ตอนที่ 7 : Show Case 3 : Update Data (iOS C# (Xamarin.iOS) and Mobile Services) |
ตอนที่ 7 : Show Case 3 : Update Data (iOS C# (Xamarin.iOS) and Mobile Services) บทความการเขียน iOS C# (Xamarin.iOS) ด้วย Tools ของ Visual Studio เพื่อทำการติดต่อกับ Azure Mobile Services และการเรียกข้อมูลจาก Mobile Services เพื่อ Update หรือแก้ไขข้อมูลในตาราง โดยหลักการก็คือจะเรียกข้อมูลจาก ID หรือ Key ที่กำหนด จากนั้นค่อยส่งค่าที่ต้องการ Update ไปยัง Table หรือตารางที่อยู่บน Mobile Services
iOS C# (Xamarin.iOS) Mobile Service Update Form
สำหรับขั้นตอนนั้นก็คือสร้าง Activity คือกำหนด ID หรือค่าที่ต้องการดึงข้อมูลที่อยู่บน Table จากนั้นดึงข้อมูลมาจาก Mobile Services มาแสดงบน Activity โดย Activity นี้จะมีหน้าที่ไปดึงพวกรายละเอียดต่าง ๆ มาแสดงใน EditText เพื่อรอทำการแก้ไข Update ข้อมูล
รูปแแบบการอ่านข้อมูลมาแสดงเพื่อรอการ Update
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
//*** Get Data Info ***//
var info = memberTable.Where(item => item.Username == strUsername).ToCollectionAsync();
if (info.Count > 0)
{
MyMember member = info[0];
txtUsername.Text = member.Username.ToString();
txtPassword.Text = member.Password.ToString();
txtName.Text = member.Name.ToString();
txtEmail.Text = member.Email.ToString();
txtTel.Text = member.Tel.ToString();
}
รูปแบบการ Update ข้อมูลและบันทึกไปยัง Mobile Services
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
//*** Get Data for Update ***//
var update = memberTable.Where(item => item.Username == strUsername).ToCollectionAsync();
if (update.Count > 0)
{
MyMember member = update[0];
member.Name = txtName.Text;
member.Password = txtPassword.Text;
member.Name = txtName.Text;
member.Email = txtEmail.Text;
member.Tel = txtTel.Text;
//*** Update Record ***/
memberTable.UpdateAsync(member);
new UIAlertView("Result", "Update Data Successfully.", null, "OK", null).Show();
}
Example ตัวอย่างการทำระบบแก้ไข Update Data ด้วย iOS C# (Xamarin.iOS)
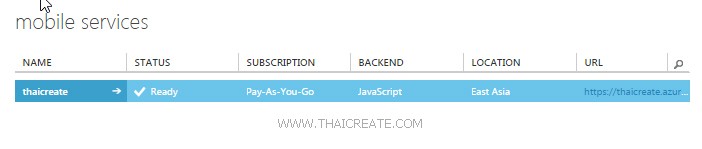
ตอนนี้เรามี Mobile Service อยู่ 1 รายการ
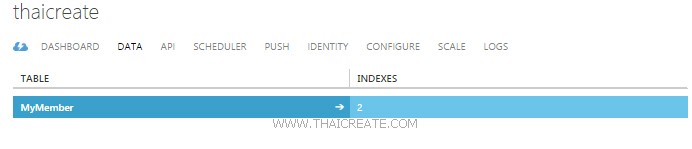
ชื่อตารางว่า MyMember
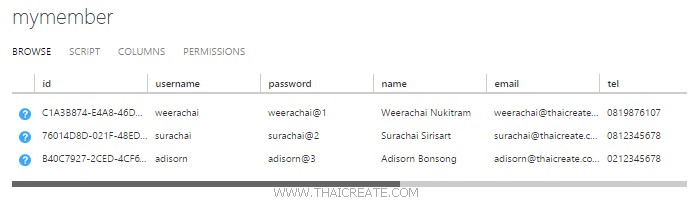
มีข้อมูลอยู่ทั้งหมด 3 รายการ
กลับมายัง iOS C# Project บน Visual Studio
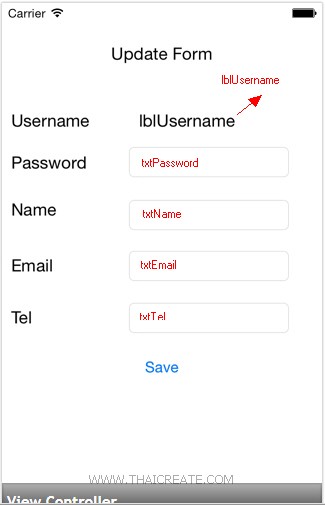
ออกแบบหน้าจอ View ประกอบด้วย Control ต่าง ๆ และกำหนนด ID/Name ดังรูป
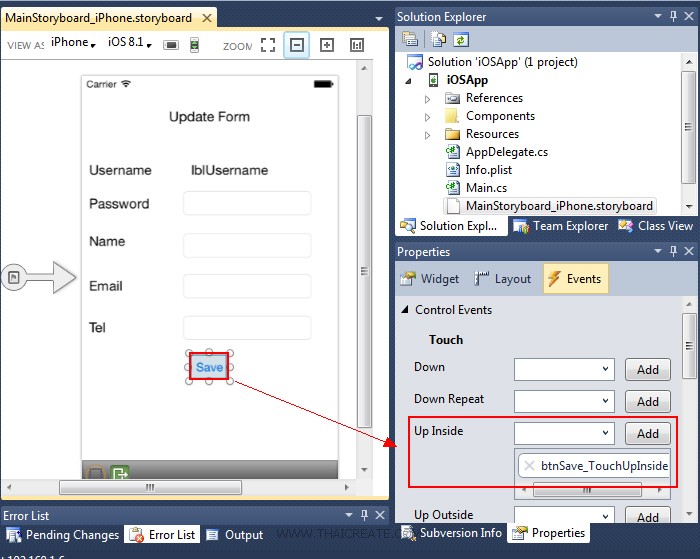
สร้าง Event ของ Button ดังรูป
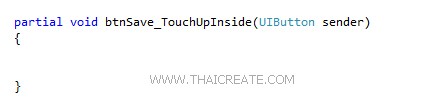
Event บน C# ที่ได้ โดยเราจะเขียนคำสั่งต่าง ๆ ลงใน Method นี้
RootViewController.cs (Code สำหรับการ Update)
using System;
using System.Drawing;
using System.Collections.Generic;
using MonoTouch.Foundation;
using MonoTouch.UIKit;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace iOSApp
{
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "username")]
public string Username { get; set; }
[JsonProperty(PropertyName = "password")]
public string Password { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
[JsonProperty(PropertyName = "tel")]
public string Tel { get; set; }
}
public partial class RootViewController : UIViewController
{
public const string ApplicationURL = @"https://thaicreate.azure-mobile.net/";
public const string ApplicationKey = @"IqeWShAjBflTUrTaaGUNJRyZDpcyeh72";
private MobileServiceClient client; // Mobile Service Client references
private IMobileServiceTable<MyMember> memberTable; // Mobile Service Table used to access data
string strUsername = "surachai";
public RootViewController(IntPtr handle)
: base(handle)
{
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.LoadInfo();
}
public override void DidReceiveMemoryWarning()
{
// Releases the view if it doesn't have a superview.
base.DidReceiveMemoryWarning();
}
private void LoadInfo()
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
//*** Get Data Info ***//
var info = memberTable.Where(item => item.Username == strUsername).ToCollectionAsync();
if (info.Count > 0)
{
MyMember member = info[0];
txtUsername.Text = member.Username.ToString();
txtPassword.Text = member.Password.ToString();
txtName.Text = member.Name.ToString();
txtEmail.Text = member.Email.ToString();
txtTel.Text = member.Tel.ToString();
}
}
partial void btnSave_TouchUpInside(UIButton sender)
{
try
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
//*** Get Data for Update ***//
var update = memberTable.Where(item => item.Username == strUsername).ToCollectionAsync();
if (update.Count > 0)
{
MyMember member = update[0];
member.Name = txtName.Text;
member.Password = txtPassword.Text;
member.Name = txtName.Text;
member.Email = txtEmail.Text;
member.Tel = txtTel.Text;
//*** Update Record ***/
memberTable.UpdateAsync(member);
new UIAlertView("Result", "Update Data Successfully.", null, "OK", null).Show();
}
}
catch (Exception ex)
{
new UIAlertView("Result", "Error : " + ex.Message, null, "OK", null).Show();
}
}
}
}
ในตัวอย่างที่ทดสอบดึงข้อมูล Username ของ surachai
string strUsername = "surachai";
ทดสอบการทำงาน
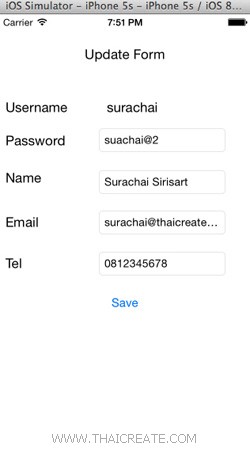
แสดงรายการสำหรับ Update
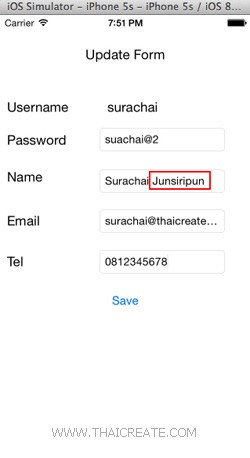
ทดสอบแก้ไขข้อมูล
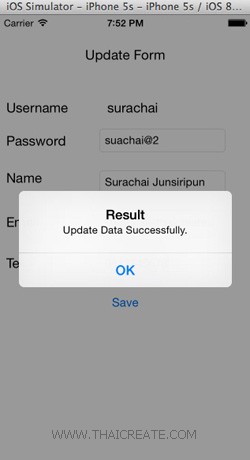
หลังจากที่แก้ไขข้อมูลเรียบร้อยแล้ว
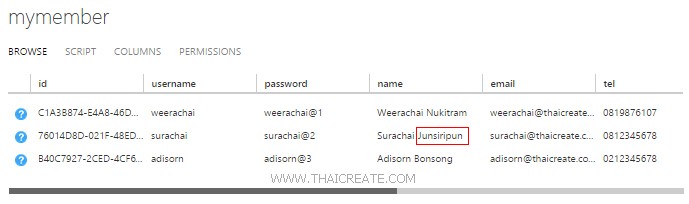
เมื่อกลับไปดู DATA ที่ Mobile Services ข้อมูลก็จะถูกแก้ไข Update
อ่านเพิ่มเติม
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-10-24 14:02:48 /
2017-03-26 08:46:21 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|