Windows Form ตอนที่ 6 : Delete Data (LINQ, Entity Framework) |
Windows Form ตอนที่ 6 : Delete Data (LINQ, Entity Framework) ในตัวอย่างของตอนที่ 6 นี้จะเป็นการใช้ Entity Framework อ่านข้อมูลจาก Model Entities แสดงผลบน DataGridView และคลิกเลือกรายการลบน DataGridView เพื่อลบหรือ Delete ข้อมูล
Example : การใช้งาน LINQ to Entities แสดงผลบน DataGridView และเลือกรายการที่จะ Delete (ลบ)
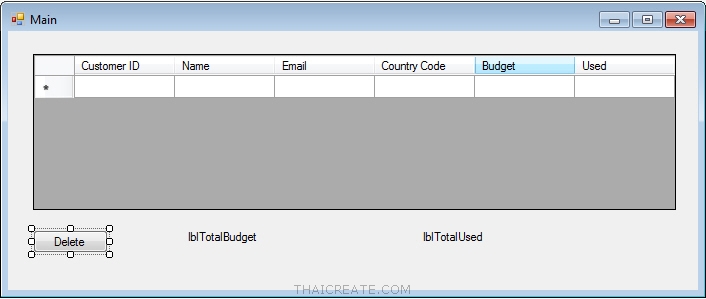
ออกแบบ Form ด้วย DataGridView และปุ่ม Delete สำหรับลบข้อมูล
Code frmMain.cs (C#)
public partial class frmMain : Form
{
public frmMain()
{
InitializeComponent();
}
private void frmMain_Load(object sender, EventArgs e)
{
BindData();
}
private void BindData()
{
// Create new entities Object
using (var db = new myDatabaseEntities())
{
// Get data from CUSTOMER
var ds = (from c in db.CUSTOMER
select new
{
c.CUSTOMER_ID,
c.NAME,
c.EMAIL,
c.COUNTRY_CODE,
c.BUDGET,
c.USED
}).ToList();
// Assign to DataGridView
if (ds.Count > 0)
{
// New DataSource
this.myDataGridView.AutoGenerateColumns = false;
this.myDataGridView.AllowUserToAddRows = false;
this.myDataGridView.SelectionMode = DataGridViewSelectionMode.FullRowSelect;
this.myDataGridView.DataSource = ds;
}
// Summary Budget Column
decimal iSumBudget = 0;
foreach (DataGridViewRow row in this.myDataGridView.Rows)
{
iSumBudget = iSumBudget + Convert.ToDecimal(row.Cells["BUDGET"].Value);
}
this.lblTotalBudget.Text = String.Format("Total Budget : {0}", iSumBudget.ToString("#,###.00"));
// Summary Used Column
decimal iSumUsed = 0;
foreach (DataGridViewRow row in this.myDataGridView.Rows)
{
iSumUsed = iSumUsed + Convert.ToDecimal(row.Cells["USED"].Value);
}
this.lblTotalUsed.Text = String.Format("Total Used : {0}", iSumUsed.ToString("#,###.00"));
}
}
private void btnDelete_Click(object sender, EventArgs e)
{
string strCustomerID = this.myDataGridView.Rows[this.myDataGridView.CurrentCell.RowIndex].Cells["CUSTOMER_ID"].Value.ToString();
if (MessageBox.Show(string.Format("Are you sure to delete {0}?", strCustomerID), "Confirm.",
MessageBoxButtons.YesNo, MessageBoxIcon.Question,
MessageBoxDefaultButton.Button1) == DialogResult.Yes)
{
// Create new entities from Entities
using (var db = new myDatabaseEntities())
{
// Delete CUSTOMER
var del = (from c in db.CUSTOMER
where c.CUSTOMER_ID == strCustomerID
select c).FirstOrDefault();
if (del != null)
{
db.CUSTOMER.Remove(del);
}
db.SaveChanges();
MessageBox.Show(string.Format("Delete {0} Successfully.", strCustomerID));
}
BindData();
}
}
}
Screenshot
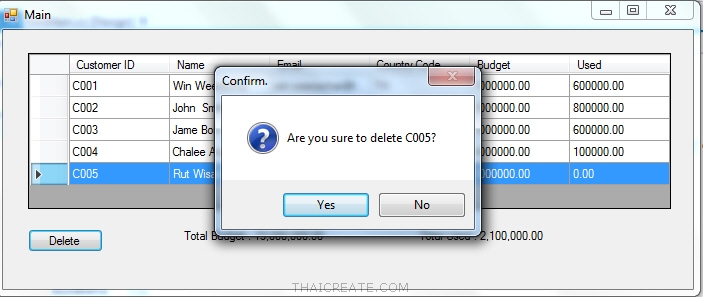
เลือกรายการที่ต้องการจะลบ และคลิกที Delete ซึ่งระบบจะแสดง Dialog ให้ Confirm การ Delete ข้อมูล
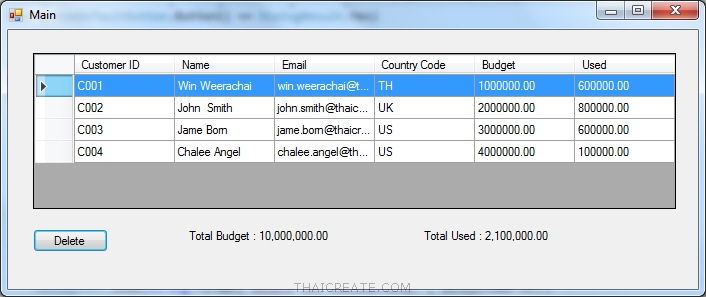
หลังจากที่ Delete เรียบร้อยแล้วจะทำการ Bind ข้อมูลใน DataGridView ใหม่
Code frmMain.vb (VB.Net)
Public Class frmMain
Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
BindData()
End Sub
Private Sub BindData()
' Create new entities Object
Using db = New myDatabaseEntities()
' Get data from CUSTOMER
Dim ds = (From c In db.CUSTOMER
Select New With { _
c.CUSTOMER_ID, _
c.NAME, _
c.EMAIL, _
c.COUNTRY_CODE, _
c.BUDGET, _
c.USED _
}).ToList()
' Assign to DataGridView
If ds.Count > 0 Then
' New DataSource
Me.myDataGridView.AutoGenerateColumns = False
Me.myDataGridView.AllowUserToAddRows = False
Me.myDataGridView.SelectionMode = DataGridViewSelectionMode.FullRowSelect
Me.myDataGridView.DataSource = ds
End If
' Summary Budget Column
Dim iSumBudget As Decimal = 0
For Each row As DataGridViewRow In Me.myDataGridView.Rows
iSumBudget = iSumBudget + Convert.ToDecimal(row.Cells("BUDGET").Value)
Next
Me.lblTotalBudget.Text = String.Format("Total Budget : {0}", iSumBudget.ToString("#,###.00"))
' Summary Used Column
Dim iSumUsed As Decimal = 0
For Each row As DataGridViewRow In Me.myDataGridView.Rows
iSumUsed = iSumUsed + Convert.ToDecimal(row.Cells("USED").Value)
Next
Me.lblTotalUsed.Text = String.Format("Total Used : {0}", iSumUsed.ToString("#,###.00"))
End Using
End Sub
Private Sub btnDelete_Click(sender As Object, e As EventArgs) Handles btnDelete.Click
Dim strCustomerID As String = Me.myDataGridView.Rows(Me.myDataGridView.CurrentCell.RowIndex).Cells("CUSTOMER_ID").Value.ToString()
If MessageBox.Show(String.Format("Are you sure to delete {0}?", strCustomerID), "Confirm.", MessageBoxButtons.YesNo, MessageBoxIcon.Question, MessageBoxDefaultButton.Button1) = DialogResult.Yes Then
' Create new entities from Entities
Using db = New myDatabaseEntities()
' Delete CUSTOMER
Dim del = (From c In db.CUSTOMER
Where c.CUSTOMER_ID = strCustomerID
Select c).FirstOrDefault()
If Not IsNothing(del) Then
db.CUSTOMER.Remove(del)
End If
db.SaveChanges()
MessageBox.Show(String.Format("Delete {0} Successfully.", strCustomerID))
End Using
BindData()
End If
End Sub
End Class
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2015-10-02 21:20:50 /
2017-03-24 23:03:26 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|