iOS/iPhone Storyboard and TableView Master-Detail (Objective-C,iPhone,iPad) |
iOS/iPhone Storyboard and TableView Master-Detail (Objective-C,iPhone,iPad) การทำ Master-Detail บน Storyboard ที่ประกอบด้วย 2 View โดย View แรกเปรียบเสมือน Master ที่แสดงข้อมูลบน Table View และ View สองเปรียบเหมือน Detail ที่ทำหน้าที่แสดงรายละเอียดต่าง ๆ ที่ได้จากมาจาก Master หลัก ๆ แล้วในตัวอย่างนี้จะอ้างอิงจากบทความก่อน ๆ ที่ได้แนะนำไว้ โดยจะประกอบด้วย Table View , Storyboard , Navigation Controller และการรับส่งค่า
iOS/iPhone Storyboard and TableView Master-Detail
และเพื่อความเข้าใจพื้นฐานเกี่ยวกับการสร้าง Table View บน Storyboard แนะนำให้อ่านและทำความเข้าใจกับบทความเหล่านี้ซะก่อน แต่ถ้ามีพื้นฐานมาแล้วก็สามารถข้ามไปได้เลยครับ
ในการเขียน iOS อาจจะมีขั้นตอนที่ซับซ้อนกว่าการเขียนโปรแกรมบน Windows Phone หรือ Android แต่ความซับซ้อนเหล่านี้ก็ช่วยให้การออกแบบหน้าจอ Interface ได้ง่ายและสะดวกยิ่งขึ้น ซึ่งอาจจะต้องทำความเข้าใจพื้นฐานจากบทความแรก ๆ ให้เข้าใจซะก่อน
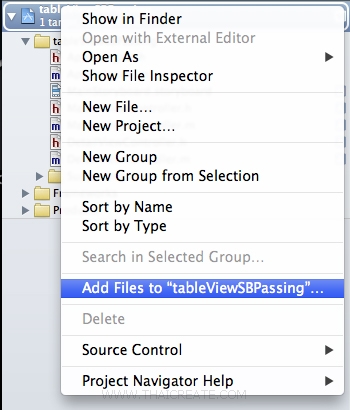
ขั้นแรกให้ Copy ไฟล์รูปภาพเข้ามาใน Project ซะก่อน
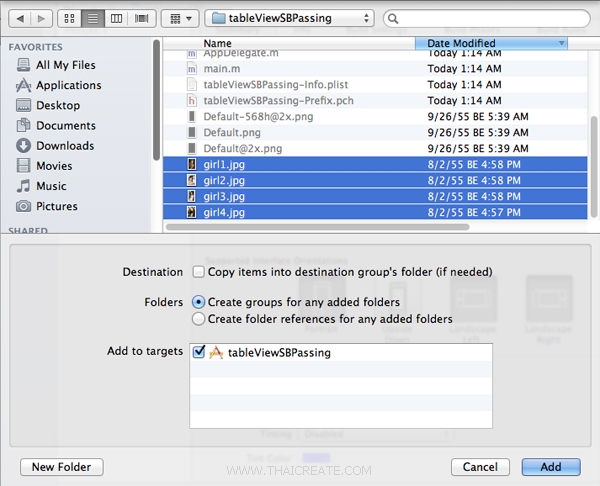
Add เข้ามาใน Project
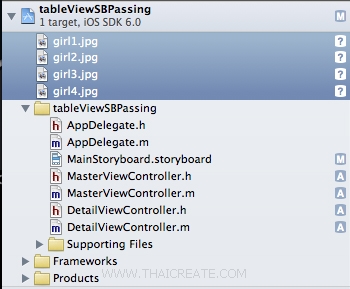
ได้ไฟล์เรียบร้อย
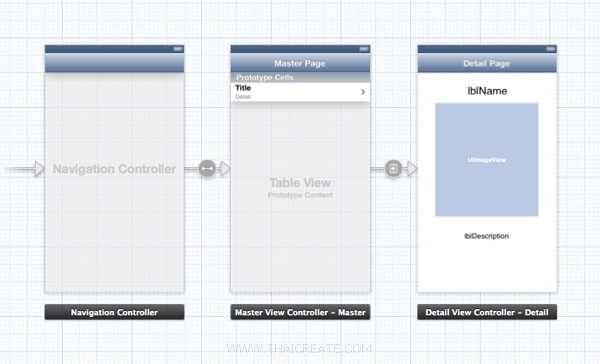
เราจะออกแบบ View บน Stroryboard ขึ้นมา 2 View โดยใช้ Navigation Controller และเชื่อม Action Segue แบบ Push สามารถอ่านเพิ่มเติมได้ที่บทความนี้
iOS/iPhone Storyboard and Passing Data Between View (Objective-C,iPhone)
โดยทำการเชื่อม Class ระหว่า View 1 กับ MasterViewController และ View 2 กับ DetailViewController

หน้าจอ View 1 ตรง Table Cell ให้เลือก Style และ Identifier (เลือกเป็น Cell) ดังรูป
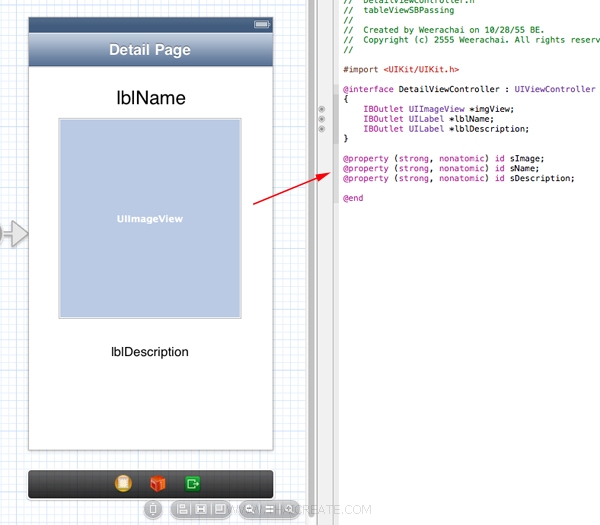
หน้าจอของ View 2 ใช้สำหรับแสดง Detail ให้ออกแบบและเชื่อม IBOutlet และ IBAction ดังรูป
ที่เหลือจะเป็น Code สำหรับ Class ทั้ง 4 ไฟล์
MasterViewController.h
//
// MasterViewController.h
//
// Created by Weerachai on 10/28/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface MasterViewController : UITableViewController
@end
MasterViewController.m
//
// MasterViewController.m
//
// Created by Weerachai on 10/28/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "MasterViewController.h"
#import "DetailViewController.h"
@interface MasterViewController ()
@end
@implementation MasterViewController
{
// A array Object
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *images;
NSString *name;
NSString *description;
}
- (id)initWithStyle:(UITableViewStyle)style
{
self = [super initWithStyle:style];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Define keys
images = @"Images";
name = @"Name";
description = @"Description";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
// Create three dictionaries
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"girl1.jpg", images,
@"Girl 1", name,
@"Girl 1 Description", description,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"girl2.jpg", images,
@"Girl 2", name,
@"Girl 2 Description", description,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"girl3.jpg", images,
@"Girl 3", name,
@"Girl 3 Description", description,
nil];
[myObject addObject:dict];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
@"girl4.jpg", images,
@"Girl 4", name,
@"Girl 4 Description", description,
nil];
[myObject addObject:dict];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
return myObject.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
UIImage* theImage = [UIImage imageNamed:[tmpDict objectForKey:images]];
cell.imageView.image = theImage;
cell.textLabel.text = [tmpDict objectForKey:name];
cell.detailTextLabel.text= [tmpDict objectForKey:description];
return cell;
}
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender
{
if ([[segue identifier] isEqualToString:@"showDetail"]) {
NSIndexPath *indexPath = [self.tableView indexPathForSelectedRow];
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
[[segue destinationViewController] setImageItem:[tmpDict objectForKey:images]];
[[segue destinationViewController] setNameItem:[tmpDict objectForKey:name]];
[[segue destinationViewController] setDescriptionItem:[tmpDict objectForKey:description]];
}
}
@end
DetailViewController.h
//
// DetailViewController.h
//
// Created by Weerachai on 10/28/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface DetailViewController : UIViewController
{
IBOutlet UIImageView *imgView;
IBOutlet UILabel *lblName;
IBOutlet UILabel *lblDescription;
}
@property (strong, nonatomic) id sImage;
@property (strong, nonatomic) id sName;
@property (strong, nonatomic) id sDescription;
- (void)setNameItem:(id)newName
- (void)setDescriptionItem:(id)newDescription
@end
DetailViewController.m
//
// DetailViewController.m
//
// Created by Weerachai on 10/28/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "DetailViewController.h"
@interface DetailViewController ()
@end
@implementation DetailViewController
@synthesize sImage;
@synthesize sName;
@synthesize sDescription;
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view.
UIImage* theImage = [UIImage imageNamed:[self.sImage description]];
imgView.image = theImage;
lblName.text = [sName description];
lblDescription.text = [sDescription description];
}
- (void)setImageItem:(id)newImage
{
sImage = newImage;
}
- (void)setNameItem:(id)newName
{
sName = newName;
}
- (void)setDescriptionItem:(id)newDescription
{
sDescription = newDescription;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[imgView release];
[lblName release];
[lblDescription release];
[super dealloc];
}
@end
Screenshot ทดสอบการทำงานบน iOS iPhone Simulator
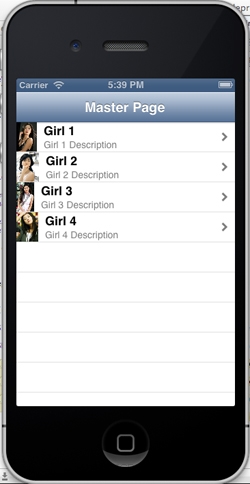
แสดง View แรก กับ Table View และ Navigation Controller
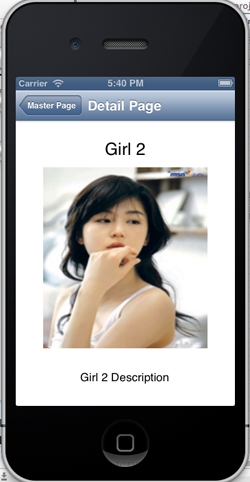
แสดงรายละเอียดจาก View แรก และ Navigation Controller สำหรับการ Back กลับไป
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-10-29 15:21:56 /
2017-03-26 09:49:57 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|