|
 |
|
สอบถามปัญหาครับ เพิ่มรายการรายละเอียดการซ์้อเข้าไม่ได้ครับ |
|
 |
|
|
 |
 |
|
หน้ารายละเอียดซ์้อเข้า (Purchase_detail.php) โดยหน้านี้เป็นของ product_id=1 ครับ
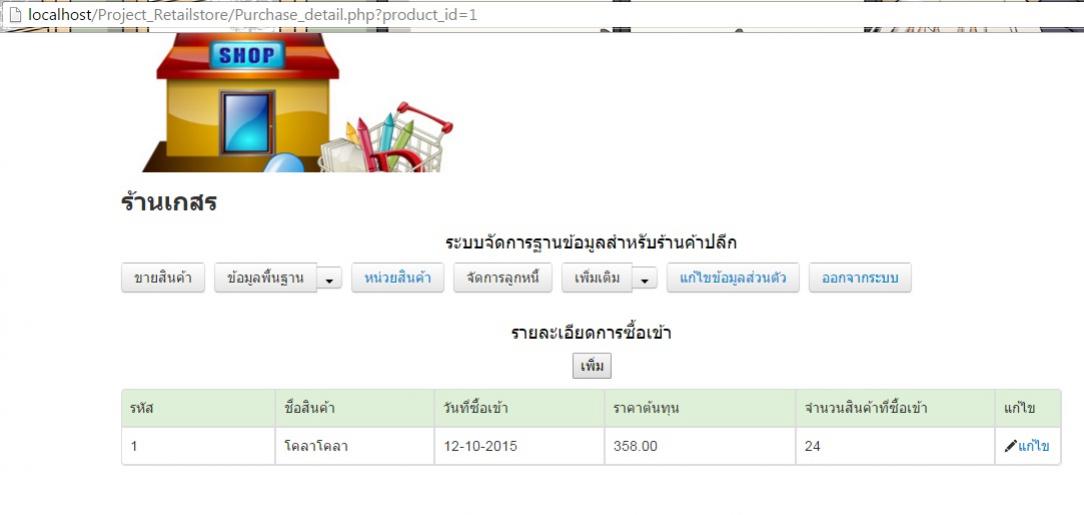
ส่วนอันนี้คือหน้าเพิ่มรายละเอียดซ์้อเข้าครับ (addpurchase_detail.php)
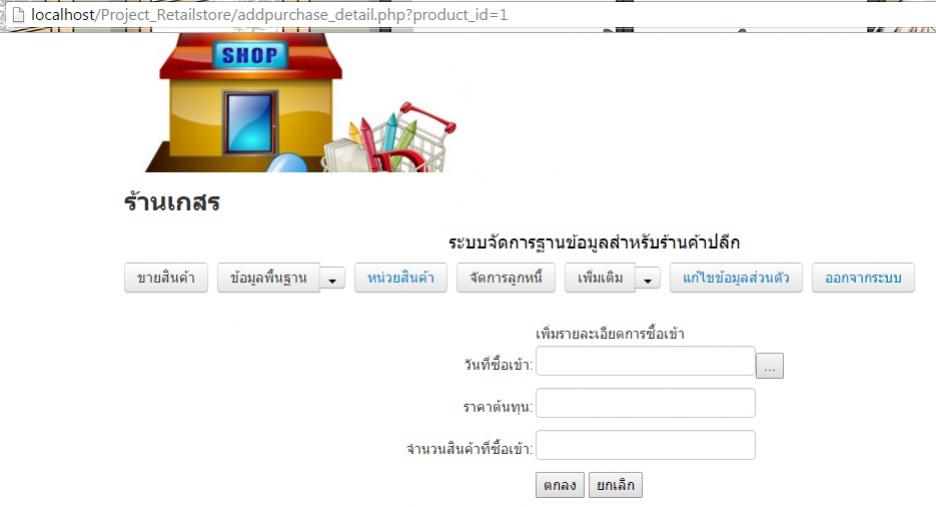
ลองเพิ่มข้อมูลที่ 2 ของรายละเอียดซ์้อเข้า product_id=1 ครับ
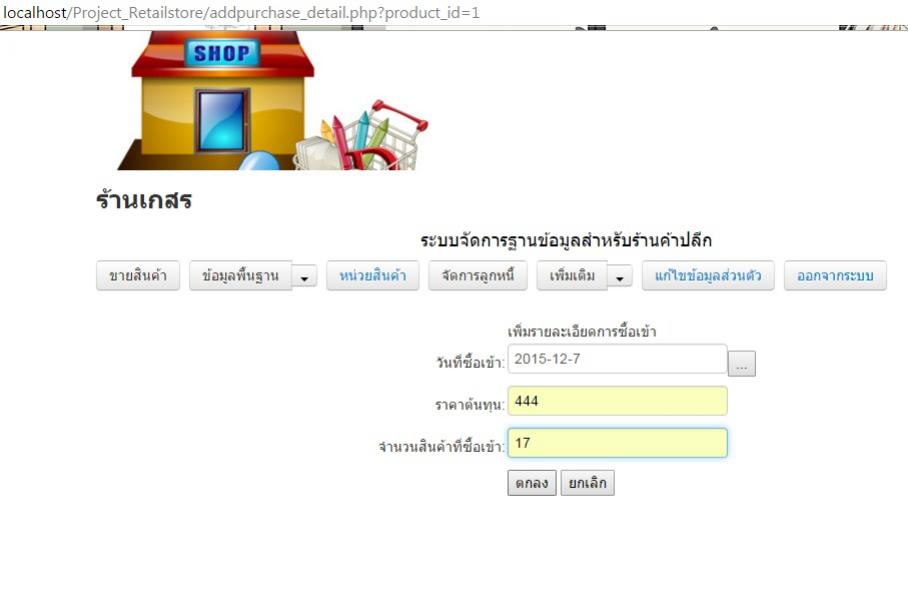
ส่วนนี้คือปัญหาครับ เพิ่มข้อมูลที่ 2 ไปแล้ว ข้อมูลไม่ยอม show ครับและในฐานข้อมูล purchase_detail
ข้อมูลที่ 2 ตรงproduct_id เป็น 0 เฉยเลยครับ
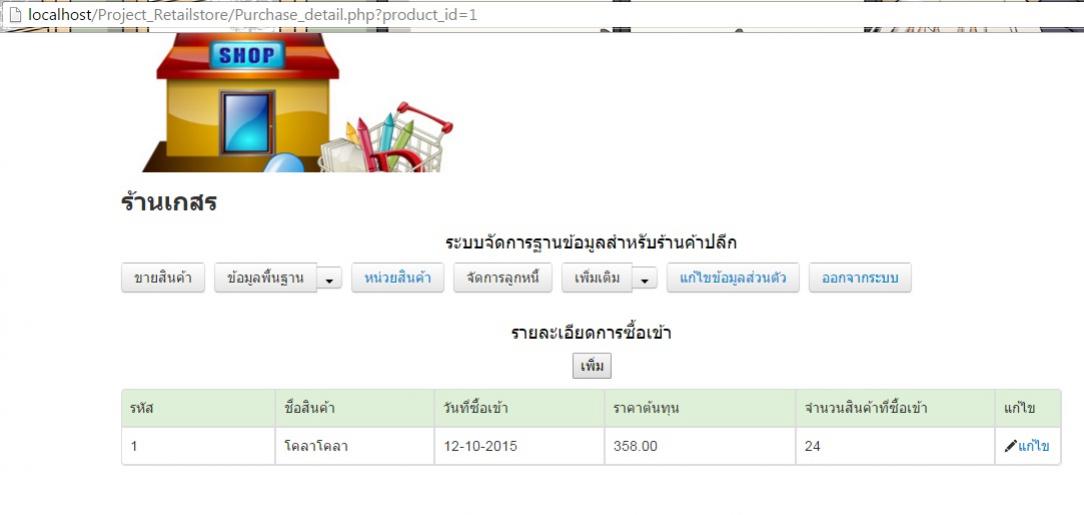
pur_detail_id เป็น PK และ product_id เป็น FK ครับ
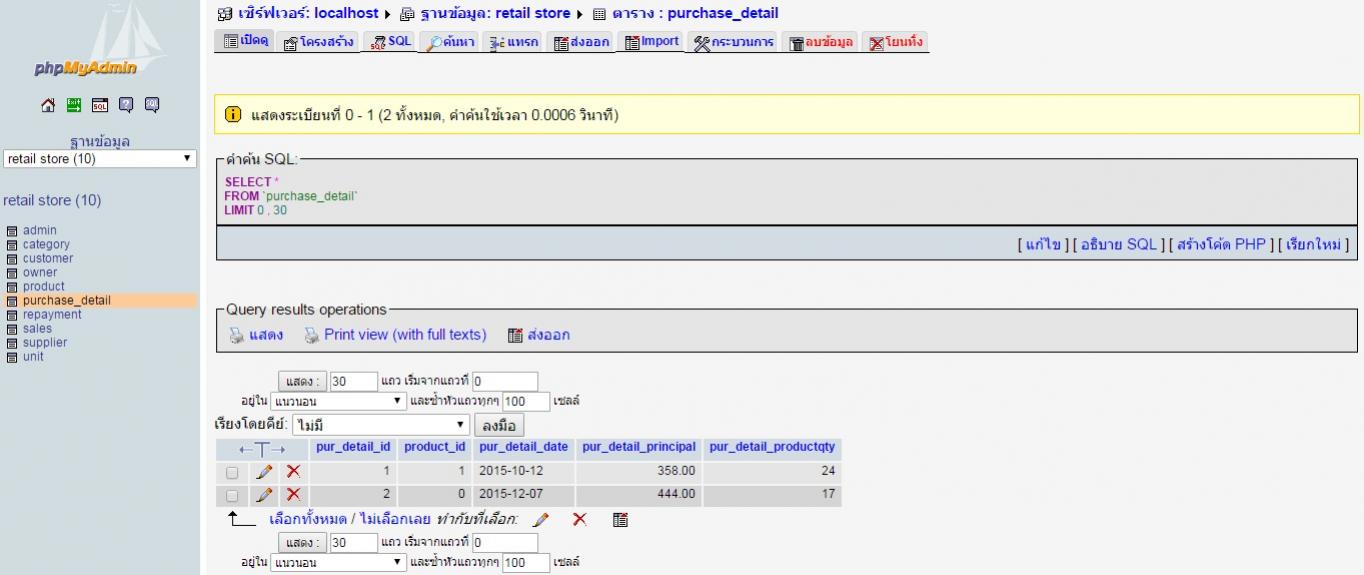
code Purchase_detail.php
Code (PHP)
<?php require_once('Connections/MyConnect.php');
mysql_select_db($database_MyConnect, $MyConnect);
$pd=intval($_REQUEST['product_id']);
$SQL="select
pur_detail_id id,
product_name name,
DATE_FORMAT(pur_detail_date, '%d-%m-%Y') pur_date,
pur_detail_principal pur_princ,
pur_detail_productqty product_qty
from
purchase_detail INNER JOIN product
ON purchase_detail.pur_detail_id = product.product_id
WHERE product.product_id=$pd
";
$QUERY= mysql_query($SQL) or die ("Error Query [".$SQL."]".mysql_error());
$sql .= "ORDER BY `product_id` ASC ";
$sdl .= "$i=1;
while($i<=5) condition";
$i++;
?>
<?php
if (!isset($_SESSION)) {
session_start();
}
$MM_authorizedUsers = "";
$MM_donotCheckaccess = "true";
// *** Restrict Access To Page: Grant or deny access to this page
function isAuthorized($strUsers, $strGroups, $UserName, $UserGroup) {
// For security, start by assuming the visitor is NOT authorized.
$isValid = False;
// When a visitor has logged into this site, the Session variable MM_Username set equal to their username.
// Therefore, we know that a user is NOT logged in if that Session variable is blank.
if (!empty($UserName)) {
// Besides being logged in, you may restrict access to only certain users based on an ID established when they login.
// Parse the strings into arrays.
$arrUsers = Explode(",", $strUsers);
$arrGroups = Explode(",", $strGroups);
if (in_array($UserName, $arrUsers)) {
$isValid = true;
}
// Or, you may restrict access to only certain users based on their username.
if (in_array($UserGroup, $arrGroups)) {
$isValid = true;
}
if (($strUsers == "") && true) {
$isValid = true;
}
}
return $isValid;
}
$MM_restrictGoTo = "admin/index.php";
if (!((isset($_SESSION['MM_Username'])) && (isAuthorized("",$MM_authorizedUsers, $_SESSION['MM_Username'], $_SESSION['MM_UserGroup'])))) {
$MM_qsChar = "?";
$MM_referrer = $_SERVER['PHP_SELF'];
if (strpos($MM_restrictGoTo, "?")) $MM_qsChar = "&";
if (isset($_SERVER['QUERY_STRING']) && strlen($_SERVER['QUERY_STRING']) > 0)
$MM_referrer .= "?" . $_SERVER['QUERY_STRING'];
$MM_restrictGoTo = $MM_restrictGoTo. $MM_qsChar . "accesscheck=" . urlencode($MM_referrer);
header("Location: ". $MM_restrictGoTo);
exit;
}
?>
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$maxRows_purchaseset1 = 5;
$pageNum_purchaseset1 = 0;
if (isset($_GET['pageNum_purchaseset1'])) {
$pageNum_purchaseset1 = $_GET['pageNum_purchaseset1'];
}
$startRow_purchaseset1 = $pageNum_purchaseset1 * $maxRows_purchaseset1;
$query_purchaseset1 = "SELECT * FROM purchase_detail";
$query_limit_purchaseset1 = sprintf("%s LIMIT %d, %d", $query_purchaseset1, $startRow_purchaseset1, $maxRows_purchaseset1);
$purchaseset1 = mysql_query($query_limit_purchaseset1, $MyConnect) or die(mysql_error());
$row_purchaseset1 = mysql_fetch_assoc($purchaseset1);
if (isset($_GET['totalRows_purchaseset1'])) {
$totalRows_purchaseset1 = $_GET['totalRows_purchaseset1'];
} else {
$all_purchaseset1 = mysql_query($query_purchaseset1);
$totalRows_purchaseset1 = mysql_num_rows($all_purchaseset1);
}
$totalPages_purchaseset1 = ceil($totalRows_purchaseset1/$maxRows_purchaseset1)-1;
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>purchase_detail</title>
<script src="js/jquery.js" type="text/javascript"></script>
<link href="css/bootstrap.min.css" rel="stylesheet" type="text/css" />
</head>
<style type="text/css">
#MenuBar1 {
font-size: 230%;
font-family: "Courier New", Courier, monospace;
}
body p {
font-size: 18px;
text-align: center;
color: #000;
}
body h2 {
text-align: center;
}
</style>
<body class='container'>
<p><img src="shop.jpg" width="1117" height="149" />
</p>
<h3>ร้านเกสร
</h3>
<p>ระบบจัดการฐานข้อมูลสำหรับร้านค้าปลีก</p>
<div class="btn-group">
<button class="btn">ขายสินค้า</button>
</div>
<div class="btn-group">
<button class="btn">ข้อมูลพื้นฐาน</button>
<button class="btn dropdown-toggle" data-toggle="dropdown">
<span class="caret"></span>
</button>
<ul class="dropdown-menu">
<li><a href="Product.php">ข้อมูลสินค้า</a></li>
<li><a href="Category.php">ข้อมูลประเภทสินค้า</a></li>
<li><a href="Supplier.php">ข้อมูลผู้จัดจำหน่าย</a></li>
<!-- dropdown menu links -->
</ul>
</div>
<div class="btn-group">
<button class="btn"><a href="Unit.php">หน่วยสินค้า</a></button>
</div>
<div class="btn-group">
<button class="btn">จัดการลูกหนี้</button>
</div>
<div class="btn-group">
<button class="btn">เพิ่มเติม</button>
<button class="btn dropdown-toggle" data-toggle="dropdown">
<span class="caret"></span>
</button>
<ul class="dropdown-menu">
<li><a href="#">ดูสินค้าใกล้หมด</a></li>
<li><a href="#">เช็คสต๊อกสินค้า</a></li>
<!-- dropdown menu links -->
</ul>
</div>
<div class="btn-group">
<button class="btn"><a href="edit-profile.php">แก้ไขข้อมูลส่วนตัว</a></button>
</div>
<div class="btn-group">
<button class="btn"><a href="admin/index.php">ออกจากระบบ</a></button>
</div>
<p> </p>
<p>รายละเอียดการซื้อเข้า</p>
<p>
<a href="addpurchase_detail.php?product_id=<?php echo $row_purchaseset1['product_id']; ?>">
<input type="submit" name="btn" id="btn" value="เพิ่ม" />
</a></p>
<table table class="table table-bordered" width="105%" border="1">
<tr class="success">
<td width="196">รหัส</td>
<td width="196">ชื่อสินค้า</td>
<td width="213">วันที่ซื้อเข้า</td>
<td width="238">ราคาต้นทุน</td>
<td width="252">จำนวนสินค้าที่ซื้อเข้า</td>
<td width="55">แก้ไข</td>
</tr>
<?php while($rs=mysql_fetch_array($QUERY)) { ?>
<tr>
<td><?php echo $rs['id']; ?></td>
<td><?php echo $rs['name']; ?></td>
<td><?php echo $rs['pur_date']; ?></td>
<td><?php echo $rs['pur_princ']; ?></td>
<td><?php echo $rs['product_qty']; ?></td>
<td><i class="icon-pencil"></i><a href="editpurchase_detail.php?pur_detail_id =<?php echo $rs['pur_detail_id']; ?>">แก้ไข</a></td>
</tr>
<?php } while ($row_purchaseset1 = mysql_fetch_assoc($purchaseset1)); ?>
</table>
<script src="js/jquery.js"></script>
<script src="js/bootstrap.min.js"></script>
</body>
</html>
<?php
mysql_free_result($purchaseset1);
?>
code addpurchase_detail.php
Code (PHP)
<?php require_once('Connections/MyConnect.php'); ?>
<?php
if (!isset($_SESSION)) {
session_start();
}
$MM_authorizedUsers = "";
$MM_donotCheckaccess = "true";
// *** Restrict Access To Page: Grant or deny access to this page
function isAuthorized($strUsers, $strGroups, $UserName, $UserGroup) {
// For security, start by assuming the visitor is NOT authorized.
$isValid = False;
// When a visitor has logged into this site, the Session variable MM_Username set equal to their username.
// Therefore, we know that a user is NOT logged in if that Session variable is blank.
if (!empty($UserName)) {
// Besides being logged in, you may restrict access to only certain users based on an ID established when they login.
// Parse the strings into arrays.
$arrUsers = Explode(",", $strUsers);
$arrGroups = Explode(",", $strGroups);
if (in_array($UserName, $arrUsers)) {
$isValid = true;
}
// Or, you may restrict access to only certain users based on their username.
if (in_array($UserGroup, $arrGroups)) {
$isValid = true;
}
if (($strUsers == "") && true) {
$isValid = true;
}
}
return $isValid;
}
$MM_restrictGoTo = "admin/index.php";
if (!((isset($_SESSION['MM_Username'])) && (isAuthorized("",$MM_authorizedUsers, $_SESSION['MM_Username'], $_SESSION['MM_UserGroup'])))) {
$MM_qsChar = "?";
$MM_referrer = $_SERVER['PHP_SELF'];
if (strpos($MM_restrictGoTo, "?")) $MM_qsChar = "&";
if (isset($_SERVER['QUERY_STRING']) && strlen($_SERVER['QUERY_STRING']) > 0)
$MM_referrer .= "?" . $_SERVER['QUERY_STRING'];
$MM_restrictGoTo = $MM_restrictGoTo. $MM_qsChar . "accesscheck=" . urlencode($MM_referrer);
header("Location: ". $MM_restrictGoTo);
exit;
}
?>
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$editFormAction = $_SERVER['PHP_SELF'];
if (isset($_SERVER['QUERY_STRING'])) {
$editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']);
}
if ((isset($_POST["MM_insert"])) && ($_POST["MM_insert"] == "form1")) {
$insertSQL = sprintf("INSERT INTO purchase_detail (pur_detail_date, pur_detail_principal, pur_detail_productqty) VALUES (%s, %s, %s)",
GetSQLValueString($_POST['pur_detail_date'], "date"),
GetSQLValueString($_POST['pur_detail_principal'], "double"),
GetSQLValueString($_POST['pur_detail_productqty'], "int"));
mysql_select_db($database_MyConnect, $MyConnect);
$Result1 = mysql_query($insertSQL, $MyConnect) or die(mysql_error());
$insertGoTo = "Purchase_detail.php";
if (isset($_SERVER['QUERY_STRING'])) {
$insertGoTo .= (strpos($insertGoTo, '?')) ? "&" : "?";
$insertGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $insertGoTo));
}
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>addpurchase_detail</title>
<?php
$jquery_ui_v="1.8.5";
$theme=array(
"0"=>"base",
"1"=>"black-tie",
"2"=>"blitzer",
"3"=>"cupertino",
"4"=>"dark-hive",
"5"=>"dot-luv",
"6"=>"eggplant",
"7"=>"excite-bike",
"8"=>"flick",
"9"=>"hot-sneaks",
"10"=>"humanity",
"11"=>"le-frog",
"12"=>"mint-choc",
"13"=>"overcast",
"14"=>"pepper-grinder",
"15"=>"redmond",
"16"=>"smoothness",
"17"=>"south-street",
"18"=>"start",
"19"=>"sunny",
"20"=>"swanky-purse",
"21"=>"trontastic",
"22"=>"ui-darkness",
"23"=>"ui-lightness",
"24"=>"vader"
);
$jquery_ui_theme=$theme[22];
?>
<link type="text/css" rel="stylesheet" href="http://ajax.googleapis.com/ajax/libs/jqueryui/<?=$jquery_ui_v?>/themes/<?=$jquery_ui_theme?>/jquery-ui.css" />
<style type="text/css">
/* ปรับขนาดตัวอักษรของข้อความใน tabs
สามารถปรับเปลี่ยน รายละเอียดอื่นๆ เพิ่มเติมเกี่ยวกับ tabs
*/
.ui-tabs{
font-family:tahoma;
font-size:11px;
}
</style>
<style type="text/css">
/* Overide css code กำหนดความกว้างของปฏิทินและอื่นๆ */
.ui-datepicker{
width:220px;
font-family:tahoma;
font-size:11px;
text-align:center;
}
</style>
<style type="text/css">
#MenuBar1 {
font-size: 230%;
font-family: "Courier New", Courier, monospace;
}
body p {
font-size: 18px;
text-align: center;
color: #000;
}
body h2 {
text-align: center;
}
</style>
<script src="js/jquery.js" type="text/javascript"></script>
<link href="css/bootstrap.min.css" rel="stylesheet" type="text/css" />
</head>
<body class='container'>
<p><img src="shop.jpg" width="1117" height="149" />
</p>
<h3>ร้านเกสร
</h3>
<p>ระบบจัดการฐานข้อมูลสำหรับร้านค้าปลีก</p>
<div class="btn-group">
<button class="btn">ขายสินค้า</button>
</div>
<div class="btn-group">
<button class="btn">ข้อมูลพื้นฐาน</button>
<button class="btn dropdown-toggle" data-toggle="dropdown">
<span class="caret"></span>
</button>
<ul class="dropdown-menu">
<li><a href="Product.php">ข้อมูลสินค้า</a></li>
<li><a href="Category.php">ข้อมูลประเภทสินค้า</a></li>
<li><a href="Supplier.php">ข้อมูลผู้จัดจำหน่าย</a></li>
<!-- dropdown menu links -->
</ul>
</div>
<div class="btn-group">
<button class="btn"><a href="Unit.php">หน่วยสินค้า</a></button>
</div>
<div class="btn-group">
<button class="btn">จัดการลูกหนี้</button>
</div>
<div class="btn-group">
<button class="btn">เพิ่มเติม</button>
<button class="btn dropdown-toggle" data-toggle="dropdown">
<span class="caret"></span>
</button>
<ul class="dropdown-menu">
<li><a href="#">ดูสินค้าใกล้หมด</a></li>
<li><a href="#">เช็คสต๊อกสินค้า</a></li>
<!-- dropdown menu links -->
</ul>
</div>
<div class="btn-group">
<button class="btn"><a href="edit-profile.php">แก้ไขข้อมูลส่วนตัว</a></button>
</div>
<div class="btn-group">
<button class="btn"><a href="admin/index.php">ออกจากระบบ</a></button>
</div>
<p> </p>
<script language="javascript">
function fncSubmit()
{
if(document.form1.pur_detail_date.value == "")
{
alert("กรุณากรอกข้อมูล วัน เดือน ปี");
document.form1.pur_detail_date.focus();
return false;
}
if(document.form1.pur_detail_principal.value == "")
{
alert("กรุณากรอกข้อมูลให้ครบ");
document.form1.pur_detail_principal.focus();
return false;
}
if(document.form1.pur_detail_productqty.value == "")
{
alert("กรุณากรอกข้อมูลให้ครบ");
document.form1.pur_detail_productqty.focus();
return false;
}
document.form1.submit();
}
</script>
<form action="<?php echo $editFormAction; ?>" method="post" name="form1" id="form1"onSubmit="JavaScript:return fncSubmit();">
<table align="center">
<tr valign="baseline">
<td nowrap="nowrap" align="right"> </td>
<td>เพิ่มรายละเอียดการซื้อเข้า</td>
</tr>
<tr valign="baseline">
<tr valign="baseline">
<td nowrap="nowrap" align="right">วันที่ซื้อเข้า:</td>
<td><input name="pur_detail_date" type="text" id="pur_detail_date" value="" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">ราคาต้นทุน:</td>
<td><input type="text" name="pur_detail_principal" onKeyUp="if(isNaN(this.value)){ alert('กรุณากรอกข้อมูลเป็นตัวเลขเท่านั้น'); this.value='';}" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">จำนวนสินค้าที่ซื้อเข้า:</td>
<td><input type="text" name="pur_detail_productqty" onKeyUp="if(isNaN(this.value)){ alert('กรุณากรอกข้อมูลเป็นตัวเลขเท่านั้น'); this.value='';}" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right"> </td>
<td><input type="submit" value="ตกลง" /> <input type="submit" name="btn" id="btn" value="ยกเลิก" /></td>
</tr>
</table>
<input type="hidden" name="MM_insert" value="form1" />
</form>
<p> </p>
<p> </p>
<script src="js/jquery.js"></script>
<script src="js/bootstrap.min.js"></script>
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script src="//ajax.googleapis.com/ajax/libs/jqueryui/1.8.5/jquery-ui.min.js"></script>
<script type="text/javascript">
$(function(){
var dateBefore=null;
$("#pur_detail_date").datepicker({
dateFormat: 'yy-mm-dd',
showOn: 'button',
// buttonImage: 'http://jqueryui.com/demos/datepicker/images/calendar.gif',
buttonImageOnly: false,
dayNamesMin: ['อา', 'จ', 'อ', 'พ', 'พฤ', 'ศ', 'ส'],
monthNamesShort: ['มกราคม','กุมภาพันธ์','มีนาคม','เมษายน','พฤษภาคม','มิถุนายน','กรกฎาคม','สิงหาคม','กันยายน','ตุลาคม','พฤศจิกายน','ธันวาคม'],
changeMonth: true,
changeYear: true,
beforeShow:function(){
if($(this).val()!=""){
var arrayDate=$(this).val().split("-");
arrayDate[2]=parseInt(arrayDate[2])-543;
$(this).val(arrayDate[0]+"-"+arrayDate[1]+"-"+arrayDate[2]);
}
setTimeout(function(){
$.each($(".ui-datepicker-year option"),function(j,k){
var textYear=parseInt($(".ui-datepicker-year option").eq(j).val())+543;
$(".ui-datepicker-year option").eq(j).text(textYear);
});
},50);
},
onChangeMonthYear: function(){
setTimeout(function(){
$.each($(".ui-datepicker-year option"),function(j,k){
var textYear=parseInt($(".ui-datepicker-year option").eq(j).val())+543;
$(".ui-datepicker-year option").eq(j).text(textYear);
});
},50);
},
onClose:function(){
if($(this).val()!="" && $(this).val()==dateBefore){
var arrayDate=dateBefore.split("-");
arrayDate[2]=parseInt(arrayDate[2])+543;
$(this).val(arrayDate[0]+"-"+arrayDate[1]+"-"+arrayDate[2]);
}
},
onSelect: function(dateText, inst){
dateBefore=$(this).val();
var arrayDate=dateText.split("-");
arrayDate[2]=parseInt(arrayDate[2])+543;
$(this).val(arrayDate[0]+"-"+arrayDate[1]+"-"+arrayDate[2]);
}
});
});
</script>
</body>
</html>
code Product.php
Code (PHP)
<?php require_once('Connections/MyConnect.php');
session_start();
mysql_select_db($database_MyConnect, $MyConnect);
$SQL="SELECT * FROM product
LEFT JOIN category ON (product.cate_id=category.cate_id)
LEFT JOIN unit ON (product.unit_id=unit.unit_id)";
$QUERY= mysql_query($SQL) or die ("Error Query [".$SQL."]".mysql_error());
$sql .= "ORDER BY `product_id` ASC ";
?>
<?php
if (!isset($_SESSION)) {
}
$MM_authorizedUsers = "";
$MM_donotCheckaccess = "true";
// *** Restrict Access To Page: Grant or deny access to this page
function isAuthorized($strUsers, $strGroups, $UserName, $UserGroup) {
// For security, start by assuming the visitor is NOT authorized.
$isValid = False;
// When a visitor has logged into this site, the Session variable MM_Username set equal to their username.
// Therefore, we know that a user is NOT logged in if that Session variable is blank.
if (!empty($UserName)) {
// Besides being logged in, you may restrict access to only certain users based on an ID established when they login.
// Parse the strings into arrays.
$arrUsers = Explode(",", $strUsers);
$arrGroups = Explode(",", $strGroups);
if (in_array($UserName, $arrUsers)) {
$isValid = true;
}
// Or, you may restrict access to only certain users based on their username.
if (in_array($UserGroup, $arrGroups)) {
$isValid = true;
}
if (($strUsers == "") && true) {
$isValid = true;
}
}
return $isValid;
}
$MM_restrictGoTo = "admin/index.php";
if (!((isset($_SESSION['MM_Username'])) && (isAuthorized("",$MM_authorizedUsers, $_SESSION['MM_Username'], $_SESSION['MM_UserGroup'])))) {
$MM_qsChar = "?";
$MM_referrer = $_SERVER['PHP_SELF'];
if (strpos($MM_restrictGoTo, "?")) $MM_qsChar = "&";
if (isset($_SERVER['QUERY_STRING']) && strlen($_SERVER['QUERY_STRING']) > 0)
$MM_referrer .= "?" . $_SERVER['QUERY_STRING'];
$MM_restrictGoTo = $MM_restrictGoTo. $MM_qsChar . "accesscheck=" . urlencode($MM_referrer);
header("Location: ". $MM_restrictGoTo);
exit;
}
?>
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$maxRows_Productset = 5;
$pageNum_Productset = 0;
if (isset($_GET['pageNum_Productset'])) {
$pageNum_Productset = $_GET['pageNum_Productset'];
}
$startRow_Productset = $pageNum_Productset * $maxRows_Productset;
$query_Productset = "SELECT * FROM product";
$query_limit_Productset = sprintf("%s LIMIT %d, %d", $query_Productset, $startRow_Productset, $maxRows_Productset);
$Productset = mysql_query($query_limit_Productset, $MyConnect) or die(mysql_error());
$row_Productset = mysql_fetch_assoc($Productset);
if (isset($_GET['totalRows_Productset'])) {
$totalRows_Productset = $_GET['totalRows_Productset'];
} else {
$all_Productset = mysql_query($query_Productset);
$totalRows_Productset = mysql_num_rows($all_Productset);
}
$totalPages_Productset = ceil($totalRows_Productset/$maxRows_Productset)-1;
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Product</title>
<style type="text/css">
#MenuBar1 {
font-size: 230%;
font-family: "Courier New", Courier, monospace;
}
body p {
font-size: 18px;
text-align: center;
color: #000;
}
body h2 {
text-align: center;
}
#form1 #word {
text-align: left;
}
#form1 #btnSearch {
text-align: center;
}
#form1 {
text-align: center;
}
</style>
<script src="js/jquery.js" type="text/javascript"></script>
<link href="css/bootstrap.min.css" rel="stylesheet" type="text/css" />
</head>
<body class='container'>
<p><img src="shop.jpg" width="1117" height="149" />
</p>
<h3>ร้านเกสร
</h3>
<p>ระบบจัดการฐานข้อมูลสำหรับร้านค้าปลีก</p>
<div class="btn-group">
<button class="btn">ขายสินค้า</button>
</div>
<div class="btn-group">
<button class="btn">ข้อมูลพื้นฐาน</button>
<button class="btn dropdown-toggle" data-toggle="dropdown">
<span class="caret"></span>
</button>
<ul class="dropdown-menu">
<li><a href="Product.php">ข้อมูลสินค้า</a></li>
<li><a href="Category.php">ข้อมูลประเภทสินค้า</a></li>
<li><a href="Supplier.php">ข้อมูลผู้จัดจำหน่าย</a></li>
<!-- dropdown menu links -->
</ul>
</div>
<div class="btn-group">
<button class="btn"><a href="Unit.php">หน่วยสินค้า</a></button>
</div>
<div class="btn-group">
<button class="btn">จัดการลูกหนี้</button>
</div>
<div class="btn-group">
<button class="btn">เพิ่มเติม</button>
<button class="btn dropdown-toggle" data-toggle="dropdown">
<span class="caret"></span>
</button>
<ul class="dropdown-menu">
<li><a href="#">ดูสินค้าใกล้หมด</a></li>
<li><a href="#">เช็คสต๊อกสินค้า</a></li>
<!-- dropdown menu links -->
</ul>
</div>
<div class="btn-group">
<button class="btn"><a href="edit-profile.php">แก้ไขข้อมูลส่วนตัว</a></button>
</div>
<div class="btn-group">
<button class="btn"><a href="admin/index.php">ออกจากระบบ</a></button>
</div>
<p> </p>
<form id="form1" name="form1" method="post" action="searchproduct.php">
<label for="word">ค้นหา:<i class="icon-zoom-in"></i></label>
<input type="text" name="word" id="word" />
<input type="submit" name="btnSearch" id="btnSearch" value="ค้นหา" />
</form>
<p>
<a href="insertproduct.php">
<input type="submit" name="btn" id="btn" value="เพิ่มสินค้า" /></a></p>
<p>แสดงสินค้า </p>
<table class="table table-bordered" width="500" border="1" align="center">
<tr class="success">
<td width="159">รหัสสินค้า</td>
<td width="181">ชื่อสินค้า</td>
<td width="136">ประเภทสินค้า</td>
<td width="132">หน่วยสินค้า</td>
<td width="195">คงเหลือต่ำสุด</td>
<td width="179">ราคา</td>
<td width="27">แก้ไข</td>
<td width="27">ลบ</td>
<td width="27" align="center">เพิ่มเติม</td>
</tr>
<?php while($rs=mysql_fetch_array($QUERY)) { ?>
<tr>
<td><?php echo $rs['product_id']; ?></td>
<td><?php echo $rs['product_name']; ?></td>
<td><?php echo $rs['cate_name']; ?></td>
<td><?php echo $rs['unit_name']; ?></td>
<td><?php echo $rs['product_balance']; ?></td>
<td><?php echo $rs['product_price']; ?></td>
<td><i class="icon-pencil"></i><a href="editproduct.php?product_id=<?php echo $rs['product_id']; ?>">แก้ไข</a></td>
<td align="center"><i class="icon-trash"></i><a onclick="return confirm_delete()" href="deleteproduct.php?product_id=<?php echo $rs['product_id'];?>">ลบ</a></td>
<td><i class="icon-asterisk"></i><a href="Purchase_detail.php?product_id=<?php
echo $rs['product_id']; ?>">เพิ่มเติม</a></td>
</tr>
<?php } $rs= mysql_fetch_assoc($Productset); ?>
</table>
<p>
หน้าที่:
<?php
for($dw_i=0;$dw_i<=$totalPages_Productset;$dw_i++){
echo '<a href="?pageNum_Productset=',$dw_i,'"class="pageNum">',($dw_i+1),'</a> ';
}
?>
</p>
<script src="js/jquery.js"></script>
<script src="js/bootstrap.min.js"></script>
<script type="text/javascript">
function confirm_delete() {
return confirm('ยืนยันการลบข้อมูล !');
}
</script>
<?php
mysql_free_result($Productset);
?>
Tag : PHP, MySQL, HTML/CSS, JavaScript

|
ประวัติการแก้ไข 2015-12-06 23:44:04
|
 |
 |
 |
 |
Date :
2015-12-06 23:41:26 |
By :
suwidha |
View :
1234 |
Reply :
4 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอน insert ลืมใส่ field product_id หรือเปล่าครับ ผมยังไม่เห็นในโค๊ด
|
 |
 |
 |
 |
Date :
2015-12-06 23:49:52 |
By :
PlaKriM |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ปัญหามันคือ product_id เป้น 0 ไม่ใช่หรอครับ ไม่ insert เข้าไปมันจะมีค่าหรอครับ
หรือผมเข้าใจโจทย์ผิด
|
 |
 |
 |
 |
Date :
2015-12-07 18:05:00 |
By :
PlaKriM |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 01
|