iOS/iPhone Table View(UITableView) Sections from an NSArray/NSMutableArray/NSDictionary (Objective-C,iPhone, iPad) |
iOS/iPhone Table View(UITableView) Sections from an NSArray/NSMutableArray/NSDictionary (Objective-C,iPhone, iPad) บทความกน่อนหน้านี้เราได้รู้วิธีการสร้าง Table View และ Section แบบ Static Cell แล้ว และบทความนี้เรามารู้วิธีการสร้างแบบ Dynamic Cell ที่ดึงและอ่านข้อมูลต่าง ๆ มาจาก Array จากนั้นก็ทำการแสดงผลตาม Section ของข้อมูลที่อยู่ในรูปแบบตามที่กำหนดขึ้น
iOS/iPhone Table View(UITableView) Sections from an NSArray/NSMutableArray/NSDictionary
และเพื่อให้ได้ข้อมูลที่อยุ่ในรุปแบบเป็นแบบ Array ที่ประกอบด้วยหลาย Key และ Value เราจะต้องใช้ NSMutableArray ทำงานรวมกับ NSDictionary ซึ่งภายในแต่ล่ะ Index ของ NSMutableArray ก็จะประกอบด้วย NSDictionary ที่ประกอบด้วย Key และ Value ต่าง ๆ ตามตัวอย่าง
//Initialize the array.
listOfItems = [[NSMutableArray alloc] init];
// Section Group 1
NSArray *arrGroup1 = [NSArray arrayWithObjects:@"Item 1", @"Item 2", @"Item 3", @"Item 4", nil];
NSDictionary *dictGroup1 = [NSDictionary dictionaryWithObject:arrGroup1 forKey:@"GroupItem"];
// Section Group 2
NSArray *arrGroup2 = [NSArray arrayWithObjects:@"Item 5", @"Item 6", @"Item 7", @"Item 8", nil];
NSDictionary *dictGroup2 = [NSDictionary dictionaryWithObject:arrGroup2 forKey:@"GroupItem"];
[listOfItems addObject:dictGroup1];
[listOfItems addObject:dictGroup2];
เริ่มต้นการสร้าง Project บน Xcode เพื่อสร้าง Dynamic Cell แบบ Sections
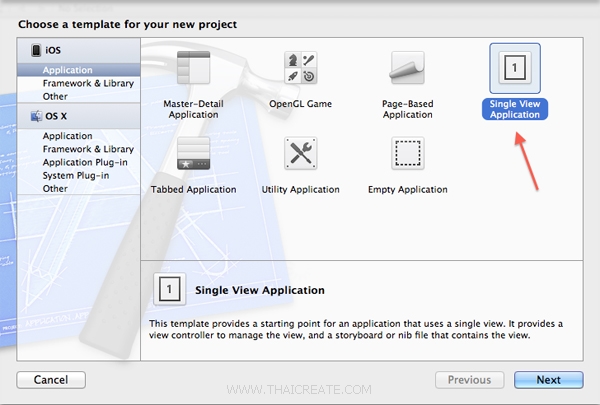
สร้าง Application แบบ Single View Application
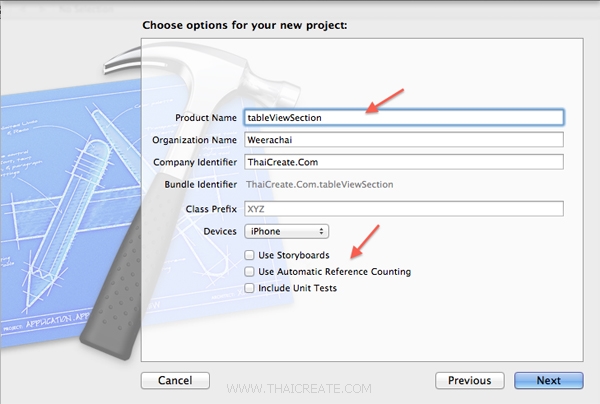
เลือกและไม่เลือกรายการดังรูป
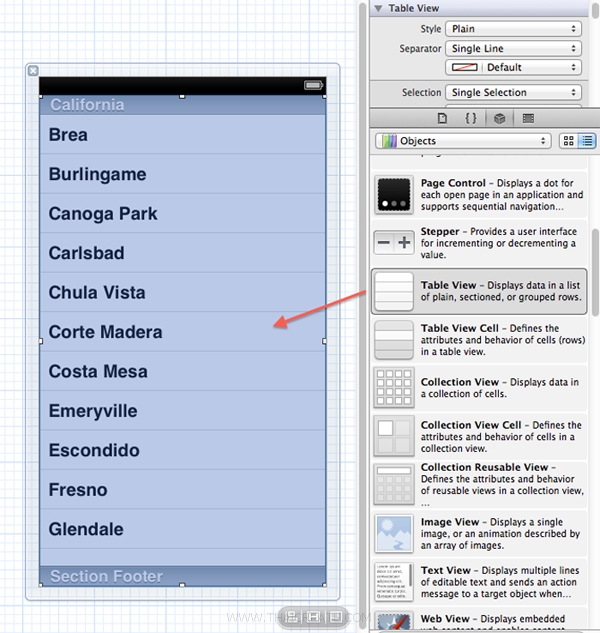
หลังจากนั้นเราจะได้ View บนไฟล์ .xib ที่ยังเป็นหน้าจอขาว ๆ ว่างเปล่า ให้ลาก Table View มายังวางไว้ที่ View หลัก
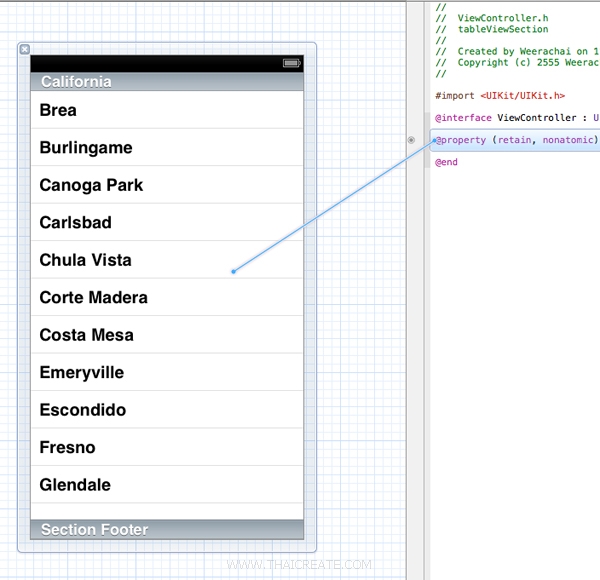
สร้าง IBOutlet ให้กับ Table View
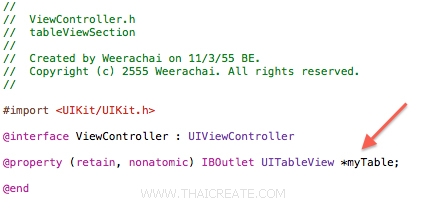
ได้ IBOutlet ดังรูป
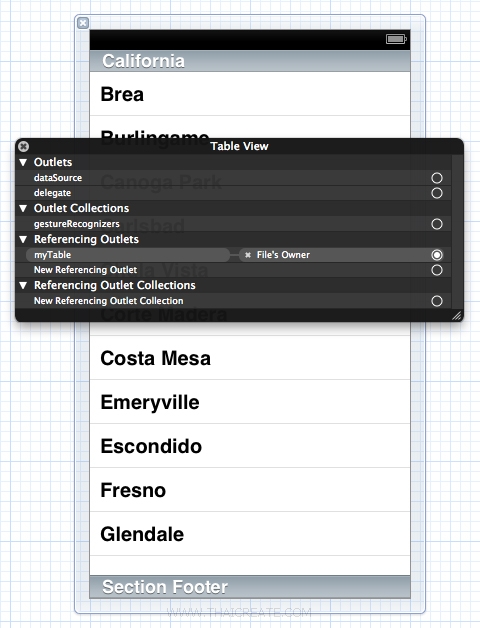
คลิกขวาที่ Table View จะได้หน้าจอดังรูป

ทำการลาก dataSource ไปยังตำแหน่งของ File's Owner
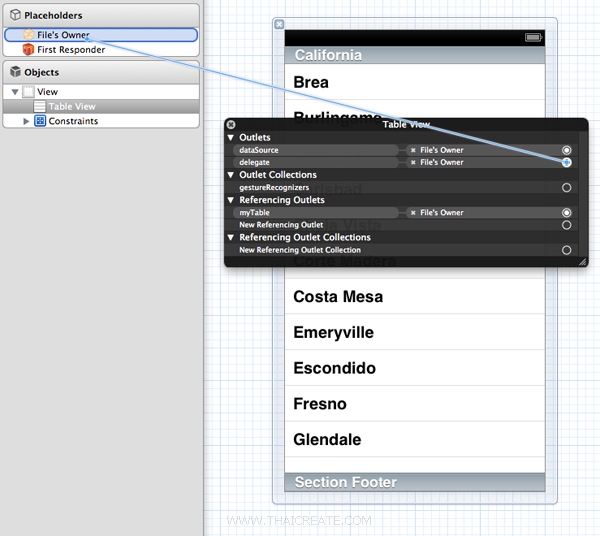
เชื่อม delegate ด้วย
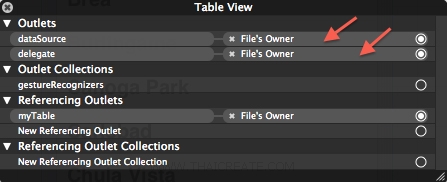
จะได้ผลลัพธ์ดังรูป จากนั้นจะเป้นส่วนของ Coding ด้วยภาษา Objective-C
ViewController.h
//
// ViewController.h
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
@property (retain, nonatomic) IBOutlet UITableView *myTable;
@end
ViewController.m
//
// ViewController.m
//
// Created by Weerachai on 11/3/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
{
NSMutableArray *listOfItems;
}
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
//Initialize the array.
listOfItems = [[NSMutableArray alloc] init];
// Section Group 1
NSArray *arrGroup1 = [NSArray arrayWithObjects:@"Item 1", @"Item 2", @"Item 3", @"Item 4", nil];
NSDictionary *dictGroup1 = [NSDictionary dictionaryWithObject:arrGroup1 forKey:@"GroupItem"];
// Section Group 2
NSArray *arrGroup2 = [NSArray arrayWithObjects:@"Item 5", @"Item 6", @"Item 7", @"Item 8", nil];
NSDictionary *dictGroup2 = [NSDictionary dictionaryWithObject:arrGroup2 forKey:@"GroupItem"];
[listOfItems addObject:dictGroup1];
[listOfItems addObject:dictGroup2];
//Set Title
self.navigationItem.title = @"Group Option Item";
}
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
return [listOfItems count];
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
NSDictionary *dictionary = [listOfItems objectAtIndex:section];
NSArray *array = [dictionary objectForKey:@"GroupItem"];
return [array count];
}
- (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section {
if(section == 0)
return @"Group 1";
else
return @"Group 2";
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSDictionary *dictionary = [listOfItems objectAtIndex:indexPath.section];
NSArray *array = [dictionary objectForKey:@"GroupItem"];
NSString *cellValue = [array objectAtIndex:indexPath.row];
cell.textLabel.text = cellValue;
return cell;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[_myTable release];
[super dealloc];
}
@end
สำหรับ Code ลองไล่ดูครับ ไม่ยากอะไรมากมาย

Screenshot
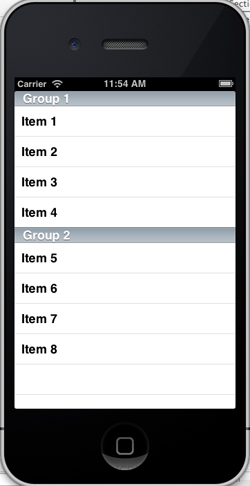
ได้ Table View ที่แบางตาม Section ดังรูป
เพิ่มเติม
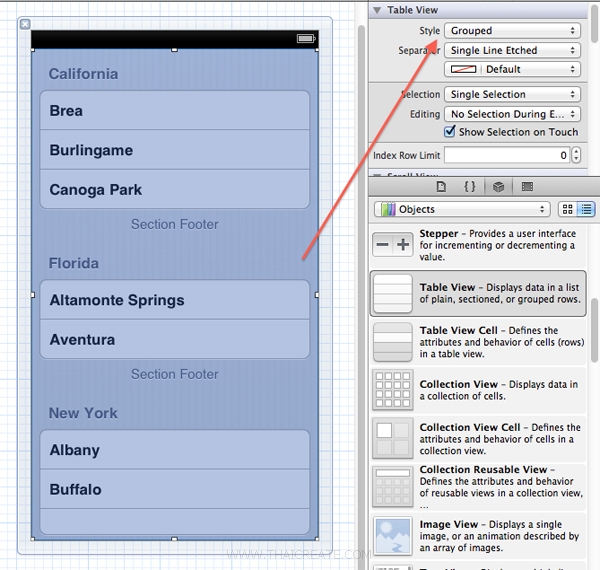
ลองเปลี่ยนมุมมอง Style ให้เป็นแบบ Grouped
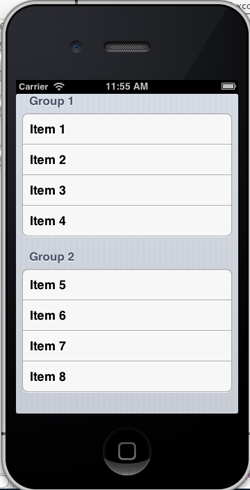
ได้ Sections ที่เป็นแบบ Group สวยงาม
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-11-07 10:36:27 /
2017-03-26 09:44:01 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|