ตอนที่ 10 : Google Maps API การปักหมุด (Marker) บนแผนที่ จาก PHP/MySQL (Json) |
Google Maps API การปักหมุด (Marker) บนแผนที่ จาก PHP/MySQL (Json) บทความนี้จะเป็นตัวอย่างการเขียน Google Map API และการปักหมุด (Marker) บนแผนที่ของกูเกิ้ล โดยจะใช้การดึงค่าสถานที่ ที่เป็น Latitude และ Longitude มาจากฐานข้อมูล MySQL ด้วยการใช้ PHP อ่านค่าให้อยู่ในรูปแบบของ Json จากนั้นใช้ jQuery กับ Ajax เพื่ออ่านค่ามา Loop และทำการปักหมุดลงในแผนที่
Google Maps API Marker and PHP/MySQL (Json)
jQuery Library
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
Step 1 : เริ่มต้นให้สร้าง Table สำหรับการจัดเก็บ Location
location
CREATE TABLE `location` (
`LOC_ID` int(10) NOT NULL auto_increment,
`LOC_NAME` varchar(250) NOT NULL,
`LAT` varchar(50) NOT NULL,
`LNG` varchar(50) NOT NULL,
PRIMARY KEY (`LOC_ID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=5 ;
--
-- dump ตาราง `location`
--
INSERT INTO `location` VALUES (1, 'วัดลาดปลาเค้า', '13.846876', '100.604481');
INSERT INTO `location` VALUES (2, 'หมู่บ้านอารียา', '13.847766', '100.605768');
INSERT INTO `location` VALUES (3, 'สปีดเวย์', '13.845235', '100.602711');
INSERT INTO `location` VALUES (4, 'สเต็ก ลุงหนวด', '13.862970', '100.613834');
นำ Query นี้ไปรันเพื่อสร้าง Table
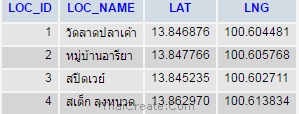
Table ที่ได้
Step 2 : สร้างไฟล์สำหรับอ่านข้อมูลให้อยู่ในรูปแบบของ Json
json.php
<?php
header('Content-Type: application/json');
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
mysql_query("SET NAMES UTF8");
$strSQL = "SELECT * FROM location ";
$objQuery = mysql_query($strSQL);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
array_push($resultArray,$obResult);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
Step 3 : อ่านค่า Json แล้ว Loop ค่าเพื่อปักหมุดลงใน Map
$.getJSON( "json.php", function( jsonObj ) {
$.each(jsonObj, function(i, item){
// item.LAT
// item.LNG
// item.LOC_NAME
}); // loop
});
จะได้เป็น
$.getJSON( "json.php", function( jsonObj ) {
//*** loop
$.each(jsonObj, function(i, item){
marker = new google.maps.Marker({
position: new google.maps.LatLng(item.LAT, item.LNG),
map: maps,
title: item.LOC_NAME
});
info = new google.maps.InfoWindow();
google.maps.event.addListener(marker, 'click', (function(marker, i) {
return function() {
info.setContent(item.LOC_NAME);
info.open(maps, marker);
}
})(marker, i));
}); // loop
});
ผลลัพธ์ที่ได้

Code ทั้งหมด
<!DOCTYPE html>
<html>
<head>
<title>Simple Map</title>
<meta name="viewport" content="initial-scale=1.0">
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<meta charset="utf-8">
<style>
/* Always set the map height explicitly to define the size of the div
* element that contains the map. */
#map {
height: 100%;
}
/* Optional: Makes the sample page fill the window. */
html {
height: 100%;
margin: 0;
padding: 0;
text-align: center;
}
#map {
height: 500px;
width: 600px;
}
</style>
</head>
<body>
<div id="map"></div>
<script>
function initMap() {
var mapOptions = {
center: {lat: 13.847860, lng: 100.604274},
zoom: 14,
}
var maps = new google.maps.Map(document.getElementById("map"),mapOptions);
var marker, info;
$.getJSON( "json.php", function( jsonObj ) {
//*** loop
$.each(jsonObj, function(i, item){
marker = new google.maps.Marker({
position: new google.maps.LatLng(item.LAT, item.LNG),
map: maps,
title: item.LOC_NAME
});
info = new google.maps.InfoWindow();
google.maps.event.addListener(marker, 'click', (function(marker, i) {
return function() {
info.setContent(item.LOC_NAME);
info.open(maps, marker);
}
})(marker, i));
}); // loop
});
}
</script>
<script src="https://maps.googleapis.com/maps/api/js?key=AIzaSyAK3RgqSLy1toc4lkh2JVFQ5ipuRB106vU&callback=initMap" async defer></script>
</body>
</html>

|