iOS/iPhone PHP/MySQL and JSON Parsing (Objective-C) |
iOS/iPhone PHP/MySQL and JSON Parsing (Objective-C) บทความนี้เป็นการเขียน iOS บน iPhone กับการแสดงข้อมูลจาก PHP และ MySQL Database ที่อยู่บน Web Server โดยการใช้ iOS ในการเรียกข้อมูลจาก URL ที่อยู่บน Web site และจะได้ข้อมูลในรูปแบบของ JSON กลับมา หลังจากได้ข้อมูลในรูปแบบของ JSON แล้ว iOS ซึ่งเขียนด้วยภาษา Objective-C จะทำการแปลงข้อมูลเหล่านั้นให้อยู่ในรูปแบบของ Array ที่จะสามารถนำไปแสดงผลบน Table View (UITableView) ได้
iOS/iPhone PHP/MySQL and JSON Parsing
สำหรับการเขียน iOS App ด้วย Objective-C การอ่านข้อมูลที่อยู่บน Web Server (PHP & MySQL) นั้นสามารถทำได้ง่าย ๆ ด้วยการใช้ dataWithContentsOfURL ทำการระบุ URL ปลายทางก็จะสามารถรับข้อมูลต่าง ๆ มาจัดเก็บไว้ที่ NSData ได้ในทันที และข้อมูลเหล่านั้นก็จะผ่านการ NSJSONSerialization ซึ่งจะได้ข้อมูลในรูปแบบของ Array ที่จะแสดงผลบน Table View ได้
Web Server โครงสร้าง PHP และ MySQL Database ที่อยู่บน Web Server
MySQL Database
CREATE TABLE `member` (
`MemberID` int(11) NOT NULL auto_increment,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
PRIMARY KEY (`MemberID`)
) ENGINE=MyISAM AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'Weerachai', '0819876107');
INSERT INTO `member` VALUES (2, 'Win', '021978032');
INSERT INTO `member` VALUES (3, 'Eak', '0876543210');
โครงสร้างของ MySQL Database ที่อยู่บน Web Server
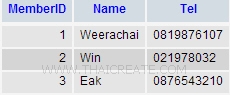
getJSON.php ไฟล์ PHP สำหรับอ่านข้อมูลจาก MySQL และส่งออกเป็น JSON
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
ไฟล์ PHP อ่านข้อมูลจาก MySQL Database ให้อยู่ในรูปแบบขแง JSON Code
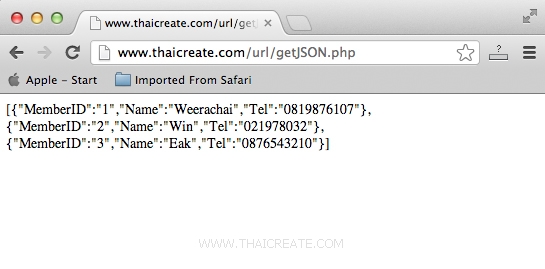
ไฟล์ PHP และ JSON ที่ได้เมื่อเรียกผ่าน Web Browser
จาก PHP และ MySQL Database และ JSON ทีได้ เราจะสามารถอ่าน JSON ให้อยู่ในรูปแบบของ NSMutableArray ที่จะใช้สำหรับ Table View ได้ดังนี้
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *memberid;
NSString *name;
NSString *tel;
// Define keys
memberid = @"MemberID";
name = @"Name";
tel = @"Tel";
// [{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSData *jsonData = [NSData dataWithContentsOfURL:
[NSURL URLWithString:@"https://www.thaicreate.com/url/getJSON.php"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strMemberID, memberid,
strName, name,
strTel, tel,
nil];
[myObject addObject:dict];
}
รูปแบบการอ่าน JSON และการแปลงค่า JSON ให้อยู่ในรูปแบบของ NSMutableArray ที่จะใช้แสดงผลบน Table View ลองมาดูซะตัวอย่าง
iOS/iPhone TableView and UITableView (Objective-C, iPhone, iPad)
Example การอ่าน JSON จาก PHP และ MySQL Database และแสดงผลบน Table View (UITableView)
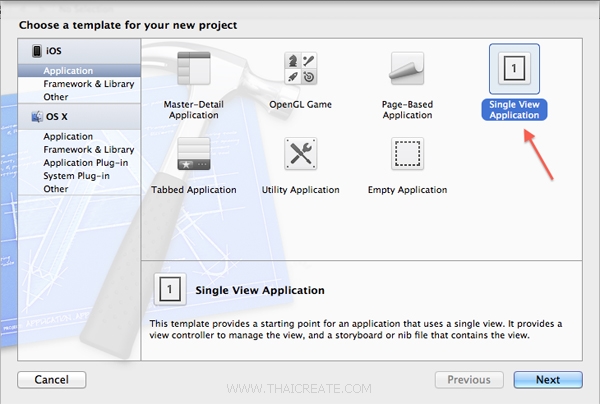
เริ่มต้นการสร้าง Application แบบง่าย ๆ ด้วย Single View Application
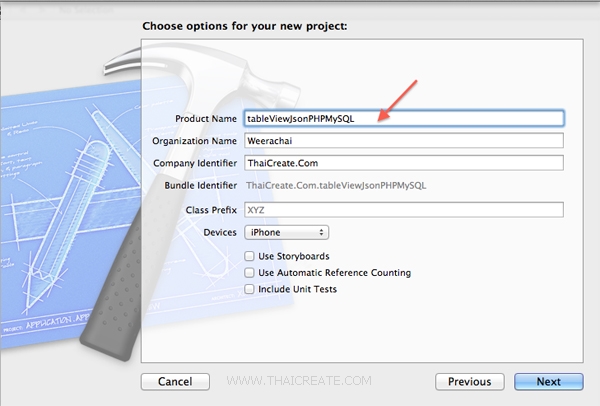
เลือกและไม่เลือกรายการดังรูป
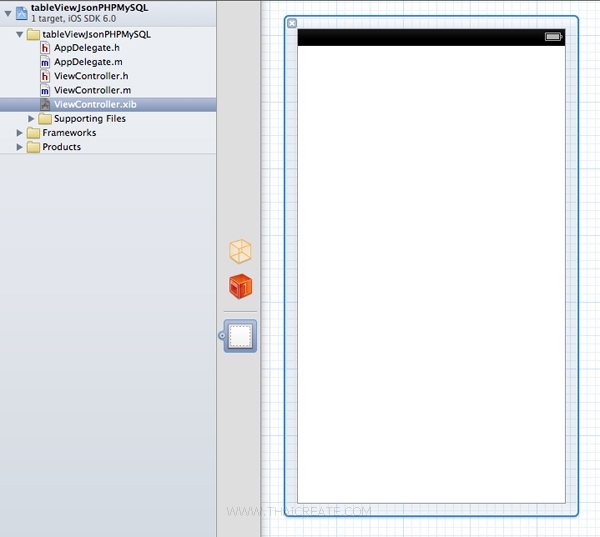
ตอนนี้หน้าจอ View จะยังว่าง ๆ
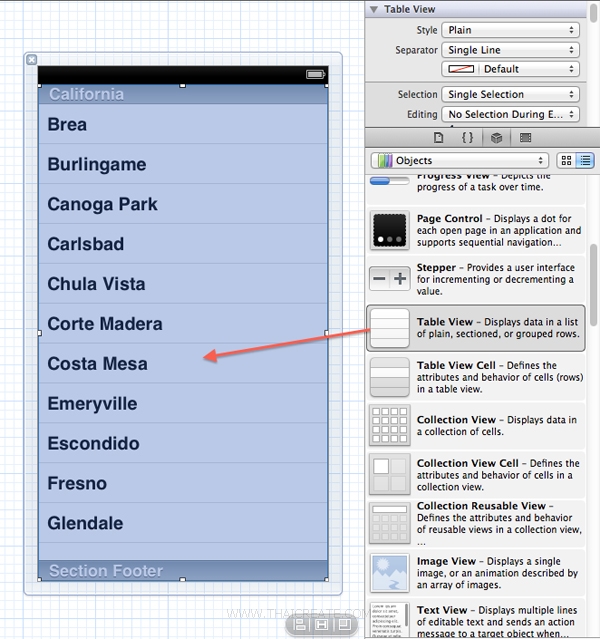
ทำการลาก Table View มาไว้ที่หน้าจอของ View
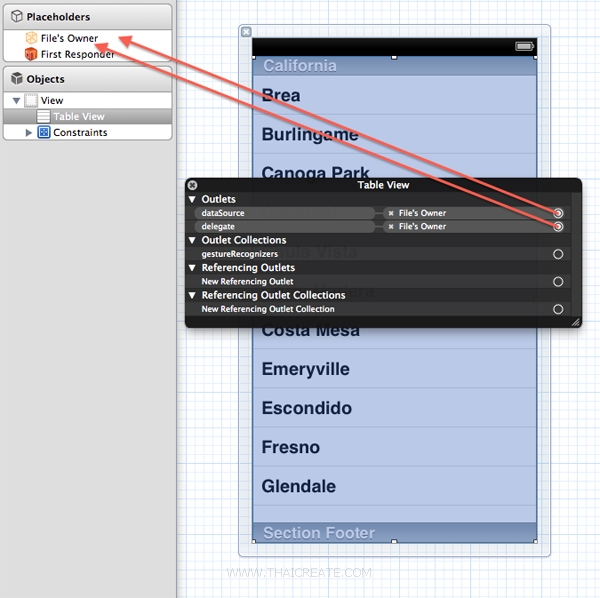
คลิกขวาที่ Table View ให้ทำการเชื่อม dataSource กับ delegate กับ File's Owner
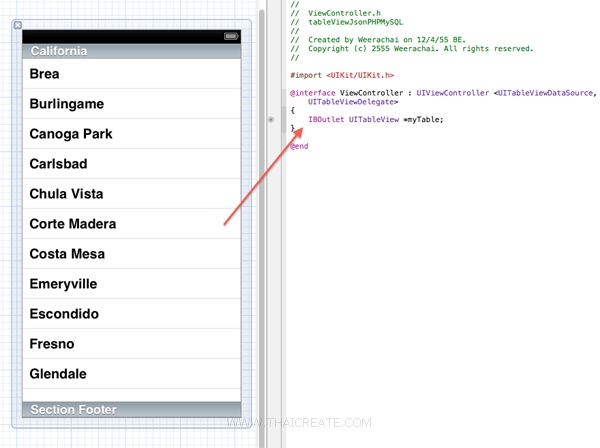
ใน Class ของ .h ให้เชื่อม IBOutlet ให้เรียบร้อย และอย่าลืมใส่ <UITableViewDataSource,UITableViewDelegate> ด้วย จากนั้น Code ทั้งหมดเป็นดังนี้
ViewController.h
//
// ViewController.h
// tableViewJsonPHPMySQL
//
// Created by Weerachai on 12/4/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController <UITableViewDataSource,UITableViewDelegate>
{
IBOutlet UITableView *myTable;
}
@end
ViewController.m
//
// ViewController.m
// tableViewJsonPHPMySQL
//
// Created by Weerachai on 12/4/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
{
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *memberid;
NSString *name;
NSString *tel;
}
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Define keys
memberid = @"MemberID";
name = @"Name";
tel = @"Tel";
// [{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSData *jsonData = [NSData dataWithContentsOfURL:
[NSURL URLWithString:@"https://www.thaicreate.com/url/getJSON.php"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strMemberID, memberid,
strName, name,
strTel, tel,
nil];
[myObject addObject:dict];
}
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return myObject.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
// MemberID
NSMutableString *text;
text = [NSString stringWithFormat:@"MemberID : %@",[tmpDict objectForKey:memberid]];
// Name & Tel
NSMutableString *detail;
detail = [NSString stringWithFormat:@"Name : %@ , Tel : %@"
,[tmpDict objectForKey:name]
,[tmpDict objectForKey:tel]];
cell.textLabel.text = text;
cell.detailTextLabel.text= detail;
//[tmpDict objectForKey:memberid]
//[tmpDict objectForKey:name]
//[tmpDict objectForKey:tel]
return cell;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[myTable release];
[super dealloc];
}
@end
Screenshot
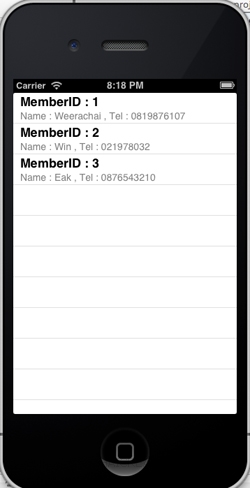
แสดงข้อมูลจาก JSON จาก PHP และ MySQL Database ที่อยู่บน Web Server ด้วย Object ของ Table View (UITableView)
เพิ่มเติม
จากตัวอย่างนี้เป็นการแสดงข้อมูลบน Table View แบบง่าย ๆ และไม่มีอะไรซับซ้อน และสำหรับคำอธิบายสามารถอ่านได้จากพื้นฐานของบทความ Table View และในกรณีที่ข้อมูลมีความซับซ้อน หรือ การจัดรูปแบบ Cell ตามต้องการ ก็สามารถอ่านได้จากบทความของ Table View Custom Cell ตามลิงค์นี้
iOS/iPhone TableView and UITableView (Objective-C, iPhone, iPad)
iOS/iPhone Table View and Table View Cell - Custom Cell Column (iPhone, iPad)
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2012-12-06 12:08:42 /
2017-03-26 09:04:44 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|