ตอนที่ 17 : Show Case 4 : Delete Data (Android and Mobile Services) |
ตอนที่ 17 : Show Case 4 : Delete Data (Android and Mobile Services) ตัวอย่าง Show Case การเขียน Android กับ Mobile Services เพื่อที่จะทำการลบ Delete ข้อมูลในที่อยู่ในตาราง Table ด้วยการแสดงรายการทั้งหมดบน ListView จากนั้นผู้ใช้สามารที่จะเลือกรายการที่ต้องการลบ และในการลบจะมี Dialog สำหรับการ Confirm หลังจาก Confirm แล้วข้อมูลก็จะถูกลบออกจากรายการของ ListView รวมทั้งลบออกจากรายการของ Mobile Services ที่อยู่บน Windows Azure
Android Mobile Services Delete Data
สำหรับขั้นตอนนั้นก็คือสร้าง Activity ด้วย ListView จากนั้นดึงข้อมูลมาจาก Mobile Services และแต่ล่ะ Item สามารถที่จะเลือกรายการเพื่อ Delete ลบข้อมูลของ Mobile Services ที่อยู่บน Windows Azure ได้
Example ตัวอย่างการทำระบบลบข้อมูล Delete Data

ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
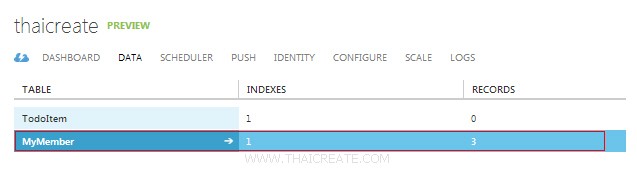
ชื่อตารางว่า MyMember
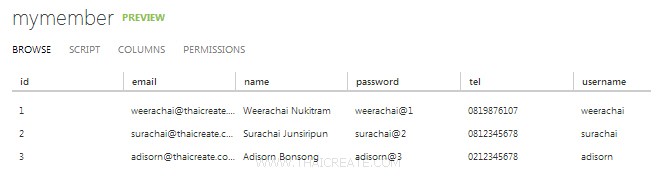
มีข้อมูลอยู่ทั้งหมด 4 รายการ

กลับมายัง Android Project บน Eclipse
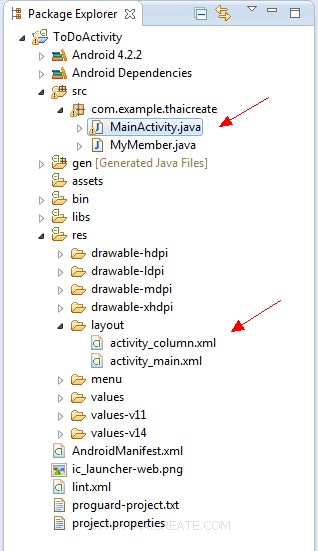
โครงสร้างไฟล์ทั้งหมด
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
activity_column.xml
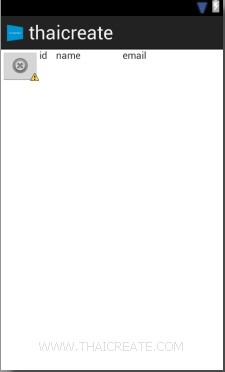
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<ImageButton
android:id="@+id/btnDelete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@android:drawable/ic_notification_clear_all" />
<TextView
android:id="@+id/col_id"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.5"
android:text="id"/>
<TextView
android:id="@+id/col_username"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="name"/>
<TextView
android:id="@+id/col_name"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="3"
android:text="email" />
</LinearLayout>
MyMember.java
package com.example.thaicreate;
public class MyMember {
@com.google.gson.annotations.SerializedName("id")
private int mId;
@com.google.gson.annotations.SerializedName("username")
private String mUsername;
@com.google.gson.annotations.SerializedName("password")
private String mPassword;
@com.google.gson.annotations.SerializedName("name")
private String mName;
@com.google.gson.annotations.SerializedName("tel")
private String mTel;
@com.google.gson.annotations.SerializedName("email")
private String mEmail;
public MyMember() {
// empty
}
public MyMember(String username, String password,
String name,String tel,String email) {
this.setUsername(username);
this.setPassword(password);
this.setName(name);
this.setTel(tel);
this.setEmail(email);
}
public final void setId(int id) {
mId = id;
}
public int getId() {
return mId;
}
public final void setUsername(String username) {
mUsername = username;
}
public String getUsername() {
return mUsername;
}
public final void setPassword(String password) {
mPassword = password;
}
public String getPassword() {
return mPassword;
}
public final void setName(String name) {
mName = name;
}
public String getName() {
return mName;
}
public final void setTel(String tel) {
mTel = tel;
}
public String getTel() {
return mTel;
}
public final void setEmail(String email) {
mEmail = email;
}
public String getEmail() {
return mEmail;
}
}
MainActivity.java
package com.example.thaicreate;
import java.net.MalformedURLException;
import java.util.List;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.Color;
import android.os.Bundle;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageButton;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import com.microsoft.windowsazure.mobileservices.MobileServiceClient;
import com.microsoft.windowsazure.mobileservices.MobileServiceTable;
import com.microsoft.windowsazure.mobileservices.ServiceFilterResponse;
import com.microsoft.windowsazure.mobileservices.TableDeleteCallback;
import com.microsoft.windowsazure.mobileservices.TableQueryCallback;
public class MainActivity extends Activity {
private MobileServiceClient mClient;
private MobileServiceTable<MyMember> mMyMember;
private MyMemberAdapter mAdapter;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
/*** Create to Mobile Service ***/
try {
mClient = new MobileServiceClient(
"https://thaicreate.azure-mobile.net/",
"QUjngFknhHZjdaGgYAAzdoXkOzKoxi24",
this);
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
/*** Create getTable ***/
mMyMember = mClient.getTable(MyMember.class);
// listView1
final ListView lstView1 = (ListView)findViewById(R.id.listView1);
mAdapter = new MyMemberAdapter(this,R.layout.activity_column);
lstView1.setAdapter(mAdapter);
registerForContextMenu(lstView1);
refreshItemsFromTable();
}
private void refreshItemsFromTable() {
mMyMember.execute(new TableQueryCallback<MyMember>() {
public void onCompleted(List<MyMember> result, int count, Exception exception,
ServiceFilterResponse response) {
if (exception == null) {
mAdapter.clear();
for (MyMember item : result) {
mAdapter.add(item);
}
} else {
Log.d("Error","Error Load Data from Mobile Service");
}
}
});
}
public class MyMemberAdapter extends ArrayAdapter<MyMember> {
Context mContext;
int mLayoutResourceId;
public MyMemberAdapter(Context context, int layoutResourceId) {
super(context, layoutResourceId);
mContext = context;
mLayoutResourceId = layoutResourceId;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View row = convertView;
final MyMember currentItem = getItem(position);
if (row == null) {
LayoutInflater inflater = ((Activity) mContext).getLayoutInflater();
row = inflater.inflate(mLayoutResourceId, parent, false);
}
row.setTag(currentItem);
// imgCmdDelete
ImageButton cmdDelete = (ImageButton) row.findViewById(R.id.btnDelete);
cmdDelete.setBackgroundColor(Color.TRANSPARENT);
final AlertDialog.Builder adb = new AlertDialog.Builder(MainActivity.this);
cmdDelete.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
adb.setTitle("Delete?");
adb.setMessage("Are you sure delete ?");
adb.setNegativeButton("Cancel", null);
adb.setPositiveButton("Ok", new AlertDialog.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
//** Select Record ***//
mMyMember.where().field("id").eq(currentItem.getId())
.execute(new TableQueryCallback<MyMember>() {
public void onCompleted(List<MyMember> result, int count, Exception exception,
ServiceFilterResponse response) {
if (exception == null) {
MyMember item = result.get(0);
// Delete Record ***/
mMyMember.delete(item, new TableDeleteCallback() {
public void onCompleted(Exception exception,
ServiceFilterResponse response) {
if(exception == null){
Toast.makeText(MainActivity.this, "Delete Data Successfully", Toast.LENGTH_SHORT).show();
}
else
{
Toast.makeText(MainActivity.this, "Delete Failed!", Toast.LENGTH_SHORT).show();
Log.d("Error : ",exception.getMessage());
}
}
});
} else {
Log.d("Error","Error Load Data from Mobile Service");
}
}
});
refreshItemsFromTable(); // Reload New Data
}});
adb.show();
}
});
// Column id
final TextView txt_id = (TextView) row.findViewById(R.id.col_id);
txt_id.setText(String.valueOf(currentItem.getId()));
// Column username
final TextView txt_name = (TextView) row.findViewById(R.id.col_username);
txt_name.setText(currentItem.getUsername());
// Column name
final TextView txt_email = (TextView) row.findViewById(R.id.col_name);
txt_email.setText(currentItem.getName());
return row;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot
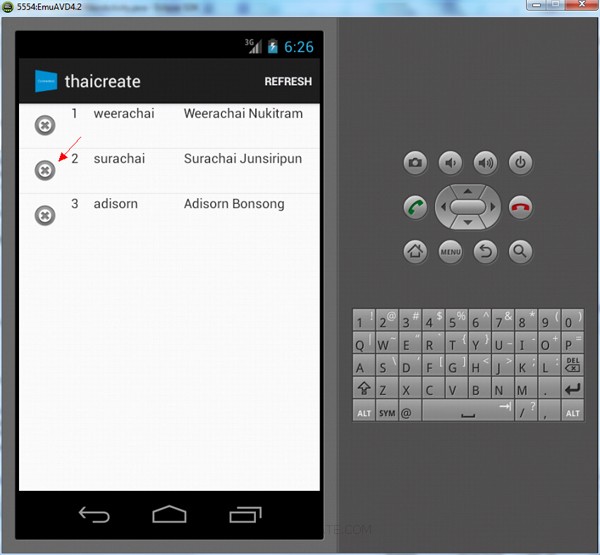
แสดงข้อมูลบน ListView และจะมีปุ่ม Button สำหรับ Delete ในแต่ล่ะ item
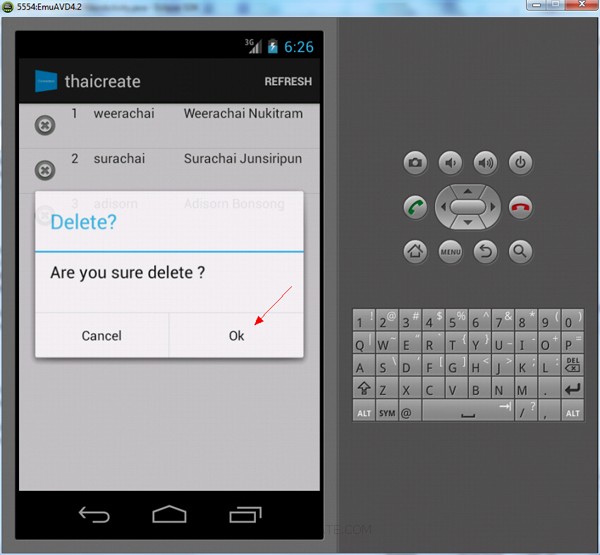
มี Dialog ในการที่จะ Confirm ข้อมูล
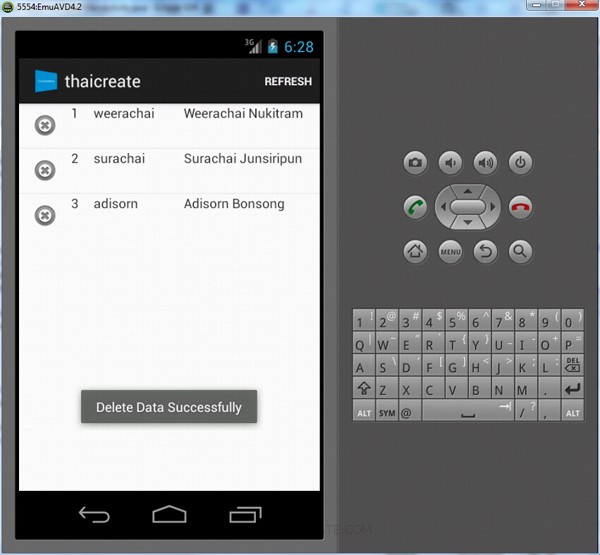
กรณีที่ Delete ข้อมูล
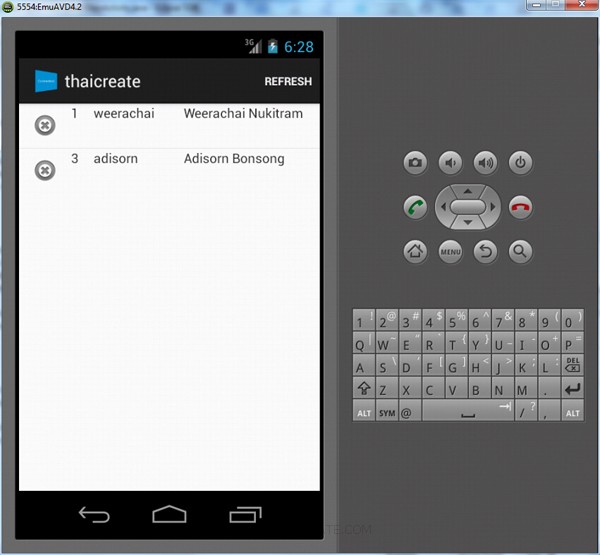
ข้อมูลจะหายไปจากรายการของ ListView
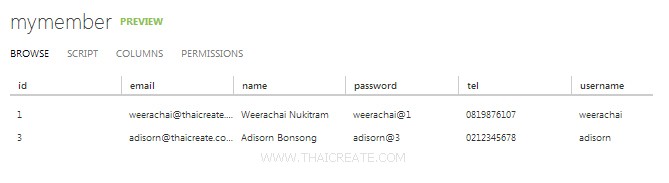
เมื่อกลับไปดูที่ Data บน Mobile Service ข้อมูลก็จะถูกลบออกไปด้วย
บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|