Windows Phone and Data Binding |
Windows Phone and Data Binding ในการเขียนโปรแกรมบน Windows Phone สิ่งที่ขาดไม่ได้ก็คือการทำ Binding ข้อมูลกับชุดของข้อมูลและ Interace ที่เป็น XAML ความหมายของ Data Binding คือการผูกเชื่อมชุดของข้อมูลที่อยู่ในรูปแบบของ Object ต่าง ๆ (จาก Code Behind และรูปแบบอื่น ๆ ) กับ Interace หน้าจอที่อยู่ในหน้า Page บน XAML Layout เช่น การอ่านข้อมูลจาก Database เพื่อแสดงผลในหน้า Page หรือชุดข้อมูลจากแหล่งอื่น ๆ เช่น Web Server , JSON หรือ XML เป็นต้น
Windows Phone and Data Binding
Example ตัวอย่างการทำ Data Binding แบบง่าย ๆ โดยแสดงข้อมูลบน Textbox
MainPage.xaml
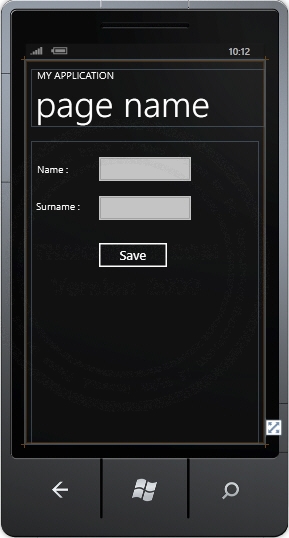
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="ApplicationTitle" Text="MY APPLICATION" Style="{StaticResource PhoneTextNormalStyle}"/>
<TextBlock x:Name="PageTitle" Text="page name" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<TextBlock Height="30" HorizontalAlignment="Left" Margin="12,43,0,0" Name="txtTileName" Text="Name : " VerticalAlignment="Top" />
<TextBox Height="72" HorizontalAlignment="Left" Margin="124,21,0,0" Name="txtName" Text="{Binding Name}" VerticalAlignment="Top" Width="208" />
<TextBlock Height="30" HorizontalAlignment="Left" Margin="10,118,0,0" Name="txtTitleSurname" Text="Surname : " VerticalAlignment="Top" />
<TextBox Height="72" HorizontalAlignment="Left" Margin="124,99,0,0" Name="txtSurname" Text="{Binding Surname}" VerticalAlignment="Top" Width="208" />
<Button Content="Save" Height="72" HorizontalAlignment="Left" Margin="124,193,0,0" Name="btnSave" VerticalAlignment="Top" Width="160" />
</Grid>
</Grid>
MainPage.xaml.vb (VB.NET)
Partial Public Class MainPage
Inherits PhoneApplicationPage
' Constructor
Public Sub New()
InitializeComponent()
AddHandler Loaded, AddressOf MainPage_Loaded
End Sub
Private Sub MainPage_Loaded(sender As Object, e As System.Windows.RoutedEventArgs)
Dim iPerson As New Person("Weerachai", "Nukitram")
ContentPanel.DataContext = iPerson
End Sub
End Class
Public Class Person
Public Property Name() As String
Get
Return m_Name
End Get
Set(value As String)
m_Name = value
End Set
End Property
Public Property Surname() As String
Get
Return m_Surname
End Get
Set(value As String)
m_Surname = value
End Set
End Property
Private m_Name As String
Private m_Surname As String
Public Sub New(ByVal strName As String, ByVal strSurname As String)
Name = strName
Surname = strSurname
End Sub
End Class

MainPage.xaml.cs (C#)
using System;
using System.Windows;
using Microsoft.Phone.Controls;
namespace PhoneApp
{
public partial class MainPage : PhoneApplicationPage
{
// Constructor
public MainPage()
{
InitializeComponent();
Loaded += MainPage_Loaded;
}
private void MainPage_Loaded(object sender, System.Windows.RoutedEventArgs e)
{
Person iPerson = new Person("Weerachai", "Nukitram");
ContentPanel.DataContext = iPerson;
}
}
public class Person
{
public string Name
{
get { return m_Name; }
set { m_Name = value; }
}
public string Surname
{
get { return m_Surname; }
set { m_Surname = value; }
}
private string m_Name;
private string m_Surname;
public Person(string strName, string strSurname)
{
Name = strName;
Surname = strSurname;
}
}
}
ในตัวอย่างนี้มี Code ทั้งที่เป็น VB.NET และ C# และสามารถดาวน์โหลด All Code ทั้งหมดได้จากส่วนท้ายของบทความ (Login สมาชิกก่อน)
Screenshot
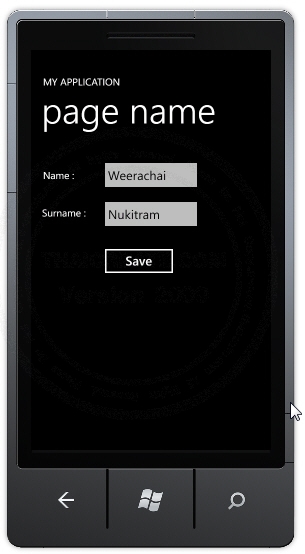
แสดงข้อมูลที่ได้จากการ Binding Data
.
|