ตอนที่ 17 : PHP เรียกใช้ EXEC/CALL - SQL Server Stored Procedure ด้วย sqlsrv และ pdo |
ตอนที่ 17 : PHP เรียกใช้ EXEC/CALL - SQL Server Stored Procedure ด้วย sqlsrv และ pdo ในหัวข้อนี้เราจะมาเรียนรู้วิธีการเรียกใช้งาน Stored Procedure ของ SQL Server Database ด้วย PHP ซึ่งในการเรียกใช้งาน Stored Procedure จะต้องใช้ function ของ sqlsrv หรือในฟังก์ชั่นรุ่นใหม่ ๆ เช่น PDO ส่วนการเรียกนั้น เราสามารถใช้คำสั่ง EXEC procedure_name() ได้ทันที ถ้าหากมีค่า Parameters ก็จะต้องทำการ Pass ค่าไปกับ Query ด้วย หลังจากนั้นก็สามารถใช้คำสั่ง sqlsrv_query() ข้อมูลได้เลย พร้อมกับอ่านค่า Result ที่ Stored Procedure นั้นได้ส่งกลับมาให้
EXEC SQL Server Stored Procedure
{call procedure_name(agr1,agr2,...)}
โครงสร้างของตาราง CUSTOMER
Table : CUSTOMER

Example 1 : การใช้ PHP เรียก Stored Procedure แบบ Query ข้อมูลออกมา (sqlsrv)
Stored Procedure Name : getCustomer()
CREATE PROCEDURE [dbo].[getCustomer]
@pCountryCode VARCHAR(2)
AS
BEGIN
-- set customer
SELECT * FROM CUSTOMER WHERE COUNTRY_CODE = @pCountryCode
END
Code (PHP)
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server Stored Procedure (sqlsrv)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "sa";
$userPassword = "";
$dbName = "mydatabase";
$connectionInfo = array("Database"=>$dbName, "UID"=>$userName, "PWD"=>$userPassword, "MultipleActiveResultSets"=>true);
$conn = sqlsrv_connect( $serverName, $connectionInfo);
if( $conn === false ) {
die( print_r( sqlsrv_errors(), true));
}
$strCountryCode = "US";
$sql = "{call getCustomer(?)}";
$params = array(
array($strCountryCode, SQLSRV_PARAM_IN)
);
$query = sqlsrv_query($conn, $sql, $params);
?>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<?php
while($result = sqlsrv_fetch_array($query, SQLSRV_FETCH_ASSOC))
{
?>
<tr>
<td><div align="center"><?php echo $result["CUSTOMER_ID"];?></div></td>
<td><?php echo $result["NAME"];?></td>
<td><?php echo $result["EMAIL"];?></td>
<td><div align="center"><?php echo $result["COUNTRY_CODE"];?></div></td>
<td align="right"><?php echo $result["BUDGET"];?></td>
<td align="right"><?php echo $result["USED"];?></td>
</tr>
<?php
}
?>
</table>
<?php
sqlsrv_close($conn);
?>
</body>
</html>
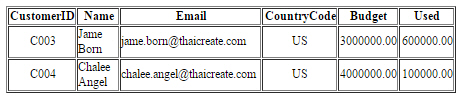

Example 2 : การใช้ PHP เรียก Stored Procedure แบบ Query เพื่อ Insert ข้อมูล
Stored Procedure Name : insertCustomer()
CREATE PROCEDURE [dbo].[insertCustomer]
@pCustomerID VARCHAR(4),
@pName VARCHAR(50),
@pEmail VARCHAR(50),
@pCountryCode VARCHAR(2),
@pBudget DECIMAL(18,2),
@pUsed DECIMAL(18,2)
AS
BEGIN
-- insert customer
INSERT INTO CUSTOMER VALUES (@pCustomerID,@pName,@pEmail,@pCountryCode,@pBudget,@pUsed);
END
add.php
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server Stored Procedure (sqlsrv)</title>
</head>
<body>
<form action="save.php" name="frmAdd" method="post">
<table width="284" border="1">
<tr>
<th width="120">CustomerID</th>
<td width="238"><input type="text" name="txtCustomerID" size="5"></td>
</tr>
<tr>
<th width="120">Name</th>
<td><input type="text" name="txtName" size="20"></td>
</tr>
<tr>
<th width="120">Email</th>
<td><input type="text" name="txtEmail" size="20"></td>
</tr>
<tr>
<th width="120">CountryCode</th>
<td><input type="text" name="txtCountryCode" size="2"></td>
</tr>
<tr>
<th width="120">Budget</th>
<td><input type="text" name="txtBudget" size="5"></td>
</tr>
<tr>
<th width="120">Used</th>
<td><input type="text" name="txtUsed" size="5"></td>
</tr>
</table>
<input type="submit" name="submit" value="submit">
</form>
</body>
</html>
save.php
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server Stored Procedure (sqlsrv)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "sa";
$userPassword = '';
$dbName = "mydatabase";
$connectionInfo = array("Database"=>$dbName, "UID"=>$userName, "PWD"=>$userPassword, "MultipleActiveResultSets"=>true);
$conn = sqlsrv_connect( $serverName, $connectionInfo);
if( $conn === false ) {
die( print_r( sqlsrv_errors(), true));
}
$strCustomerID = $_POST["txtCustomerID"];
$strName = $_POST["txtName"];
$strEmail = $_POST["txtEmail"];
$strCountryCode = $_POST["txtCountryCode"];
$strBudget = $_POST["txtBudget"];
$strUsed = $_POST["txtUsed"];
$sql = "{call insertCustomer(?, ?, ?, ?, ?, ?)}";
$params = array(
array($strCustomerID, SQLSRV_PARAM_IN),
array($strName, SQLSRV_PARAM_IN),
array($strEmail, SQLSRV_PARAM_IN),
array($strCountryCode, SQLSRV_PARAM_IN),
array($strBudget, SQLSRV_PARAM_IN),
array($strUsed, SQLSRV_PARAM_IN)
);
$query = sqlsrv_query( $conn, $sql, $params);
if($query === false ) {
die( print_r( sqlsrv_errors(), true));
}
else
{
echo "Record add successfully";
}
sqlsrv_close($conn);
?>
</body>
</html>
ส่วนวิธีการ UPDATE และ DELETE ก็ใช้หลักการเดียวกับการ INSERT ข้อมูล
สำหรับตัวอย่างที่ 3 และ 4 จะเป็นการอ่านจาก OUTPUT หรือ OUT
ตอนที่ 10 : การใช้ OUTPUT และ OUT เพื่อส่งค่ากลับ (SQL Server : Stored Procedure)
Example 3 : การใช้ PHP เรียก Stored Procedure และอ่านค่า OUTPUT พร้อมกับ SELECT ข้อมูล
Stored Procedure Name : getCustomer()
CREATE PROCEDURE [dbo].[getCustomer]
@pCountryCode VARCHAR(2),
@pRowFound INT OUTPUT
AS
BEGIN
-- set rowcount
SELECT @pRowFound = COUNT(*) FROM CUSTOMER WHERE COUNTRY_CODE = @pCountryCode
-- set customer
SELECT * FROM CUSTOMER WHERE COUNTRY_CODE = @pCountryCode
END
Code (php)
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server Stored Procedure (sqlsrv)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "sa";
$userPassword = "";
$dbName = "mydatabase";
$connectionInfo = array("Database"=>$dbName, "UID"=>$userName, "PWD"=>$userPassword, "MultipleActiveResultSets"=>true);
$conn = sqlsrv_connect( $serverName, $connectionInfo);
if( $conn === false ) {
die( print_r( sqlsrv_errors(), true));
}
$strCountryCode = "US";
$strRowFound = 0;
$sql = " EXEC getCustomer @pCountryCode=?, @pRowFound=?";
$params = array(
array($strCountryCode, SQLSRV_PARAM_IN),
array($strRowFound, SQLSRV_PARAM_OUT)
);
$query = sqlsrv_query($conn, $sql, $params);
echo "pRowFound = ". $strRowFound;
?>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<?php
while($result = sqlsrv_fetch_array($query, SQLSRV_FETCH_ASSOC))
{
?>
<tr>
<td><div align="center"><?php echo $result["CUSTOMER_ID"];?></div></td>
<td><?php echo $result["NAME"];?></td>
<td><?php echo $result["EMAIL"];?></td>
<td><div align="center"><?php echo $result["COUNTRY_CODE"];?></div></td>
<td align="right"><?php echo $result["BUDGET"];?></td>
<td align="right"><?php echo $result["USED"];?></td>
</tr>
<?php
}
?>
</table>
<?php
sqlsrv_close($conn);
?>
</body>
</html>
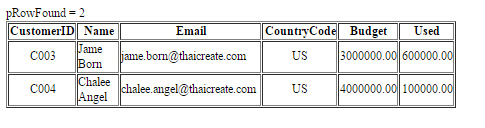
ค่าที่ถูกส่งมาทั้ง SELECT และ Parameters ที่เป็นแบบ OUT
Example 4 : การใช้ .NET เรียก Stored Procedure และอ่านค่า OUTPUT ที่มีตั้งแต่ 2 ค่าขึ้นไป
Stored Procedure Name : insertCustomer()
CREATE PROCEDURE [dbo].[insertCustomer]
@pCustomerID VARCHAR(4),
@pName VARCHAR(50),
@pEmail VARCHAR(50),
@pCountryCode VARCHAR(2),
@pBudget DECIMAL(18,2),
@pUsed DECIMAL(18,2),
@pResult INT OUTPUT,
@pMessage VARCHAR(500) OUTPUT
AS
BEGIN
BEGIN TRY
-- insert customer
INSERT INTO CUSTOMER VALUES (@pCustomerID,@pName,@pEmail,@pCountryCode,@pBudget,@pUsed);
SET @pResult = @@ROWCOUNT;
SET @pMessage = 'Insert Data Successfully';
END TRY
BEGIN CATCH
SET @pResult = 0;
SELECT @pMessage = ERROR_MESSAGE();
END CATCH
END
Code (PHP)
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server Stored Procedure (sqlsrv)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "sa";
$userPassword = '';
$dbName = "mydatabase";
$connectionInfo = array("Database"=>$dbName, "UID"=>$userName, "PWD"=>$userPassword, "MultipleActiveResultSets"=>true);
$conn = sqlsrv_connect( $serverName, $connectionInfo);
if( $conn === false ) {
die( print_r( sqlsrv_errors(), true));
}
$strCustomerID = "C005";
$strName = "Fun Wipa";
$strEmail = "[email protected]";
$strCountryCode = "TH";
$strBudget = 1000000.00;
$strUsed = 0.00;
$strResult = 0;
$strMessage = "";
$sql = "{call insertCustomer(?, ?, ?, ?, ?, ?, ?, ?)}";
$params = array(
array($strCustomerID, SQLSRV_PARAM_IN),
array($strName, SQLSRV_PARAM_IN),
array($strEmail, SQLSRV_PARAM_IN),
array($strCountryCode, SQLSRV_PARAM_IN),
array($strBudget, SQLSRV_PARAM_IN),
array($strUsed, SQLSRV_PARAM_IN),
array($strResult, SQLSRV_PARAM_OUT),
array($strMessage, SQLSRV_PARAM_OUT)
);
$query = sqlsrv_query( $conn, $sql, $params);
if($query === false ) {
die( print_r( sqlsrv_errors(), true));
}
else
{
echo "pResult = ".$strResult;
echo "<br>";
echo "pMessage = ".$strMessage;
}
sqlsrv_close($conn);
?>
</body>
</html>

กรณีที่ Insert ข้อมูลสำเร็จ

กรณีที่ Insert ข้อมูลไม่สำเร็จ
Code สำหรับ PDO กับ SQL Server Stored Procedure
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server Stored Procedure (PDO)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "sa";
$userPassword = "";
$dbName = "mydatabase";
$conn = new PDO("sqlsrv:server=$serverName ; Database = $dbName", $userName, $userPassword);
$strCountryCode = "US";
$sql = "EXEC getCustomer ?";
$stmt = $conn->prepare($sql);
$stmt->bindParam(1,$strCountryCode,PDO::PARAM_STR);
$stmt->execute();
?>
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
</tr>
<?php
while($result = $stmt->fetch( PDO::FETCH_ASSOC ))
{
?>
<tr>
<td><div align="center"><?php echo $result["CUSTOMER_ID"];?></div></td>
<td><?php echo $result["NAME"];?></td>
<td><?php echo $result["EMAIL"];?></td>
<td><div align="center"><?php echo $result["COUNTRY_CODE"];?></div></td>
<td align="right"><?php echo $result["BUDGET"];?></td>
<td align="right"><?php echo $result["USED"];?></td>
</tr>
<?php
}
?>
</table>
<?php
$conn = null;
?>
</body>
</html>
<html>
<head>
<title>ThaiCreate.Com PHP & SQL Server Stored Procedure (PDO)</title>
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "sa";
$userPassword = "";
$dbName = "mydatabase";
$conn = new PDO("sqlsrv:server=$serverName ; Database = $dbName", $userName, $userPassword);
$strCustomerID = $_POST["txtCustomerID"];
$strName = $_POST["txtName"];
$strEmail = $_POST["txtEmail"];
$strCountryCode = $_POST["txtCountryCode"];
$strBudget = $_POST["txtBudget"];
$strUsed = $_POST["txtUsed"];
$stmt = $conn->prepare(" EXEC insertCustomer ?, ?, ?, ?, ?, ? ");
$stmt->bindParam(1,$strCustomerID,PDO::PARAM_STR);
$stmt->bindParam(2,$strName,PDO::PARAM_STR);
$stmt->bindParam(3,$strEmail,PDO::PARAM_STR);
$stmt->bindParam(4,$strCountryCode,PDO::PARAM_STR);
$stmt->bindParam(5,$strBudget,PDO::PARAM_INT);
$stmt->bindParam(6,$strUsed,PDO::PARAM_INT);
$stmt->execute();
$conn = null;
?>
</body>
</html>
|