|
 |
|
สอบถามการทำ paging แบบ ย่อครับ จาก ลิ้ง [PHP MySQL Pagination] |
|
 |
|
|
 |
 |
|
จากที่มา : https://www.thaicreate.com/community/php-mysql-pagination.html
เมื่อผม อยุ่ที่ หน้าที่ 2 - 3 - 4 หรืออื่นๆ ผมกด ตัวเลขได้ กด ปุ่ม next ได้ครับ แต่เมื่อกด Previous แล้วมันเกิด เออเร่อว่า
Error Query [SELECT * FROM tb_pr ORDER BY id DESC LIMIT -5 , 5]
จากโค้ดครับ
Code (PHP)
<?php
class Paginator{
var $items_per_page;
var $items_total;
var $current_page;
var $num_pages;
var $mid_range;
var $low;
var $high;
var $limit;
var $return;
var $default_ipp;
var $querystring;
var $url_next;
function Paginator()
{
$this->current_page = 1;
$this->mid_range = 7;
$this->items_per_page = $this->default_ipp;
$this->url_next = $this->url_next;
}
function paginate()
{
if(!is_numeric($this->items_per_page) OR $this->items_per_page <= 0) $this->items_per_page = $this->default_ipp;
$this->num_pages = ceil($this->items_total/$this->items_per_page);
if($this->current_page < 1 Or !is_numeric($this->current_page)) $this->current_page = 1;
if($this->current_page > $this->num_pages) $this->current_page = $this->num_pages;
$prev_page = $this->current_page-1;
$next_page = $this->current_page+1;
if($this->num_pages > $this->mid_range)/*10*/
{
$this->return = ($this->current_page != 1 And $this->items_total >= $this->mid_range)/*10*/ ? "<a class=\"paginate\" href=\"".$this->url_next.$this->$prev_page."\">« Previous</a> ":"<span class=\"inactive\" href=\"#\">« Previous</span> ";
$this->start_range = $this->current_page - floor($this->mid_range/2);
$this->end_range = $this->current_page + floor($this->mid_range/2);
if($this->start_range <= 0)
{
$this->end_range += abs($this->start_range)+1;
$this->start_range = 1;
}
if($this->end_range > $this->num_pages)
{
$this->start_range -= $this->end_range-$this->num_pages;
$this->end_range = $this->num_pages;
}
$this->range = range($this->start_range,$this->end_range);
for($i=1;$i<=$this->num_pages;$i++)
{
if($this->range[0] > 2 And $i == $this->range[0]) $this->return .= " ... ";
if($i==1 Or $i==$this->num_pages Or in_array($i,$this->range))
{
$this->return .= ($i == $this->current_page And $_GET['PagePR'] != 'All') ? "<a title=\"Go to page $i of $this->num_pages\" class=\"current\" href=\"#\">$i</a> ":"<a class=\"paginate\" title=\"Go to page $i of $this->num_pages\" href=\"".$this->url_next.$i."\">$i</a> ";
}
if($this->range[$this->mid_range-1] < $this->num_pages-1 And $i == $this->range[$this->mid_range-1]) $this->return .= " ... ";
}
$this->return .= (($this->current_page != $this->num_pages And $this->items_total >= $this->mid_range)/*10*/ And ($_GET['PagePR'] != 'All')) ? "<a class=\"paginate\" href=\"".$this->url_next.$next_page."\">Next »</a>\n":"<span class=\"inactive\" href=\"#\">» Next</span>\n";
}
else
{
for($i=1;$i<=$this->num_pages;$i++)
{
$this->return .= ($i == $this->current_page) ? "<a class=\"current\" href=\"#\">$i</a> ":"<a class=\"paginate\" href=\"".$this->url_next.$i."\">$i</a> ";
}
}
$this->low = ($this->current_page-1) * $this->items_per_page;
$this->high = ($_GET['ipp'] == 'All') ? $this->items_total:($this->current_page * $this->items_per_page)-1;
$this->limit = ($_GET['ipp'] == 'All') ? "":" LIMIT $this->low,$this->items_per_page";
}
function display_pages()
{
return $this->return;
}
}
?>
<style type="text/css">
<!--
.paginate {
font-family: Arial, Helvetica, sans-serif;
font-size: .7em;
}
a.paginate {
border: 1px solid #000080;
padding: 2px 6px 2px 6px;
text-decoration: none;
color: #000080;
}
h2 {
font-size: 12pt;
color: #003366;
}
h2 {
line-height: 1.2em;
letter-spacing:-1px;
margin: 0;
padding: 0;
text-align: left;
}
a.paginate:hover {
background-color: #FCF;
color: #FFF;
text-decoration: underline;
}
a.current {
border: 1px solid #000080;
font: bold .7em Arial,Helvetica,sans-serif;
padding: 2px 6px 2px 6px;
cursor: default;
background:#FCF;
color: #FFF;
text-decoration: none;
}
span.inactive {
border: 1px solid #999;
font-family: Arial, Helvetica, sans-serif;
font-size: .7em;
padding: 2px 6px 2px 6px;
color: #999;
cursor: default;
}
-->
</style>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title></title>
</head>
<body>
<?php
include("db_conn.inc.php");
$strSQL = "SELECT * FROM tb_pr";
$objQuery = mysql_query($strSQL)or die ("Error Query [".$strSQL."]");
$Num_Rows = mysql_num_rows($objQuery);
$Per_Page = 5;
$Page = isset($_GET['PagePR']) ? $_GET['PagePR'] : 1;
$Prev_Page = $Page-1;
$Next_Page = $Page+1;
$Page_Start = (($Per_Page*$Page)-$Per_Page);
if($Num_Rows<=$Per_Page)
{
$Num_Pages =1;
}
else if(($Num_Rows % $Per_Page)==0)
{
$Num_Pages =($Num_Rows/$Per_Page);
}
else
{
$Num_Pages =($Num_Rows/$Per_Page)+1;
$Num_Pages = (int)$Num_Pages;
}
$strSQL .= " ORDER BY id DESC LIMIT $Page_Start , $Per_Page";
$objQuery = mysql_query($strSQL)or die ("Error Query [".$strSQL."]");
?>
<br />
<?php
$news_item=0;
while($objResult = mysql_fetch_assoc($objQuery))
{
$pr_send = $objResult["id"];
?>
<ul>
<li>
<a href="index2.php?page=detailPR&id_pr=<?=$pr_send;?>" target="_blank"><?=$objResult["Topic"];?></a>
<br>
<?=date("d-m-Y H:i:s",strtotime($objResult["Date_Post"]));?>
<?php
$strSQL2 = "SELECT * FROM tb_pr WHERE id= '$objResult[id]' ";
$objQuery2 = mysql_query($strSQL2)or die(mysql_error());
$objResult2 = mysql_fetch_array($objQuery2);
$strDateAdd = date('Y-m-d',strtotime("-7 day"));
if($objResult["Date_Post"]>=$strDateAdd)
{
echo "<img src=\"img/new.gif\"> ";
}
?>
</li>
</ul>
<?php
}
?>
<table width="550" border="0" cellspacing="0">
<tr>
<td width="562" align="center" class="table">
<?php
$pages = new Paginator;
$pages->items_total = $Num_Rows;
$pages->mid_range = 3;
$pages->current_page = $Page;
$pages->default_ipp = $Per_Page;
$pages->url_next = $_SERVER["PHP_SELF"]."?live=home&QueryString=value&PagePR=";
$pages->paginate();
echo $pages->display_pages();
?>
</td>
</tr>
</table>
<?php
mysql_close();
?>
</body>
</html>
Tag : PHP, MySQL

|
ประวัติการแก้ไข 2014-01-17 13:00:57 2014-01-17 13:02:02 2014-01-17 13:05:35
|
 |
 |
 |
 |
Date :
2014-01-17 12:59:51 |
By :
angelkiller9 |
View :
1703 |
Reply :
5 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมทำตามในเว็บนี้แล้ว กด previous มีปัญหาเหมือนกันครับ
อยากได้แบบนำมาใช้ได้จริง
ขอบคุณก๊าบ
|
 |
 |
 |
 |
Date :
2014-01-17 14:21:01 |
By :
angelkiller9 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 2 เขียนโดย : arm8957 เมื่อวันที่ 2014-01-17 21:42:04
รายละเอียดของการตอบ ::
ปรากฏว่า ผมทดลองใช้แล้วมันไม่ได้อ่ะครับทำไงอ่า
ผมมีไฟล์ kgPager.class.php แล้วนะครับ ทำตาม ลิ้ง https://www.thaicreate.com/community/php-mysql-split-page-with-kgpager-class.html.html
Code (PHP)
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Pagingation</title>
<style type="text/css">
body { font:0.8em/1.8em tahoma, helvetica, sans serif; color#333; }
#pager_links a { text-decoration:none; color:#ff3300; background:#fff; border:1px solid #e0e0e0; padding:1px 4px 1px 4px; margin:2px; }
#pager_links a:hover { text-decoration:none; color:#3399ff; background:#f2f2f2; border:1px solid #3399ff; padding:1px 4px 1px 4px; margin:2px; }
#current_page { border:1px solid #333; padding:1px 4px 1px 4px; margin:2px; color:#333; }
</style>
</head>
<body>
<?php
$objConnect = mysql_connect("127.0.0.1","","") or die("Error Connect to Database");
$objDB = mysql_select_db("apr");
mysql_query("SET NAMES 'utf8'");
error_reporting(0);
require_once('kgPager.class.php');
(empty($_GET['page']))? $page = 1: $page = $_GET['page'];
$query = "SELECT * FROM tb_pr";
$sql = mysql_query($query) or die ("Error Query [".$query."]");
$Num_Rows = mysql_num_rows($objQuery);
$scroll_page = 3;//จำนวนแบ่งหน้าที่จะให้แสดง คือ <ก่อนหน้า , 1 , 2 , 3 , ต่อไป>
$per_page = 5;//จำนวนข้อมูลที่ต้องการแสดงในแต่ละหน้า
$current_page = $page; //รับค่าเพจ
$pager_url = 'pagging.php?page='; //URL ตามตัวครับ
$inactive_page_tag = 'id="current_page"'; //ตำแหน่งหน้าปัจจุบัน ไม่ต้องระบุค่าอะไรทั้งนั้น
$previous_page_text = '< ย้อนกลับ'; //ปุ่มย้อนกลับ จะใส่ตัวหนังสือหรือภาพก็ได้ตามสไตล์
$next_page_text = 'ถัดไป >'; //ปุ่มถัดไป จะใส่ตัวหนังสือหรือภาพก็ได้ตามสไตล์
$first_page_text = '<< หน้าแรก'; //ปุ่มหน้าแรก
$last_page_text = 'หน้าสุดท้าย >>'; //ปุ่มหน้าสุดท้าย
$kgPagerOBJ = new KgPager(); #เรียกใช้งาน class
$kgPagerOBJ -> pager_set($pager_url, $total_records, $scroll_page, $per_page, $current_page, $inactive_page_tag, $previous_page_text, $next_page_text, $first_page_text, $last_page_text, $pager_url_last);
#-------------ส่วนแสดงผลจำนวน record ทั้งหมด--------------#
echo '<p><strong>Toplam Sayfa :</strong>';
echo $kgPagerOBJ -> total_pages;
echo '</p>';
#-------------จบส่วนแสดงผลจำนวน record ทั้งหมด--------------#
$sql = mysql_query($query." ORDER BY TopicID ASC LIMIT ".$kgPagerOBJ -> start.", ".$kgPagerOBJ -> per_page."");
?>
<table width="600" border="1">
<tr>
<th width="50"> <div align="center">TopicID </div></th>
<th width="534"> <div align="center">Topic </div></th>
</tr>
<?php
while($objResult = mysql_fetch_assoc($sql))
{
?>
<tr>
<td><div align="center"><?=$objResult["TopicID"];?></div></td>
<td><?=$objResult["Topic"];?></td>
</tr>
<?php
}
?>
</table>
<br>
<?php
#------ตัวแบ่งหน้า คือ <ก่อนหน้า , 1 , 2 , 3 , ต่อไป> จะเอาไปแปะตรงไหนก็ได้ในหน้าเพจ----#
echo '<p id="pager_links">';
echo $kgPagerOBJ -> first_page;
echo $kgPagerOBJ -> previous_page;
echo $kgPagerOBJ -> page_links;
echo $kgPagerOBJ -> next_page;
echo $kgPagerOBJ -> last_page;
echo '</p>';
?>
<?php
mysql_close($objConnect);
?>
</body>
</html>
มันก็ยังไม่ได้ครับ ตามรูป ไม่มีอะไรขึ้นเรย :'(
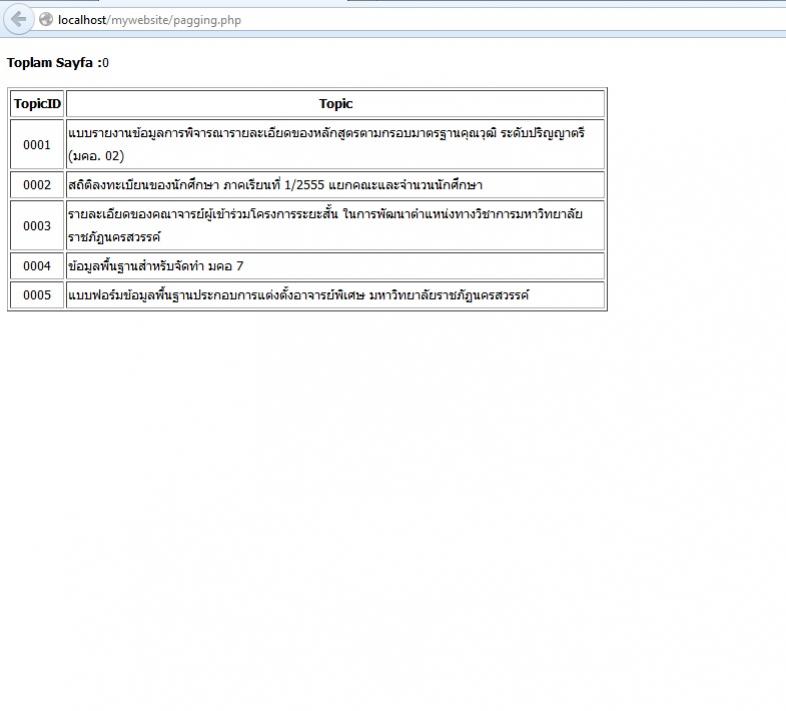
|
 |
 |
 |
 |
Date :
2014-01-21 13:57:29 |
By :
angelkiller9 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เอ่อออ ได้ละครับ ผมตั้งตัวแปรผิด อิอิ ขอโต๊ดค๊าบบบ
|
 |
 |
 |
 |
Date :
2014-01-21 14:22:28 |
By :
angelkiller9 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 05
|