PHP SQL Server (sqlsrv) : UTF-8 Encoding (Multiple Language/รองรับภาษาไทย) |
PHP SQL Server (sqlsrv) : UTF-8 Encoding (Multiple Language/รองรับภาษาไทย) ในการเขียน PHP เพื่อติดต่อกับ Database ของ SQL Server ด้วยฟิังก์ชั่นของ sqlsrv ปัญหาที่หลาย ๆ คนเจอคือมีปัญหาเรื่องภาษาไทย และ ภาษาอื่น ๆ เช่น ญี่ปุ่น , จีน หรืออื่น ๆ ซึ่งวิธีการแก้ไขนั้นต้องบอกว่าปัญหาเหล่านี้ในตัว PHP , Connector และ Database ได้ถูกปรับปรุงและแก้ไขได้ 100% แต่เราจะต้องทำการ Config ค่าและเรียกใช้มันให้เป็น มันถึงจะทำงานได้อย่างถูกต้อง และ รองรับได้ทุกภาษาที่ต้องการ
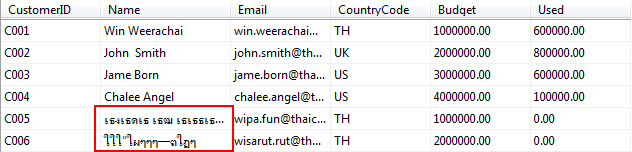
การทำงานที่ถูกต้องจะต้องแสดงผลภาษาได้อย่างถูกต้อง ทั้งดูใน Database และแสดงบนหน้าเว็บ ในกรณีที่แสดงผลผิดเพี้ยนที่ใดที่หนึ่ง แสดงว่ามีการกำหนดค่าที่ผิดแน่นอน และมันจะเป็นปัญหาสะสมในอนาคตแน่นอน ในการใช้งานที่ถูกต้องจะต้องมีขั้นตอนดังนี้
Step 1 : กำหนด DataType ของ Column เป็นแบบ NVARCHAR()
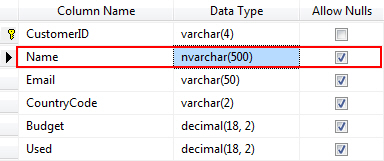
Step 2 : ในหน้าเว็บจะต้องกำหนด meta ในส่วนของ header โดยให้ charset=utf-8
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
Step 3 : เพิ่ม "CharacterSet" => 'UTF-8' ในส่วนของ Connection
$connectionInfo = array("Database"=>$dbName, "UID"=>$userName, "PWD"=>$userPassword,
"MultipleActiveResultSets"=>true,"CharacterSet" => 'UTF-8');
โดยจะต้องกำหนดให้ถูกต้องทั้ง 3 ส่วนนี้ให้ถูกก่อน
Note!!. ในกรณีที่ข้อมูลเก่าก่อนการใช้งาน ยังเป็นข้อมูลที่ผิดอยู่ จะไม่สามารถใช้ขั้นตอนนี้ในการแสดงผลภาษาไทยให้ถูกต้องได้ ซึ่งจะต้องกลับไปแก้ไขข้อมูลเก่าให้ถูกต้อง

Example ตัวอย่างการ Insert และแสดงข้อมูลภาษาไทยด้วย UTF-8 (sqlsrv)
add.php
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>ThaiCreate.Com PHP & SQL Server (sqlsrv)</title>
</head>
<body>
<form action="save.php" name="frmAdd" method="post">
<table width="284" border="1">
<tr>
<th width="120">CustomerID</th>
<td width="238"><input type="text" name="txtCustomerID" size="5"></td>
</tr>
<tr>
<th width="120">Name</th>
<td><input type="text" name="txtName" size="20"></td>
</tr>
<tr>
<th width="120">Email</th>
<td><input type="text" name="txtEmail" size="20"></td>
</tr>
<tr>
<th width="120">CountryCode</th>
<td><input type="text" name="txtCountryCode" size="2"></td>
</tr>
<tr>
<th width="120">Budget</th>
<td><input type="text" name="txtBudget" size="5"></td>
</tr>
<tr>
<th width="120">Used</th>
<td><input type="text" name="txtUsed" size="5"></td>
</tr>
</table>
<input type="submit" name="submit" value="submit">
</form>
</body>
</html>
save.php
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>ThaiCreate.Com PHP & SQL Server (sqlsrv)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost\SQL2012";
$userName = "sa";
$userPassword = '';
$dbName = "mydatabase";
$connectionInfo = array("Database"=>$dbName, "UID"=>$userName, "PWD"=>$userPassword,
"MultipleActiveResultSets"=>true,"CharacterSet" => 'UTF-8');
$conn = sqlsrv_connect( $serverName, $connectionInfo);
if( $conn === false ) {
die( print_r( sqlsrv_errors(), true));
}
$sql = "INSERT INTO customer (CustomerID, Name, Email, CountryCode, Budget, Used) VALUES (?, ?, ?, ?, ?, ?)";
$params = array($_POST["txtCustomerID"], $_POST["txtName"], $_POST["txtEmail"], $_POST["txtCountryCode"], $_POST["txtBudget"], $_POST["txtUsed"]);
$stmt = sqlsrv_query( $conn, $sql, $params);
if( $stmt === false ) {
die( print_r( sqlsrv_errors(), true));
}
else
{
echo "Record add successfully";
}
sqlsrv_close($conn);
?>
</body>
</html>
list.php
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>ThaiCreate.Com PHP & SQL Server (sqlsrv)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost\SQL2012";
$userName = "sa";
$userPassword = "";
$dbName = "mydatabase";
$connectionInfo = array("Database"=>$dbName, "UID"=>$userName, "PWD"=>$userPassword,
"MultipleActiveResultSets"=>true,"CharacterSet" => 'UTF-8');
$conn = sqlsrv_connect( $serverName, $connectionInfo);
if( $conn === false ) {
die( print_r( sqlsrv_errors(), true));
}
$stmt = "SELECT * FROM customer";
$query = sqlsrv_query($conn, $stmt);
?>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<?php
while($result = sqlsrv_fetch_array($query, SQLSRV_FETCH_ASSOC))
{
?>
<tr>
<td><div align="center"><?php echo $result["CustomerID"];?></div></td>
<td><?php echo $result["Name"];?></td>
<td><?php echo $result["Email"];?></td>
<td><div align="center"><?php echo $result["CountryCode"];?></div></td>
<td align="right"><?php echo $result["Budget"];?></td>
<td align="right"><?php echo $result["Used"];?></td>
</tr>
<?php
}
?>
</table>
<?php
sqlsrv_close($conn);
?>
</body>
</html>

Screenshot
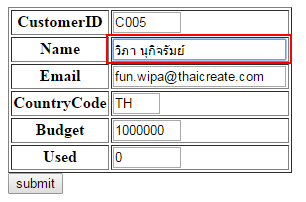
ทดสอบการ Insert ข้อมูลภาษาไทย
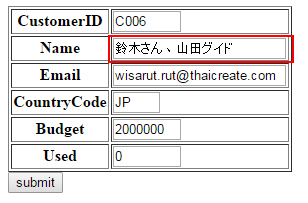
ทดสอบการ Insert ข้อมูลภาษาญี่ปุ่น
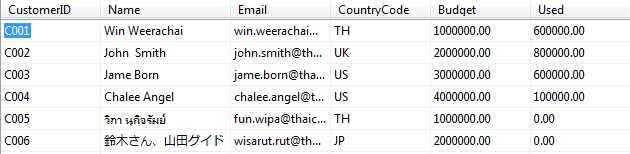
ใน Table จัดเก็บข้อมูลอย่างถูกต้องทั้งภาษาไทยและภาษาญี่ปุ่น
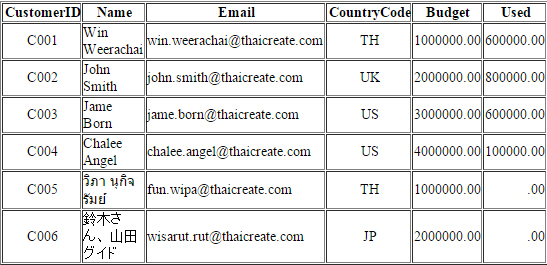
เมื่อดึงมาแสดงผลก็สามารถแสดงผลได้อย่างถูกต้อง
|