 |
สอบถามปัญหา เกี่ยวกับการตั้ง Auto ให้ตัวเลขเรียงลำดับเวลาเพิ่มข้อมูลลงในฐานข้อมูลอะครับ |
|
 |
|
|
 |
 |
|
ส่วนที่ 1
Code (PHP)
<?php require_once("../Connections/docconnection.php"); ?>
<?php
if (!isset($_SESSION)) {
session_start();
}
include ("../function.php");
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? "'" . doubleval($theValue) . "'" : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
mysql_select_db($database_schoolsmile, $schoolsmile);
$query_GroupRS = sprintf("SELECT * FROM tbl_group ORDER BY id_g ASC");
$GroupRS = mysql_query($query_GroupRS, $schoolsmile) or die(mysql_error());
$row_GroupRS = mysql_fetch_assoc($GroupRS);
$totalRows_GroupRS = mysql_num_rows($GroupRS);
?>
<?php
if ((isset($_POST['hiddenDelID'])) && ($_POST['hiddenDelID'] != "")) {
$deleteSQL = sprintf("DELETE FROM tbl_group WHERE id_g = %s",
GetSQLValueString($_POST['hiddenDelID'], "int"));
mysql_select_db($database_schoolsmile, $schoolsmile);
$Result1 = mysql_query($deleteSQL, $schoolsmile) or die(mysql_error());
$deleteGoTo = "showGroup.php";
if (isset($_SERVER['QUERY_STRING'])) {
$deleteGoTo .= (strpos($deleteGoTo, '?')) ? "&" : "?";
$deleteGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $deleteGoTo));
}
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Frameset//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-frameset.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>ระบบจัดการเว็บไซต์</title>
<link href="adminstyle.css" rel="stylesheet" type="text/css">
<style type="text/css">
<!--
.style1 {color: #FF0000}
-->
</style>
</head>
<table width="560" border="0" align="center" cellpadding="0" cellspacing="0">
<tr>
<td align="left"><table width="550" border="1" align="center" cellpadding="0" cellspacing="0" bordercolor="#CCCCCC">
<tr>
<td height="25" colspan="4" align="center" bordercolor="#FFFFFF" bgcolor="#E4CAAF"><img src="../images/14.png" width="16" height="16" /> แสดงส่วนงาน</td>
</tr>
<tr>
<td height="10" colspan="4" align="right" bordercolor="#FFFFFF">จำนวนทั้งหมด <?php echo $totalRows_GroupRS; ?></td>
</tr>
<tr>
<td width="140" height="25" align="center" bordercolor="#FFFFFF" bgcolor="#CCCCCC">รหัสส่วนงาน</td>
<td align="center" bordercolor="#FFFFFF" bgcolor="#CCCCCC">ชื่อส่วนงาน</td>
<td width="40" align="center" bordercolor="#FFFFFF" bgcolor="#CCCCCC">แก้ไข</td>
<td width="40" align="center" bordercolor="#FFFFFF" bgcolor="#CCCCCC">ลบ</td>
</tr>
<?php if ($totalRows_GroupRS > 0) { // Show if recordset not empty ?>
<?php do { ?>
<tr >
<td height="1" colspan="4" align="center" bordercolor="#FFFFFF" bgcolor="#CCCCCC"><img src="../images/px1.gif" width="1" height="1"></td>
</tr>
<tr >
<td bordercolor="#FFFFFF" bgcolor="#F5F5F5"> <strong><?php echo $row_GroupRS['id_g']; ?></strong></td>
<td align="left" bordercolor="#FFFFFF" bgcolor="#F5F5F5"> <strong><?php echo $row_GroupRS['gname']; ?></strong>
</td>
<td align="center" bordercolor="#FFFFFF" bgcolor="#F5F5F5">
<form name="formEdit" method="post" action="showGroup.php#editGroup">
<input type="image" name="edit" value="Submit" src="../images/b_edit.png" alt="แก้ไขข้อมูล">
<br>
<input name="hiddenEditID" type="hidden" id="hiddenEditID" value="<?php echo $row_GroupRS['id_g']; ?>">
</form></td>
<td align="center" bordercolor="#FFFFFF" bgcolor="#F5F5F5">
<form name="formDelete" method="post" action="showGroup.php" onsubmit="return R_confirm('<?php echo $row_GroupRS['gname']; ?>')">
<input type="image" name="edit" value="Submit" src="../images/b_drop.png" alt="ลบข้อมูล" >
<br>
<input name="hiddenDelID" type="hidden" id="hiddenDelID" value="<?php echo $row_GroupRS['id_g']; ?>">
</form></td>
</tr>
<?php } while ($row_GroupRS = mysql_fetch_assoc($GroupRS)); ?>
<?php } // Show if recordset not empty ?>
</table>
<br>
<a name="editGroup"></a>
<?php
if ((isset($_POST['hiddenEditID'])) && ($_POST['hiddenEditID'] != "")) {
include("editGroup.php");
}
else {
include ("addGroup.php");
}
?>
<br>
</td>
</tr>
</table>
<script language="JavaScript">
function R_confirm(msg)
{
var x=window.confirm("ต้องการลบข้อมูลนี้\n'"+msg+"'\nใช่หรือไม่?");
return (x);
}
</script>
<?php
mysql_free_result($GroupRS);
?>
ส่วนที่ 2
Code (PHP)
<?php require_once('../Connections/docconnection.php'); ?>
<?php
if (!isset($_SESSION)) {
session_start();
}
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? "'" . doubleval($theValue) . "'" : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$editFormAction = "addGroup.php";
if (isset($_SERVER['QUERY_STRING'])) {
$editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']);
}
if ((isset($_POST["MM_insert"])) && ($_POST["MM_insert"] == "frmAddGroup")) {
$insertSQL = sprintf("INSERT INTO tbl_group (gname) VALUES (%s)",
GetSQLValueString($_POST['txtName'], "text"));
mysql_select_db($database_schoolsmile, $schoolsmile);
$Result1 = mysql_query($insertSQL, $schoolsmile) or die(mysql_error());
$insertGoTo = "showGroup.php";
if (isset($_SERVER['QUERY_STRING'])) {
$insertGoTo .= (strpos($insertGoTo, '?')) ? "&" : "?";
$insertGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $insertGoTo));
}
?>
<form action="<?php echo $editFormAction; ?>" method="POST" enctype="multipart/form-data" name="frmAddGroup">
<table width="550" border="1" align="center" cellpadding="0" cellspacing="1">
<tr>
<td height="25" colspan="3" bordercolor="#FFFFFF" bgcolor="#CCCCCC"><img src="../images/b_new.png" width="16" height="16">เพิ่มส่วนงาน..
</td>
</tr>
<tr>
<td width="163" height="25" align="right" bordercolor="#FFFFFF">ชื่อส่วนงาน :</td>
<td width="5" bordercolor="#FFFFFF"> </td>
<td width="370" bordercolor="#FFFFFF"><input name="txtName" type="text" id="txtName" size="40"></td>
</tr>
<tr>
<td height="25" align="right" bordercolor="#FFFFFF"> </td>
<td bordercolor="#FFFFFF"> </td>
<td bordercolor="#FFFFFF"><label>
<input type="submit" name="button" id="button" value="บันทึกข้อมูล...">
<input type="button" name="back" value="ยกเลิก" onclick="javascript:history.go(-1)"/></label></td>
</tr>
</table>
<input type="hidden" name="MM_insert" value="frmAddGroup">
</form>
ฐานข้อมูลผมเซตเป็น auto_increment ซึ่งเมื่อผมลบข้อมูลออก 1 ตัว ลำดับมันไม่ไปแทนที่เดิม
เช่น
ข้อมูลมี 5 ลำดับ ผมลบลำดับที่ 5 ออก พอเพิ่มเข้าไปใหม่กลายเป็นลำดับที่ 6 ซะงั้น
พอเพิ่ม
Tag : PHP
|
|
 |
 |
 |
 |
Date :
2013-09-17 10:45:38 |
By :
Jumper |
View :
654 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
หน้าส่วนที่ 1
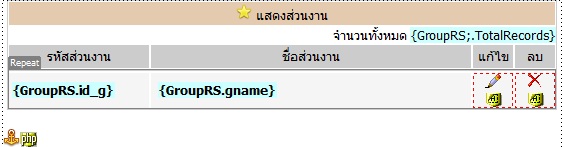
หน้าส่วนที่ 2

|
 |
 |
 |
 |
Date :
2013-09-17 10:47:18 |
By :
jumper |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แนะนำอย่าทำอย่างนั้นเลยครับ ไม่เป็นผลดีต่อระบบครับ ถ้าอยากให้มันเรียง ก็สร้างเลขลำดับเอาเองครับ ส่วนที่เป็นอินเด็กก็ให้มันอยู่อย่างนั้นแหละครับ เช่น
$i=0;
while()
$i++;
เอา $i ไปใช้ครับ มันจะเรียงลำดับให้ครับ
|
 |
 |
 |
 |
Date :
2013-09-17 10:52:15 |
By :
Dragons_first |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|