ตอนที่ 1 : รู้จัก Windows Service การสร้าง Application ให้รันบน Windows (VB.Net,C#) |
ตอนที่ 1 : รู้จัก Windows Service การสร้าง Application ให้รันบน Windows (VB.Net,C#) โปรแกรมประเภท Windows Service ช่วยให้เราสามารถสร้างแอ็พพลิเคชันที่สามารถเรียกใช้งานและทำงานได้นานและพร้อมที่จะทำงานได้ตลอดเวลา ซึ่งมันจะทำงานในระบบ Session ของ Windows โดยโปรเซสการทำงานของโปรแกรม สามารถเริ่มต้นโดยอัตโนมัติเมื่อเริ่มระบบคอมพิวเตอร์ หรือเปิดเครื่องคอมพิวเตอร์ เราสามารถทำการควบคุมโปรแกรมผ่าน Services Control Manager ด้วยการ paused (หยุดชั่วคราว) หรือ restarted (รีสตาร์ท) มันได้ โดยโปรแกรมประเภท Windows Service จะเหมาะกับ Process ที่ไม่ต้องการแสดงผล User Interface หรือทำงานในลักษณะ Background Process คุณลักษณะเหล่านี้ทำให้บริการเหมาะสำหรับการใช้งานบนเซิร์ฟเวอร์หรือเมื่อใดก็ตามที่คุณต้องการใช้งานที่ยาวนานซึ่งไม่รบกวนผู้ใช้คนอื่น ๆ ที่ทำงานบนคอมพิวเตอร์เครื่องเดียวกัน
ตัวอย่าง Windows Service ที่ทำงานอยู่ที่ใน Services Control Manager
การเขียนโปรแกรมประเภท Windows Service ถือว่าเป็นการเขียนโปรแกรมอีกขั้นหนึ่งที่โปรแกรมเมอร์จะพัฒนาฝีมือให้เป็นการเขียนแบบ Advanced เพราะมีน้อยคนนักที่จะเขียนโปรแกรมแบบ Windows Service และกระบวนการทำงานของมันได้เจาะลึกไปอีกขั้นของการเขียนโปรแกรมเพื่อทำงานบน Windows และมันจะเกิดไอเดียอีกมากมายเมื่อเราได้ศึกษาการเขียนในรูปแบบนี้
เริ่มต้นด้วยการสร้าง Windows Service
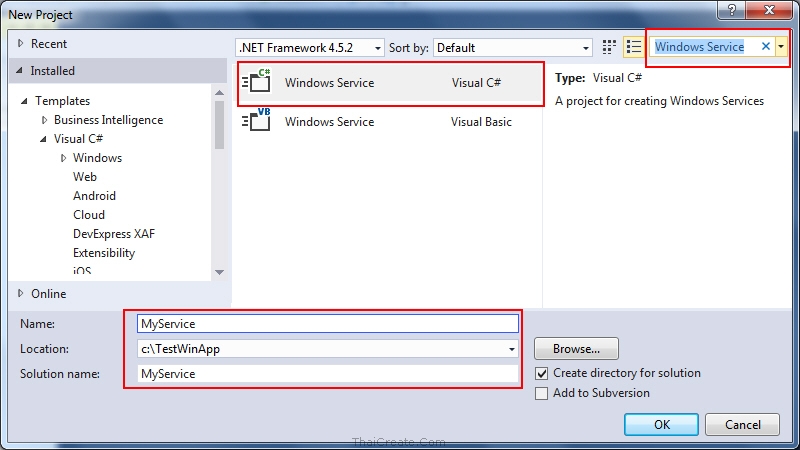
ใน Visual Studio 2015 หรือ 2017 ในส่วนของ Template Project อาจจะต้องใช้การค้นหา "Windows Service"
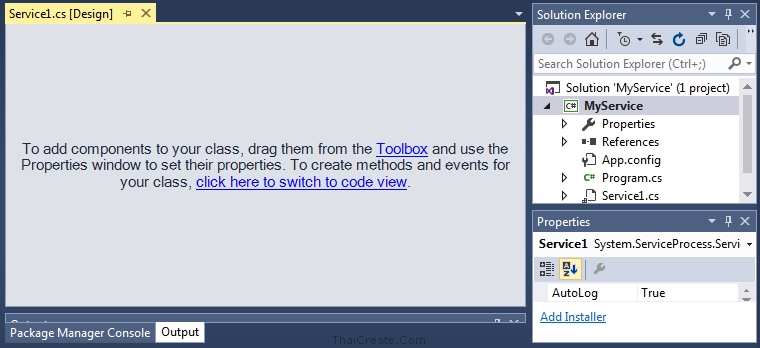
โปรเจคที่ได้
จากนั้นเราจะได้ไฟล์ที่สำคัญๆ อยู่ 2 ไฟล์คือ
Program.cs (เป็นไฟล์สำหรับการ Startup)
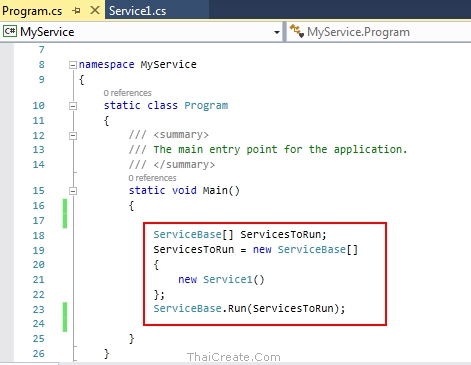
ตัวอย่างไฟล์ Program.cs ซึ่งเป็นไฟล์ Startup แบะจะเรียกเปิด Service1 ขึ้นมาทำงาน
Service1.cs เป็นไฟล์สำหรับการทำงานของ Service
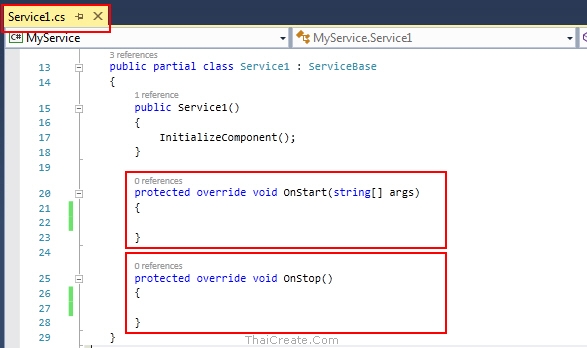
ซึ่งจะประกอบด้วย OnStart (เมื่อ Service Start) และ OnStop (เมื่อ Service Stop)
ทดสอบการ Run โปรแกรม Windows Service
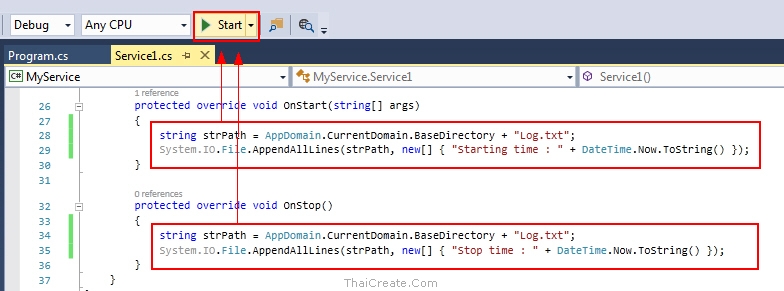
เมื่อกดปุ่มที่ Run ในโหมดของการ Debug
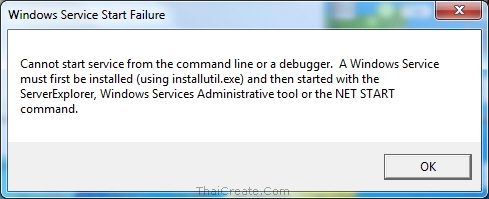
จะแสดง Error
Cannot start service from the command line or debugger. A winwows Service must first be installed(using installutil.exe) and then started with the ServerExplorer, Windows Services Afministrative tool or the NET START command.
สาเหตุเพราะโปรแกรมประเภท Windows Service ไม่สามารถทำการรันด้วยการเปิดตามปกติ เพราะมันจะต้องทำงานภายใต้ Services Control Manager ด้วยการ Start, Stop เท่านั้น
แต่เราสามารถทำเป็นโหมดในการ Debug ไว้สำหรับการ Develop ได้ โดยเพิ่ม
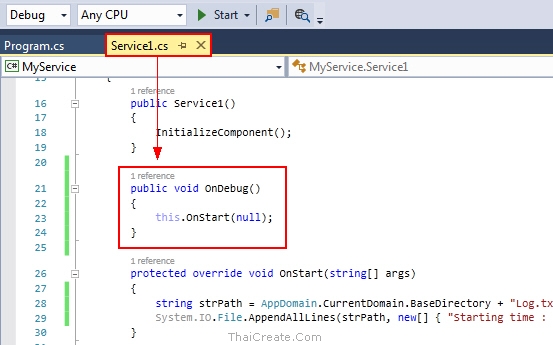
Service1.cs
public void OnDebug()
{
this.OnStart(null);
}
เป็นการสร้าง Method ไว้สำหรับ Debug และเรียก OnStart
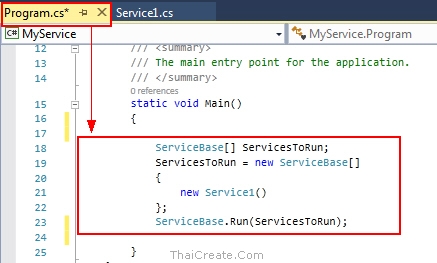
และเพิ่มโหมดสำหรับเงื่อนไขการ Debug
Program.cs
#if DEBUG
#else
#endif
หมายถึงว่าจะให้ทำงานในส่วน block ของ DEBUG หรือแบบไม่ใช่การ Debug
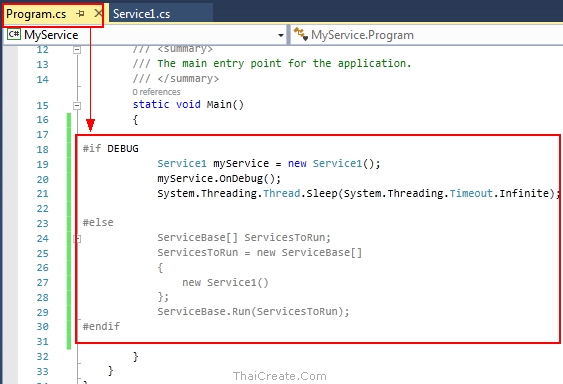
จะได้ใหม่เป็น
#if DEBUG
Service1 myService = new Service1();
myService.OnDebug();
System.Threading.Thread.Sleep(System.Threading.Timeout.Infinite);
#else
ServiceBase[] ServicesToRun;
ServicesToRun = new ServiceBase[]
{
new Service1()
};
ServiceBase.Run(ServicesToRun);
#endif
จากนั้นในไฟล์ Service1.cs ให้เพิ่มคำสั่งต่างๆ ดังนี้
Service1.cs
protected override void OnStart(string[] args)
{
string strPath = AppDomain.CurrentDomain.BaseDirectory + "Log.txt";
System.IO.File.AppendAllLines(strPath, new[] { "Starting time : " + DateTime.Now.ToString() });
}
protected override void OnStop()
{
string strPath = AppDomain.CurrentDomain.BaseDirectory + "Log.txt";
System.IO.File.AppendAllLines(strPath, new[] { "Stop time : " + DateTime.Now.ToString() });
}
จาก Code จะเป็นเพียงการสร้างไฟล์ชื่อ Log.txt และเขียนข้อความพร้อมเวลาเข้าไปใน Text
ทดสอบการทำงาน
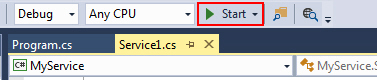
ที่คลิกรันเพื่อ Debug โปรแกรม
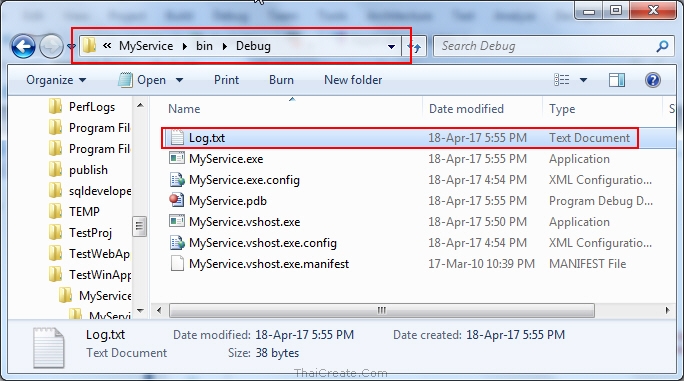
ไฟล์ Log.txt ที่ถูกสร้าง
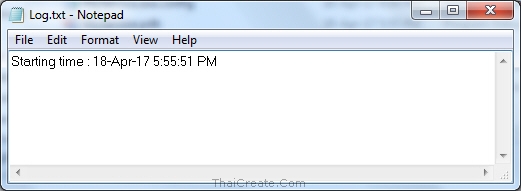
ข้อความที่ถูกเขียนลงไป
Note! เนื่องจากโหมดนี้เป็นเพียงการสร้าง Debug โหมดให้เรียกโดยตรง ฉะนั้นมันจะทำงานแค่ในส่วนของ OnStart เท่านั้น แต่ OnStop จะไม่ทำงาน แต่จะทำงานเมื่อตอนที่ได้ติดตั้งลงใน Services และมีการ Start, Stop เท่านั้น
Code ทั้งหมด
C#
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.ServiceProcess;
using System.Text;
using System.Threading.Tasks;
namespace MyService
{
static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
static void Main()
{
#if DEBUG
Service1 myService = new Service1();
myService.OnDebug();
System.Threading.Thread.Sleep(System.Threading.Timeout.Infinite);
#else
ServiceBase[] ServicesToRun;
ServicesToRun = new ServiceBase[]
{
new Service1()
};
ServiceBase.Run(ServicesToRun);
#endif
}
}
}
Service1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Diagnostics;
using System.Linq;
using System.ServiceProcess;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace MyService
{
public partial class Service1 : ServiceBase
{
public Service1()
{
InitializeComponent();
}
public void OnDebug()
{
this.OnStart(null);
}
protected override void OnStart(string[] args)
{
string strPath = AppDomain.CurrentDomain.BaseDirectory + "Log.txt";
System.IO.File.AppendAllLines(strPath, new[] { "Starting time : " + DateTime.Now.ToString() });
}
protected override void OnStop()
{
string strPath = AppDomain.CurrentDomain.BaseDirectory + "Log.txt";
System.IO.File.AppendAllLines(strPath, new[] { "Stop time : " + DateTime.Now.ToString() });
}
}
}
VB.Net
ใน VB.Net จะไม่มีไฟล์โปรแกรม Program.vb แต่จะอยู่ที่ Service1.Designer.vb
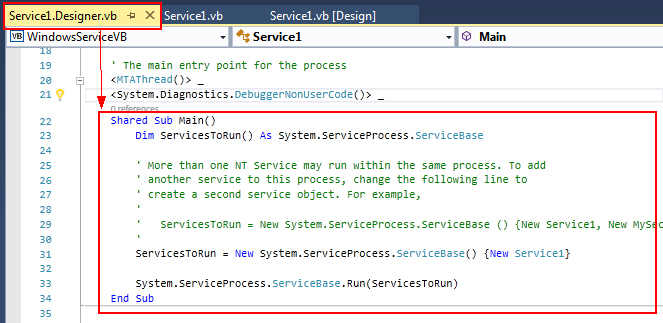
Service1.Designer.vb
Shared Sub Main()
#If DEBUG Then
Dim myService As New Service1()
myService.OnDebug()
System.Threading.Thread.Sleep(System.Threading.Timeout.Infinite)
#Else
Dim ServicesToRun() As System.ServiceProcess.ServiceBase
' More than one NT Service may run within the same process. To add
' another service to this process, change the following line to
' create a second service object. For example,
'
' ServicesToRun = New System.ServiceProcess.ServiceBase () {New Service1, New MySecondUserService}
'
ServicesToRun = New System.ServiceProcess.ServiceBase() {New Service1}
System.ServiceProcess.ServiceBase.Run(ServicesToRun)
#End If
End Sub
Service1.vb
Public Class Service1
Public Sub OnDebug()
Me.OnStart(Nothing)
End Sub
Protected Overrides Sub OnStart(ByVal args() As String)
Dim strPath As String = AppDomain.CurrentDomain.BaseDirectory + "Log.txt"
Dim lines() As String = {"Starting time : " + DateTime.Now.ToString()}
System.IO.File.AppendAllLines(strPath, lines)
End Sub
Protected Overrides Sub OnStop()
Dim strPath As String = AppDomain.CurrentDomain.BaseDirectory + "Log.txt"
Dim lines() As String = {"Stop time : " + DateTime.Now.ToString()}
System.IO.File.AppendAllLines(strPath, lines)
End Sub
End Class
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2017-04-19 15:32:50 /
2017-04-20 13:04:47 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|