Update Entity : Update Rows in Database (LINQ, Entity Framework) |
Update Entity : Update Rows in Database (LINQ, Entity Framework) ในการ Update Entity ด้วย LINQ to Entities บน Entity Framework จะแตกกต่างกัน SQL Statement ตรงที่ ปกติแล้ว SQL Statement เราจะเขียน Update แล้ว Set รายการ Column และเพิ่มเงื่อนไข Where ให้อัพเดดเฉพาะ Record ที่ต้องการ แต่บน Entity Framework เราจะต้องทำการ Select รายการข้อมูลขึ้นมาก่อน และหลังจากที่ได้รายการข้อมูลล้วเราค่อยทำการเปลี่ยนแปลงค่าหรืออัพเดดค่าใหม่ แล้วค่อย Save เข้าไปใหม่
Note!! : ข้อจำกัดของ Entity Framework คือ ไม่สามารถอัพเดดฟิวด์ที่เป็น Key หรือ Primary Key ได้
Example 1 : การ Update Entity หรือ Update ข้อมูลที่มีเพียงรายการหรือ Record เดียว
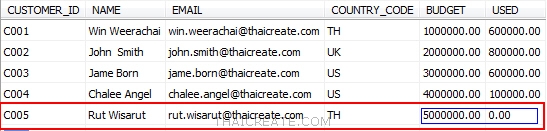
ข้อมูลที่ต้องการอัพเดดต้องการอัพเดด USED ของ CUSTOMER_ID = C005
Code (C#)
private void frmMain_Load(object sender, EventArgs e)
{
// Create new entities from Entities
using (var db = new myDatabaseEntities())
{
// Update CUSTOMER
string strCustomerID = "C005";
// Update Statement
var update = db.CUSTOMER.Where(o => o.CUSTOMER_ID == strCustomerID).FirstOrDefault();
if (update != null)
{
update.BUDGET = 6000000;
update.USED = 100000;
}
db.SaveChanges();
}
}
Code (VB.Net)
Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
' Create new entities from Entities
Using db = New myDatabaseEntities()
' Update CUSTOMER
Dim strCustomerID As String = "C005"
' Update Statement
Dim update = db.CUSTOMER.Where(Function(o) o.CUSTOMER_ID = strCustomerID).FirstOrDefault()
If update IsNot Nothing Then
update.BUDGET = 6000000
update.USED = 100000
End If
db.SaveChanges()
End Using
End Sub
สามารถเขียนได้อีกรูปบบคือ
Code (C#)
private void frmMain_Load(object sender, EventArgs e)
{
// Create new entities from Entities
using (var db = new myDatabaseEntities())
{
// Update CUSTOMER
string strCustomerID = "C005";
// Update Statement
var update = (from c in db.CUSTOMER
where c.CUSTOMER_ID == strCustomerID
select c).FirstOrDefault();
if (update != null)
{
update.BUDGET = 6000000;
update.USED = 100000;
}
db.SaveChanges();
}
}
Code (VB.Net)
Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
' Create new entities from Entities
Using db = New myDatabaseEntities()
' Update CUSTOMER
Dim strCustomerID As String = "C005"
' Update Statement
Dim update = (From c In db.CUSTOMER Where c.CUSTOMER_ID = strCustomerID
Select c).FirstOrDefault()
If update IsNot Nothing Then
update.BUDGET = 6000000
update.USED = 100000
End If
db.SaveChanges()
End Using
End Sub
Screenshot
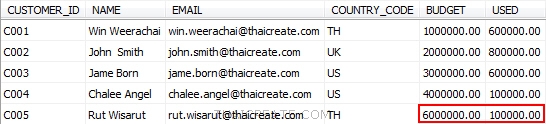
Example 2 : การ Update Entity หรือ Update ข้อมูลในหลาย ๆ รายการ หรือหลาย Record
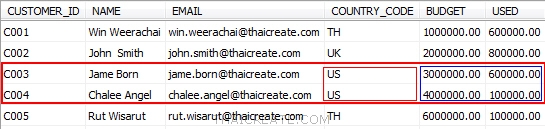
ข้อมูลที่ต้องการอัพเดด โดยเลือกอัพเดดรายการ BUDGET และ USED ที่ COUNTRY_CODE ตรงกับเงื่อนไขที่เขียนขึ้น
Code (C#)
private void frmMain_Load(object sender, EventArgs e)
{
// Create new entities from Entities
using (var db = new myDatabaseEntities())
{
// Update CUSTOMER
string strCountryCode = "US";
// Update Statement
var update = (from c in db.CUSTOMER
where c.COUNTRY_CODE == strCountryCode
select c).ToList();
if (update.Count() > 0)
{
// Loop update
foreach (var item in update)
{
item.BUDGET = 5000000;
item.USED = 200000;
}
}
db.SaveChanges();
}
}
Code (VB.Net)
Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
' Create new entities from Entities
Using db = New myDatabaseEntities()
' Update CUSTOMER
Dim strCountryCode As String = "US"
' Update Statement
Dim update = (From c In db.CUSTOMER Where c.COUNTRY_CODE = strCountryCode
Select c).ToList()
If update.Count() > 0 Then
' Loop update
For Each item In update
item.BUDGET = 5000000
item.USED = 200000
Next
End If
db.SaveChanges()
End Using
End Sub
เขียนได้อีกวิธี ซึ่งทั้ง 2 วิธ๊นี้ได้ผลลัพธ์ที่ไม่แตกต่างกัน
Code (C#)
private void frmMain_Load(object sender, EventArgs e)
{
// Create new entities from Entities
using (var db = new myDatabaseEntities())
{
// Update CUSTOMER
string strCountryCode = "US";
// Update Statement
var update = db.CUSTOMER.Where(o => o.COUNTRY_CODE == strCountryCode).ToList();
if (update.Count() > 0)
{
// Loop update
update.ForEach(o => { o.BUDGET = 5000000; o.USED = 100000; });
}
db.SaveChanges();
}
}
Code (VB.Net)
Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
' Create new entities from Entities
Using db = New myDatabaseEntities()
' Update CUSTOMER
Dim strCountryCode As String = "US"
' Update Statement
Dim update = db.CUSTOMER.Where(Function(o) o.COUNTRY_CODE = strCountryCode).ToList()
If update.Count() > 0 Then
' Loop update
update.ForEach(Function(o) {o.BUDGET = 5000000, o.USED = 100000})
End If
db.SaveChanges()
End Using
End Sub
Screenshot
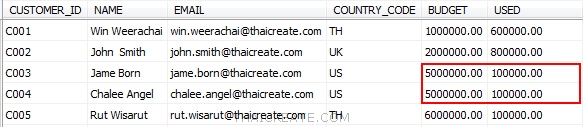
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2015-10-02 21:17:44 /
2017-03-24 23:00:09 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|