PHP MySQL Multiple Rows Edit/Update Record |
PHP MySQL Rows Textbox Edit/Update Record เป็นการเขียนโปรแกรม PHP กับ MySQL เพื่อแก้ไขข้อมูลหลาย ๆ แถวภายใน Form เดียวกัน คล้าย ๆ กับ DataGrid
ตัวอย่าง
phpMySQLEditRecord.php
<html>
<head>
<title>ThaiCreate.Com PHP & MySQL Tutorial</title>
</head>
<body>
<?php
$objConnect = mysql_connect("localhost","root","root") or die("Error Connect to Database");
$objDB = mysql_select_db("mydatabase");
//*** Update Condition ***//
if($_GET["Action"] == "Save")
{
for($i=1;$i<=$_POST["hdnLine"];$i++)
{
$strSQL = "UPDATE customer SET ";
$strSQL .="CustomerID = '".$_POST["txtCustomerID$i"]."' ";
$strSQL .=",Name = '".$_POST["txtName$i"]."' ";
$strSQL .=",Email = '".$_POST["txtEmail$i"]."' ";
$strSQL .=",CountryCode = '".$_POST["txtCountryCode$i"]."' ";
$strSQL .=",Budget = '".$_POST["txtBudget$i"]."' ";
$strSQL .=",Used = '".$_POST["txtUsed$i"]."' ";
$strSQL .="WHERE CustomerID = '".$_POST["hdnCustomerID$i"]."' ";
$objQuery = mysql_query($strSQL);
}
//header("location:$_SERVER[PHP_SELF]");
//exit();
}
$strSQL = "SELECT * FROM customer ORDER BY CustomerID ASC";
$objQuery = mysql_query($strSQL) or die ("Error Query [".$strSQL."]");
?>
<form name="frmMain" method="post" action="phpMySQLEditRecord.php?Action=Save">
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<?php
$i =0;
while($objResult = mysql_fetch_array($objQuery))
{
$i = $i + 1;
?>
<tr>
<td><div align="center">
<input type="hidden" name="hdnCustomerID<?php echo $i;?>" size="5" value="<?php echo $objResult["CustomerID"];?>">
<input type="text" name="txtCustomerID<?php echo $i;?>" size="5" value="<?php echo $objResult["CustomerID"];?>">
</div></td>
<td><input type="text" name="txtName<?php echo $i;?>" size="20" value="<?php echo $objResult["Name"];?>"></td>
<td><input type="text" name="txtEmail<?php echo $i;?>" size="20" value="<?php echo $objResult["Email"];?>"></td>
<td><div align="center"><input type="text" name="txtCountryCode<?php echo $i;?>" size="2" value="<?php echo $objResult["CountryCode"];?>"></div></td>
<td align="right"><input type="text" name="txtBudget<?php echo $i;?>" size="5" value="<?php echo $objResult["Budget"];?>"></td>
<td align="right"><input type="text" name="txtUsed<?php echo $i;?>" size="5" value="<?php echo $objResult["Used"];?>"></td>
</tr>
<?php
}
?>
</table>
<input type="submit" name="submit" value="submit">
<input type="hidden" name="hdnLine" value="<?php echo $i;?>">
</form>
<?php
mysql_close($objConnect);
?>
</body>
</html>
Screenshot
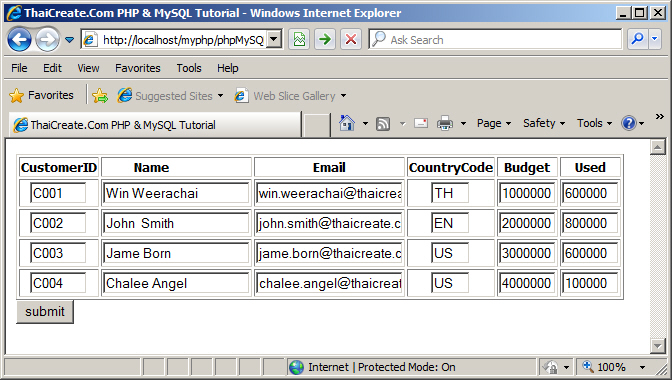
ตัวอย่าง 2 ตรง CountryCode เป็นแบบ Select menu หรือ DropDownList และการกำหนดค่า Default
phpMySQLEditRecord2.php
<html>
<head>
<title>ThaiCreate.Com PHP & MySQL Tutorial</title>
</head>
<body>
<?php
$objConnect = mysql_connect("localhost","root","root") or die("Error Connect to Database");
$objDB = mysql_select_db("mydatabase");
//*** Update Condition ***//
if($_GET["Action"] == "Save")
{
for($i=1;$i<=$_POST["hdnLine"];$i++)
{
$strSQL = "UPDATE customer SET ";
$strSQL .="CustomerID = '".$_POST["txtCustomerID$i"]."' ";
$strSQL .=",Name = '".$_POST["txtName$i"]."' ";
$strSQL .=",Email = '".$_POST["txtEmail$i"]."' ";
$strSQL .=",CountryCode = '".$_POST["txtCountryCode$i"]."' ";
$strSQL .=",Budget = '".$_POST["txtBudget$i"]."' ";
$strSQL .=",Used = '".$_POST["txtUsed$i"]."' ";
$strSQL .="WHERE CustomerID = '".$_POST["hdnCustomerID$i"]."' ";
$objQuery = mysql_query($strSQL);
}
//header("location:$_SERVER[PHP_SELF]");
//exit();
}
$strSQL = "SELECT * FROM customer ORDER BY CustomerID ASC";
$objQuery = mysql_query($strSQL) or die ("Error Query [".$strSQL."]");
?>
<form name="frmMain" method="post" action="phpMySQLEditRecord2.php?Action=Save">
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<?php
$i =0;
while($objResult = mysql_fetch_array($objQuery))
{
$i = $i + 1;
?>
<tr>
<td><div align="center">
<input type="hidden" name="hdnCustomerID<?php echo $i;?>" size="5" value="<?php echo $objResult["CustomerID"];?>">
<input type="text" name="txtCustomerID<?php echo $i;?>" size="5" value="<?php echo $objResult["CustomerID"];?>">
</div></td>
<td><input type="text" name="txtName<?php echo $i;?>" size="20" value="<?php echo $objResult["Name"];?>"></td>
<td><input type="text" name="txtEmail<?php echo $i;?>" size="20" value="<?php echo $objResult["Email"];?>"></td>
<td><div align="center">
<select name="txtCountryCode<?php echo $i;?>">
<?php
$strSQL2 = "SELECT * FROM country ORDER BY CountryCode ASC";
$objQuery2 = mysql_query($strSQL2) or die ("Error Query [".$strSQL2."]");
while($objResult2 = mysql_fetch_array($objQuery2))
{
if($objResult["CountryCode"] == $objResult2["CountryCode"])
{
$sel = "selected";
}
else
{
$sel = "";
}
?>
<option value="<?php echo $objResult2["CountryCode"];?>" <?php echo $sel;?>><?php echo $objResult2["CountryName"];?></option>
<?php
}
?>
</select>
</div></td>
<td align="right"><input type="text" name="txtBudget<?php echo $i;?>" size="5" value="<?php echo $objResult["Budget"];?>"></td>
<td align="right"><input type="text" name="txtUsed<?php echo $i;?>" size="5" value="<?php echo $objResult["Used"];?>"></td>
</tr>
<?php
}
?>
</table>
<input type="submit" name="submit" value="submit">
<input type="hidden" name="hdnLine" value="<?php echo $i;?>">
</form>
<?php
mysql_close($objConnect);
?>
</body>
</html>
Screenshot
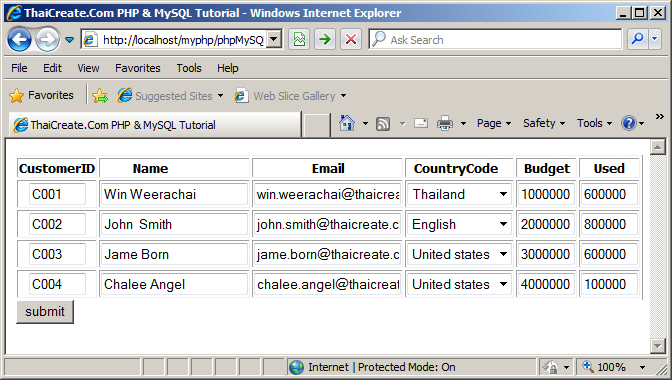
Note! สำหรับ function ของ mysql
เนื่องจาก function ของ mysql จะถูก deprecated ใน version ใหม่ ๆ ฉะนั้นแนะนำให้เปลี่ยนไปใช้ function ของ mysqli
อ่านเพิ่มเติม : PHP MySQL Database (mysqli)
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2009-10-18 17:56:22 /
2017-03-14 15:50:43 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|