Windows Phone Add Insert Data into Web Server (PHP and MySQL) |
Windows Phone Add Insert Data into Web Server (PHP and MySQL) ตัวอย่างการเขียน App บน Windows Phone ในการรับข้อมูลจากผู้ใช้ และนำข้อมูลที่ได้นั้นไปบันทึกและจัดเก็บไว้ที่ Web Server สำหรับวิธีนี้เราจะใช้ PHP ทำงานร่วมกับ MySQL โดยเราจะต้องออกเขียนโปรแกรม PHP ในฝั่ง Web Server ที่จะรอรับข้อมูลจาก Windows Phone ที่ทำหน้าที่เป็น Web Client ส่งค่าผ่าน URL และนำข้อมูลที่ได้นั้นไปจัดเก็บไว้ที่ Server พร้อมกับการแจ้งสถานะต่าง ๆ กลับมายัง Windows Phone ว่าข้อมูลที่ถูกส่งไปนั้นสามารถที่จะบันทึกได้หรือไม่
Windows Phone Add Insert Data into Web Server
Web Server ออกแบบโปรแกรมสำหรับ Insert ข้อมูลในฝั่งของ Web Server
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');
โครงสร้างตารางและข้อมูลบน Web Server จะใช้ PHP กับ MySQL

saveData.php
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
/*** for Sample
$_POST["sUsername"] = "a";
$_POST["sPassword"] = "b";
$_POST["sName"] = "c";
$_POST["sEmail"] = "d";
$_POST["sTel"] = "e";
*/
$strUsername = $_POST["sUsername"];
$strPassword = $_POST["sPassword"];
$strName = $_POST["sName"];
$strEmail = $_POST["sEmail"];
$strTel = $_POST["sTel"];
/*** Check Username Exists ***/
$strSQL = "SELECT * FROM member WHERE Username = '".$strUsername."' ";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
if($objResult)
{
echo "0|Username Exists!";
exit();
}
/*** Check Email Exists ***/
$strSQL = "SELECT * FROM member WHERE Email = '".$strEmail."' ";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
if($objResult)
{
echo "0|Email Exists!";
exit();
}
/*** Insert ***/
$strSQL = "INSERT INTO member (Username,Password,Name,Email,Tel)
VALUES (
'".$strUsername."',
'".$strPassword."',
'".$strName."',
'".$strEmail."',
'".$strTel."'
)
";
$objQuery = mysql_query($strSQL);
if(!$objQuery)
{
echo "0|Cannot save data!";
exit();
}
else
{
echo "1|";
exit();
}
/**
return
x|y
x // (0=Failed , 1=Complete)
y // Error Message
*/
mysql_close($objConnect);
?>
ไฟล์ php ที่ทำหน้าที่บันทึก้อมูลที่ถูกส่งมาจาก Windows Phone และทำการส่งค่าสถานะกลับไป โดยในตัวอย่างนี้ขะใช้เครื่องหมาย | ในการคั้นค่า result ที่ส่งกลับไป โดบ
x|y
x // (0=Failed , 1=Complete)
y // Error Message
และใน Code ของ php จะเห็นว่าจะมีการตรวจสอบความถูกต้องของข้อมูลก่อนการ Insert ด้วยทุกครั้ง และมีการแจ้งสถานะต่าง ๆ ไปยัง Windows Phone ว่าข้อมูลที่ส่งมานั้น สามารถที่จะ Insert ได้หรือไม่

Windows Phone Project
MainPage.xaml
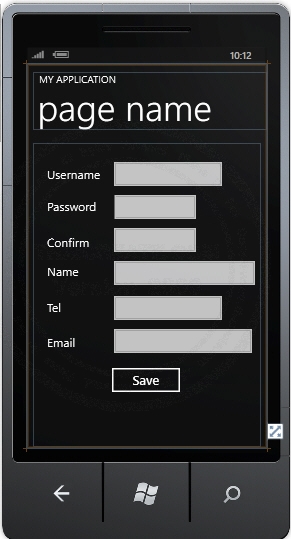
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="ApplicationTitle" Text="MY APPLICATION" Style="{StaticResource PhoneTextNormalStyle}"/>
<TextBlock x:Name="PageTitle" Text="page name" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Margin="12,159,12,2" Grid.RowSpan="2">
<TextBlock Height="30" HorizontalAlignment="Left" Margin="28,45,0,0" Text="Username" VerticalAlignment="Top" FontSize="24" />
<TextBox Height="72" HorizontalAlignment="Left" Margin="150,25,0,0" Name="txtUsername" Text="" VerticalAlignment="Top" Width="240" />
<TextBlock Height="30" HorizontalAlignment="Left" Margin="28,110,0,0" Text="Password" VerticalAlignment="Top" FontSize="24" />
<PasswordBox Height="72" HorizontalAlignment="Right" Margin="0,0,118,444" Name="txtPassword" VerticalAlignment="Bottom" Width="187" />
<TextBlock Height="30" HorizontalAlignment="Left" Margin="28,182,0,0" Text="Confirm" VerticalAlignment="Top" FontSize="24" />
<PasswordBox Height="72" HorizontalAlignment="Left" Margin="150,157,0,0" Name="txtConPassword" VerticalAlignment="Top" Width="188" />
<TextBlock Height="30" HorizontalAlignment="Left" Margin="28,240,0,0" Text="Name" VerticalAlignment="Top" FontSize="24" />
<TextBox Height="72" HorizontalAlignment="Left" Margin="150,223,0,0" Name="txtName" Text="" VerticalAlignment="Top" Width="306" />
<TextBlock Height="30" HorizontalAlignment="Left" Margin="28,312,0,0" Text="Tel" VerticalAlignment="Top" FontSize="24" />
<TextBox Height="72" HorizontalAlignment="Left" Margin="150,293,0,0" Name="txtTel" Text="" VerticalAlignment="Top" Width="240" />
<TextBlock Height="30" HorizontalAlignment="Left" Margin="28,382,0,0" Text="Email" VerticalAlignment="Top" FontSize="24" />
<TextBox Height="72" HorizontalAlignment="Left" Margin="150,359,0,0" Name="txtEmail" Text="" VerticalAlignment="Top" Width="300" />
<Button Content="Save" Height="72" HorizontalAlignment="Left" Margin="146,437,0,0" Name="btnSave" Click="btnSave_Click" VerticalAlignment="Top" Width="160" />
</Grid>
</Grid>
MainPage.xaml.vb (VB.NET)
Imports System.Text
Partial Public Class MainPage
Inherits PhoneApplicationPage
' Constructor
Public Sub New()
InitializeComponent()
End Sub
Dim prog As ProgressIndicator
Private Sub btnSave_Click(sender As System.Object, e As System.Windows.RoutedEventArgs)
If Me.txtUsername.Text = "" Then
MessageBox.Show("Please input (Username)")
Exit Sub
End If
If Me.txtPassword.Password.ToString = "" Then
MessageBox.Show("Please input (Password)")
Exit Sub
End If
If Me.txtConPassword.Password.ToString = "" Then
MessageBox.Show("Please input (Confirm Username)")
Exit Sub
End If
If Me.txtPassword.Password.ToString <> Me.txtConPassword.Password.ToString Then
MessageBox.Show("Password Not Match!")
Exit Sub
End If
If Me.txtName.Text = "" Then
MessageBox.Show("Please input (Name)")
Exit Sub
End If
If Me.txtTel.Text = "" Then
MessageBox.Show("Please input (Tel)")
Exit Sub
End If
If Me.txtEmail.Text = "" Then
MessageBox.Show("Please input (Email)")
Exit Sub
End If
Dim url As String = "http://localhost/myphp/saveData.php"
Dim uri As Uri = New Uri(url, UriKind.Absolute)
Dim postData As StringBuilder = New StringBuilder()
postData.AppendFormat("{0}={1}", "sUsername", HttpUtility.UrlEncode(Me.txtUsername.Text))
postData.AppendFormat("&{0}={1}", "sPassword", HttpUtility.UrlEncode(Me.txtPassword.Password.ToString))
postData.AppendFormat("&{0}={1}", "sName", HttpUtility.UrlEncode(Me.txtName.Text))
postData.AppendFormat("&{0}={1}", "sTel", HttpUtility.UrlEncode(Me.txtTel.Text))
postData.AppendFormat("&{0}={1}", "sEmail", HttpUtility.UrlEncode(Me.txtEmail.Text))
Dim client As WebClient
client = New WebClient()
client.Headers(HttpRequestHeader.ContentType) = "application/x-www-form-urlencoded"
client.Headers(HttpRequestHeader.ContentLength) = postData.Length.ToString()
AddHandler client.UploadStringCompleted, AddressOf client_UploadStringCompleted
AddHandler client.UploadProgressChanged, AddressOf client_UploadProgressChanged
client.UploadStringAsync(uri, "POST", postData.ToString())
prog = New ProgressIndicator()
prog.IsIndeterminate = True
prog.IsVisible = True
prog.Text = "Loading...."
SystemTray.SetProgressIndicator(Me, prog)
End Sub
Private Sub client_UploadProgressChanged(sender As Object, e As UploadProgressChangedEventArgs)
'Me.txtResult.Text = "Uploading.... " & e.ProgressPercentage & "%"
End Sub
Private Sub client_UploadStringCompleted(sender As Object, e As UploadStringCompletedEventArgs)
If e.Cancelled = False And e.Error Is Nothing Then
Dim result As String() = e.Result.ToString.Split("|")
'*** Check Status
If result(0).ToString = "0" Then
MessageBox.Show(result(1))
Else
MessageBox.Show("Save Data Successfully")
Me.txtUsername.Text = ""
Me.txtPassword.Password = ""
Me.txtConPassword.Password = ""
Me.txtName.Text = ""
Me.txtTel.Text = ""
Me.txtEmail.Text = ""
End If
prog.IsVisible = False
End If
End Sub
End Class
MainPage.xaml.cs (C#)
using System;
using System.Windows;
using Microsoft.Phone.Controls;
using System.IO;
using System.Text;
using Microsoft.Phone.Shell;
using System.Net;
namespace PhoneApp
{
public partial class MainPage : PhoneApplicationPage
{
// Constructor
public MainPage()
{
InitializeComponent();
}
ProgressIndicator prog;
private void btnSave_Click(System.Object sender, System.Windows.RoutedEventArgs e)
{
if (string.IsNullOrEmpty(this.txtUsername.Text))
{
MessageBox.Show("Please input (Username)");
return;
}
if (string.IsNullOrEmpty(this.txtPassword.Password.ToString()))
{
MessageBox.Show("Please input (Password)");
return;
}
if (string.IsNullOrEmpty(this.txtConPassword.Password.ToString()))
{
MessageBox.Show("Please input (Confirm Username)");
return;
}
if (this.txtPassword.Password.ToString() != this.txtConPassword.Password.ToString())
{
MessageBox.Show("Password Not Match!");
return;
}
if (string.IsNullOrEmpty(this.txtName.Text))
{
MessageBox.Show("Please input (Name)");
return;
}
if (string.IsNullOrEmpty(this.txtTel.Text))
{
MessageBox.Show("Please input (Tel)");
return;
}
if (string.IsNullOrEmpty(this.txtEmail.Text))
{
MessageBox.Show("Please input (Email)");
return;
}
string url = "http://localhost/myphp/saveData.php";
Uri uri = new Uri(url, UriKind.Absolute);
StringBuilder postData = new StringBuilder();
postData.AppendFormat("{0}={1}", "sUsername", HttpUtility.UrlEncode(this.txtUsername.Text));
postData.AppendFormat("&{0}={1}", "sPassword", HttpUtility.UrlEncode(this.txtPassword.Password.ToString()));
postData.AppendFormat("&{0}={1}", "sName", HttpUtility.UrlEncode(this.txtName.Text));
postData.AppendFormat("&{0}={1}", "sTel", HttpUtility.UrlEncode(this.txtTel.Text));
postData.AppendFormat("&{0}={1}", "sEmail", HttpUtility.UrlEncode(this.txtEmail.Text));
WebClient client = default(WebClient);
client = new WebClient();
client.Headers[HttpRequestHeader.ContentType] = "application/x-www-form-urlencoded";
client.Headers[HttpRequestHeader.ContentLength] = postData.Length.ToString();
client.UploadStringCompleted += client_UploadStringCompleted;
client.UploadProgressChanged += client_UploadProgressChanged;
client.UploadStringAsync(uri, "POST", postData.ToString());
prog = new ProgressIndicator();
prog.IsIndeterminate = true;
prog.IsVisible = true;
prog.Text = "Loading....";
SystemTray.SetProgressIndicator(this, prog);
}
private void client_UploadProgressChanged(object sender, UploadProgressChangedEventArgs e)
{
//Me.txtResult.Text = "Uploading.... " & e.ProgressPercentage & "%"
}
private void client_UploadStringCompleted(object sender, UploadStringCompletedEventArgs e)
{
if (e.Cancelled == false & e.Error == null)
{
string[] result = e.Result.ToString().Split('|');
//*** Check Status
if (result[0].ToString() == "0")
{
MessageBox.Show(result[1].ToString());
}
else
{
MessageBox.Show("Save Data Successfully");
this.txtUsername.Text = "";
this.txtPassword.Password = "";
this.txtConPassword.Password = "";
this.txtName.Text = "";
this.txtTel.Text = "";
this.txtEmail.Text = "";
}
prog.IsVisible = false;
}
}
}
}
ในตัวอย่างนี้มี Code ทั้งที่เป็น VB.NET และ C# และสามารถดาวน์โหลด All Code ทั้งหมดได้จากส่วนท้ายของบทความ (Login สมาชิกก่อน)
Screenshot
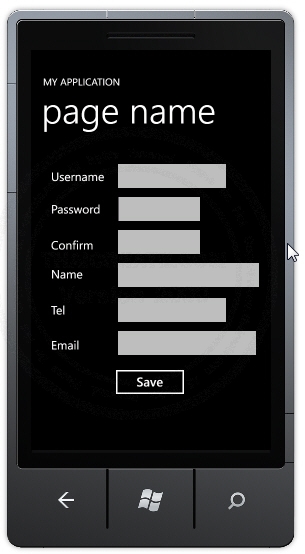
หน้าจอ Page สำหรับ Insert ข้อมูลบนหน้า Page ของ Windows Phone
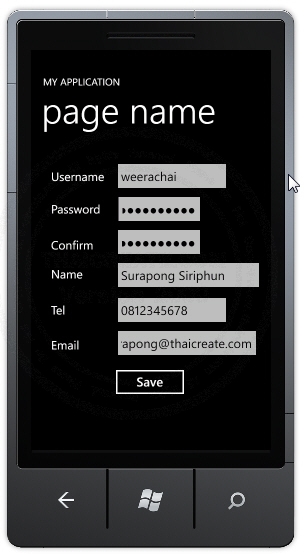
ทดสอบการ Input ข้อมูล
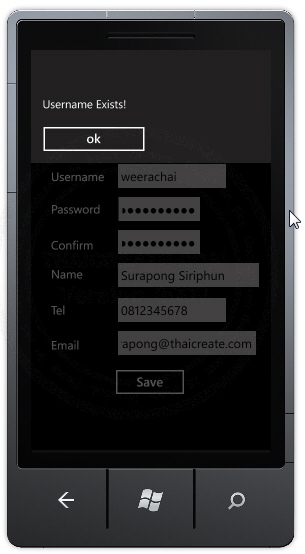
Server ส่งค่ากลับในกรณีที่ Insert ข้อมูล Username ซ้ำ
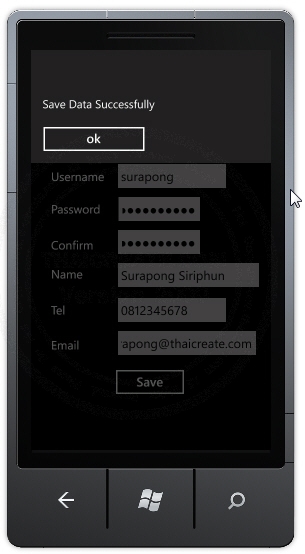
ในกรณีที่ Insert ข้อมูลผ่าน
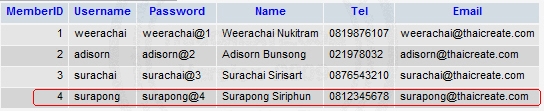
หลังจาก Insert ข้อมูลผ่านแล้ว เมื่อทดสอบเปิดดูตารางบน phpMyAdmin ในฝั่ง Web Server ก็จะเห็นว่าข้อมูลได้ถูก Insert ส่งไปเรียบร้อย
.
|