 |
รบกวนถามเรื่อง auto complete ด้วยค่ะ คือตอนนี้ ทำ auto complete ที่ดึงมาจากฐานข้อมูลค่ะ ตอนที่ ดึงข้อมูลมา มันมาทั้ง ฟิลด์ค่ะ มันไม่เอาแค่คำมาค่ะ ต้องทำอย่างไรค่ะ |
|
 |
|
|
 |
 |
|
มีตัวอย่าง code ที่เขียนไว้ไหมครับ
|
 |
 |
 |
 |
Date :
2014-02-10 20:27:55 |
By :
FreshyMusiC |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|

|
 |
 |
 |
 |
Date :
2014-02-11 07:42:44 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (PHP)
<?php
header("Content-type:application/json; charset=UTF-8");
header("Cache-Control: no-store, no-cache, must-revalidate");
header("Cache-Control: post-check=0, pre-check=0", false);
// ส่วนของการเชิ่อมต่อกับฐานข้อมูล
mysql_connect("localhost","root","1234") or die("Cannot connect the Server");
mysql_select_db("mh") or die("Cannot select database");
mysql_query("set character set utf8");
?>
<?php
$pagesize = 50; // จำนวนรายการที่ต้องการแสดง
$table_db="med"; // ตารางที่ต้องการค้นหา
$find_field="keyword"; // ฟิลที่ต้องการค้นหา
if($_GET['term']!=""){
$q = $_GET["term"];
$sql = "select * from $table_db where locate('$q', $find_field) > 0 order by locate('$q', $find_field), $find_field limit $pagesize";
}else{
$sql = "select * from $table_db where 1";
}
$qr=mysql_query($sql);
$total=mysql_num_rows($qr);
echo '[ ';
$i=0;
while ($rs=mysql_fetch_array($qr)) {
$i++;
echo '{"id":"'.$rs['medID'].'","label":"'.str_replace(""","",htmlentities($rs['keyword'], ENT_QUOTES, "UTF-8")).'","value":"'.str_replace(""","",htmlentities($rs['treat'], ENT_QUOTES, "UTF-8")).'"}';
if($i<$total){
echo ",";
}
}
echo ' ]';
mysql_close();
exit;
?>
-- นี้ค่ะ
|
 |
 |
 |
 |
Date :
2014-02-11 14:21:16 |
By :
stampbnp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
รบกวนด้วยค่ะ
|
 |
 |
 |
 |
Date :
2014-02-12 17:44:59 |
By :
stampbnp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมเข้าใจหลักการ auto complete นะ .... เมื่อเราใส่อักษรตัวแรกเข้าไป มันจะเข้าไปค้นหาข้อมูล เมื่อเราเพิ่มตัวอักษรไปเรื่อย ๆ ข้อมูลก็จะโชว์ให้เราเลือกน้อยลง....
แต่คำว่ามาทั้ง ฟิลด์ มันหมายถึงอย่างไร มีรูปปลากรอบไหม??
ยิ่งถ้าเอา Code จากเว็บบนี้ไปใช้ ผมยืนยันว่าไม่เคยพลาด!!
|
 |
 |
 |
 |
Date :
2014-02-12 19:13:13 |
By :
apisitp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อันนี้ เป็นตัวอย่าง ผลลัพธ์ ที่ได้ตอนนี้ค่ะ
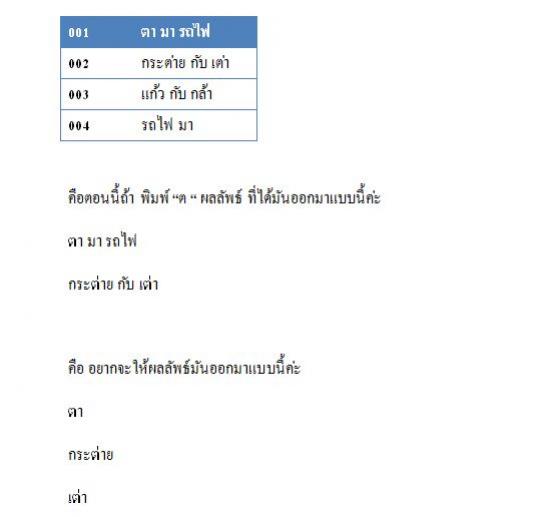
|
 |
 |
 |
 |
Date :
2014-02-13 01:17:08 |
By :
stampbnp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
รบกวนด้วยยนะค่ะ
|
 |
 |
 |
 |
Date :
2014-02-13 01:22:25 |
By :
stampbnp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
- query ตามเดิมก่อน
- explode คำออกมาตามการเว้นวรรค (explode(" ", $result))
- foreach แล้วใช้ strpos หาว่ามีคำมั้ย ถ้ามีก็แสดง ไม่มีก็ไม่ต้อง
|
 |
 |
 |
 |
Date :
2014-02-13 06:45:33 |
By :
itpcc |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
พี่ค่ะ รบกวนทำตัวอย่างให้ดูได้ไหมค่ะ ไม่เป็นจริงๆค่ะ
|
 |
 |
 |
 |
Date :
2014-02-13 20:57:12 |
By :
stampbnp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มันจะดีนะ 555+
|
 |
 |
 |
 |
Date :
2014-02-14 00:13:26 |
By :
apisitp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
หนูขอบคุณ พี่ทุกคนที่เข้ามาตอบจริงๆค่ะ หนูทำงานได้เพราะ พี่ๆในเว็บนี้เลย สุดยอดๆ
|
 |
 |
 |
 |
Date :
2014-02-14 12:05:43 |
By :
stampbnp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|