|
 |
|
ขอความช่วยเหลือหน่อยคะอยากทราบว่าเวลาเรากรอกค่าในช่อง input แล้วต้องการให้มันเช็คค่าที่เรากรอกโดยที่เรายังไม่กดปุ่ม submit ทำยังไงหรอคะ |
|
 |
|
|
 |
 |
|
อยากทราบคะว่า เรากรอกค่าในช่อง [font=Verdana]จำนวน[/font] แล้วค่าห้ามเป็น 0 หรือ ติดลบ
พอกรอกแล้วเมื่อค่าเป็น 0 หรือติดลบ ให้ขึ้นแจ้งเตือนว่า [font=Verdana]กรุณากรอกค่าใหม่[/font] โดยที่ยังไม่กดปุ่ม submit ทำยังไงหรอคะ
รบกวนขอความช่วยเหลือหน่อยนะคะ
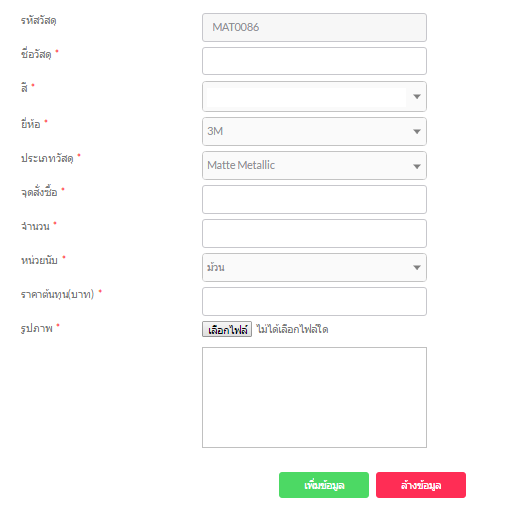
material.php
<?php
session_start();
include("connect.php");
include ("sidebar.php");
mysql_query("SET NAMES UTF8");
?>
<!DOCTYPE html>
<html lang="en">
<head>
<?php
// if (!isset($_SESSION['username']))
// {
// $message = "กรุณาเข้าสู่ระบบ!";
// echo "<script type='text/javascript'>alert('$message');</script>";
// echo "<script type='text/javascript'>location='home.php';</script>";
// exit();
// }
// if($_SESSION['status'] != "user")
// {
// $message = "เข้าได้เฉพาะ user เท่านั้น!";
// echo "<script type='text/javascript'>alert('$message');</script>";
// echo "<script type='text/javascript'>location='home.php';</script>";
// exit();
// }
?>
<!-- <msdropdown> -->
<link rel="stylesheet" type="text/css" href="css/msdropdown/dd.css" />
<script src="js/msdropdown/jquery.dd.js"></script>
<script language="javascript">
jQuery(document).ready(function(e) {
try {
jQuery("body select").msDropDown();
} catch(e) {
alert(e.message);
}
});
</script>
<!-- /////////////// -->
<script language="JavaScript" type="text/javascript">
$(document).ready(function(){
$("a.delete").click(function(e){
if(!confirm('คุณจะลบข้อมูลหรือไม่?')){
e.preventDefault();
return false;
}
return true;
});
});
</script>
<script>
function goBack() {
window.history.back();
}
</script>
<!-- โชว์ตัวอย่างรูปที่เลือก -->
<script language="JavaScript">
function showPreview(ele)
{
$('#imgAvatar').attr('src', ele.value); // for IE
if (ele.files && ele.files[0]) {
var reader = new FileReader();
reader.onload = function (e) {
$('#imgAvatar').attr('src', e.target.result);
}
reader.readAsDataURL(ele.files[0]);
}
}
</script>
<style type="text/css">
#thumbnail img{width:120px;height:120px;margin:5px;}
canvas{border:1px solid red;}
</style>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>SC Sticker</title>
</head>
<body>
<?php
$test_id = "select MAX(SUBSTRING(mat_id,6)) as num from material ";
$tmp = mysql_query($test_id) or die (mysql_error()." Error Query [".$test_id."]");
$rows = mysql_fetch_array($tmp);
if($rows){
$num = $rows['num'];
if($num==Null){
$num = 0;
}
$test_id = $num+1;
if($test_id < 10){
$mat_id = "MAT000".$test_id;
}
elseif ($test_id < 100) {
$mat_id= "MAT00".$test_id;
}
elseif ($test_id < 1000) {
$mat_id = "MAT0".$test_id;
}
}
?>
<?php
if (isset($_POST['submit']))
{
$mat_id = mysql_real_escape_string(htmlspecialchars($_POST['mat_id']));
$mat_name = mysql_real_escape_string(htmlspecialchars($_POST['mat_name']));
$color_id = mysql_real_escape_string(htmlspecialchars($_POST['color_id']));
$brand_id =mysql_real_escape_string(htmlspecialchars($_POST['brand_id']));
// $mat_pic =mysql_real_escape_string(htmlspecialchars($_POST['mat_pic']));
$typemat_id =mysql_real_escape_string(htmlspecialchars($_POST['typemat_id']));
$mat_point_order =mysql_real_escape_string(htmlspecialchars($_POST['mat_point_order']));
$mat_amount =mysql_real_escape_string(htmlspecialchars($_POST['mat_amount']));
$unit_id =mysql_real_escape_string(htmlspecialchars($_POST['unit_id']));
$costprice =mysql_real_escape_string(htmlspecialchars($_POST['costprice']));
$mat_pic = $_FILES['mat_pic']['tmp_name'];
$mat_pic_name = $_FILES['mat_pic']['name'];
mysql_query("SET character_set_results=utf8");
mysql_query("SET character_set_client=utf8");
mysql_query("SET character_set_connection=utf8");
if ($mat_amount<=0) {
echo "<script language=\"javascript\">";
echo "alert('กรุณากรอกจำนวนใหม่อีกครั้ง');";
echo "window.location='history.back()";
echo "</script>";
exit();
}
$sq = "SELECT mat_id FROM material ORDER BY mat_id ";//DESC LIMIT 1
$result = mysql_query($sq)
or die(mysql_error());
while($row = mysql_fetch_array( $result ))
{
$sq2 = $mat_id;
}
if($mat_pic){
$arraypic = explode(".",$mat_pic_name);//แบ่งชื่อไฟล์กับนามสกุลออกจากกัน
$filename = $sq2;//ชื่อไฟล์
$filetype = $arraypic[1];//นามสกุลไฟล์
$filetyper = "jpg";
if($filetype=="jpg" || $filetype=="jpeg" || $filetype=="png"
|| $filetype=="gif"){
$newimage = $filename.".".$filetyper;//รวมชื่อไฟล์กับนามสกุลเข้าด้วยกัน
$mat_path='C:/xampp/htdocs/scsticker_test/img/mat_img/'.$newimage; //หาวิธีเอา \ มาใช้
copy($mat_pic,"img/mat_img/".$newimage); //โฟลเดอร์สำหรับเก็บรูป/ไฟล์รูป
}else {
echo "<h3>ERROR : ไม่สามารถ Upload รูปภาพ</h3>";
}
}
$query = mysql_query("SELECT COUNT(mat_name) FROM material WHERE mat_name = '". $mat_name ."'");
$row2 = mysql_fetch_array($query);
if ($row2[0] > 0) {
$message = "ข้อมูลซ้ำซ้อน";
echo "<script type='text/javascript'>alert('$message');</script>";
echo '<script>location = "material.php"</script>';
}
else if($row2[0]==0)
{
$message = "เพิ่มข้อมูลสำเร็จ";
echo "<script type='text/javascript'>alert('$message');</script>";
$sql = "INSERT INTO `scsticker`.`material` (`mat_id`, `mat_name`, `color_id`, `brand_id`, `mat_pic`, `typemat_id`, `mat_point_order`, `mat_amount`, `unit_id`, `costprice`)
VALUES ('".$mat_id."', '".$mat_name."', '".$color_id."', '".$brand_id."', '".$mat_path."', '".$typemat_id."', '".$mat_point_order."', '".$mat_amount."', '".$unit_id."', '".$costprice."')";
mysql_query($sql) or die(mysql_error());
echo '<script>location = "material_show.php"</script>';
}
}
?>
<section id="main-content"> <!-- อย่าลบเลย -->
<section class="wrapper"> <!-- อย่าลบเลย -->
<!--overview start-->
<div class="row">
<div class="row">
<!-- <div class="col-lg-12"> -->
<div class="panel panel-default">
<div class="panel-body">
<ol class="breadcrumb">
<li><i class="fa fa-home"></i><a href="index.html">หน้าเเรก</a></li>
<li><i class="fa fa-laptop"></i><a href="material_show.php">ข้อมูลวัสดุ</a></li>
<li><i class="fa fa-laptop"></i>เพิ่มข้อมูลวัสดุ</li>
</ol>
<h3 class="page-header"><i class="fa fa-laptop"></i> เพิ่มข้อมูลวัสดุ</h3>
<div class="row">
<form action="" method="post" id="form" enctype="multipart/form-data">
<div class="col-sm-2"></div>
<div class="col-sm-2">รหัสวัสดุ</div>
<div class="col-sm-8"><input class="form-control" style="width:300px;" id="mat_id" name="mat_id" type="text" readonly="readonly" value="<?=$mat_id?>"></div>
<div class="col-sm-2"></div>
<div class="col-sm-2">ชื่อวัสดุ<font color="red"> *</font></div>
<div class="col-sm-8"><input class="form-control" id="mat_name" name="mat_name" style="width:300px;"></div>
<div class="col-sm-2"></div>
<div class="col-sm-2" >สี<font color="red"> *</font></div>
<div class="col-sm-8"><select name="color_id" class="form-control" style="width:300px;">
<?php
$result1 = mysql_query("SELECT * FROM color ORDER BY color_name") or die(mysql_error());
while ($row1 = mysql_fetch_array($result1))
{
?>
<option value="<?php echo $row1["color_id"]?>" data-image="img/color/<?=$row1['color_id']?>.jpg"></option>
<?php
}
?>
</select>
</div>
<div class="col-sm-2"></div>
<div class="col-sm-2" >ยี่ห้อ<font color="red"> *</font></div>
<div class="col-sm-8" ><select name="brand_id" class="form-control" style="width:300px;">
<?php
$result = mysql_query("SELECT * FROM brand ORDER BY brand_name") or die(mysql_error());
while ($row = mysql_fetch_array($result))
{
?>
<option value="<?php echo $row["brand_id"]?>"><?php echo $row["brand_name"]?></option>
<?php
}
?>
</select>
</div>
<div class="col-sm-2"></div>
<div class="col-sm-2" >ประเภทวัสดุ<font color="red"> *</font></div>
<div class="col-sm-8" ><select name="typemat_id" class="form-control" style="width:300px;">
<?php
$result = mysql_query("SELECT * FROM type_material ") or die(mysql_error());
while ($row = mysql_fetch_array($result))
{
?>
<option value="<?php echo $row["typemat_id"]?>"><?php echo $row["typemat_name"]?></option>
<?php
}
?>
</select>
</div>
<div class="col-sm-2"></div>
<div class="col-sm-2" >จุดสั่งซื้อ<font color="red"> *</font></div>
<div class="col-sm-8" ><input class="form-control" id="mat_point_order" name="mat_point_order" style="width:300px;" ></div>
<div class="col-sm-2"></div>
<div class="col-sm-2" >จำนวน<font color="red"> *</font></div>
<div class="col-sm-8" ><input class="form-control" id="mat_amount" name="mat_amount" style="width:300px;"></div>
<div class="col-sm-2"></div>
<div class="col-sm-2" >หน่วยนับ<font color="red"> *</font></div>
<div class="col-sm-8" ><select name="unit_id" class="form-control" style="width:300px;">
<?php
$result = mysql_query("SELECT * FROM unit WHERE typeun_name = 'สินค้า'") or die(mysql_error());
while ($row = mysql_fetch_array($result))
{
?>
<option value="<?php echo $row["unit_id"]?>"><?php echo $row["unit_name"]?></option>
<?php
}
?>
</select>
</div>
<div class="col-sm-2"></div>
<div class="col-sm-2">ราคาต้นทุน(บาท)<font color="red"> *</font></div>
<div class="col-sm-8"><input class="form-control" id="costprice" name="costprice" style="width:300px;"></div>
<!-- <div class="col-sm-9"> </div> <!-- <div id="thumbnail"></div> -->
<div class="col-sm-2"></div>
<div class="col-sm-2">รูปภาพ<font color="red"> *</font></div>
<div class="col-sm-8" display:inline><input type="file" name="mat_pic" id="filUpload" size="35" OnChange="showPreview(this)"></div>
<div class="col-sm-2"></div>
<div class="col-sm-2"></div><br>
<div class="col-sm-8"><img id="imgAvatar" width="300px" height="140px"></div>
<div class="col-sm-6"><br><button type="submit" class="btn btn-success " value="submit" name="submit" style= "width: 120px; float: right;">เพิ่มข้อมูล</button></div>
<div class="col-sm-6"><br><button type="button" class="btn btn-danger " style="width: 120px; float: left;" value="Reload Page" onClick="window.location.reload()">ล้างข้อมูล</button></div>
</form>
</div>
<br>
</div>
</div>
</div>
</div>
</div>
</div>
<script language="javascript">
$(document).ready(function() {
$('#dataTables-example').DataTable({
responsive: true,
"bFilter": false
});
});
</script>
<script>
$(document).ready(function () {
$("input#submit").click(function(){
$.ajax({
type: "POST",
url: "process.php", //
data: $('form.contact').serialize(),
success: function(msg){
$("#thanks").html(msg)
$("#form-content").modal('hide');
// window.location.reload();
},
error: function(){
alert("failure");
}
});
});
});
</script>
<script>
$(document).on("click", ".open-EditDialog", function () {
var id = $(this).data('id');
var name = $(this).data('name');
$(".modal-body #id").val( id );
$(".modal-body #name").val( name );
});
</script>
</body>
</html>
Tag : PHP

|
ประวัติการแก้ไข 2016-10-11 00:43:11
|
 |
 |
 |
 |
Date :
2016-10-11 00:42:29 |
By :
POMP |
View :
1673 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
คือตอนนี้ลองใช้ onKeyUp="if(isNaN(this.value)){ alert('กรุณากรอกตัวเลข'); this.value='';}" ที่หามาจากในเว็บ
ผลที่ได้คือใส่เป็นตัวหนังสือ หรือ - มันขึ้นแจ้งเตือนแล้วคะ แต่ถ้าใส่เป็น 0 มันไม่ขึ้นแจ้งเตือนคะ
ช่วยหนูหน่อยนะคะ TT
material.php
<div class="col-sm-2"></div>
<div class="col-sm-2" >จำนวน<font color="red"> *</font></div>
<div class="col-sm-8" ><input class="form-control" id="mat_amount" name="mat_amount" style="width:300px;" onKeyUp="if(isNaN(this.value)){ alert('กรุณากรอกตัวเลข'); this.value='';}"></div>
|
ประวัติการแก้ไข 2016-10-11 01:08:10
 |
 |
 |
 |
Date :
2016-10-11 01:07:36 |
By :
POMP |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 00
|