ตอนที่ 4 : iOS C# (Xamarin.iOS) อ่าน Data จาก Table ของ Mobile Services และแสดงผลบน App |
ตอนที่ 4 : iOS C# (Xamarin.iOS) อ่าน Data จาก Table ของ Mobile Services และแสดงผลบน App บทความนี้จะเป็นการใช้ iOS C# (Xamarin.iOS) อ่านข้อมูลจาก Table ของ Azure Mobile Services ที่อยู่บน Windows Azure โดยข้อมูลของ Table ได้ถูกจัดเก็บแบบ Column และ Rows คล้าย ๆ กับ Table ของ SQL Database ทั่ว ๆ ไป และใน iOS จะมีการใช้ UITableView ซึ่งจะแสดงข้อมูลที่อยู่ในรูปแบบของ TableSource ด้วยการ Loop ข้อมูลและนำข้อมูลที่ได้แสดงผลรายการใน UITableView
iOS C# (Xamarin.iOS) & Azure Mobile Services
รูปแบบการอ่านข้อมูลจาก Table ของ Mobile Services ด้วย iOS C#
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
var items = memberTable.ToListAsync();
Mapping ข้อมูลที่ได้กับ UITableView เพื่อแสดงข้อมูลบน Column ผ่าน TableSource
this.myTable.AutoresizingMask = UIViewAutoresizing.All;
this.myTable.Source = new TableSource(items);
การแสดงผลต่าง ๆ สามารถเขียนคำสั่งต่าง ๆ ได้ใน UITableViewSource ซึ่งจะ Delegate ตัว method ต่าง ๆ ที่จำเป็นดังนี้
public class TableSource : UITableViewSource
{
public TableSource(List<MyMember> items)
{
}
public override int NumberOfSections(UITableView tableView)
{
}
public override int RowsInSection(UITableView tableview, int section)
{
}
public override UITableViewCell GetCell(UITableView tableView, MonoTouch.Foundation.NSIndexPath indexPath)
{
}
}
รูปแบบการใช้ iOS C# (Xamarin.iOS) อ่านข้อมูลจาก Mobile Services บน Windows Azure
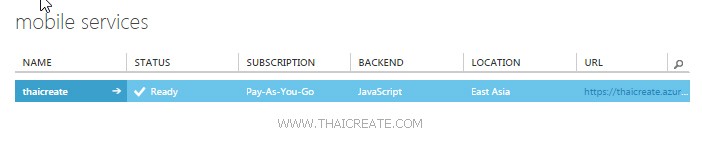
ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
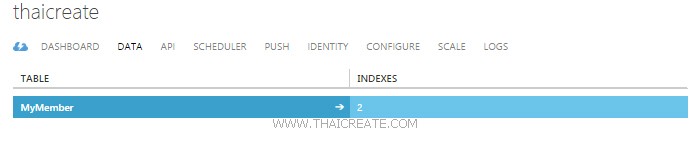
ชื่อตารางว่า MyMember
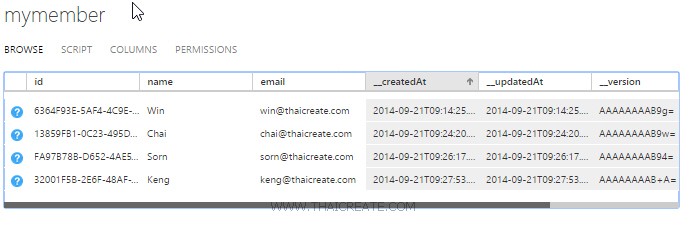
ข้อมูลในตาราง MyMember ประกอบด้วย Column ชื่อว่า id,name,email
กลับมายัง iOS C# โปรเจคบน Visual Studio
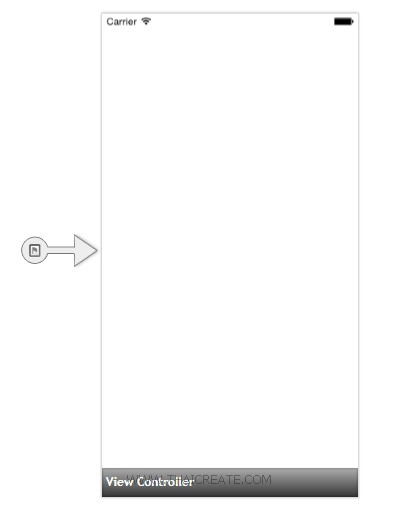
ตอนนี้เรามีไฟล์ View อยู่บน Storyboard จำนวน 1 View ซึ่ง View นี้ทำงานคู่กับ Class ที่มีชื่อว่า RootViewController.cs
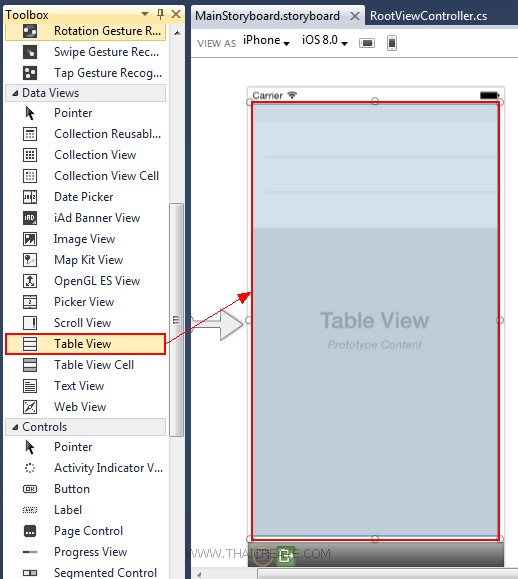
ลาก Control ของ Table View ไปไว้บนหน้าจอของ View
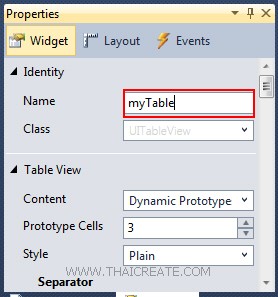
สร้าง ID/Name ว่า myTable จากนั้นเขียน Code ทั้งหมดดังนี้
RootViewController.cs
using System;
using System.Drawing;
using System.Collections.Generic;
using MonoTouch.Foundation;
using MonoTouch.UIKit;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace iOSApp
{
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
}
public partial class RootViewController : UIViewController
{
public const string ApplicationURL = @"https://thaicreate.azure-mobile.net/";
public const string ApplicationKey = @"IqeWShAjBflTUrTaaGUNJRyZDpcyeh72";
private MobileServiceClient client; // Mobile Service Client references
private IMobileServiceTable<MyMember> memberTable; // Mobile Service Table used to access data
public RootViewController(IntPtr handle)
: base(handle)
{
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
CreateTableItems();
}
protected void CreateTableItems()
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
var items = memberTable.ToListAsync();
this.myTable.AutoresizingMask = UIViewAutoresizing.All;
this.myTable.Source = new TableSource(items);
}
public override void DidReceiveMemoryWarning()
{
// Releases the view if it doesn't have a superview.
base.DidReceiveMemoryWarning();
}
}
public class TableSource : UITableViewSource
{
protected List<MyMember> tableItems;
protected string cellIdentifier = "TableCell";
public TableSource(List<MyMember> items)
{
tableItems = items;
}
/// <summary>
/// Called by the TableView to determine how many sections(groups) there are.
/// </summary>
public override int NumberOfSections(UITableView tableView)
{
return 1;
}
/// <summary>
/// Called by the TableView to determine how many cells to create for that particular section.
/// </summary>
public override int RowsInSection(UITableView tableview, int section)
{
return tableItems.Count;
}
/// <summary>
/// Called when a row is touched
/// </summary>
public override void RowSelected(UITableView tableView, NSIndexPath indexPath)
{
var item = tableItems[indexPath.Row];
new UIAlertView("Row Selected"
, item.Name, null, "OK", null).Show();
tableView.DeselectRow(indexPath, true);
}
/// <summary>
/// Called by the TableView to get the actual UITableViewCell to render for the particular row
/// </summary>
public override UITableViewCell GetCell(UITableView tableView, MonoTouch.Foundation.NSIndexPath indexPath)
{
UITableViewCell cell = tableView.DequeueReusableCell(cellIdentifier);
var item = tableItems[indexPath.Row];
//---- if there are no cells to reuse, create a new one
if (cell == null)
{ cell = new UITableViewCell(UITableViewCellStyle.Default, cellIdentifier); }
cell.TextLabel.Text = string.Format("{0} - {1}", item.Name, item.Email);
return cell;
}
}
}
ทดสอบการทำงาน
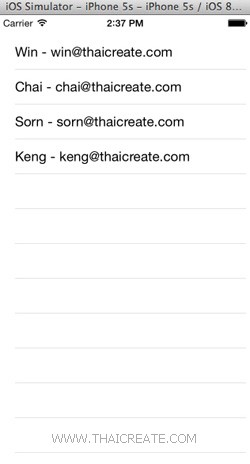
แสดงข้อมูลจาก Table ของ Mobile Services บน iOS App
อ่านเพิ่มเติม
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-10-24 14:01:52 /
2017-03-26 08:44:16 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|