|
 |
|
แจกวิธีการ [PHP] การนำข้อมูลใน Excel ที่อ่านค่าด้วย PHPExcel มาใส่ข้อมูลลงใน input form แบบ autofill ในรูปแบบเลือกช่อง Excel ที่ต้องการ ครับ |
|
 |
|
|
 |
 |
|
แจกวิธีการ [PHP] การนำข้อมูลใน Excel ที่อ่านค่าด้วย PHPExcel มาใส่ข้อมูลลงใน input form แบบ autofill ในรูปแบบเลือกช่อง Excel ที่ต้องการ + รองรับไม่ว่าจะกรอกเป็นตัวอักษรขนาดไหน ก็จะกลายเป็นตัวพิมพ์ใหญ่เสมอ ครับ
[PHP] การนำข้อมูลใน Excel ที่อ่านค่าด้วย PHPExcel มาใส่ข้อมูลลงใน input form แบบ autofill ในรูปแบบเลือกช่อง Excel ที่ต้องการ + รองรับไม่ว่าจะกรอกเป็นตัวอักษรขนาดไหน ก็จะกลายเป็นตัวพิมพ์ใหญ่เสมอ บทความเรื่องการนำข้อมูลใน Excel ที่อ่านค่าด้วย PHPExcel มาใส่ข้อมูลลงใน input form แบบ autofill ในรูปแบบเลือกช่อง Excel ที่ต้องการ + รองรับไม่ว่าจะกรอกเป็นตัวอักษรขนาดไหน ก็จะกลายเป็นตัวพิมพ์ใหญ่เสมอครับ
ซึ่งการนำข้อมูลใน Excel ที่อ่านค่าด้วย PHPExcel มาใส่ข้อมูลลงใน input form แบบ autofill ในรูปแบบเลือกช่อง Excel ที่ต้องการนั้น ได้คำตอบมาจาก link ในเว็บ Ninenik ด้านล่างครับ
http://niik.in/que_3011_6720
และเรื่อง รองรับไม่ว่าจะกรอกเป็นตัวอักษรขนาดไหน ก็จะกลายเป็นตัวพิมพ์ใหญ่เสมอ ได้แนวทางมาจาก เว็บ jQuery Forum ด้วยเช่นกันครับ
https://forum.jquery.com/topic/convert-text-to-uppercase
ซึ่งปรับปรุงเป็นฉบับของตัวเอง Code ทั้งหมด มีดังนี้ครับ
1. excelimport.php
Code (PHP)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
h2,h4 {display: inline;}
</style>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data" name="myform1" id="myform1">
<details>
<summary>กรอกช่อง Excel ที่ต้องการจะเลือก</summary>
<br><h4 for="selectcell1">ช่อง Excel ที่ต้องการจะเลือกในส่วน First name : </h4><input type="text" id="selectcell1" name="selectcell1"><br><br>
<h4 for="selectcell2">ช่อง Excel ที่ต้องการจะเลือกในส่วน Middle name : </h4><input type="text" id="selectcell2" name="selectcell2"><br><br>
<h4 for="selectcell3">ช่อง Excel ที่ต้องการจะเลือกในส่วน Last name : </h4><input type="text" id="selectcell3" name="selectcell3">
</details><br>
<h2 for="myfile1">Select files : </h2><input type="file" name="excelFile" id="excelFile" /><br><br>
<h2 for="fname">First name : </h2><input type="text" id="fname" name="fname"><br><br>
<h2 for="lname">Middle name : </h2><input type="text" id="lname" name="lname"><br><br>
<h2 for="lname">Last name : </h2><input type="text" id="mname" name="mname"><br><br>
<input type="submit" name="btnSubmit" id="btnSubmit" value="Submit" />
</form>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
// เมื่อฟอร์มการเรียกใช้ evnet submit ข้อมูล
$("#excelFile").on("change",function(e){
e.preventDefault(); // ปิดการใช้งาน submit ปกติ เพื่อใช้งานผ่าน ajax
// เตรียมข้อมูล form สำหรับส่งด้วย FormData Object
var formData = new FormData($("#myform1")[0]);
// ส่งค่าแบบ POST ไปยังไฟล์ read_excel.php รูปแบบ ajax แบบเต็ม
$.ajax({
url: 'read_excel.php',
type: 'POST',
data: formData,
/*async: false,*/
cache: false,
contentType: false,
processData: false
}).done(function(data){
console.log(data); // ทดสอบแสดงค่า ดูผ่านหน้า console
/* การใช้งาน console log เพื่อ debug javascript ใน chrome firefox และ ie
http://www.ninenik.com/content.php?arti_id=692 via @ninenik */
$('#selectcell1').val($('#selectcell1').val().toUpperCase());
$('#selectcell2').val($('#selectcell2').val().toUpperCase());
$('#selectcell3').val($('#selectcell3').val().toUpperCase());
if($("#selectcell1").val() != ""){
$("#fname").val(eval("data." + $("#selectcell1").val()));
}
if($("#selectcell2").val() != ""){
$("#lname").val(eval("data." + $("#selectcell2").val()));
}
if($("#selectcell3").val() != ""){
$("#mname").val(eval("data." + $("#selectcell3").val()));
}
});
});
});
</script>
</body>
</html>
2. read_excel.php (ยก Code จากคำตอบในเว็บ Ninenik มาใช้งานได้เลยครับ)
Code (PHP)
<?php
header("Content-type:application/json; charset=UTF-8");
header("Cache-Control: no-store, no-cache, must-revalidate");
header("Cache-Control: post-check=0, pre-check=0", false);
/** Error reporting */
error_reporting(E_ALL);
ini_set('display_errors', TRUE);
ini_set('display_startup_errors', TRUE);
date_default_timezone_set('Asia/Bangkok');
// http://php.net/manual/en/timezones.php
require_once("PHPExcel/Classes/PHPExcel.php");
?>
<?php
if(isset($_FILES['excelFile']['name']) && $_FILES['excelFile']['name']!=""){
$tmpFile = $_FILES['excelFile']['tmp_name'];
$fileName = $_FILES['excelFile']['name']; // เก็บชื่อไฟล์
$_fileup = $_FILES['excelFile'];
$info = pathinfo($fileName);
$allow_file = array("csv","xls","xlsx");
/* print_r($info); // ข้อมูลไฟล์
print_r($_fileup);*/
if($fileName!="" && in_array($info['extension'],$allow_file)){
// อ่านไฟล์จาก path temp ชั่วคราวที่เราอัพโหลด
$objPHPExcel = PHPExcel_IOFactory::load($tmpFile);
// ดึงข้อมูลของแต่ละเซลในตารางมาไว้ใช้งานในรูปแบบตัวแปร array
$cell_collection = $objPHPExcel->getActiveSheet()->getCellCollection();
// วนลูปแสดงข้อมูล
$v=1;
$json_data = array();
foreach ($cell_collection as $cell) {
// ค่าสำหรับดูว่าเป็นคอลัมน์ไหน เช่น A B C ....
$column = $objPHPExcel->getActiveSheet()->getCell($cell)->getColumn();
// คำสำหรับดูว่าเป็นแถวที่เท่าไหร่ เช่น 1 2 3 .....
$row = $objPHPExcel->getActiveSheet()->getCell($cell)->getRow();
// ค่าของข้อมูลในเซลล์นั้นๆ เช่น A1 B1 C1 ....
$data_value = $objPHPExcel->getActiveSheet()->getCell($cell)->getValue();
// เท่านี้เราก็สามารถแสดงข้อมูลจากการอ่านไฟล์ได้แล้ว และสามารถนำข้อมูลเหล่านี้
// ทำการบันทักลงฐานข้อมูล หรือแสดงได้เลย
$json_data["$column$row"] = $data_value;
// echo $v." ---- ".$data_value."<br>";
$v++;
}
// แปลง array เป็นรูปแบบ json string
if(isset($json_data)){
$json= json_encode($json_data);
if(isset($_GET['callback']) && $_GET['callback']!=""){
echo $_GET['callback']."(".$json.");";
}else{
echo $json;
}
}
}
}
?>
3. PHPExcel ซึ่งโหลดจากเว็บ https://github.com/PHPOffice/PHPExcel/ ซึ่งมีโครงสร้างของไฟล์ทั้งหมด ดังนี้ครับ
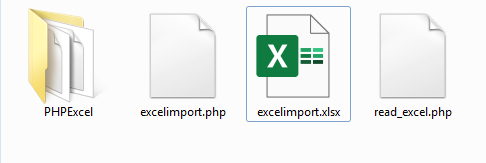
4. excelimport.xlsx - http://doanga2007.github.io/excelimport.xlsx
Screenshot ครับ
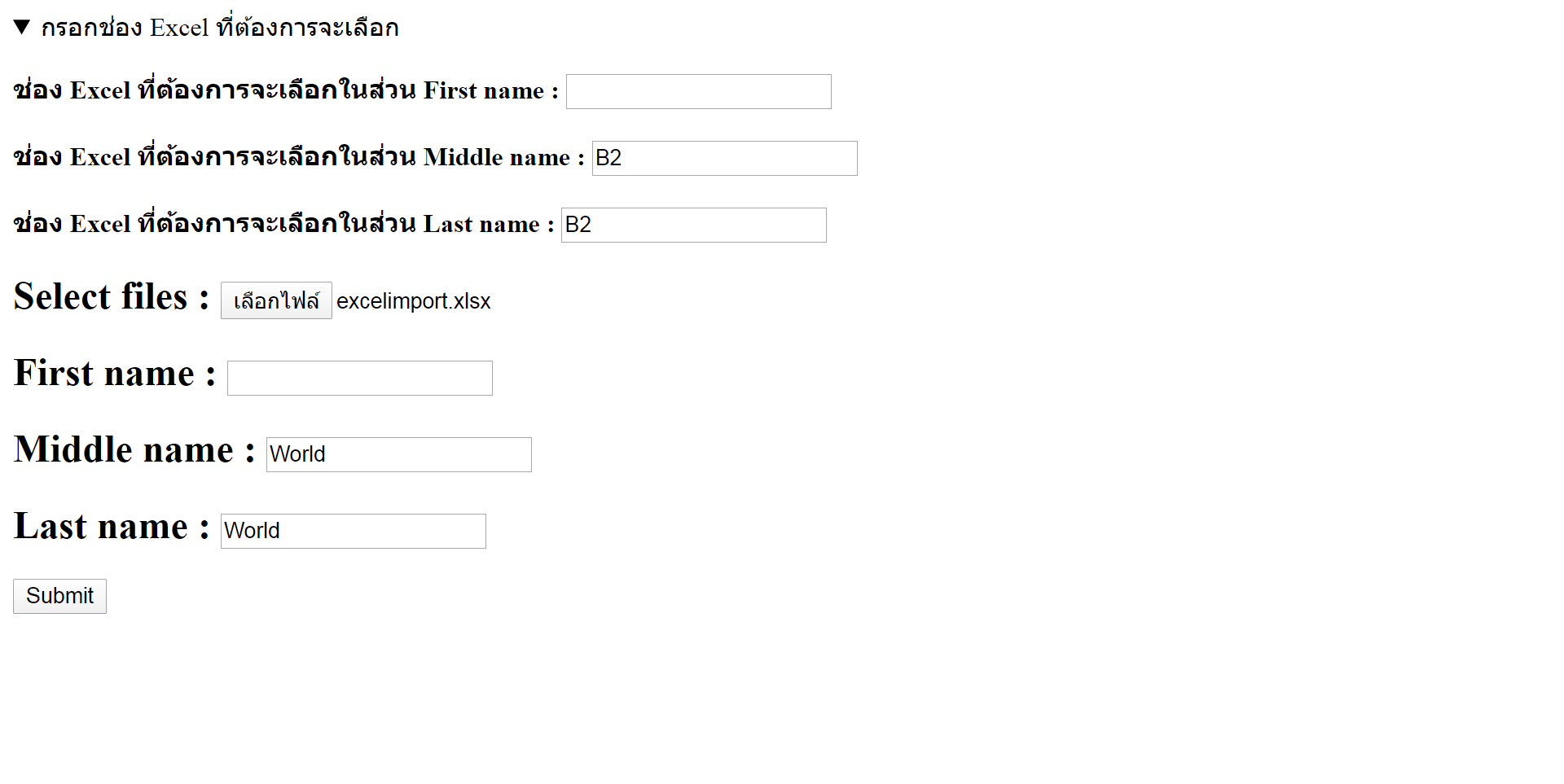
Link บทความครับ
Tag : PHP, HTML, HTML5, JavaScript, Excel (Excel.Application), XAMPP

|
ประวัติการแก้ไข 2020-03-16 15:25:52 2020-03-18 13:35:05 2020-03-20 09:14:32
|
 |
 |
 |
 |
Date :
2020-03-14 20:29:57 |
By :
doanga2007 |
View :
2540 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Thanks 
|
 |
 |
 |
 |
Date :
2020-08-03 13:17:00 |
By :
PhrayaDev |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|
|

|
Load balance : Server 02
|