ตอนที่ 19 : PHP ฟังก์ชั่น oci และการใช้ oci เรียกใช้ Oracle Stored Procedure (IN, OUT) |
ตอนที่ 19 : PHP ฟังก์ชั่น oci และการใช้ oci เรียกใช้ Oracle Stored Procedure (IN, OUT) ในหัวข้อนี้เราจะมาเรียนรู้วิธีการเรียกใช้งาน Stored Procedure ของ Oracle Database ด้วย PHP ซึ่งในการเรียกใช้งาน Stored Procedure จะต้องใช้ function ของ oci หรือในฟังก์ชั่นรุ่นใหม่ ๆ เช่น PDO ส่วนการเรียกนั้น เราสามารถใช้คำสั่ง BEGIN procedure_name(); END; ได้ทันที ถ้าหากมีค่า Parameters ก็จะต้องทำการ Pass ค่าไปกับ Query ด้วย หลังจากนั้นก็สามารถใช้คำสั่ง oci_execute() ข้อมูลได้เลย พร้อมกับอ่านค่า Result ที่ Stored Procedure นั้นได้ส่งกลับมาให้ในรูปอบบของ Parameters ที่เป็น OUT
Table : CUSTOMER
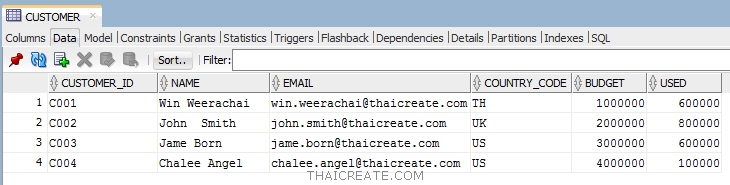
Call Oracle Stored Procedure
BEGIN procedure_name(agr1,agr2,...) END;
ในการเปิดใช้งาน oci จะต้องเปิดในส่วนของ extension บน php.ini
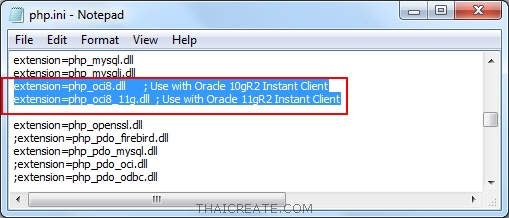
php.ini
extension=php_oci8.dll ; Use with Oracle 10gR2 Instant Client
extension=php_oci8_11g.dll ; Use with Oracle 11gR2 Instant Client
PHP Oracle Config OCI/OCI8 (XAMPP Web Server)
Error Case : ORA-12154: TNS:could not resolve the connect identifier specified
Example 1 : การใช้ PHP เรียก Stored Procedure แบบ Query ข้อมูลออกมา
Stored Procedure Name : GET_CUSTOMER()
CREATE OR REPLACE PROCEDURE GET_CUSTOMER
(pCountryCode IN VARCHAR2, pRowFound OUT NUMBER, pCustomer OUT SYS_REFCURSOR)
AS
BEGIN
-- OUT pCustomer
OPEN pCustomer FOR
SELECT *
FROM CUSTOMER WHERE COUNTRY_CODE = pCountryCode;
-- OUT pRowFound
SELECT COUNT(*) INTO pRowFound
FROM CUSTOMER WHERE COUNTRY_CODE = pCountryCode;
END;
Call
VAR pRowFound NUMBER;
VAR pCustomer REFCURSOR;
EXEC GET_CUSTOMER('US',:pRowFound,:pCustomer)
PRINT pRowFound;
PRINT pCustomer;
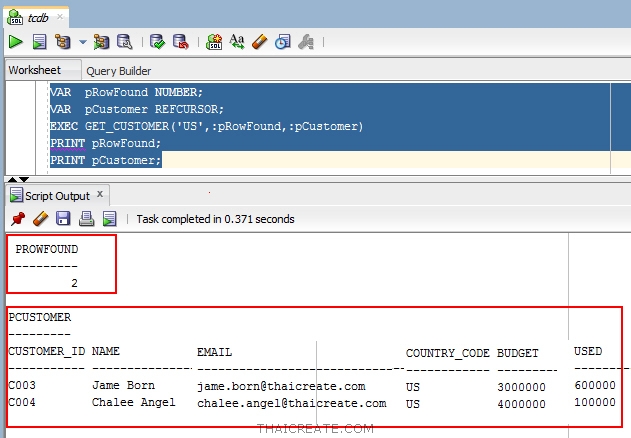
Code : การเรียกผ่าน BEGIN GET_CUSTOMER(:pCountryCode, :pRowFound, :pCustomer); END;
<html>
<head>
<title>ThaiCreate.Com Call Oracle Stored Procedure</title>
</head>
<body>
<?php
// Connect
$objConnect = oci_connect("myuser","mypassword","TCDB");
// Statement
$strSQL = "BEGIN GET_CUSTOMER(:pCountryCode, :pRowFound, :pCustomer); END;";
$objParse = oci_parse($objConnect, $strSQL);
// Declare cursor for OUT SYS_REFCURSOR
$objCursor = oci_new_cursor($objConnect);
// Variable
$strCountryCode = "US";
$strRowFound = 0;
// Bind parameters
oci_bind_by_name($objParse, ':pCountryCode', $strCountryCode,2) ;
oci_bind_by_name($objParse, ':pRowFound', $strRowFound) ;
oci_bind_by_name($objParse, ':pCustomer', $objCursor, -1, OCI_B_CURSOR);
// Execute
oci_execute ($objParse);
oci_execute($objCursor);
// Display OUT : pRowFound
echo "Rows Count : ".$strRowFound;
?>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<?php
// Loop display OUT : pCustomer SYS_REFCURSOR
while (OCIFetchInto($objCursor,$objResult,OCI_ASSOC))
{
?>
<tr>
<td><div align="center"><?php echo $objResult["CUSTOMER_ID"];?></div></td>
<td><?php echo $objResult["NAME"];?></td>
<td><?php echo $objResult["EMAIL"];?></td>
<td><div align="center"><?php echo $objResult["COUNTRY_CODE"];?></div></td>
<td align="right"><?php echo $objResult["BUDGET"];?></td>
<td align="right"><?php echo $objResult["USED"];?></td>
</tr>
<?php
}
?>
</table>
<?php
oci_close($objConnect);
?>
</body>
</html>
Result
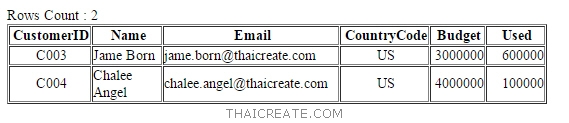
Example 2 : การใช้ PHP เรียก Stored Procedure แบบ Query เพื่อ Insert ข้อมูล
Stored Procedure Name : INSERT_CUSTOMER()
CREATE OR REPLACE PROCEDURE INSERT_CUSTOMER
(
pCustomerID IN VARCHAR2,
pName IN VARCHAR2,
pEmail IN VARCHAR2,
pCountryCode IN VARCHAR2,
pBudget IN DECIMAL,
pUsed IN DECIMAL,
pResult OUT NUMBER,
pMessage OUT VARCHAR2
)
AS
BEGIN
-- Insert Statement 1
INSERT INTO CUSTOMER (CUSTOMER_ID, NAME, EMAIL, COUNTRY_CODE, BUDGET, USED)
VALUES (pCustomerID, pName, pEmail, pCountryCode, pBudget, pUsed);
-- Return row effected
pResult := SQL%ROWCOUNT;
pMessage := 'Insert Data Successfully';
-- Return error exception
EXCEPTION
WHEN OTHERS THEN
BEGIN
pResult := 0;
pMessage := 'Error - ' || SQLERRM;
END;
END;
Call
VAR pResult NUMBER;
VAR pMessage VARCHAR2;
EXEC INSERT_CUSTOMER('C005','Fun Wipa','[email protected]','TH','100000','0',:pResult,:pMessage);
PRINT pResult;
PRINT pMessage;
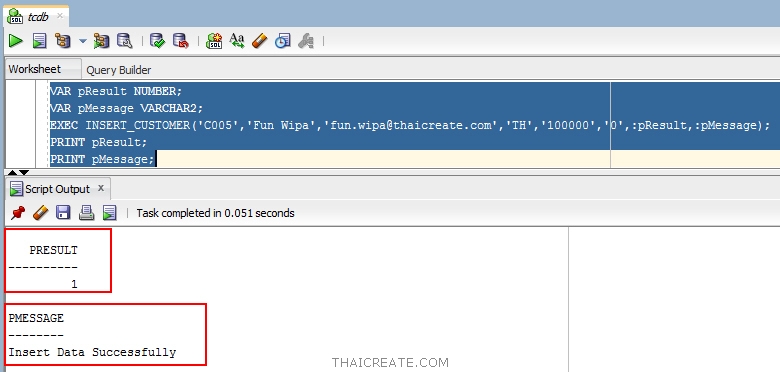
add.php
<html>
<head>
<title>ThaiCreate.Com Call Oracle Stored Procedure</title>
</head>
<body>
<form action="save.php" name="frmAdd" method="post">
<table width="284" border="1">
<tr>
<th width="120">CustomerID</th>
<td width="238"><input type="text" name="txtCustomerID" size="5"></td>
</tr>
<tr>
<th width="120">Name</th>
<td><input type="text" name="txtName" size="20"></td>
</tr>
<tr>
<th width="120">Email</th>
<td><input type="text" name="txtEmail" size="20"></td>
</tr>
<tr>
<th width="120">CountryCode</th>
<td><input type="text" name="txtCountryCode" size="2"></td>
</tr>
<tr>
<th width="120">Budget</th>
<td><input type="text" name="txtBudget" size="5"></td>
</tr>
<tr>
<th width="120">Used</th>
<td><input type="text" name="txtUsed" size="5"></td>
</tr>
</table>
<input type="submit" name="submit" value="submit">
</form>
</body>
</html>
save.php
<html>
<head>
<title>ThaiCreate.Com Call Oracle Stored Procedure</title>
</head>
<body>
<?php
// Connect
$objConnect = oci_connect("myuser","mypassword","TCDB");
// Get parameters input
$strCustomerID = $_POST["txtCustomerID"];
$strName = $_POST["txtName"];
$strEmail = $_POST["txtEmail"];
$strCountryCode = $_POST["txtCountryCode"];
$strBudget = $_POST["txtBudget"];
$strUsed = $_POST["txtUsed"];
// Result OUT = pResult,pMessage
$strSQL = "BEGIN INSERT_CUSTOMER('$strCustomerID','$strName','$strEmail','$strCountryCode','$strBudget','$strUsed', :pResult, :pMessage); END;";
// Parse Statement
$objParse = oci_parse($objConnect, $strSQL);
// Result out
$strResult = 0;
$strMessage = "";
// Bind OUT parameters
oci_bind_by_name($objParse, ':pResult', $strResult) ;
oci_bind_by_name($objParse, ':pMessage', $strMessage,500);
// Execute
$result = oci_execute ($objParse);
// Display OUT result
if($strResult == 1)
{
echo $strMessage;
}
else
{
echo $strMessage;
}
?>
</body>
</html>
Result
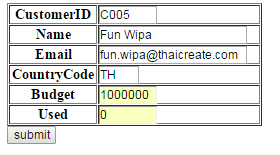
Insert ข้อมูลและ Save

กรณีที่ Insert ข้อมูลสำเร็จ

กรณีที่ Insert ข้อมูลไม่สำเร็จ
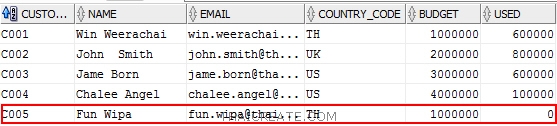
เขียนได้อีกรูปแบบหนึ่งคือ
<html>
<head>
<title>ThaiCreate.Com Call Oracle Stored Procedure</title>
</head>
<body>
<?php
// Connect
$objConnect = oci_connect("myuser","mypassword","TCDB");
// Get parameters input
$strCustomerID = $_POST["txtCustomerID"];
$strName = $_POST["txtName"];
$strEmail = $_POST["txtEmail"];
$strCountryCode = $_POST["txtCountryCode"];
$strBudget = $_POST["txtBudget"];
$strUsed = $_POST["txtUsed"];
// Statement
$strSQL = "BEGIN INSERT_CUSTOMER(:pCustomerID, :pName, :pEmail, :pCountryCode, :pBudget, :pUsed, :pResult, :pMessage); END;";
// Parse Statement
$objParse = oci_parse($objConnect, $strSQL);
// Result OUT
$strResult = 0;
$strMessage = "";
// Bind IN parameters
oci_bind_by_name($objParse, ':pCustomerID', $strCustomerID,4);
oci_bind_by_name($objParse, ':pName', $strName,100);
oci_bind_by_name($objParse, ':pEmail', $strEmail,150);
oci_bind_by_name($objParse, ':pCountryCode', $strCountryCode,2);
oci_bind_by_name($objParse, ':pBudget', $strBudget);
oci_bind_by_name($objParse, ':pUsed', $strUsed);
// Bind OUT parameters
oci_bind_by_name($objParse, ':pResult', $strResult) ;
oci_bind_by_name($objParse, ':pMessage', $strMessage,500);
// Execute
$result = oci_execute ($objParse);
// Display OUT result
if($strResult == 1)
{
echo $strMessage;
}
else
{
echo $strMessage;
}
?>
</body>
</html>
|