WebSocket ตอนที่ 6 : การ รับ-ส่ง ข้อมูล Real Time และการจัดเก็บลงใน Database ด้วย PHP |
WebSocket ตอนที่ 6 : การ รับ-ส่ง ข้อมูล Real Time และการจัดเก็บลงใน Database ด้วย PHP บทความนี้จะเป็นตัวอย่างการประยุกต์ใช้ WebSocket ในการรับ-ส่งข้อมูลแบบ Real time และการจัดเก็บ ข้อมูลที่รับส่งลงใน MySQL หรือ MariaDB Database จากนั้จะนำค่า Result ทั้งหมดจาก Database แปลงเป็น JSON เพื่อส่ง Result ทั้งหมดไปยัง Client และ Client ก็จะรับค่า JSON มาแสดงในรูปแบบของ HTML Table หรือ Grid
WebSocket Real time and MySQL/MariaDb Database
สร้าง MySQL หรือ MariaDB Database
CREATE TABLE `mytable` (
`id` int(11) NOT NULL,
`name` varchar(150) NOT NULL,
`email` varchar(150) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
--
-- Indexes for dumped tables
--
--
-- Indexes for table `mytable`
--
ALTER TABLE `mytable`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `mytable`
--
ALTER TABLE `mytable`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
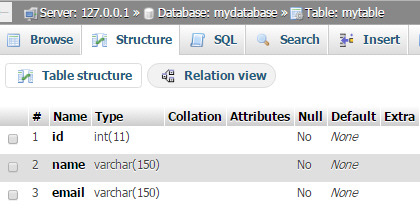
โครงสร้างของ Table ที่ได้
สิ่งที่เราจะเพิ่มขึ้นมาคือ Connection.php
เพิ่มคำสั่งสำหรับจัดเก็บลงใน MySQL
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
$sql = "INSERT INTO mytable (name, email)
VALUES ('".$obj->name."','".$obj->email."')";
$query = mysqli_query($conn,$sql);
แปลง Result ที่ได้จาก MySQL ทั้งหมดเป็น JSON เพื่อส่งไปยังทุกๆ Client
$myArray = array();
$sql = "SELECT * FROM mytable ORDER BY id ASC ";
$query = mysqli_query($conn,$sql);
while($result=mysqli_fetch_array($query,MYSQLI_ASSOC))
{
$myArray[] = $result;
}
$json = json_encode($myArray);
Push หรือส่งข้อความไปให้ Client
$client->send($json); //*** send json
Code ทั้งหมดของ Connection.php
<?php
namespace MyApp;
use Ratchet\MessageComponentInterface;
use Ratchet\ConnectionInterface;
class Connection implements MessageComponentInterface {
protected $clients;
public function __construct() {
$this->clients = new \SplObjectStorage;
echo "Congratulations! the server is now running\n";
}
public function onOpen(ConnectionInterface $conn) {
// Store the new connection to send messages to later
$this->clients->attach($conn);
echo "New connection! ({$conn->resourceId})\n";
}
public function onMessage(ConnectionInterface $from, $msg) {
$numRecv = count($this->clients) - 1;
echo sprintf('Connection %d sending message "%s" to %d other connection%s' . "\n"
, $from->resourceId, $msg, $numRecv, $numRecv == 1 ? '' : 's');
//*** Insert data
$obj = json_decode($msg);
$serverName = "localhost";
$userName = "root";
$userPassword = "";
$dbName = "mydatabase";
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
$sql = "INSERT INTO mytable (name, email)
VALUES ('".$obj->name."','".$obj->email."')";
$query = mysqli_query($conn,$sql);
//*** Get data to json
$myArray = array();
$sql = "SELECT * FROM mytable ORDER BY id ASC ";
$query = mysqli_query($conn,$sql);
while($result=mysqli_fetch_array($query,MYSQLI_ASSOC))
{
$myArray[] = $result;
}
$json = json_encode($myArray);
mysqli_close($conn);
foreach ($this->clients as $client) {
if ($from !== $client) {
// The sender is not the receiver, send to each client connected
$client->send($json); //*** send json
}
}
}
public function onClose(ConnectionInterface $conn) {
// The connection is closed, remove it, as we can no longer send it messages
$this->clients->detach($conn);
echo "Connection {$conn->resourceId} has disconnected\n";
}
public function onError(ConnectionInterface $conn, \Exception $e) {
echo "An error has occurred: {$e->getMessage()}\n";
$conn->close();
}
}
?>
ในการแก้ไขที่ Server จะต้องปิดและรัน Services ใหม่ทุกครั้ง

ในส่วนของ Client เราจะสร้างไฟล์ขึ้นมา 2 หน้าคือ post.php (ไว้ส่งข้อควาใ), index.php (ไว้แสดงข้อความ)
post.php
<!DOCTYPE html>
<html>
<head>
<title>PHP Real Time</title>
<link type="text/css" rel="stylesheet" href="style.css" />
<script src="jquery-1.7.2.min.js"></script>
</head>
<style type="text/css">
body {
font:12px arial;
color: #222;
text-align:center;
padding:35px;
}
</style>
<body>
<div id="ws_support"></div>
<div id="connect"></div>
<div id="wrapper">
Input Name and Email!<br><br>
Name <input name="txtName" type="text" id="txtName" size="20"/>
Email <input name="txtEmail" type="text" id="txtEmail" size="20"/>
<input name="btnSend" type="button" id="btnSend" value="Send" />
</body>
</html>
<script language="javascript">
var socket;
function webSocketSupport()
{
if (browserSupportsWebSockets() === false) {
$('#ws_support').html('<h2>Sorry! Your web browser does not supports web sockets</h2>');
$('#wrapper').hide();
return;
}
// Open Connection
socket = new WebSocket('ws://localhost:8089');
socket.onopen = function(e) {
$('#connect').html("You have have successfully connected to the server<br><br>");
};
socket.onmessage = function(e) {
//
};
socket.onerror = function(e) {
onError(e)
};
}
function onError(e) {
alert('Error!!');
}
function doSend() {
var jsonSend = JSON.stringify({ "name": $('#txtName').val(), "email" : $('#txtEmail').val() });
// {"name":"wisarut","email":"[email protected]"}
if ($('#txtName').val() == '') {
alert('Enter your [Name]');
$('#txtName').focus();
return '';
} else if ($('#txtEmail').val() == '') {
alert('Enter your [Email]');
$('#txtEmail').focus();
return '';
}
socket.send(jsonSend);
$('#txtName').val('');
$('#txtEmail').val('');
alert('Done!!');
}
function browserSupportsWebSockets() {
if ("WebSocket" in window)
{ return true; }
else
{ return false; }
}
$(document).ready(function() {
webSocketSupport();
});
$('#btnSend').click(function () {
doSend();
});
</script>
ในไฟล์ post.php จะไม่มีอะไรมาก มีเพพียงการเชื่อมต่อและส่งข้อมูลไปยัง Server เท่านั้น
index.php
<!DOCTYPE html>
<html>
<head>
<title>PHP WebSocket</title>
<link type="text/css" rel="stylesheet" href="style.css" />
<script src="jquery-1.7.2.min.js"></script>
</head>
<style type="text/css">
body {
font:12px arial;
color: #222;
text-align:center;
padding:35px;
}
</style>
<body>
<div id="ws_support"></div>
<div id="connect"></div>
<div id="wrapper">
<center>
<table width="400" border="1" id="myTable">
<!-- head table -->
<thead>
<tr>
<td width="200"> <div align="center">Name </div></td>
<td width="200"> <div align="center">Email </div></td>
</tr>
</thead>
<!-- body dynamic rows -->
<tbody></tbody>
</table>
</center>
</div>
</body>
</html>
<script language="javascript">
var socket;
function webSocketSupport()
{
if (browserSupportsWebSockets() === false) {
$('#ws_support').html('<h2>Sorry! Your web browser does not supports web sockets</h2>');
$('#wrapper').hide();
return;
}
// Open Connection
socket = new WebSocket('ws://localhost:8089');
socket.onopen = function(e) {
$('#connect').html("You have have successfully connected to the server<br><br>");
};
socket.onmessage = function(e) {
onMessage(e)
};
socket.onerror = function(e) {
onError(e)
};
}
function onMessage(e) {
$('#myTable > tbody:last').empty();
var obj = jQuery.parseJSON(e.data);
$.each(obj, function (key, val) {
var name = val["name"];
var email = val["email"];
var tr = "<tr>";
tr = tr + "<td>" + name + "</td>";
tr = tr + "<td>" + email + "</td>";
tr = tr + "</tr>";
$('#myTable > tbody:last').append(tr);
});
}
function onError(e) {
alert('Error!!');
}
function browserSupportsWebSockets() {
if ("WebSocket" in window)
{ return true; }
else
{ return false; }
}
$(document).ready(function() {
webSocketSupport();
});
</script>
ในส่วนของไฟล์ index.php จะรับข้อความจาก Server ที่อยู่ในรูปแบบของ JSON จากนั้น decode มันมาแล้วแสดงบน HTML Table
ทดสอบการทำงานบ
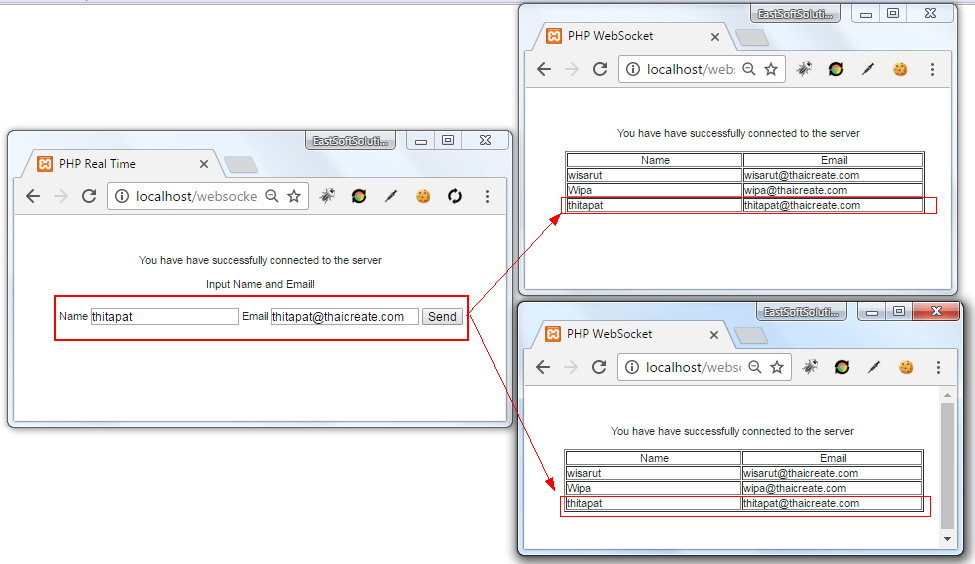
ทดสอบการส่งข้อมูล และ แสดงข้อมูลไปยังทุกๆ Client
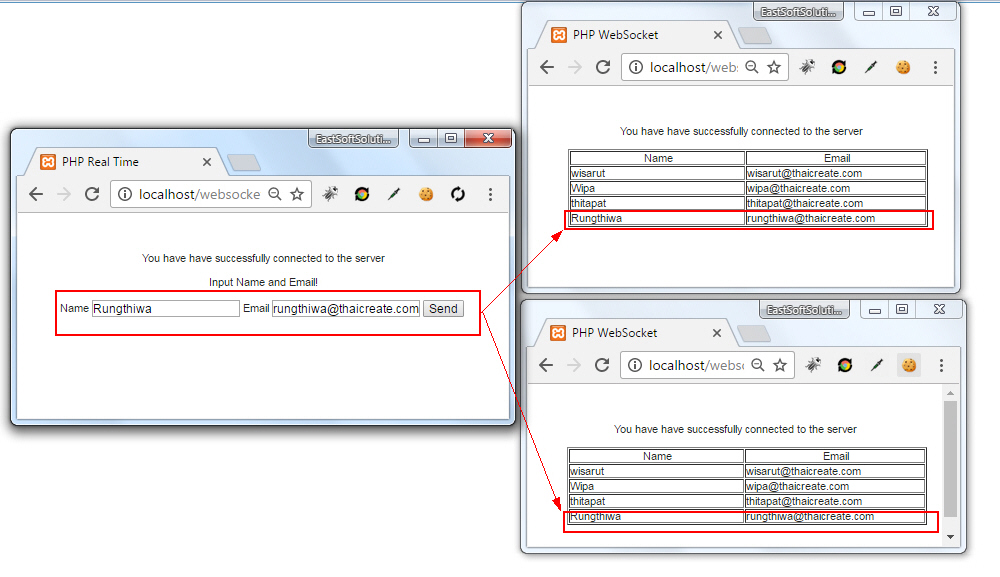
ทดสอบการส่งข้อมูล และ แสดงข้อมูลไปยังทุกๆ Client
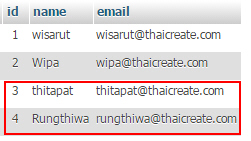
เมื่อดูใน Database จะเห็นว่าข้อมูลถูกจัดเก็บลงใน MySql หรือ MariaDB
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2017-01-26 13:24:42 /
2017-03-25 01:59:55 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|