ตอนที่ 5 : Google Maps API การปักหมุด (Marker) บนแผนที่ จาก Lat,Lon และรายละเอียด |
ตอนที่ 5 : Google Maps API การปักหมุด (Marker) บนแผนที่ จาก Lat,Lon และรายละเอียด ในการใช้งาน Google Map สิ่งที่สำคัญและขาดไม่ได้คือ การปักหมุด (Marker) ลงบนแผนที่ เป็นวิธีการระบุหรือกำหนดสถานที่เป้าหมาย ที่ต้องการระบุลงไป โดยการจะปักหมุด (Maker) ลงในแผนที่จะมีการอ้างถึงค่า Latitude และ Longitude ตำแหน่งที่จะปัก ซึ่งเมื่อได้ค่านี้แล้ว ก็เพียงเพิ่มคำสั่งให้ปักลงไปใน Map ก็จะสามารถระบุตำแหน่งของสถานที่ ที่ต้องการได้
Google Maps API and Marker
ในการใช้งาน Google Map การปักหมุดถือว่าเป็นฟีเจอร์ที่มีการจำกัดการใช้งาน ส่วนว่าจะปักได้กี่จุดนั้น จะต้องไปหาข้อมูลเพิ่มเติม แต่ในการใช้งานทั่วๆ ไปที่ไม่ได้อยู่ในขอบเขตของธุรกิจ ก็จะสามารถใช้งานได้ฟรี ไม่ต้องเสียค่าบริการแต่อย่างใด แต่ทั้งนี้ต้องอยู่ขึ้นกับจำนวน Request และการ Limit ค่าอื่นๆ ได้
รูปแบบการปักหมุดบน Google Mp API
สร้าง Map ปกติ
var mapOptions = {
center: {lat: 13.847860, lng: 100.604274},
zoom: 15,
}
var maps = new google.maps.Map(document.getElementById("map"),mapOptions);
กำหนดจุดที่จะปักหมุด (Marker) ด้วยค่า Latitude และ Longitude
var marker = new google.maps.Marker({
position: new google.maps.LatLng(13.847616, 100.604736),
map: maps,
title: 'ถนน ลาดปลาเค้า'
});
เพียงเท่านี้ก็จะได้หมุดหรือ Marker บน Google Map แบบง่ายๆ
Ex 1 : การปักหมุด Marker บน Google Map แบบง่าย ๆ
<script>
function initMap() {
var mapOptions = {
center: {lat: 13.847860, lng: 100.604274},
zoom: 15,
}
var maps = new google.maps.Map(document.getElementById("map"),mapOptions);
var marker = new google.maps.Marker({
position: new google.maps.LatLng(13.847616, 100.604736),
map: maps,
title: 'ถนน ลาดปลาเค้า'
});
}
</script>
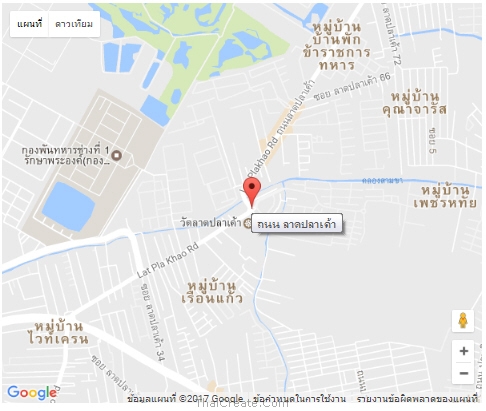
Ex 2 : การปักหมุด Marker และการเปลี่ยน Icon ที่ปักลง Map
<script>
function initMap() {
var mapOptions = {
center: {lat: 13.847860, lng: 100.604274},
zoom: 18,
}
var maps = new google.maps.Map(document.getElementById("map"),mapOptions);
var marker = new google.maps.Marker({
position: new google.maps.LatLng(13.847616, 100.604736),
map: maps,
title: 'ถนน ลาดปลาเค้า',
icon: 'images/camping-icon.png',
});
}
</script>
ตรวจสอบ Path ของรูปให้ถูกต้อง
icon: 'images/camping-icon.png',
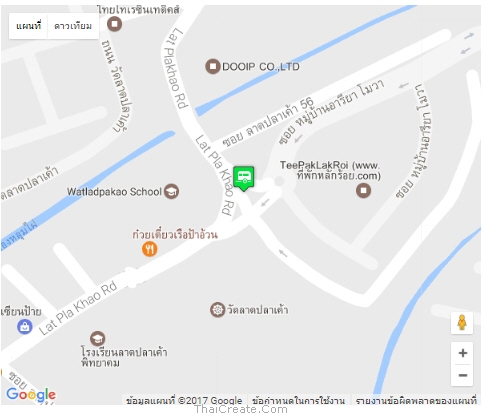
Ex 3 : การปักหมุด Marker มากกว่าหนึ่งจุด หรือ สองจุดขึ้นไป
<script>
function initMap() {
var mapOptions = {
center: {lat: 13.847860, lng: 100.604274},
zoom: 18,
}
var maps = new google.maps.Map(document.getElementById("map"),mapOptions);
var marker1 = new google.maps.Marker({
position: new google.maps.LatLng(13.847616, 100.604736),
map: maps,
title: 'ถนน ลาดปลาเค้า',
icon: 'images/camping-icon.png',
});
var marker2 = new google.maps.Marker({
position: new google.maps.LatLng(13.847077, 100.606973),
map: maps,
title: 'หมู่บ้านอารียา',
icon: 'images/camping-icon.png',
});
}
</script>
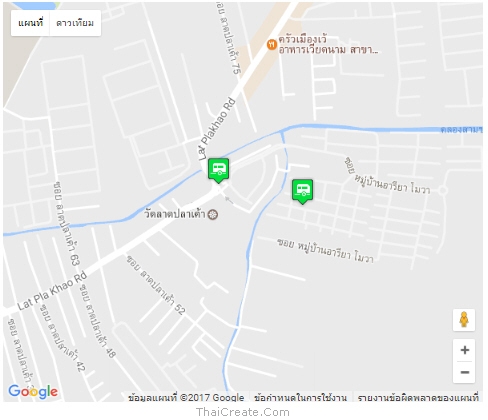
Ex 4 : การปักหมุด Marker และเพิ่ม Event Click ที่หมุด แล้วจะแสดง Window Info ของจุดนั้นๆ
<script>
function initMap() {
var mapOptions = {
center: {lat: 13.847860, lng: 100.604274},
zoom: 18,
}
var maps = new google.maps.Map(document.getElementById("map"),mapOptions);
var marker = new google.maps.Marker({
position: new google.maps.LatLng(13.847616, 100.604736),
map: maps,
title: 'ถนน ลาดปลาเค้า',
icon: 'images/camping-icon.png',
});
var info = new google.maps.InfoWindow({
content : '<div style="font-size: 25px;color: red">ThaiCreate.Com Camping</div>'
});
google.maps.event.addListener(marker, 'click', function() {
info.open(maps, marker);
});
}
</script>
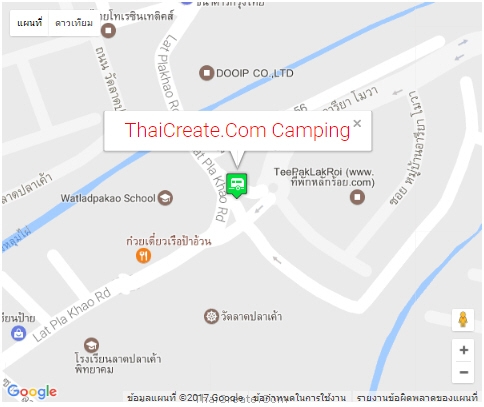
แสดง InfoWindow ของ Marker นั้นๆ เมื่อคลิกที่ หมุด
Code ทั้งหมด
<!DOCTYPE html>
<html>
<head>
<title>Simple Map</title>
<meta name="viewport" content="initial-scale=1.0">
<meta charset="utf-8">
<style>
/* Always set the map height explicitly to define the size of the div
* element that contains the map. */
#map {
height: 100%;
}
/* Optional: Makes the sample page fill the window. */
html {
height: 100%;
margin: 0;
padding: 0;
text-align: center;
}
#map {
height: 500px;
width: 600px;
}
</style>
</head>
<body>
<div id="map"></div>
<script>
function initMap() {
var mapOptions = {
center: {lat: 13.847860, lng: 100.604274},
zoom: 18,
}
var maps = new google.maps.Map(document.getElementById("map"),mapOptions);
var marker = new google.maps.Marker({
position: new google.maps.LatLng(13.847616, 100.604736),
map: maps,
title: 'ถนน ลาดปลาเค้า',
icon: 'images/camping-icon.png',
});
var info = new google.maps.InfoWindow({
content : '<div style="font-size: 25px;color: red">ThaiCreate.Com Camping</div>'
});
google.maps.event.addListener(marker, 'click', function() {
info.open(maps, marker);
});
}
</script>
<script src="https://maps.googleapis.com/maps/api/js?key=AIzaSyAK3RgqSLy1toc4lkh2JVFQ5ipuRB106vU&callback=initMap" async defer></script>
</body>
</html>
|