|
 |
|
ขอคำแนะนำเรื่อง จะเปลี่ยนข้อมูลจาก ปี-เดือน-วัน เป็น วัน-เดือน-ปี ครับ |
|
 |
|
|
 |
 |
|
ตามในรูปเลยครับ จะเปลี่ยนจาก Y-m-d เป็น d-m-Y ยังไงครับ
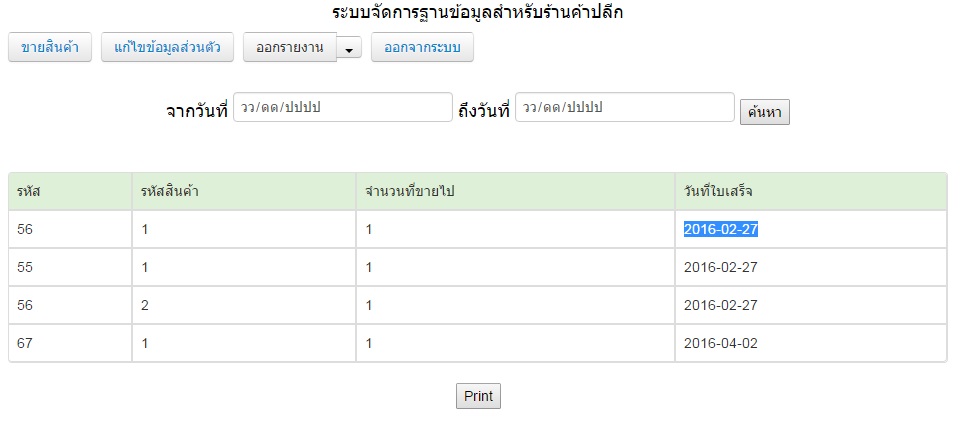
เพิ่มเติมในส่วน code ครับ
Code (PHP)
<?php require_once('Connections/MyConnect.php');
mysql_select_db($database_MyConnect, $MyConnect);
?>
<?php
if (!isset($_SESSION)) {
session_start();
}
$MM_authorizedUsers = "";
$MM_donotCheckaccess = "true";
// *** Restrict Access To Page: Grant or deny access to this page
function isAuthorized($strUsers, $strGroups, $UserName, $UserGroup) {
// For security, start by assuming the visitor is NOT authorized.
$isValid = False;
// When a visitor has logged into this site, the Session variable MM_Username set equal to their username.
// Therefore, we know that a user is NOT logged in if that Session variable is blank.
if (!empty($UserName)) {
// Besides being logged in, you may restrict access to only certain users based on an ID established when they login.
// Parse the strings into arrays.
$arrUsers = Explode(",", $strUsers);
$arrGroups = Explode(",", $strGroups);
if (in_array($UserName, $arrUsers)) {
$isValid = true;
}
// Or, you may restrict access to only certain users based on their username.
if (in_array($UserGroup, $arrGroups)) {
$isValid = true;
}
if (($strUsers == "") && true) {
$isValid = true;
}
}
return $isValid;
}
$MM_restrictGoTo = "owner/Login.php";
if (!((isset($_SESSION['MM_Username'])) && (isAuthorized("",$MM_authorizedUsers, $_SESSION['MM_Username'], $_SESSION['MM_UserGroup'])))) {
$MM_qsChar = "?";
$MM_referrer = $_SERVER['PHP_SELF'];
if (strpos($MM_restrictGoTo, "?")) $MM_qsChar = "&";
if (isset($_SERVER['QUERY_STRING']) && strlen($_SERVER['QUERY_STRING']) > 0)
$MM_referrer .= "?" . $_SERVER['QUERY_STRING'];
$MM_restrictGoTo = $MM_restrictGoTo. $MM_qsChar . "accesscheck=" . urlencode($MM_referrer);
header("Location: ". $MM_restrictGoTo);
exit;
}
?>
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$colname_salesset1 = "-1";
if (isset($_POST['dateStart'])) {
$colname_salesset1 = $_POST['dateStart'];
}
$colname2_salesset1 = "-1";
if (isset($_POST['dateEnd'])) {
$colname2_salesset1 = $_POST['dateEnd'];
}
$query_salesset1 = sprintf("SELECT * FROM sales_detail INNER JOIN sales
ON sales_detail.sales_id = sales.sales_id WHERE sales_receipt_date >= %s and sales_receipt_date<=%s Order by sales_receipt_date ASC", GetSQLValueString($colname_salesset1, "date"),GetSQLValueString($colname2_salesset1, "date"));
$salesset1 = mysql_query($query_salesset1, $MyConnect) or die(mysql_error());
$row_salesset1 = mysql_fetch_assoc($salesset1);
$totalRows_salesset1 = mysql_num_rows($salesset1);
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>btween</title>
<script src="js/jquery.js" type="text/javascript"></script>
<link href="css/bootstrap.min.css" rel="stylesheet" type="text/css" />
<script src="js/jquery.js"></script>
<script src="js/bootstrap.min.js"></script>
<style type="text/css">
#MenuBar1 {
font-size: 230%;
font-family: "Courier New", Courier, monospace;
}
body p {
font-size: 18px;
text-align: center;
color: #000;
}
body h2 {
text-align: center;
}
</style>
<script src="jquery.ui-1.5.2/jquery-1.2.6.js" type="text/javascript"></script>
<script src="jquery.ui-1.5.2/ui/ui.datepicker.js" type="text/javascript"></script>
<link href="jquery.ui-1.5.2/themes/ui.datepicker.css" rel="stylesheet" type="text/css" />
</head>
<body class='container'>
<p><img src="shop.jpg" width="1117" height="149" />
</p>
<h3>ร้านเกสร
</h3>
<p>ระบบจัดการฐานข้อมูลสำหรับร้านค้าปลีก</p>
<div class="btn-group">
<button class="btn"><a href="sales_detail.php">ขายสินค้า</a></button>
</div>
<div class="btn-group">
<button class="btn"><a href="edit-owner.php">แก้ไขข้อมูลส่วนตัว</a></button>
</div>
<div class="btn-group">
<button class="btn">ออกรายงาน</button>
<button class="btn dropdown-toggle" data-toggle="dropdown">
<span class="caret"></span>
</button>
<ul class="dropdown-menu">
<li><a href="btween-date-product.php">รายงานสินค้าคงคลัง</a></li>
<li><a href="btween-date-sales.php">รายงานการขายสินค้า</a></li>
<li><a href="btween-date-repayment.php">รายงานลูกหนี้ค้างชำระ</a></li>
<li> รายงานสินค้าขายดี </li>
<li> รายงานกำไร </li>
<!-- dropdown menu links -->
</ul>
</div>
<div class="btn-group">
<button class="btn"><a href="owner/Login.php">ออกจากระบบ</a></button>
</div>
<body>
<p> </p>
<form id="form1" name="form1" method="post" action="">
<p>จากวันที่
<input type="date" size="30" value="Click to show datepicker" name="dateStart" />
ถึงวันที่
<input type="date" size="30" value="Click to show datepicker" name="dateEnd" />
<input type="submit" name="btnsearch" id="btnsearch" value="ค้นหา" />
</p>
<p> </p>
<table class="table table-bordered" width="500" border="1" align="center">
<tr class="success">
<td>รหัส</td>
<td>รหัสสินค้า</td>
<td>จำนวนที่ขายไป</td>
<td>วันที่ใบเสร็จ</td>
</tr>
<?php
do { ?>
<tr>
<td><?php echo $row_salesset1['sales_id']; ?></td>
<td><?php echo $row_salesset1['product_id']; ?></td>
<td><?php echo $row_salesset1['sales_detail_qty']; ?></td>
<td><?php echo $row_salesset1['sales_receipt_date'];?></td> //ส่วนที่ใช้แสดง วัน-เดือน-ปี ครับ
</tr>
<?php } while ($row_salesset1 = mysql_fetch_assoc($salesset1)); ?>
</table>
<p></p>
<script type="text/javascript">
// BeginWebWidget jQuery_UI_Calendar: jQueryUICalendar2
jQuery("#jQueryUICalendar2").datepicker({dateFormat: 'yy-mm-dd'});
// EndWebWidget jQuery_UI_Calendar: jQueryUICalendar2
</script>
<p></p>
<script type="text/javascript">
// BeginWebWidget jQuery_UI_Calendar: jQueryUICalendar1
jQuery("#jQueryUICalendar1").datepicker({dateFormat: 'yy-mm-dd'});
// EndWebWidget jQuery_UI_Calendar: jQueryUICalendar1
</script>
</form>
<p>
<input type="submit" name="Submit" value="Print" onClick="window.print()">
</p>
</body>
</html>
<?php
mysql_free_result($salesset1);
?>
Tag : PHP, HTML/CSS, JavaScript, jQuery, CakePHP, iReport

|
ประวัติการแก้ไข 2016-06-26 23:21:29 2016-06-26 23:23:09 2016-06-26 23:23:55
|
 |
 |
 |
 |
Date :
2016-06-26 19:17:45 |
By :
suwidha |
View :
1295 |
Reply :
5 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
date_format(date_create(.....วันที่....),"d/m/Y");
|
ประวัติการแก้ไข 2016-06-26 21:18:22
 |
 |
 |
 |
Date :
2016-06-26 21:17:31 |
By :
mm2mail |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (PHP)
<td><?php echo date('d-m-Y',$row_salesset1['sales_receipt_date']);?></td>
|
 |
 |
 |
 |
Date :
2016-06-28 09:39:20 |
By :
tomguitar |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (PHP)
<td><?php echo date('d-m-Y',strtotime($row_salesset1['sales_receipt_date']));?></td>
อันนี้น่าจะได้นะคัฟ
|
 |
 |
 |
 |
Date :
2016-06-28 09:40:32 |
By :
tomguitar |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 04
|