nil otherButtonTitles:nil];
UIActivityIndicatorView *progress= [[UIActivityIndicatorView alloc] initWithFrame:CGRectMake(125, 50, 30, 30)];
progress.activityIndicatorViewStyle = UIActivityIndicatorViewStyleWhiteLarge;
[loading addSubview:progress];
[progress startAnimating];
[progress release];
[loading show];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
[myTable reloadData];
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
sleep(2);
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
[connection release];
[receivedData release];
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
[didFailWithErrorMessage release];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
// Hide Progress
[UIApplication sharedApplication].networkActivityIndicatorVisible = NO;
[loading dismissWithClickedButtonIndex:0 animated:YES];
// Clear Object
[myObject removeAllObjects];
if(receivedData)
{
//NSLog(@"%@",receivedData);
//NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
//NSLog(@" abc = %@",dataString);
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strMemberID, memberid,
strName, name,
strTel, tel,
nil];
[myObject addObject:dict];
}
[myTable reloadData];
}
// release the connection, and the data object
[connection release];
[receivedData release];
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
int nbCount = [myObject count];
if (nbCount == 0)
return 1;
else
return [myObject count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
int nbCount = [myObject count];
if (nbCount ==0)
cell.textLabel.text = @"";
else
{
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
// MemberID
NSMutableString *text;
text = [NSString stringWithFormat:@"MemberID : %@",[tmpDict objectForKey:memberid]];
// Name & Tel
NSMutableString *detail;
detail = [NSString stringWithFormat:@"Name : %@ , Tel : %@"
,[tmpDict objectForKey:name]
,[tmpDict objectForKey:tel]];
cell.textLabel.text = text;
cell.detailTextLabel.text= detail;
//[tmpDict objectForKey:memberid]
//[tmpDict objectForKey:name]
//[tmpDict objectForKey:tel]
}
return cell;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[txtKeyword release];
[myTable release];
[super dealloc];
}
@end
Screenshot
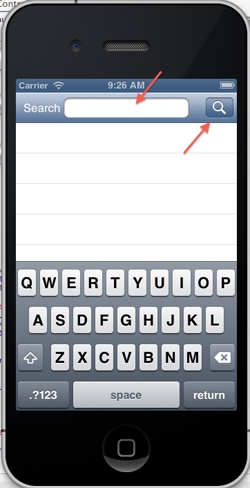
ช่องกรอกข้อมูล และ Button สำหรับค้นหา
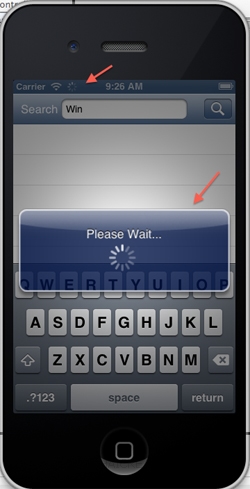
กรอก Keyword และทำการค้นหา ซึ่งจะแสดง Progress แบบ Icons หมุ่น ๆ
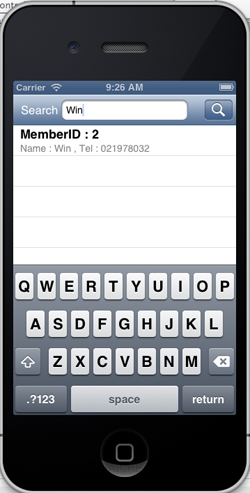
แสดงข้อมูลที่ค้นหาจาก Web Server
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-12-13 12:27:02 /
2017-03-26 08:54:31 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|