PHP SQL Server Edit/Update Data Record(PDO) |
PHP SQL Server Edit/Update Data Record(PDO) บทความนี้จะเป็นตัวอย่างของ PDO กับ sqlsrv การเขียน PHP เพื่อ Edit/Updateหรือแก้ไขข้อมูลใน Database ของ SQL Server โดยใช้ function ต่าง ๆ ของ PDO กับ sqlsrv โดยในตัวอย่างนี้จะใช้การ Update แบบ Parameters Query ซึ่งนี่นคือจะมีความปลอดภัย และลดข้อผิดพลาดที่อาจจะเกิดจากการรับข้อมูลใรูปแบบต่าง ๆ ได้
Syntax รูปแบบการใช้งาน
$sql = "UPDATE table_name SET
Col1 = ? ,
Col2 = ?
WHERE Col3 = ? ";
$params = array('Value1','Value2','Value3');
$stmt = $conn->prepare($sql);
$stmt->execute($params);
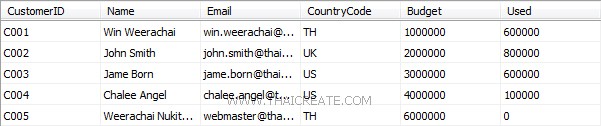
ฐานข้อมูลและตารางของ SQL Server Database
Example ตัวอย่างการเขียน PHP กับ SQL Server เพื่อ Edit/Update หรือแก้ไขข้อมูล
list.php
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server (PDO)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "sa";
$userPassword = "";
$dbName = "mydatabase";
$conn = new PDO("sqlsrv:server=$serverName ; Database = $dbName", $userName, $userPassword);
$sql = "SELECT * FROM customer";
$stmt = $conn->prepare($sql);
$stmt->execute();
?>
<table width="650" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
<th width="50"> <div align="center">Edit </div></th>
</tr>
<?php
while($result = $stmt->fetch( PDO::FETCH_ASSOC ))
{
?>
<tr>
<td><div align="center"><?php echo $result["CustomerID"];?></div></td>
<td><?php echo $result["Name"];?></td>
<td><?php echo $result["Email"];?></td>
<td><div align="center"><?php echo $result["CountryCode"];?></div></td>
<td align="right"><?php echo $result["Budget"];?></td>
<td align="right"><?php echo $result["Used"];?></td>
<td align="center"><a href="edit.php?CustomerID=<?php echo $result["CustomerID"];?>">Edit</a></td>
</tr>
<?php
}
?>
</table>
<?php
$conn = null;
?>
</body>
</html>
edit.php
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server (PDO)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$strCustomerID = null;
if(isset($_GET["CustomerID"]))
{
$strCustomerID = $_GET["CustomerID"];
}
$serverName = "localhost";
$userName = "sa";
$userPassword = "";
$dbName = "mydatabase";
$conn = new PDO("sqlsrv:server=$serverName ; Database = $dbName", $userName, $userPassword);
$sql = "SELECT * FROM customer WHERE CustomerID = ? ";
$params = array($strCustomerID);
$stmt = $conn->prepare($sql);
$stmt->execute($params);
$result = $stmt->fetch( PDO::FETCH_ASSOC );
?>
<form action="save.php" name="frmAdd" method="post">
<table width="284" border="1">
<tr>
<th width="120">CustomerID</th>
<td width="238"><input type="hidden" name="txtCustomerID" value="<?php echo $result["CustomerID"];?>"><?php echo $result["CustomerID"];?></td>
</tr>
<tr>
<th width="120">Name</th>
<td><input type="text" name="txtName" size="20" value="<?php echo $result["Name"];?>"></td>
</tr>
<tr>
<th width="120">Email</th>
<td><input type="text" name="txtEmail" size="20" value="<?php echo $result["Email"];?>"></td>
</tr>
<tr>
<th width="120">CountryCode</th>
<td><input type="text" name="txtCountryCode" size="2" value="<?php echo $result["CountryCode"];?>"></td>
</tr>
<tr>
<th width="120">Budget</th>
<td><input type="text" name="txtBudget" size="5" value="<?php echo $result["Budget"];?>"></td>
</tr>
<tr>
<th width="120">Used</th>
<td><input type="text" name="txtUsed" size="5" value="<?php echo $result["Used"];?>"></td>
</tr>
</table>
<input type="submit" name="submit" value="submit">
</form>
<?php
$conn = null;
?>
</body>
</html>
save.php
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server (PDO)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "sa";
$userPassword = "";
$dbName = "mydatabase";
$conn = new PDO("sqlsrv:server=$serverName ; Database = $dbName", $userName, $userPassword);
$sql = "UPDATE customer SET
Name = ? ,
Email = ? ,
CountryCode = ? ,
Budget = ? ,
Used = ?
WHERE CustomerID = ? ";
$params = array($_POST["txtName"], $_POST["txtEmail"], $_POST["txtCountryCode"], $_POST["txtBudget"], $_POST["txtUsed"],$_POST["txtCustomerID"]);
$stmt = $conn->prepare($sql);
$stmt->execute($params);
if( $stmt->rowCount() ) {
echo "Record update successfully";
}
$conn = null;
?>
</body>
</html>
Screenshot
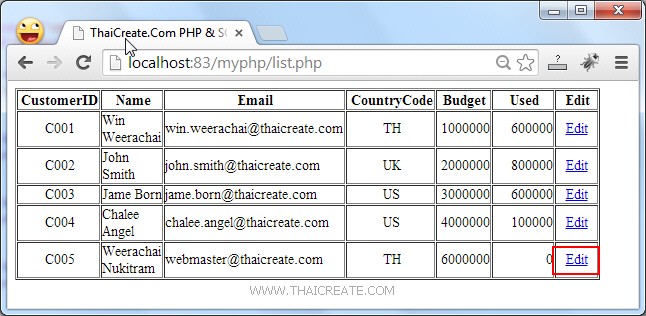
แสดงรายการข้อมูล คลิก Record ที่จะ Update
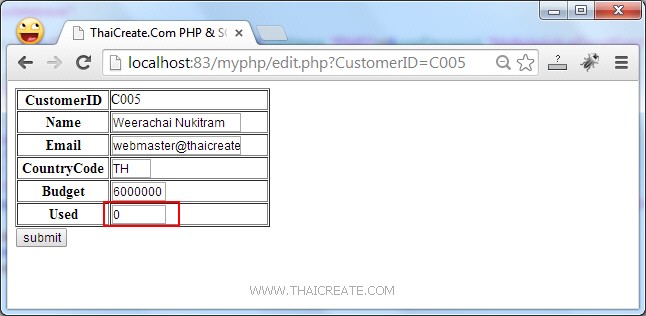
ข้อมูลก่อนการแก้ไข
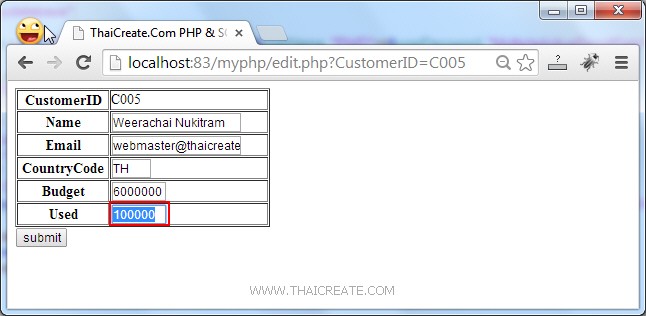
แก้ไขข้อมูล หรือเปลี่ยนแปลงข้อมูลใหม่
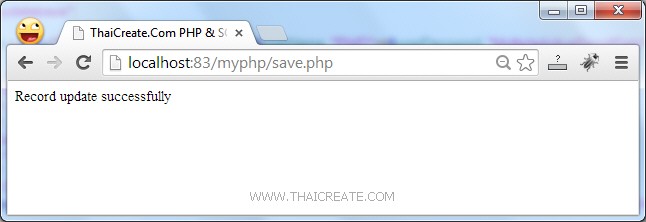
บันทึกการแก้ไข
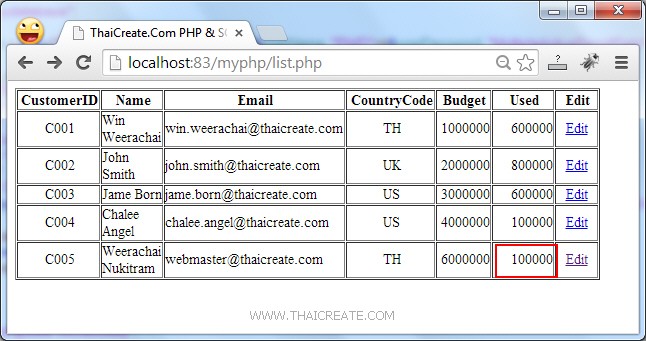
เมือ่กลับมาหน้าที่แสดงรายการ จะเห็นว่ารายการข้อมูลถูกแก้ไขเรียบร้อยแล้ว
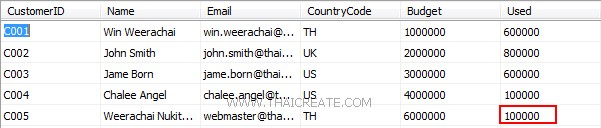
เมื่อกลับไปดูที่ Table บน SQL Server Database ก็จะพบว่าข้อมูลถูกแก้ไขเช่นเดียวกัน
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-09-05 12:51:17 /
2017-03-25 01:24:14 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|