Windows Phone and XML Parser |
Windows Phone and XML Parser การเขียน App บน Windows Phone เพื่ออ่านข้อมูล ที่อยู่ในรูปแบบของ XMLไฟล์ โดยใช้ NameSpace บน .NET Framework ที่มีชื่อว่า System.Xml.Linq เข้ามาแปลงข้อมูล XML และนำข้อมูลที่ได้ทำการ Binding ให้กับ ListBox และแสดงผลในหน้า Page ของ Application
Windows Phone and XML
ในการเขียน Windows Phone เพื่ออ่านข้อมูลที่อยู่ในรูปแบบของ XML สามารถใช้ NameSpace ของ System.Xml.Linq
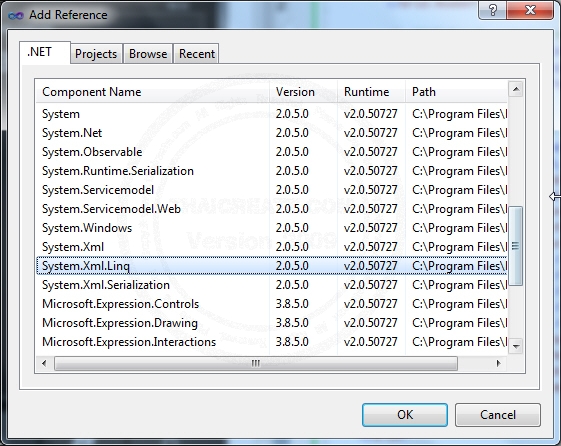
Add Reference Library ของ System.Xml.Linq
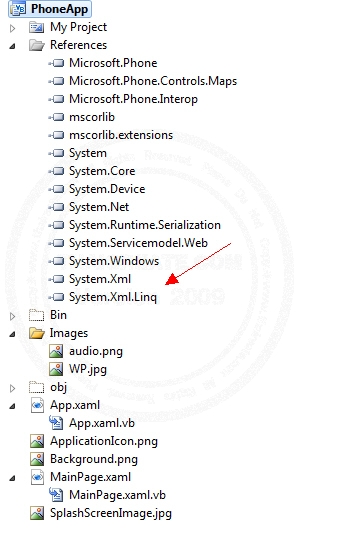
จะได้ NameSpace ของ System.Xml.Linq ดังภาพ
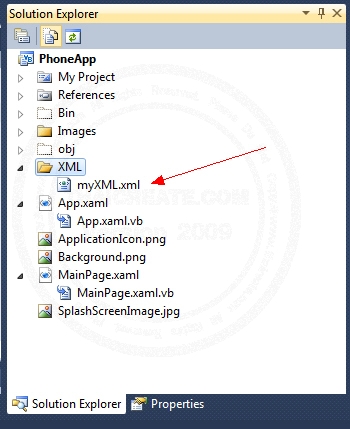
ไฟล์ XML ถูกจัดเก็บไว้ที่ XML/myXML.xml
myXML.xml
<?xml version="1.0" encoding="utf-8"?>
<customers>
<customer>
<CustomerID>C001</CustomerID>
<Name>Win Weerachai</Name>
<Email>[email protected]</Email>
<CountryCode>TH</CountryCode>
<Budget>1000000</Budget>
<Used>600000</Used>
</customer>
<customer>
<CustomerID>C002</CustomerID>
<Name>John Smith</Name>
<Email>[email protected]</Email>
<CountryCode>EN</CountryCode>
<Budget>2000000</Budget>
<Used>800000</Used>
</customer>
<customer>
<CustomerID>C003</CustomerID>
<Name>Jame Born</Name>
<Email>[email protected]</Email>
<CountryCode>US</CountryCode>
<Budget>3000000</Budget>
<Used>600000</Used>
</customer>
<customer>
<CustomerID>C004</CustomerID>
<Name>Chalee Angel</Name>
<Email>[email protected]</Email>
<CountryCode>US</CountryCode>
<Budget>4000000</Budget>
<Used>100000</Used>
</customer>
</customers>
ตัวอย่างของไฟล์ XML

Example การอ่าน XML Parser แบบง่าย NameSpace ของ System.Xml.Linq
MainPage.xaml
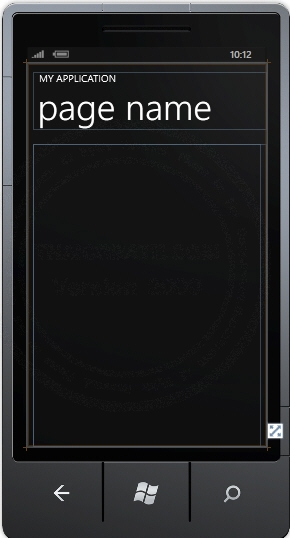
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="ApplicationTitle" Text="MY APPLICATION" Style="{StaticResource PhoneTextNormalStyle}"/>
<TextBlock x:Name="PageTitle" Text="page name" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<ListBox Margin="0,0,-12,0" x:Name="CustomerList">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel>
<TextBlock Text="{Binding CustomerID}" TextWrapping="Wrap" Style="{StaticResource PhoneTextExtraLargeStyle}"/>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
<TextBlock Text="{Binding CountryCode}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
<TextBlock Text="{Binding Budget}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
<TextBlock Text="{Binding Used}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Grid>
MainPage.xaml.vb (VB.NET)
Imports System.Runtime.Serialization
Imports System.Collections.ObjectModel
Imports System.IO
Imports System.Text
Imports System.Xml.Linq
Partial Public Class MainPage
Inherits PhoneApplicationPage
' Constructor
Public Sub New()
InitializeComponent()
AddHandler Loaded, AddressOf MainPage_Loaded
End Sub
Private Sub MainPage_Loaded(sender As Object, e As System.Windows.RoutedEventArgs)
Dim myCustomer As New List(Of Customer)
Dim loadData As XDocument = XDocument.Load("XML/myXML.xml")
Dim data = From query In loadData.Descendants("customer")
Select New Customer With { _
.CustomerID = query.Element("CustomerID"), _
.Name = query.Element("Name"), _
.Email = query.Element("Email"), _
.CountryCode = query.Element("CountryCode"), _
.Budget = query.Element("Budget"), _
.Used = query.Element("Used")
}
Me.CustomerList.ItemsSource = data
End Sub
<DataContract()> _
Public Class Customer
Sub New()
' TODO: Complete member initialization
End Sub
<DataMember()> _
Public Property CustomerID() As String
Get
Return m_CustomerID
End Get
Set(value As String)
m_CustomerID = value
End Set
End Property
<DataMember()> _
Public Property Name() As String
Get
Return m_Name
End Get
Set(value As String)
m_Name = value
End Set
End Property
<DataMember()> _
Public Property Email() As String
Get
Return m_Email
End Get
Set(value As String)
m_Email = value
End Set
End Property
<DataMember()> _
Public Property CountryCode() As String
Get
Return m_CountryCode
End Get
Set(value As String)
m_CountryCode = value
End Set
End Property
<DataMember()> _
Public Property Budget() As String
Get
Return m_Budget
End Get
Set(value As String)
m_Budget = value
End Set
End Property
<DataMember()> _
Public Property Used() As String
Get
Return m_Used
End Get
Set(value As String)
m_Used = value
End Set
End Property
Private m_CustomerID As String
Private m_Name As String
Private m_Email As String
Private m_CountryCode As String
Private m_Budget As String
Private m_Used As String
Public Sub New(ByVal strCustomerID As String,
ByVal strName As String,
ByVal strEmail As String,
ByVal strCountryCode As String,
ByVal strBudget As String,
ByVal strUsed As String)
Me.CustomerID = strCustomerID
Me.Name = strName
Me.Email = strEmail
Me.CountryCode = strCountryCode
Me.Budget = strBudget
Me.Used = strUsed
End Sub
End Class
End Class
MainPage.xaml.cs (C#)
using System;
using System.Windows;
using Microsoft.Phone.Controls;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.IO;
using System.Text;
using System.Xml;
using System.Xml.Linq;
using System.Linq;
namespace PhoneApp
{
public partial class MainPage : PhoneApplicationPage
{
// Constructor
public MainPage()
{
InitializeComponent();
Loaded += MainPage_Loaded;
}
private void MainPage_Loaded(object sender, System.Windows.RoutedEventArgs e)
{
List<Customer> myCustomer = new List<Customer>();
XDocument loadData = XDocument.Load("XML/myXML.xml");
var data = from query in loadData.Descendants("customer")
select new Customer ()
{
CustomerID = (string)query.Element("CustomerID"),
Name = (string)query.Element("Name"),
Email = (string)query.Element("Email"),
CountryCode = (string)query.Element("CountryCode"),
Budget = (string)query.Element("Budget"),
Used = (string)query.Element("Used")
};
this.CustomerList.ItemsSource = data;
}
}
public class Customer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
}
ในตัวอย่างนี้มี Code ทั้งที่เป็น VB.NET และ C# และสามารถดาวน์โหลด All Code ทั้งหมดได้จากส่วนท้ายของบทความ (Login สมาชิกก่อน)
Screenshot
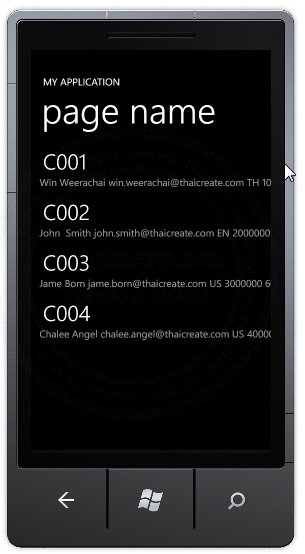
แสดง XML กับ ListBox บน Data Binding
|