PHP MySQL Used Function Database Query |
PHP MySQL Used Function Database Quer ตัวอย่างนี้ผมได้ยกตัวอย่างการเขียน PHP ติดต่อกับ MySQL โดยใช้ Function เข้ามาช่วยจัดการในด้านการเพิ่ม/ลบ/แก้ไข เพื่อความสะดวกและง่ายต่อการแก้ไขหรือพัฒนาโปรแกรมครับ
ตัวอย่าง
phpMySQLFunctionDatabase.php
<?php
/**** function connection to database ****/
$objConnect = mysql_connect("localhost","root","root") or die("Error Connect to Database");
$objDB = mysql_select_db("mydatabase");
/**** function insert record ****/
function fncInsertRecord($strTable,$strField,$strValue)
{
$strSQL = "INSERT INTO $strTable ($strField) VALUES ($strValue) ";
return @mysql_query($strSQL);
}
/**** function select record ****/
function fncSelectRecord($strTable,$strCondition)
{
$strSQL = "SELECT * FROM $strTable WHERE $strCondition ";
$objQuery = @mysql_query($strSQL);
return @mysql_fetch_array($objQuery);
}
/**** function update record ****/
function fncUpdateRecord($strTable,$strCommand,$strCondition)
{
$strSQL = "UPDATE $strTable SET $strCommand WHERE $strCondition ";
return @mysql_query($strSQL);
}
/**** function delete record ****/
function fncDeleteRecord($strTable,$strCondition)
{
$strSQL = "DELETE FROM $strTable WHERE $strCondition ";
return @mysql_query($strSQL);
}
?>
phpMySQLUsedFunctionDatabase.php
<html>
<head>
<title>ThaiCreate.Com PHP & MySQL Tutorial</title>
</head>
<body>
<?php
include("phpMySQLFunctionDatabase.php");
//**** Call to function insert record ****//
$strTable = "customer";
$strField = "CustomerID,Name,Email,CountryCode,Budget,Used";
$strValue = " 'C005','Weerachai Nukitram','[email protected]','TH','2000000','0' ";
$objInsert = fncInsertRecord($strTable,$strField,$strValue);
if(!$objInsert)
{
echo "Record already exist.<br>";
}
else
{
echo "Record inserted.<br>";
}
echo "<br>===========================<br>";
//**** Call to function select record ****//
$strTable = "customer";
$strCondition = " CustomerID = 'C005' ";
$objSelect = fncSelectRecord($strTable,$strCondition);
if(!$objSelect)
{
echo "Record not found<br>";
}
else
{
echo "Customer Detail.<br>";
echo "CustomerID = $objSelect[CustomerID]<br>";
echo "Name = $objSelect[Name]<br>";
echo "Email = $objSelect[Email]<br>";
echo "CountryCode = $objSelect[CountryCode]<br>";
echo "Budget = $objSelect[Budget]<br>";
echo "Used = $objSelect[Used]<br>";
}
echo "<br>===========================<br>";
//**** Call to function update record ****//
$strTable = "customer";
$strCommand = " BUDGET = '4000000' ";
$strCondition = " CustomerID = 'C005' ";
$objUpdate = fncUpdateRecord($strTable,$strCommand,$strCondition);
if(!$objUpdate)
{
echo "Error update record.<br>";
}
else
{
echo "Record updated.<br>";
}
echo "<br>===========================<br>";
//**** Call to function delete record ****//
$strTable = "customer";
$strCondition = " CustomerID = 'C005' ";
$objDelete = fncDeleteRecord($strTable,$strCondition);
if(!$objDelete)
{
echo "Record not delete<br>";
}
else
{
echo "Record deleted.<br>";
}
@mysql_close($objConnect);
?>
</body>
</html>
Screenshot
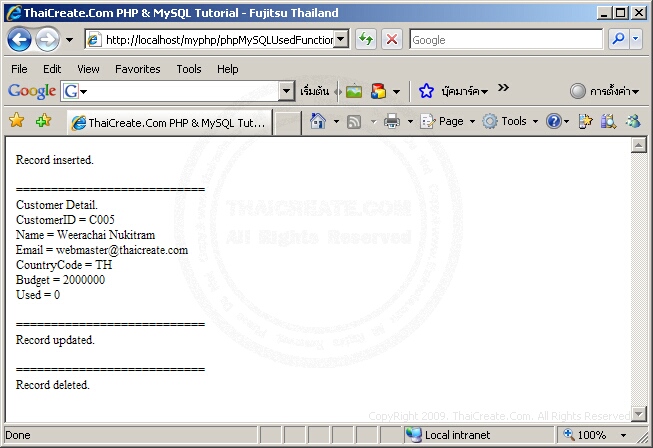

Note! สำหรับ function ของ mysql
เนื่องจาก function ของ mysql จะถูก deprecated ใน version ใหม่ ๆ ฉะนั้นแนะนำให้เปลี่ยนไปใช้ function ของ mysqli
อ่านเพิ่มเติม : PHP MySQL Database (mysqli)
Reference : https://www.thaicreate.com/php-manual/ref.mysql.html
|