 |
มือใหม่สอบถามหน่อยค่ะ เขียนโปรแกรม connect ไปใน database xampp แต่ connect ไม่ได้ (อัพรูปใหม่) |
|
 |
|
|
 |
 |
|
ขอสอบถามค่ะ คือจะส่งค่าที่อ่านได้จากเซนเซอร์ ในโปรแกรม arduino ไปเก็บบน database แต่ติดปัญหา ในส่วนของ php ที่ไม่สามารถติดต่อกับฐานข้อมูลได้ ต้องแก้ไขยังไงค่ะ ขอบคุณค่ะ
Code (PHP)
<?php
/*
Rui Santos
Complete project details at https://RandomNerdTutorials.com/esp32-esp8266-mysql-database-php/
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files.
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
*/
include "connect.php";
$api_key_value = "tPmAT5Ab3j7F9";
if ($_SERVER["REQUEST_METHOD"] == "GET") {
$api_key = $_GET["api_key"];
if($api_key == $api_key_value) {
$s1 = $_GET["s1"];
$lo1 = $_GET["lo1"];
$t1 = $_GET["t1"];
$h1 = $_GET["h1"];
$pm1 = $_GET["pm1"];
$pm2 = $_GET["pm2"];
$pm3 = $_GET["pm3"];
$sql = "INSERT INTO sensordata (sensor, location, temp, hum, pm1, pm2, pm3)
VALUES ('" . $s1 . "', '" . $lo1 . "', " . $t1 . ", " . $h1 . ", " . $pm1 . ", " . $pm2 . ", " . $pm3 . ")";
$objQuery = mysqli_query($conn,$sql);
}else {
echo "Wrong API Key provided.";
}
}else {
echo "No data getted with HTTP GET.";
}
?>
ปัญหาที่พบ:
Notice: Undefined index: api_key in C:\xampp\htdocs\esp32\post-esp-data.php on line 18
Wrong API Key provided.
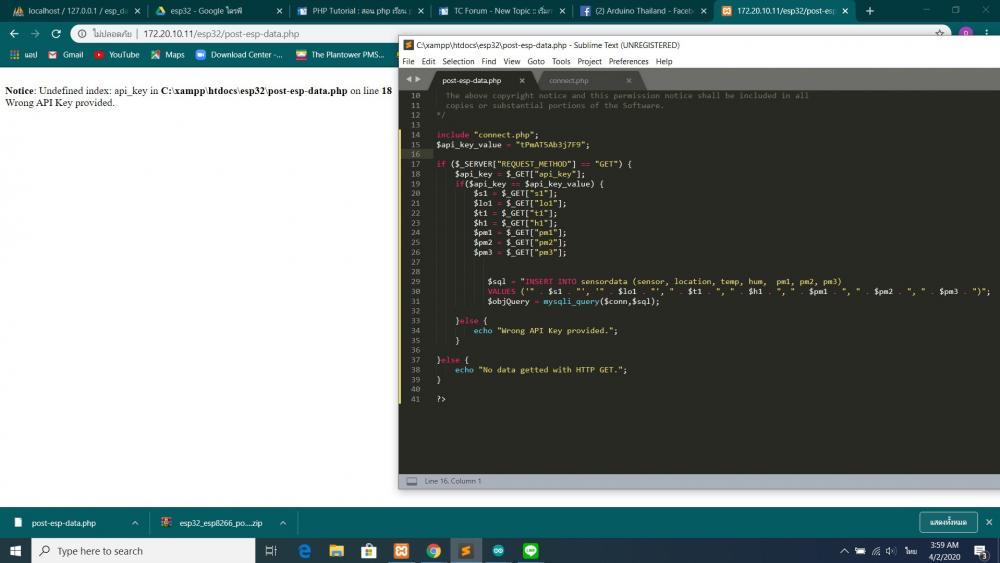
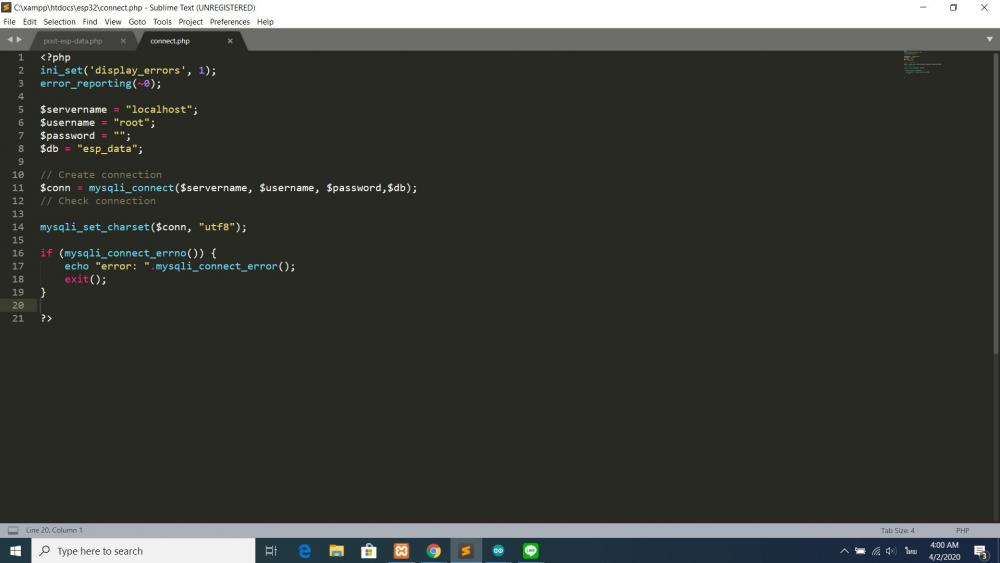
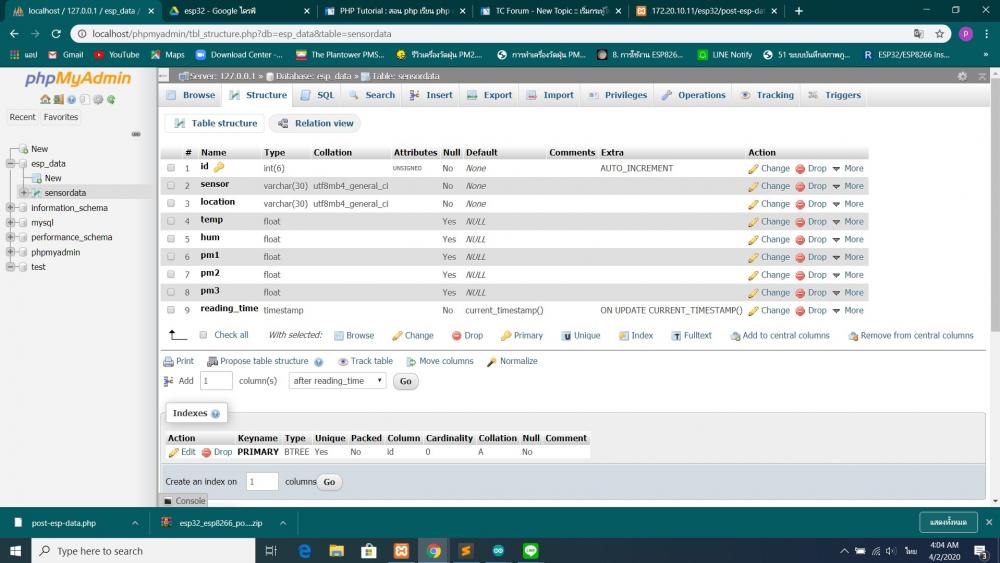
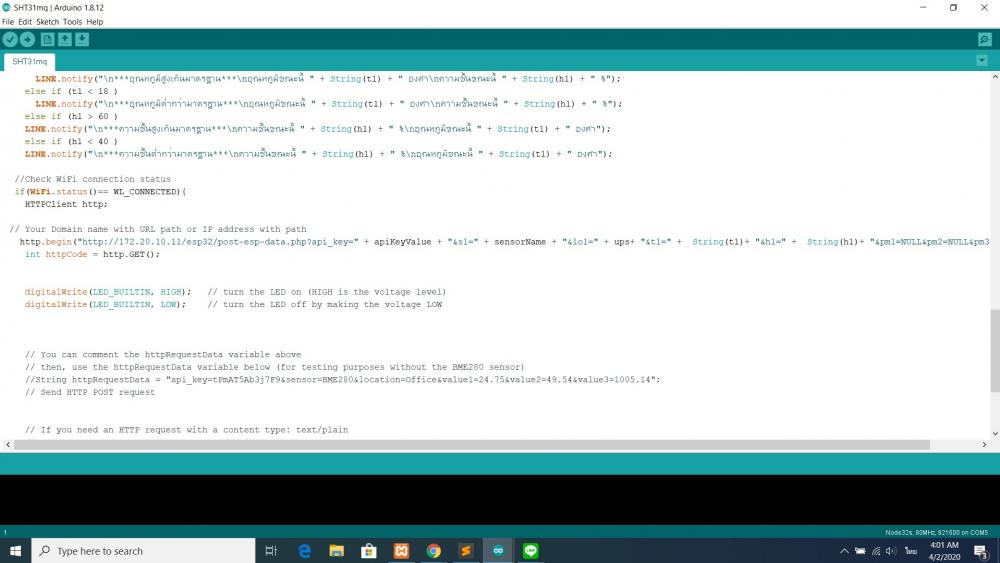
Code (C#)
/***************************************************
This is an example for the SHT31-D Humidity & Temp Sensor
Designed specifically to work with the SHT31-D sensor from Adafruit
----> https://www.adafruit.com/products/2857
These sensors use I2C to communicate, 2 pins are required to
interface
****************************************************/
#include <Arduino.h>
#include <WiFi.h>
#include <HTTPClient.h>
#include <TridentTD_LineNotify.h>
#include <Wire.h>
#include "Adafruit_SHT31.h"
Adafruit_SHT31 sht31 = Adafruit_SHT31();
#if defined(ARDUINO_ARCH_SAMD)
// for Zero, output on USB Serial console, remove line below if using programming port to program the Zero!
#define Serial SerialUSB
#endif
//config Line
#define LINE_TOKEN "H3k0goIB4KGvAQiEpRMHzHmCN5cyRV0jpI2pDoIDybZ"
// Replace with your network credentials
const char* ssid = "iPhone";
const char* password = "KKKjjj21";
// REPLACE with your Domain name and URL path or IP address with path
const char* serverName = "http://172.20.10.11/esp32/post-esp-data.php";
// Keep this API Key value to be compatible with the PHP code provided in the project page.
// If you change the apiKeyValue value, the PHP file /post-esp-data.php also needs to have the same key
String apiKeyValue = "tPmAT5Ab3j7F9";
String sensorName = "SHT31";
String ups = "1";
unsigned long last_time=0;
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
Serial.println("Connecting");
while(WiFi.status() != WL_CONNECTED) {
delay(100);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to WiFi network with IP Address: ");
Serial.println(WiFi.localIP());
LINE.setToken(LINE_TOKEN); // กำหนด Line Token
sht31.begin(0x44);
}
void loop() {
if (millis() > last_time + 5000) { //***1000 millis = 1 วินาที, 1 นาที = 60 วินาที , 30 นาที = 1000 x 60 x 30 = 1800000
float t1 = sht31.readTemperature();
float h1= sht31.readHumidity();
int adc=analogRead(A0);
if (isnan(t1) || isnan(h1))
{
Serial.println("Error reading ");
delay(1000);
return;
}
Serial.print("Humidity: ");
Serial.print(h1); // แสดงค่าความชื้น
Serial.print(" %\t");
Serial.print("Temperature: ");
Serial.print(t1); // แสดงค่าอุณหภูมิเป็นองศาเซลเซียส
Serial.println(" *C ");
if (t1 > 27 ) // กำหนดว่า อุณภูมิเกิน เมื่อไหร่ ให้แจ้งเตือนเข้าไลน์
LINE.notify("\n***อุณหภูมิสูงเกินมาตรฐาน***\nอุณหภูมิขณะนี้ " + String(t1) + " องศา\nความชื้นขณะนี้ " + String(h1) + " %");
else if (t1 < 18 )
LINE.notify("\n***อุณหภูมิต่ำกว่ามาตรฐาน***\nอุณหภูมิขณะนี้ " + String(t1) + " องศา\nความชื้นขณะนี้ " + String(h1) + " %");
else if (h1 > 60 )
LINE.notify("\n***ความชื้นสูงเกินมาตรฐาน***\nความชื้นขณะนี้ " + String(h1) + " %\nอุณหภูมิขณะนี้ " + String(t1) + " องศา");
else if (h1 < 40 )
LINE.notify("\n***ความชื้นต่ำกว่ามาตรฐาน***\nความชื้นขณะนี้ " + String(h1) + " %\nอุณหภูมิขณะนี้ " + String(t1) + " องศา");
//Check WiFi connection status
if(WiFi.status()== WL_CONNECTED){
HTTPClient http;
// Your Domain name with URL path or IP address with path
http.begin("http://172.20.10.11/esp32/post-esp-data.php?api_key=" + apiKeyValue + "&s1=" + sensorName + "&lo1=" + ups+ "&t1=" + String(t1)+ "&h1=" + String(h1)+ "&pm1=NULL&pm2=NULL&pm3=NULL");
int httpCode = http.GET();
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
// You can comment the httpRequestData variable above
// then, use the httpRequestData variable below (for testing purposes without the BME280 sensor)
//String httpRequestData = "api_key=tPmAT5Ab3j7F9&sensor=BME280&location=Office&value1=24.75&value2=49.54&value3=1005.14";
// Send HTTP POST request
// If you need an HTTP request with a content type: text/plain
//http.addHeader("Content-Type", "text/plain");
//int httpResponseCode = http.POST("Hello, World!");
// If you need an HTTP request with a content type: application/json, use the following:
//http.addHeader("Content-Type", "application/json");
//int httpResponseCode = http.POST("{\"value1\":\"19\",\"value2\":\"67\",\"value3\":\"78\"}");
last_time = millis();
delay(1000);
}
}
}
Tag : PHP, MySQL, Ms SQL Server 2016, XAMPP
|
ประวัติการแก้ไข 2020-04-02 04:14:16 2020-04-02 04:17:12 2020-04-02 04:18:08 2020-04-02 04:21:56 2020-04-02 04:23:18
|
 |
 |
 |
 |
Date :
2020-04-02 04:05:55 |
By :
kwanjaiji |
View :
1699 |
Reply :
6 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
พยายามเขียนตรวจสอบทุก รายการของคำสั่ง mysqli
Code (PHP)
$db=new mysqli($host,$user,$pswd,$db);
if( $db->errno>0) die( $db->error);
$rs=$db->query('select .....') or die($db->error);
if( $rs->num_rows<1) die('empty record');
|
 |
 |
 |
 |
Date :
2020-04-02 06:51:59 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Notice: Undefined index: api_key URI มันไม่ได้มีการแนบ parameter
ผมไม่คิดว่ามันแนบ parameter มาด้วยบน URI ไม่งั้นคุณลองใส่ if(isset($api_key)) ดู
|
 |
 |
 |
 |
Date :
2020-04-02 09:45:50 |
By :
Genesis™ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมก็คิดว่าเป็นที่พารามิเตอร์
ลองเทส url แบบนี้ครับ
http://172.20.10.11/esp32/post-esp-data.php?api_key=tPmAT5Ab3j7F9
error จะต้องเปลี่ยนเป็น Undefined index: s1
ถ้าเป็นตามนี้ก็ใส่พารามิเตอร์ให้ครบแล้วทดสอบจนไม่มี error
ปัญหาสุดท้ายคือการเชื่อมต่อจาก Micro-controller ไปยัง host (เพราะจากโค้ดไม่น่ามีปัญหาอะไร http.begin ก็ใส่พารามิเตอร์ครบแล้ว)
|
 |
 |
 |
 |
Date :
2020-04-02 12:13:46 |
By :
PhrayaDev |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|