ตอนที่ 8 : การทำ Authentication in Azure Mobile Services ด้วย iOS / iPhone |
ตอนที่ 8 : การทำ Authentication in Azure Mobile Services ด้วย iOS / iPhone ถ้าเคยใช้พวก App บน Mobile บ่อย ๆ เราอาจจะเคยเห็นว่า App บางตัวสามารถที่จะติดต่อกับ Social Network พวก Twitter , Facebook หรือ Google Account ได้ เช่นการโพสข้อความ หรือการแสดงข้อมูลต่าง ๆ จาก Social Network ที่เรา Login ในเครื่องนั้น ได้ และบน Mobile Services ของ Windows Azure ก็สามารถที่จะทำการเชื่อมต่อไปยัง Social Network เหล่านั้นได้เช่นเดียวกัน โดยในปัจจุบันของรับ 4 ตัวด้วยกันคือ
- Microsoft Account
- Facebook login
- Twitter login
- Google login
ในการเขียน Mobile Services เพื่อทำการ Authentication Account จาก Social และ Provider เหล่านี้ เราจะต้องทำการ Register เพื่อขอพวก ID จาก Provider นั้น ๆ ก่อน

ตอนนี้เรามี Mobile Services ทีได้สร้างไว้ก่อนหน้านี้แล้ว 1 ตัว
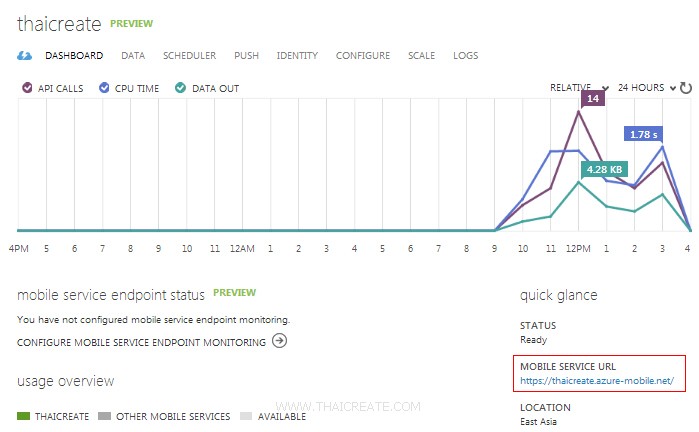
หน้าจอ Dashboard ของ Mobile Services จะมี URL ของ Mobile Services อยู่ ซึ่งเราจะต้องเอา URL นี้ไปทำการ Register กับ Social ของค่ายนั้น ๆ ก่อน
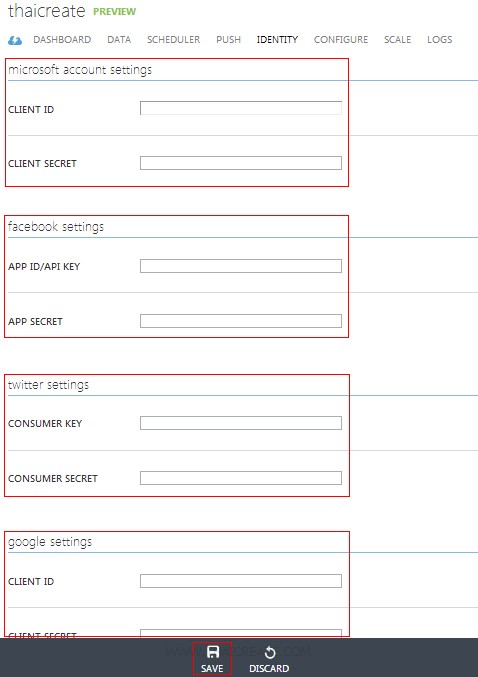
หน้าจอสำหรับการกำหนด ID ของ Social แต่ล่ะค่าย
ลงทะบียนของแต่ล่ะค่ายได้ตาม Link นี้
Example ในตัวอย่างนี้เราจะกำหนดว่าจะต้อง Login ผ่าน Social Provider ของ Google ก่อนถึงจะสามารถดูข้อมูลได้
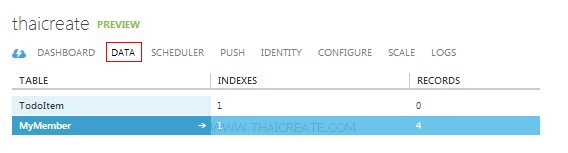
ให้ไปที่ DATA เลือก Table ที่ต้องการ
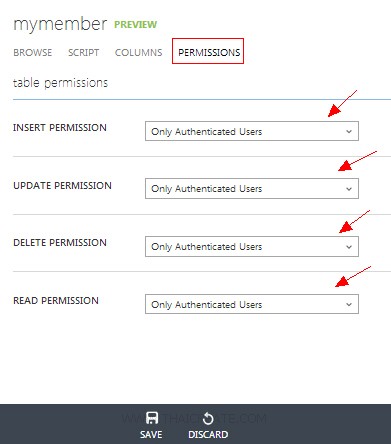
ในส่วนของ PERMISSIONS ให้เลือกเป็นแบบ Only Authenticateds Users
จากนั้นในส่วนของ Code สามารถที่จะเขียนดักการ Authentication ด้วยคำสั่งง่าย ๆ นี้
- (void)viewDidLoad
{
[super viewDidLoad];
// Start Service
self.memberService = [MyMemberService defaultService];
MSClient *client = self.memberService.client;
[client loginWithProvider:@"Google" controller:self animated:YES completion:^(MSUser *user, NSError *error) {
if (client.currentUser == nil) {
UIAlertView *alert =[[UIAlertView alloc]
initWithTitle:@"Error"
message:@"You must log in. Login Required" delegate:self
cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
}
else
{
[self.memberService refreshDataOnSuccess:^
{
[myTable reloadData];
}];
}
}];
}
มาดูตัวอย่างการทำ Authentication ผ่าน Google provider
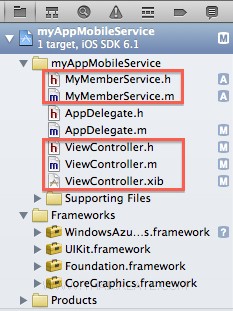
ในหน้า Project iOS บน Xcode โครงสร้างไฟล์
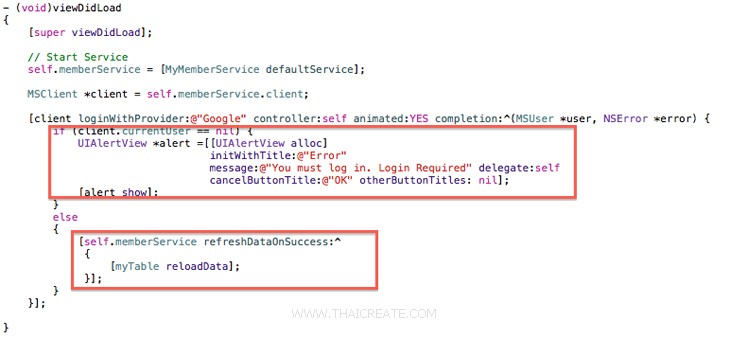
ก่อนการแสดงผลข้อมูลเราจะเขียนดักเงื่อนไขตรวจสอบการ Login ของ Account ที่ต้องการตรวจสอบหรือไม่ เป็นการสร้างเงื่อนไข if แบบง่าย ๆ
Screenshot
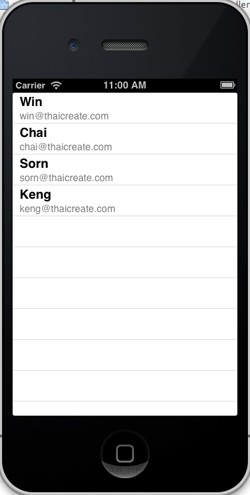
กรณีที่ Authen ผ่าน
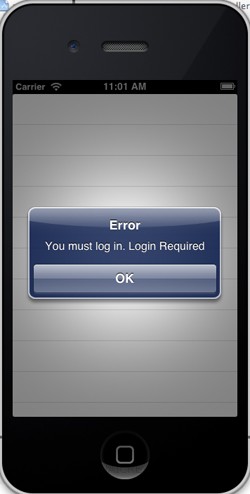
กรณีที่ Authen ไม่ผ่าน
ในไฟล์ ViewController.h และ ViewController.m เขียน Code ดังนี้
ViewController.h
//
// ViewController.h
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UITableView *myTable;
}
@end
ViewController.m
//
// ViewController.m
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import "MyMemberService.h"
#import "ViewController.h"
@interface ViewController ()
// Private properties
@property (strong, nonatomic) MyMemberService *memberService;
@end
@implementation ViewController
@synthesize memberService;
- (void)viewDidLoad
{
[super viewDidLoad];
// Start Service
self.memberService = [MyMemberService defaultService];
MSClient *client = self.memberService.client;
[client loginWithProvider:@"Google" controller:self animated:YES completion:^(MSUser *user, NSError *error) {
if (client.currentUser == nil) {
UIAlertView *alert =[[UIAlertView alloc]
initWithTitle:@"Error"
message:@"You must log in. Login Required" delegate:self
cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alert show];
}
else
{
[self.memberService refreshDataOnSuccess:^
{
[myTable reloadData];
}];
}
}];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSDictionary *item = [self.memberService.items objectAtIndex:indexPath.row];
cell.textLabel.text = [item objectForKey:@"name"];
cell.detailTextLabel.text= [item objectForKey:@"email"];
return cell;
}
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Always a single section
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of items in the todoService items array
return [self.memberService.items count];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[myTable release];
[super dealloc];
}
@end
อันนี้ Code ในไฟล์ MyMemberService.h และ MyMemberService.m
MyMemberService.h
//
// MyMemberService.h
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import <Foundation/Foundation.h>
typedef void (^QSCompletionBlock) ();
typedef void (^QSBusyUpdateBlock) (BOOL busy);
@interface MyMemberService : NSObject
@property (nonatomic, strong) NSArray *items;
@property (nonatomic, strong) MSClient *client;
@property (nonatomic, copy) QSBusyUpdateBlock busyUpdate;
+ (MyMemberService *)defaultService;
- (void)refreshDataOnSuccess:(QSCompletionBlock)completion;
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response;
@end
MyMemberService.m
//
// MyMemberService.m
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import "MyMemberService.h"
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
@interface MyMemberService() <MSFilter>
@property (nonatomic, strong) MSTable *table;
@property (nonatomic) NSInteger busyCount;
@end
@implementation MyMemberService
@synthesize items;
+ (MyMemberService *)defaultService
{
// Create a singleton instance of MyMemberService
static MyMemberService* service;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
service = [[MyMemberService alloc] init];
});
return service;
}
-(MyMemberService *)init
{
self = [super init];
if (self)
{
// Initialize the Mobile Service client with your URL and key
MSClient *client = [MSClient clientWithApplicationURLString:@"https://thaicreate.azure-mobile.net/"
applicationKey:@"uJPAhkAkcTBuTyCNxaOSnDKFzkoYqB49"];
// Add a Mobile Service filter to enable the busy indicator
self.client = [client clientWithFilter:self];
// Create an MSTable instance to allow us to work with the TodoItem table
self.table = [_client tableWithName:@"MyMember"];
self.items = [[NSMutableArray alloc] init];
self.busyCount = 0;
}
return self;
}
- (void)refreshDataOnSuccess:(QSCompletionBlock)completion
{
// Query the TodoItem table and update the items property with the results from the service
[self.table readWithCompletion:^(NSArray *results, NSInteger totalCount, NSError *error)
{
[self logErrorIfNotNil:error];
items = [results mutableCopy];
// Let the caller know that we finished
completion();
}];
}
- (void)busy:(BOOL)busy
{
// assumes always executes on UI thread
if (busy)
{
if (self.busyCount == 0 && self.busyUpdate != nil)
{
self.busyUpdate(YES);
}
self.busyCount ++;
}
else
{
if (self.busyCount == 1 && self.busyUpdate != nil)
{
self.busyUpdate(FALSE);
}
self.busyCount--;
}
}
- (void)logErrorIfNotNil:(NSError *) error
{
if (error)
{
NSLog(@"ERROR %@", error);
}
}
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response
{
// A wrapped response block that decrements the busy counter
MSFilterResponseBlock wrappedResponse = ^(NSHTTPURLResponse *innerResponse, NSData *data, NSError *error)
{
[self busy:NO];
response(innerResponse, data, error);
};
// Increment the busy counter before sending the request
[self busy:YES];
next(request, wrappedResponse);
}
@end
Download Code ทั้งหมดได้จากท้ายของบทความ
บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
|
|
|
Create/Update Date : |
2013-06-15 19:51:04 /
2017-03-24 11:50:42 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|