Windows Phone Connect to Web Server (WebClient and DownloadStringAsync) |
Windows Phone Connect to Web Server (WebClient and DownloadStringAsync) ในการเขียน App บน Windows Phone เพื่ออ่านข้อมูลที่อยู่บน Web Server (URL) และ Website สามารถทำได้ง่าย ๆ ผ่าน Function ที่มีชื่อว่า WebClient เพียงแค่ระบุ URL ปลายทางก็สามารถที่จะเรียกค่า Result ได้ในทันที โดยจะมี Method ที่ทำหน้าที่ควบคุมการทำงานอยู่ 2 ตัวคือ ProgressChanged และ Completed และใน ProgressChanged จะสามารถอ่านสถานะและ Progress การทำงานได้เช่นเดียวกัน
Windows Phone Connect to Web Server
รูปแบบการเชื่อมต่อไปยัง Web Server ผ่าน WebClient
Dim client As WebClient
Private Sub MainPage_Loaded(sender As Object, e As System.Windows.RoutedEventArgs)
Dim url As String = "http://localhost/myphp/hello.php"
Dim uri As New Uri(url)
client = New WebClient()
client.AllowReadStreamBuffering = True
AddHandler client.DownloadStringCompleted, AddressOf client_DownloadStringCompleted
AddHandler client.DownloadProgressChanged, AddressOf client_DownloadProgressChanged
client.DownloadStringAsync(uri)
End Sub
Private Sub client_DownloadStringCompleted(sender As Object, e As DownloadStringCompletedEventArgs)
End Sub
Private Sub client_DownloadProgressChanged(sender As Object, e As DownloadProgressChangedEventArgs)
End Sub
Example 1 เขียน Windows Phone เพื่อเชื่อมต่อและอ่านข้อมูลจาก Web Server แบบง่าย ๆ
hello.php
<?php
echo "Server Time : ".date("Y-m-d H:i:s");
?>
ไฟล์ php บนฝั่ง Web Server ซึ่งจะแสดง วันที่และเวลาล่าสุดของ Web Server
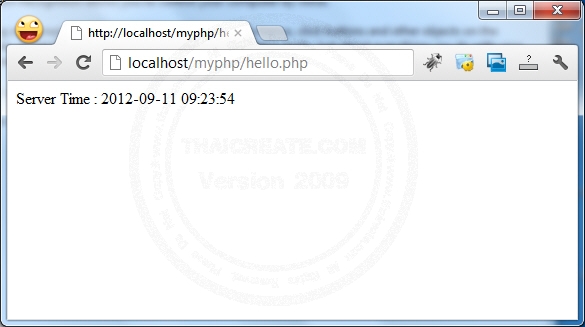
เมื่อทดสอบผ่าน Web Browser
MainPage.xaml
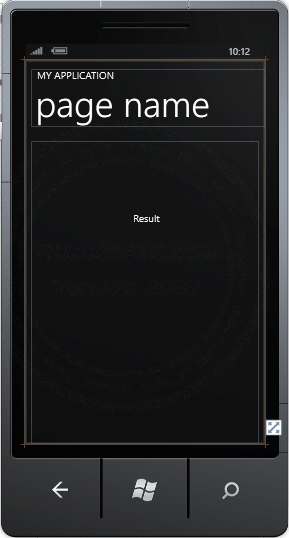
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="ApplicationTitle" Text="MY APPLICATION" Style="{StaticResource PhoneTextNormalStyle}"/>
<TextBlock x:Name="PageTitle" Text="page name" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<TextBlock Height="30" HorizontalAlignment="Left" Margin="12,141,0,0" Name="txtResult" Text="Result" VerticalAlignment="Top" Width="438" TextAlignment="Center" />
</Grid>
</Grid>

MainPage.xaml.vb (VB.NET)
Partial Public Class MainPage
Inherits PhoneApplicationPage
' Constructor
Public Sub New()
InitializeComponent()
AddHandler Loaded, AddressOf MainPage_Loaded
End Sub
Dim client As WebClient
Private Sub MainPage_Loaded(sender As Object, e As System.Windows.RoutedEventArgs)
Dim url As String = "http://localhost/myphp/hello.php"
Dim uri As New Uri(url)
client = New WebClient()
client.AllowReadStreamBuffering = True
AddHandler client.DownloadStringCompleted, AddressOf client_DownloadStringCompleted
AddHandler client.DownloadProgressChanged, AddressOf client_DownloadProgressChanged
client.DownloadStringAsync(uri)
End Sub
Private Sub client_DownloadStringCompleted(sender As Object, e As DownloadStringCompletedEventArgs)
If e.Cancelled = False And e.Error Is Nothing Then
System.Threading.Thread.Sleep(2000)
Dim textString As String = CStr(e.Result)
Me.txtResult.Text = textString
End If
End Sub
Private Sub client_DownloadProgressChanged(sender As Object, e As DownloadProgressChangedEventArgs)
Me.txtResult.Text = "Loading..."
End Sub
End Class
MainPage.xaml.cs (C#)
using System;
using System.Windows;
using Microsoft.Phone.Controls;
using Microsoft.Phone.Shell;
using System.Net;
namespace PhoneApp
{
public partial class MainPage : PhoneApplicationPage
{
// Constructor
public MainPage()
{
InitializeComponent();
Loaded += MainPage_Loaded;
}
WebClient client;
private void MainPage_Loaded(object sender, System.Windows.RoutedEventArgs e)
{
string url = "http://localhost/myphp/hello.php";
Uri uri = new Uri(url);
client = new WebClient();
client.AllowReadStreamBuffering = true;
client.DownloadStringCompleted += client_DownloadStringCompleted;
client.DownloadProgressChanged += client_DownloadProgressChanged;
client.DownloadStringAsync(uri);
}
private void client_DownloadStringCompleted(object sender, DownloadStringCompletedEventArgs e)
{
if (e.Cancelled == false && e.Error == null)
{
System.Threading.Thread.Sleep(2000);
string textString = Convert.ToString(e.Result);
this.txtResult.Text = textString;
}
}
private void client_DownloadProgressChanged(object sender, DownloadProgressChangedEventArgs e)
{
this.txtResult.Text = "Loading...";
}
}
}
ในตัวอย่างนี้มี Code ทั้งที่เป็น VB.NET และ C# และสามารถดาวน์โหลด All Code ทั้งหมดได้จากส่วนท้ายของบทความ (Login สมาชิกก่อน)
Screenshot
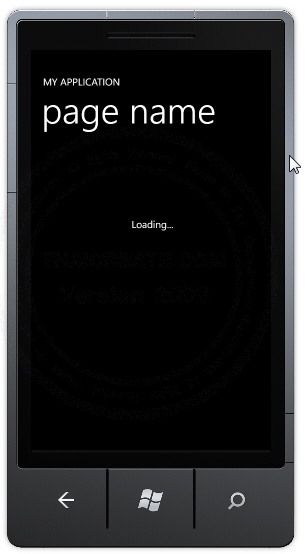
กำลังโหลดข้อมูลจาก Web Server ซึ่งจะแสดงคำว่า Loading... ในขณะที่กำลัง Reqeust
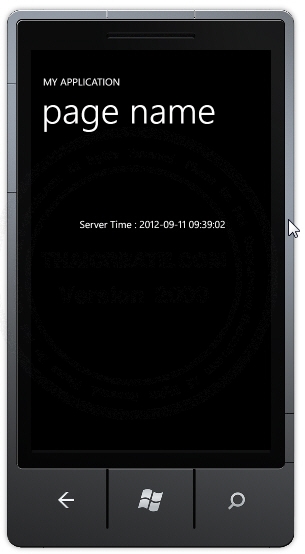
แสดงค่า Result ที่ถูกส่งมาจาก Web Server
Example 2 แสดงสถานะและ Bytes Received ที่ถูกส่งมาจาก Web Server
hello.php
<?
for($i=0;$i<=10;$i++)
{
echo "Hello Hello Hello Hello Hello";
ob_flush();
flush();
usleep(300000);
}
?>
ไฟล์ php ที่อยู่บนฝั่ง Web Server
MainPage.xaml
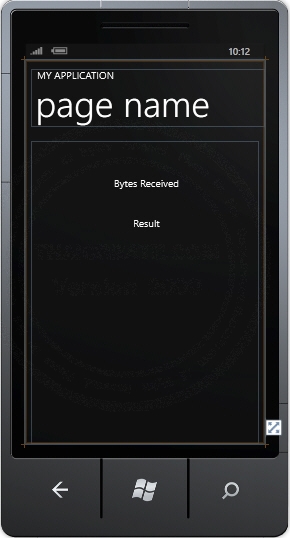
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="ApplicationTitle" Text="MY APPLICATION" Style="{StaticResource PhoneTextNormalStyle}"/>
<TextBlock x:Name="PageTitle" Text="page name" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<TextBlock Height="30" HorizontalAlignment="Left" Margin="12,72,0,0" Name="txtBytesReceived" Text="Bytes Received" TextAlignment="Center" VerticalAlignment="Top" Width="438" />
<TextBlock Height="30" HorizontalAlignment="Left" Margin="12,151,0,0" Name="txtResult" Text="Result" VerticalAlignment="Top" Width="438" TextAlignment="Center" />
</Grid>
</Grid>
MainPage.xaml.vb (VB.NET)
Partial Public Class MainPage
Inherits PhoneApplicationPage
' Constructor
Public Sub New()
InitializeComponent()
AddHandler Loaded, AddressOf MainPage_Loaded
End Sub
Dim client As WebClient
Dim prog As ProgressIndicator
Private Sub MainPage_Loaded(sender As Object, e As System.Windows.RoutedEventArgs)
Dim url As String = "http://localhost/myphp/hello.php"
Dim uri As New Uri(url)
client = New WebClient()
client.AllowReadStreamBuffering = True
AddHandler client.DownloadStringCompleted, AddressOf client_DownloadStringCompleted
AddHandler client.DownloadProgressChanged, AddressOf client_DownloadProgressChanged
client.DownloadStringAsync(uri)
'*** SystemTray ProgressBar ***'
prog = New ProgressIndicator()
prog.IsVisible = True
prog.IsIndeterminate = True
prog.Text = "Downloading...."
SystemTray.SetProgressIndicator(Me, prog)
End Sub
Private Sub client_DownloadStringCompleted(sender As Object, e As DownloadStringCompletedEventArgs)
If e.Cancelled = False And e.Error Is Nothing Then
Dim textString As String = CStr(e.Result)
Me.txtResult.Text = textString
prog.IsVisible = False
End If
End Sub
Private Sub client_DownloadProgressChanged(sender As Object, e As DownloadProgressChangedEventArgs)
Me.txtBytesReceived.Text = e.BytesReceived
Me.txtResult.Text = "Loading..."
End Sub
End Class
[--Ad2-]]
MainPage.xaml.cs (C#)
using System;
using System.Windows;
using Microsoft.Phone.Controls;
using Microsoft.Phone.Shell;
using System.Net;
namespace PhoneApp
{
public partial class MainPage : PhoneApplicationPage
{
// Constructor
public MainPage()
{
InitializeComponent();
Loaded += MainPage_Loaded;
}
WebClient client;
ProgressIndicator prog;
private void MainPage_Loaded(object sender, System.Windows.RoutedEventArgs e)
{
string url = "http://localhost/myphp/hello.php";
Uri uri = new Uri(url);
client = new WebClient();
client.AllowReadStreamBuffering = true;
client.DownloadStringCompleted += client_DownloadStringCompleted;
client.DownloadProgressChanged += client_DownloadProgressChanged;
client.DownloadStringAsync(uri);
//*** SystemTray ProgressBar ***'
prog = new ProgressIndicator();
prog.IsVisible = true;
prog.IsIndeterminate = true;
prog.Text = "Downloading....";
SystemTray.SetProgressIndicator(this, prog);
}
private void client_DownloadStringCompleted(object sender, DownloadStringCompletedEventArgs e)
{
if (e.Cancelled == false && e.Error == null)
{
System.Threading.Thread.Sleep(2000);
string textString = Convert.ToString(e.Result);
this.txtResult.Text = textString;
prog.IsVisible = false;
}
}
private void client_DownloadProgressChanged(object sender, DownloadProgressChangedEventArgs e)
{
this.txtBytesReceived.Text = e.BytesReceived.ToString();
this.txtResult.Text = "Loading...";
}
}
}
ในตัวอย่างนี้มี Code ทั้งที่เป็น VB.NET และ C# และสามารถดาวน์โหลด All Code ทั้งหมดได้จากส่วนท้ายของบทความ (Login สมาชิกก่อน)
Screenshot
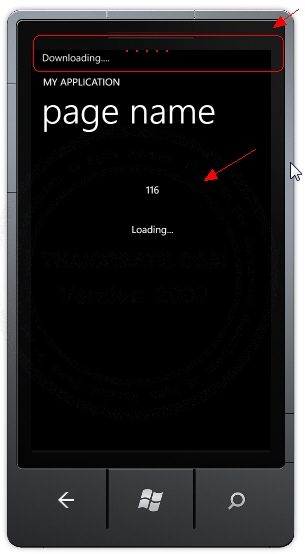
กำลังเชื่อมต่อกับ Web Server และแสดงสถานะการโหลดข้อมูล
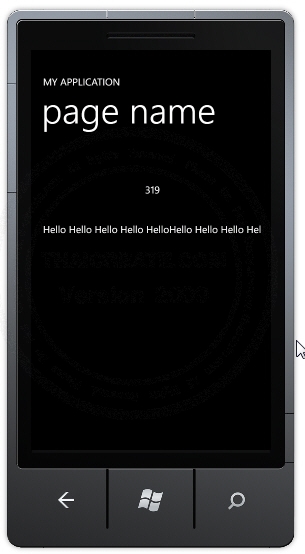
โหลดข้อมูลเรียบร้อย
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-09-15 12:53:29 /
2017-03-25 22:29:23 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|