ตอนที่ 11 : How to use Java (JSP) Delete Entity in Table Storage - ลบข้อมูลในตาราง |
ตอนที่ 11 : How to use Java (JSP) Delete Entity in Table Storage - ลบข้อมูลในตาราง บทความนี้เป็นตัวอย่างการใช้ Java (JSP) เพื่อลบหรือ Delete ข้อมูลที่อยู่บน Table Storage ของ Windows Azure โดยจะยกตัวอย่างการแสดงข้อมูลใน Table Storage ออกมาเป็นตาราง และ สามารถเลือกที่จะลบ Delete รายการข้อมูลต่าง ๆ ตามต้องการ ตัวอย่างนี้ยังคงใช้ Jar Library ของ Windows Azure ซึ่งสามารถใช้งานและเขียนได้ด้วยคำสั่งที่ง่าย ๆ และสั้นมาก
ข้อมูล Table Storage ที่อยู่บน Windows Azure
CustomerEntity.java เป็น Model Class สำหรับการเชื่อมต่อกับ Table Storage
package com.java.myapp;
import com.microsoft.windowsazure.services.table.client.*;
public class CustomerEntity extends TableServiceEntity {
public CustomerEntity(String pKey, String rKey) {
this.partitionKey = pKey;
this.rowKey = rKey;
}
public CustomerEntity() { }
String CustomerID;
String Name;
String Email;
String CountryCode;
String Budget;
String Used;
public String getCustomerID() {
return this.CustomerID;
}
public void setCustomerID(String sCustomerID) {
this.CustomerID = sCustomerID;
}
public String getName() {
return this.Name;
}
public void setName(String sName) {
this.Name = sName;
}
public String getEmail() {
return this.Email;
}
public void setEmail(String sEmail) {
this.Email = sEmail;
}
public String getCountryCode() {
return this.CountryCode;
}
public void setCountryCode(String sCountryCode) {
this.CountryCode = sCountryCode;
}
public String getBudget() {
return this.Budget;
}
public void setBudget(String sBudget) {
this.Budget = sBudget;
}
public String getUsed() {
return this.Used;
}
public void setUsed(String sUsed) {
this.Used = sUsed;
}
}
Syntax การ Delete
// Retrieve the entity with partition key of "myCustomer" and row key of "CustomerID".
TableOperation retrieveData =
TableOperation.retrieve("myCustomer", strCustomerID, CustomerEntity.class);
// Retrieve the entity with partition key of "myCustomer" and row key of "CustomerID".
CustomerEntity entity =
tableClient.execute("customer", retrieveData).getResultAsType();
// Create an operation to delete the entity.
TableOperation delete = TableOperation.delete(entity);
// Submit the delete operation to the table service.
tableClient.execute("customer", delete);
เป็นการ Delete ข้อมูลใน Table Storage ตามการ Filter ข้อมูลที่ต้องการ

Example เขียน Java (JSP) เพื่อ Delete ลบข้อมูล Table Storage บน Windows Azure
index.jsp (ไฟล์สำหรับแสดงรายการข้อมูลทั้งหมด และ สามารถเลือกรายการที่จะทำการลบได้)
<%@ page import="com.microsoft.windowsazure.services.core.storage.*" %>
<%@ page import="com.microsoft.windowsazure.services.table.client.*" %>
<%@ page import="com.microsoft.windowsazure.services.table.client.TableQuery.*" %>
<%@ page import="com.java.myapp.CustomerEntity" %>
<html>
<head>
<title>ThaiCreate.Com Azure Tutorial</title>
</head>
<body>
<%
String storageConnectionString =
"DefaultEndpointsProtocol=http;" +
"AccountName=[yourAccount];" +
"AccountKey=[yourKey]";
// Retrieve storage account from connection-string
CloudStorageAccount storageAccount = CloudStorageAccount.parse(storageConnectionString);
// Create the table client.
CloudTableClient tableClient = storageAccount.createCloudTableClient();
// Specify a partition query, using "Smith" as the partition key filter.
TableQuery<CustomerEntity> partitionQuery =
TableQuery.from("customer", CustomerEntity.class);
// Loop through the results, displaying information about the entity.
out.print("<table width=\"800\" border=\"1\">");
out.print("<tr>");
out.print("<th width=\"91\"> <div align=\"center\">PartitionKey </div></th>");
out.print("<th width=\"91\"> <div align=\"center\">RowKey </div></th>");
out.print("<th width=\"91\"> <div align=\"center\">CustomerID </div></th>");
out.print("<th width=\"98\"> <div align=\"center\">Name </div></th>");
out.print("<th width=\"198\"> <div align=\"center\">Email </div></th>");
out.print("<th width=\"97\"> <div align=\"center\">CountryCode </div></th>");
out.print("<th width=\"59\"> <div align=\"center\">Budget </div></th>");
out.print("<th width=\"71\"> <div align=\"center\">Used </div></th>");
out.print("<th width=\"71\"> <div align=\"center\">Delete </div></th>");
out.print("</tr>");
for (CustomerEntity entity : tableClient.execute(partitionQuery)) {
out.print("<tr>");
out.print("<td><div align=\"center\">" + entity.getPartitionKey() + "</div></td>");
out.print("<td><div align=\"center\">" + entity.getRowKey() + "</div></td>");
out.print("<td><div align=\"center\">" + entity.getCustomerID() + "</div></td>");
out.print("<td>" + entity.getName() + "</td>");
out.print("<td>" + entity.getEmail() + "</td>");
out.print("<td><div align=\"center\">" + entity.getCountryCode() + "</div></td>");
out.print("<td align=\"right\">" + entity.getBudget() + "</td>");
out.print("<td align=\"right\">" + entity.getUsed() + "</td>");
out.print("<td align=\"center\"><a href='delete.jsp?CusID=" + entity.getCustomerID() + "'>Del</a></td>");
out.print("</tr>");
}
out.print("</table>");
%>
</body>
</html>
delete.jsp (ไฟล์สำหรับแสดงรายการข้อมูลทั้งหมด และ สามารถเลือกรายการที่จะทำการลบได้)
<%@ page import="com.microsoft.windowsazure.services.core.storage.*" %>
<%@ page import="com.microsoft.windowsazure.services.table.client.*" %>
<%@ page import="com.microsoft.windowsazure.services.table.client.TableQuery.*" %>
<%@ page import="com.java.myapp.CustomerEntity" %>
<html>
<head>
<title>ThaiCreate.Com Azure Tutorial</title>
</head>
<body>
<%
String storageConnectionString =
"DefaultEndpointsProtocol=http;" +
"AccountName=[yourAccount];" +
"AccountKey=[yourKey]";
// Retrieve storage account from connection-string
CloudStorageAccount storageAccount = CloudStorageAccount.parse(storageConnectionString);
// Create the table client.
CloudTableClient tableClient = storageAccount.createCloudTableClient();
// Get Param
String strCustomerID = request.getParameter("CusID");
// Retrieve the entity with partition key of "myCustomer" and row key of "CustomerID".
TableOperation retrieveData =
TableOperation.retrieve("myCustomer", strCustomerID, CustomerEntity.class);
// Retrieve the entity with partition key of "myCustomer" and row key of "CustomerID".
CustomerEntity entity =
tableClient.execute("customer", retrieveData).getResultAsType();
// Create an operation to delete the entity.
TableOperation delete = TableOperation.delete(entity);
// Submit the delete operation to the table service.
tableClient.execute("customer", delete);
// Redirect to index.jsp
response.sendRedirect("index.jsp");
%>
</body>
</html>
Screenshot
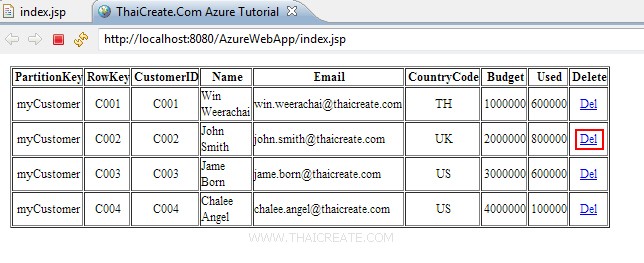
แสดงรายการข้อมูลจาก Table Storage ให้เลือกรายการที่ต้องการ Delete
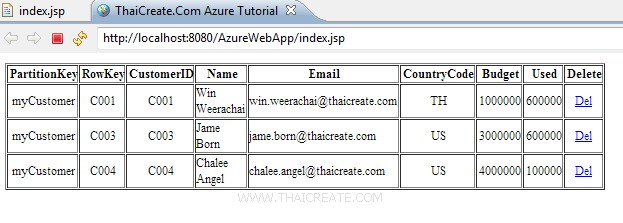
หลังจากลบแล้วข้อมูลจะหายไปทันที
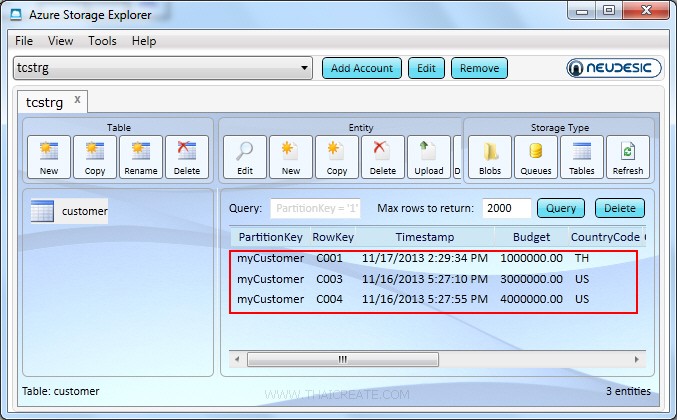
เมื่อเข้าไปดูใน Table Storage บน Windows Azure ผ่านโปรแกรม Azure Storage Explorer ข้อมูลจะมีการลบ Delete ตามที่เราลบไปก่อนหน้านี้
บทความที่เกี่ยวข้อง
บทความถัดไปที่แนะนำให้อ่าน
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-11-30 08:28:48 /
2017-03-24 14:21:51 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|