|
 |
|
สอบถามเรื่องการดึงข้อมูลจากฐานข้อมูลมาใส่ใน pdf |
|
 |
|
|
 |
 |
|
คือผมทำรายงาน เป็น pdf แต่จะดึงข้อมูลมาใส่ในรายงาน
เช่น report deposit of นายมานะ อดทน
แต่ผลลัพท์ที่ได้ report deposit "$eachResult["Name_Account"]"
ช่วยแนะนำด้วยคัฟ
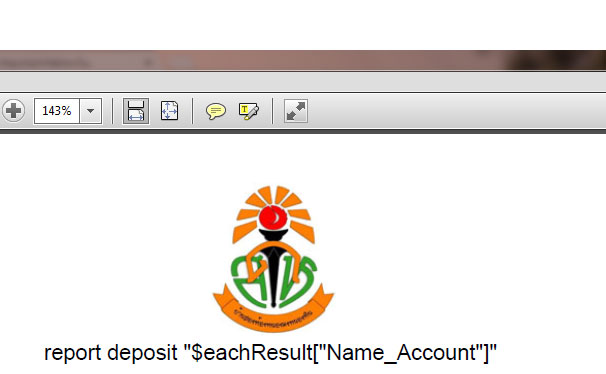
Code (PHP)
//*** Table 1 ***//
$pdf->AddPage();
$pdf->Image('pdf/logo.jpg',95,8,20); //ขนาดรูปภาพ 90 = ห่างจากขอบกระดาษ 20 = ขนาดของรูป
$pdf->Ln(25);
$pdf->Cell(0,1,'report deposit "$eachResult["Name_Account"]" ',0,1,"C");
$pdf->Ln(5);
$pdf->BasicTable($header,$resultData);
$pdf->Output("pdf/MyPDF/show-deposit.pdf","F");
Tag : PHP

|
|
 |
 |
 |
 |
Date :
2014-02-28 11:41:27 |
By :
kunnawut |
View :
905 |
Reply :
13 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (PHP)
$pdf->Cell(0,1,'report deposit "'.$eachResult["Name_Account"].'"',0,1,"C");
ลองดูครับ ผมก็ไม่ได้ใช้นานแล้ว
|
 |
 |
 |
 |
Date :
2014-02-28 12:11:44 |
By :
Nickkies |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
คุณ wut ต้อง Query ข้อมูล ใส่ตัวแปรนี้ก่อนนะครับ $eachResult["Name_Account"] มันถึงจะแสดงผลขึ้นมา
เช่น
Code (PHP)
$eachResult["Name_Account"] = "นายมานะ อดทน";
$pdf->Cell(0,1,'report deposit "'.$eachResult["Name_Account"].'"',0,1,"C");
|
 |
 |
 |
 |
Date :
2014-02-28 14:40:31 |
By :
Nickkies |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เอาไปใส่แบบนั้น มันก็มองเป็น ข้อความธรรมดาอยู่เเล้วล่ะครับ
|
 |
 |
 |
 |
Date :
2014-02-28 14:41:07 |
By :
FreshyMusiC |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
05.$pdf->Cell(0,1,'report deposit "{$eachResult["Name_Account"]}" ',0,1,"C");
|
 |
 |
 |
 |
Date :
2014-02-28 14:46:23 |
By :
sakuraei |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (PHP)
<?php
require('fpdf.php');
$data="This is testing";
$pdf=new FPDF();
$pdf->AddPage();
$pdf->SetFont('Times','B',16);
$pdf->Cell(0,10,'Welcome to www.ThaiCreate.Com',0,1);
$pdf->Cell(0,20,'Version 2009',0,1,"C");
$pdf->Cell(0,20,"test \$data: \"{$data}\" ",0,1,"C");
$pdf->Output();
?>
|
ประวัติการแก้ไข 2014-02-28 16:22:10
 |
 |
 |
 |
Date :
2014-02-28 16:21:42 |
By :
dekkuza |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
$eachResult["Name_Account"] คืออะไรครับ ลอง
echo $eachResult["Name_Account"] ;
exit;
ดูว่ามันดึงข้อมูลมายัง จะได้ไม่ตกม้าตาย
|
ประวัติการแก้ไข 2014-02-28 16:25:21
 |
 |
 |
 |
Date :
2014-02-28 16:24:52 |
By :
dekkuza |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ได้แล้วคัฟ
ขอบคุณทุกท่านนะคัฟ

Code (PHP)
include "connect.php";
$strSQL = "SELECT * FROM deposit WHERE ID_Account = '".$_SESSION['ID_Account']."' ";
mysql_query("SET NAMES TIS620");
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
$Name_Account = $objResult["Name_Account"];
echo $Name_Account;
//*** Table 1 ***//
$pdf->AddPage();
$pdf->AddFont('angsa','','angsa.php');
$pdf->SetFont('angsa','',14);
$pdf->Image('pdf/logo.jpg',95,8,20); //ขนาดรูปภาพ 90 = ห่างจากขอบกระดาษ 20 = ขนาดของรูป
$pdf->Ln(25);
$pdf->Cell(0,1,'รายงานการฝากเงินของ '.$Name_Account.' ',0,1,"C");
$pdf->Ln(5);
$pdf->Cell(0,1,'จากวันที่ '.$_GET['dateInput'].' ถึงวันที่ '.$_GET['dateInput2'].' ',0,1,"C");
$pdf->Ln(5);
$pdf->BasicTable($header,$resultData);
$pdf->Output("pdf/MyPDF/show-deposit.pdf","F");
|
 |
 |
 |
 |
Date :
2014-02-28 17:01:00 |
By :
kunnawut |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอถามอีกนิดนะคัฟ
1. คือว่า ลำดับที่ มันไม่เริ่มนับที่ 1 คัฟ มันเริ่มที่ 5,6,7
2, จะจัดตารางให้อยู่กลางหน้ากระดาษยังไงคัฟ
ช่วยแนะนำด้วยคัฟ
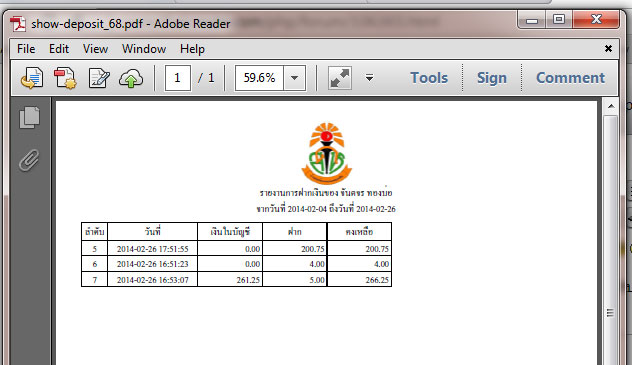
Code (PHP)
<?php
require('pdf/fpdf.php');
class PDF extends FPDF
{
//Load data
function LoadData($file)
{
//Read file lines
$lines=file($file);
$data=array();
foreach($lines as $line)
$data[]=explode(';',chop($line));
return $data;
}
//Simple table
function BasicTable($header,$data)
{
//Header
$w=array(10,35,25,25,25);
//Header
for($i=0;$i<count($header);$i++)
$this->Cell($w[$i],7,$header[$i],1,0,'C');
$this->Ln();
//Data
foreach ($data as $eachResult)
{
$this->Cell(10,6,$i++,1,0,'C');
$this->Cell(35,6,$eachResult["Date_Time"],1,0,'C');
$this->Cell(25,6,number_format($eachResult["Money_Old"],2),1,0,'R');
$this->Cell(25,6,number_format($eachResult["Deposit"],2),1,0,'R');
$this->Cell(25,6,number_format($eachResult["Balance"],2),1,0,'R');
$this->Ln();
}
}
//Colored table
function FancyTable($header,$data)
{
//Colors, line width and bold font
$this->SetFillColor(255,0,0);
$this->SetTextColor(255);
$this->SetDrawColor(128,0,0);
$this->SetLineWidth(.3);
$this->SetFont('','B');
//Header
$w=array(10,35,25,25,25);
for($i=0;$i<count($header);$i++)
$this->Cell($w[$i],7,$header[$i],1,0,'C',true);
$this->Ln();
//Color and font restoration
$this->SetFillColor(224,235,255);
$this->SetTextColor(0);
$this->SetFont('');
//Data
$fill=false;
foreach($data as $row)
{
$this->Cell($w[0],6,$row[0],'LR',0,'C',$fill);
$this->Cell($w[1],6,$row[1],'LR',0,'C',$fill);
$this->Cell($w[2],6,number_format($row[2]),'LR',0,'R',$fill);
$this->Cell($w[3],6,number_format($row[3]),'LR',0,'R',$fill);
$this->Cell($w[4],6,number_format($row[4]),'LR',0,'R',$fill);
$this->Ln();
$fill=!$fill;
}
$this->Cell(array_sum($w),0,'','T');
}
}
$pdf=new PDF();
//Column titles
$header=array('ลำดับ','วันที่','เงินในบัญชี','ฝาก','คงเหลือ');
//Data loading
//*** Load MySQL Data ***//
include"connect.php";
$strSQL = "SELECT * FROM deposit WHERE Date2 BETWEEN '".$_GET['dateInput']."' and '".$_GET['dateInput2']."' order by ID ASC ";
mysql_query("SET NAMES TIS620");
$objQuery = mysql_query($strSQL);
$resultData = array();
for ($i=0;$i<mysql_num_rows($objQuery);$i++) {
$result = mysql_fetch_array($objQuery);
array_push($resultData,$result);
}
//************************//
define('FPDF_FONTPATH','font/');
include "connect.php";
$strSQL = "SELECT * FROM deposit WHERE ID_Account = '".$_SESSION['ID_Account']."' ";
mysql_query("SET NAMES TIS620");
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
$Name_Account = $objResult["Name_Account"];
//*** Table 1 ***//
$pdf->AddPage();
$pdf->AddFont('angsa','','angsa.php');
$pdf->SetFont('angsa','',14);
$pdf->Image('pdf/logo.jpg',95,8,20); //ขนาดรูปภาพ 90 = ห่างจากขอบกระดาษ 20 = ขนาดของรูป
$pdf->Ln(25);
$pdf->Cell(0,1,'รายงานการฝากเงินของ '.$Name_Account.' ',0,1,"C");
$pdf->Ln(5);
$pdf->Cell(0,1,'จากวันที่ '.$_GET['dateInput'].' ถึงวันที่ '.$_GET['dateInput2'].' ',0,1,"C");
$pdf->Ln(5);
$pdf->BasicTable($header,$resultData);
$pdf->Output("pdf/MyPDF/show-deposit.pdf","F");
?>
|
 |
 |
 |
 |
Date :
2014-02-28 17:10:02 |
By :
kunnawut |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ไม่แน่ใจเท่าใดนักแต่ก็ลองดู
Code (PHP)
$fill=false;
int ixx=1;
foreach($data as $row)
{
$this->Cell($w[0],6,$ixx,'LR',0,'C',$fill);
$this->Cell($w[1],6,$row[1],'LR',0,'C',$fill);
$this->Cell($w[2],6,number_format($row[2]),'LR',0,'R',$fill);
$this->Cell($w[3],6,number_format($row[3]),'LR',0,'R',$fill);
$this->Cell($w[4],6,number_format($row[4]),'LR',0,'R',$fill);
$this->Ln();
$fill=!$fill;
ixx++;
}
$this->Cell(array_sum($w),0,'','T');
|
 |
 |
 |
 |
Date :
2014-02-28 19:25:17 |
By :
dekkuza |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ไม่ error ก็แปลก int ixx=1; ลืม $ ต้องเป็น int $ixx=1; แต่ผมว่าคุณคงทำได้แล้ว
|
 |
 |
 |
 |
Date :
2014-03-01 12:15:42 |
By :
dekkuza |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Quote:018.//Simple table
019.function BasicTable($header,$data)
020.{
021.//Header
022.$w=array(10,35,25,25,25);
023.//Header
024.for($i=0;$i<count($header);$i++)
025.$this->Cell($w[$i],7,$header[$i],1,0,'C');
026.$this->Ln();
027.//Data
$i = 1;
028.foreach ($data as $eachResult)
029.{
030.$this->Cell(10,6,$i++,1,0,'C');
031.$this->Cell(35,6,$eachResult["Date_Time"],1,0,'C');
032.$this->Cell(25,6,number_format($eachResult["Money_Old"],2),1,0,'R');
033.$this->Cell(25,6,number_format($eachResult["Deposit"],2),1,0,'R');
034.$this->Cell(25,6,number_format($eachResult["Balance"],2),1,0,'R');
035.$this->Ln();
036.}
037.}
|
 |
 |
 |
 |
Date :
2014-03-01 12:42:42 |
By :
sakuraei |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 02
|