|
 |
|
พี่ๆช่วยดูหน่อยครับคือผมส่งค่าจาก row มาแล้วจะให้ดค๊ต Select แต่ค่าไม่มาครับ |
|
 |
|
|
 |
 |
|
โดยที่ xml ไม่มีค่าอะไรส่งมาเลยครับ
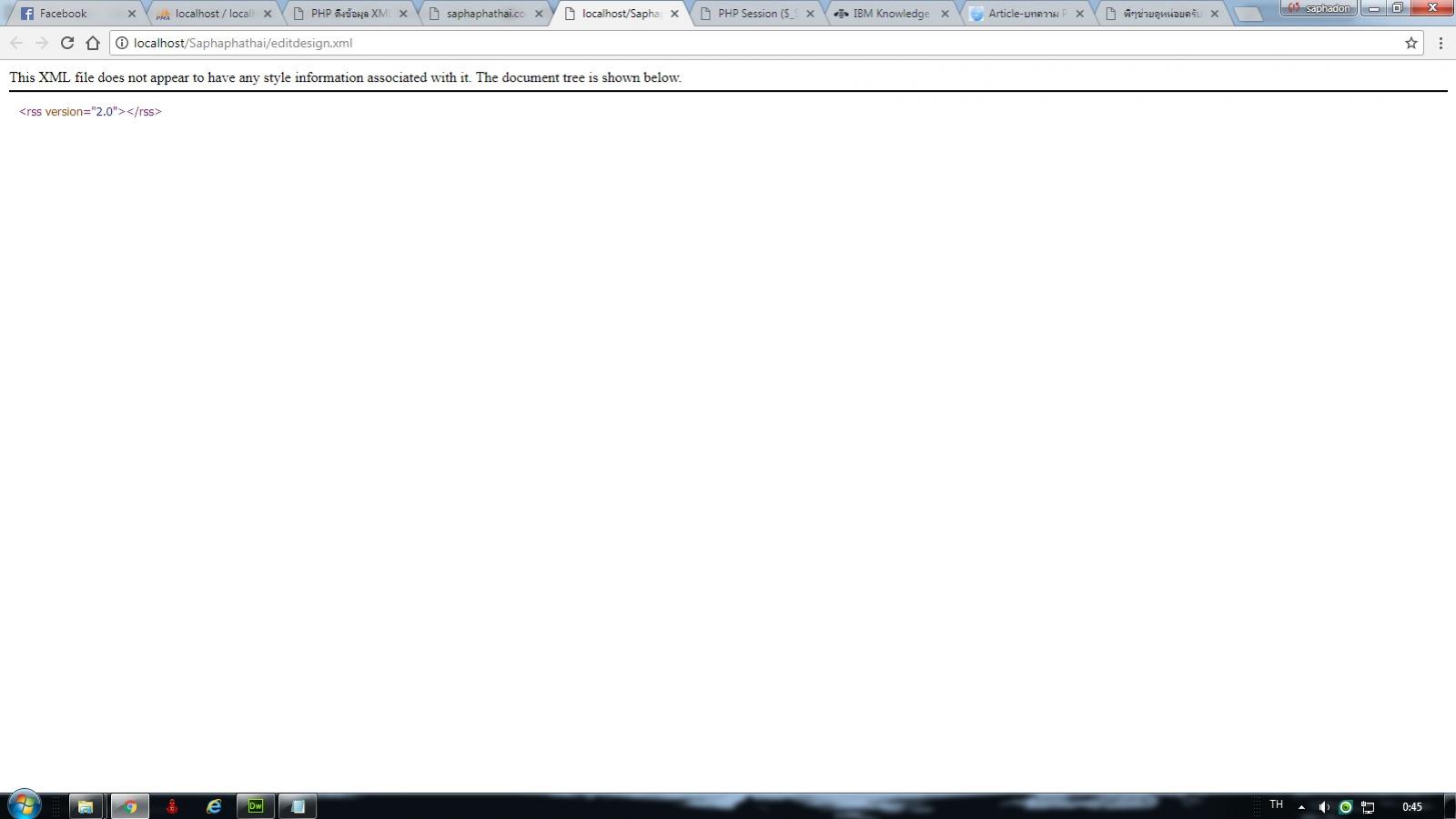
ถ้าอย่าง ผมแก้เป็น แบบนี้แล้วค่าจะมาครับ ถ้าทำแบบข้างบนค่าไม่มา
Code (PHP)
$sql=mysql_query("select * from design_detail where de_id = '2' ");
|
ประวัติการแก้ไข 2017-08-31 01:02:51
 |
 |
 |
 |
Date :
2017-08-31 00:51:03 |
By :
1427487567274575 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เช็คตัวแปรมาครบไหม
echo $_REQUEST["de_id"];
และ
Code (PHP)
$sql=mysql_query($stm="select * from design_detail where de_id = '$de_id' ");
if(mysql_num_rows($sql)==0){
echo $stm; exit;
}
|
ประวัติการแก้ไข 2017-08-31 08:31:17
 |
 |
 |
 |
Date :
2017-08-31 08:29:24 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จะผิดหลือถูกอย่าว่ากันน่ะครับ
$de_id = $_REQUEST["de_id"];
แบบว่า ค่าใน $de_id มันไม่มี ค่าอะไรเลย ที่ $_POST หลือ $_GET เข้ามา มันเลย ทำให้
$sql=mysql_query("select * from design_detail where de_id = '$de_id' ");
===> where de_id = '$de_id' อันนี้ ทำให้ ไม่มีค่ามา เป็น False
----------
ส่วนตัวนี้ คือ
$sql=mysql_query("select * from design_detail where de_id = '2' ");
where de_id = '2' มีค่าเป็น จริง True ค่าที่ได้จะออกมา แต่ Databases
วิธีแก้ ลอง สร้าง From รับค่า แล้ว POST ลองดู ข้างล่างนี้ครับ
---------
การตั้งตัวแปลแบบ $_REQUEST
https://www.thaicreate.com/php/php-request.html
|
 |
 |
 |
 |
Date :
2017-08-31 08:58:02 |
By :
Bouasavanh HararRock |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอบคุณพี่ๆ นะครับ จะลองแก้ดูครับ น่าจะตามที่พี่บอกค่ามันไม่มาจะลอง แก้ไขดูครับ
|
 |
 |
 |
 |
Date :
2017-08-31 09:09:59 |
By :
1427487567274575 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 05
|