|
 |
|
สอบถามปัญหาการสร้างตารางด้วย Bootstrap โดยใช้ jQuery เกิดการหัวข้อเบิ้ล 2 บรรทัด |
|
 |
|
|
 |
 |
|
สามารถสร้างตารางได้เป็นปกติครับ แต่ส่วนหัวของตารางจะเกิดอาการหัวข้อเบิ้ล 2 บรรทัดติดกันและตารางแสดงข้อมูลเกิน 10 ช่องที่กำหนดไว้ครับ พยายามลองแก้หลายๆอย่างแล้วครับ
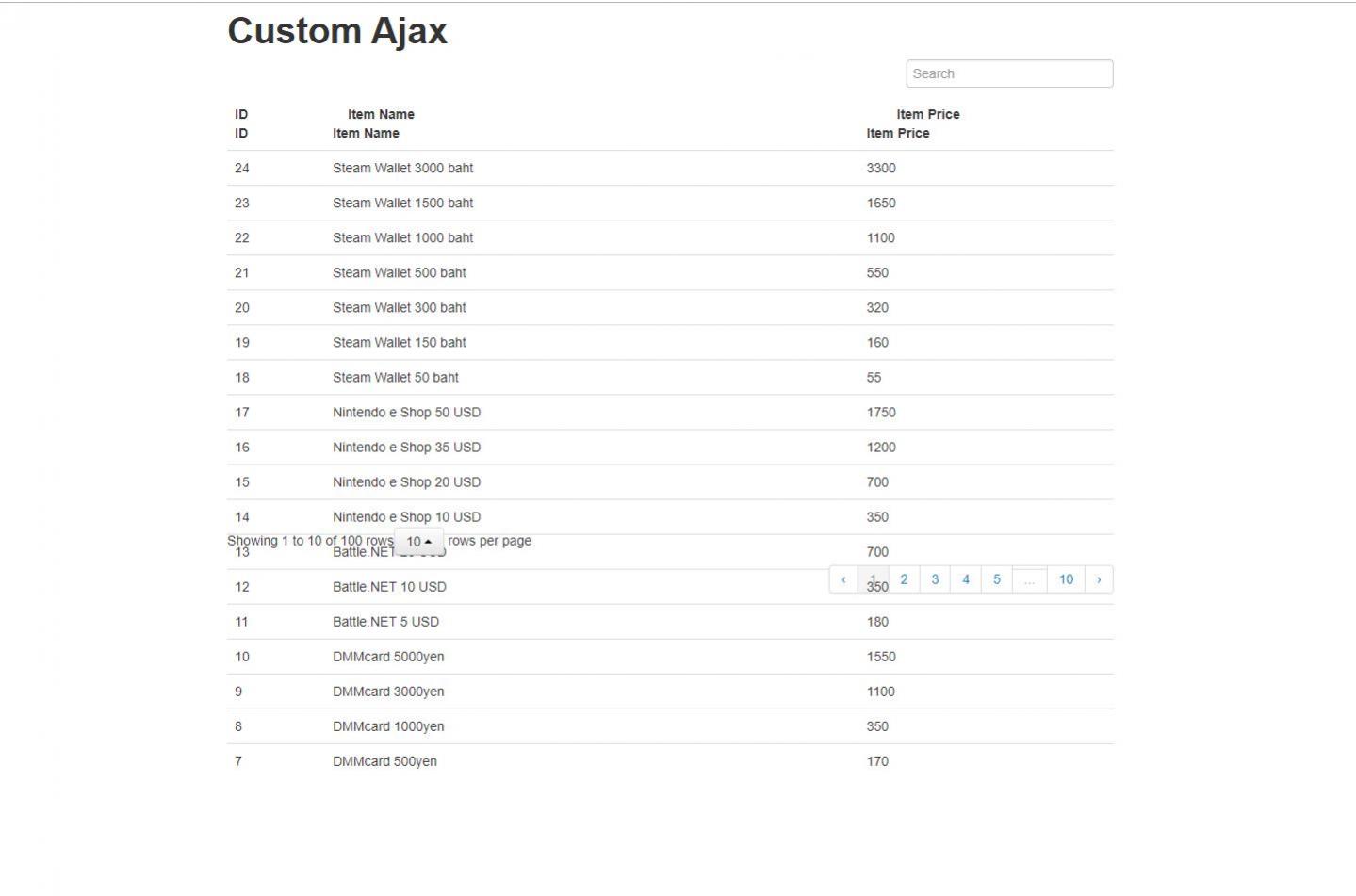
ส่วนโค้ทนะครับ
หน้าแสดงตาราง
Code (PHP)
<!DOCTYPE html>
<html>
<head>
<title>custom-ajax</title>
<meta charset="utf-8">
<link href="bootstrap/css/bootstrap.min.css" rel="stylesheet">
<script src="jquery-3.3.1.min.js"></script>
<script src="bootstrap/css/bootstrap.min.css" rel="stylesheet"></script>
<script src="bootstrap/css/bootstrap.css" rel="stylesheet"></script>
<script src="bootstrap/js/bootstrap.min.js"></script>
<script src="bootstrap/css/bootstrap-responsive.css"></script>
<script src="bootstrap/css/bootstrap-responsive.min.css"></script>
<script src="http://code.jquery.com/jquery-latest.js"></script>
<script src="bootstrap/js/bootstrap-table.js"></script>
<script src="bootstrap/js/bootstrap-table.css"></script>
<script src="bootstrap/js/examples.css"></script>
</head>
<body>
<div class="container">
<h1>Custom Ajax</h1>
<table id="table"
data-toggle="table"
data-height="460"
data-ajax="ajaxRequest"
data-search="true"
data-side-pagination="server"
data-pagination="true">
<thead>
<tr>
<th data-field="ProductID">ID</th>
<th data-field="ProductName">Item Name</th>
<th data-field="Price">Item Price</th>
</tr>
</thead>
</table>
</div>
<script>
var $table = $('#table');
var rows;
// your custom ajax request here
function ajaxRequest(params) {
$.ajax({
type: "POST",
url: "getData.php",
data: params.data,
dataType: "json",
success: function (rows) {
$(".fixed-table-loading").hide();
params.success({
"rows": rows.items,
"total": rows.total
})
},
error: function (er) {
params.error(er);
}
});
}
$table.bootstrapTable();
</script>
</body>
</html>
หน้าเรียกข้อมูลจาก db
Code (PHP)
<?php
$objConnect = mysql_connect("localhost","root","") or die(mysql_error());
$objDB = mysql_select_db("sirshell");
$strSQL = "SELECT * FROM product WHERE 1 ORDER BY ProductID DESC ";
$objQuery = mysql_query($strSQL) or die (mysql_error());
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
$resultArray2["items"] = $resultArray;
$resultArray2["total"] = 100;
echo json_encode($resultArray2);
?>
Tag : PHP, MySQL, Ajax, jQuery

|
|
 |
 |
 |
 |
Date :
2018-11-28 13:12:46 |
By :
SirShell |
View :
1073 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
สร้าง <tbody></tbody> แล้ว append มันลงที่นี่ครับ
|
 |
 |
 |
 |
Date :
2018-12-03 16:29:58 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 00
|