ตอนที่ 12 : การเขียน Scripts to authorize users in Mobile Services บน iOS / iPhone |
ตอนที่ 12 : การเขียน Scripts to authorize users in Mobile Services บน iOS / iPhone บน Mobile Services ของ Windows Azure เราสามารถทำการเขียน Script เพื่อทำการ authorize authenticated users หมายถึงการระบุข้อมูลเฉพาะ id ของตัวเอง โดยสามารถที่จะเขียน Script เพื่อให้ข้อมูลนั้น ๆ เป็นของ users และตอนที่แสดงผลก็สามารถที่จะเลือกข้อมูลเฉพาะของ users นั้น ๆ ได้ ตัวอย่างเช่น
insert() เป็นการเขียน Script ส่วนของของคำสั่ง Insert บน Mobile Services
function insert(item, user, request) {
item.userId = user.userId;
request.execute();
}
read() เป็นการเขียน Script ส่วนของของคำสั่ง Read บน Mobile Services
function insert(item, user, request) {
item.userId = user.userId;
request.execute();
}
เมื่อกำหนดเงื่อนไขตามตัวอย่างก็จะดึงเฉพาะของ User นั้น ๆ ที่ Authen เข้ามาในระบบ
User ที่ Authentication หมายถึง user ที่ authentication ผ่าน identity provider ผ่านพวก Google , Microsoft , Facebook ,Twitter
นอกจากนี้เรายังสามารถเขียน Query ในฝั่งของ Windows Phone เพื่อให้ทำงานอื่น ๆ ได้หลากหลาย เช่น
ตรวจสอบความยาวของข้อมูลจะต้องไม่เกิน 10 ตัวอีกษร
function insert(item, user, request) {
if (item.text.length > 10) {
request.respond(statusCodes.BAD_REQUEST, 'Text length must be under 10');
} else {
request.execute();
}
}
กำหนดค่าไปใน Query ตอนที่ทำการ insert()
function insert(item, user, request) {
item.scriptComment =
'this was added by a script and will be saved to the database';
request.execute();
}
กำหนดค่าเข้าไปใน Query ตอนที่มีการ update()
function update(item, user, request) {
item.scriptComment =
'this was added by a script and will be saved to the database';
request.execute();
}
สร้างเงื่อนไขตอนที่มีการ read()
function read(query, user, request) {
// Only return records for the current user
query.where({ userid: user.userId});
request.execute();
}
insert() ข้อมูล column ชื่อว่า owner เท่ากับ user ที่ทำการ authen
function insert(item, user, request) {
item.owner = user.userId;
request.execute();
}
query ข้อมูลจาก user ที่ authen
function read(query, user, request) {
query.where({
owner: user.userId
});
request.execute();
}
ตรวจสอบข้อมูล่า user ที่ส่งมาจาก item กับที่ authen ตรงกันหรือไม่
function insert(item, user, request) {
if (item.userId !== user.userId) {
request.respond(statusCodes.FORBIDDEN,
'You may only insert records with your userId.');
} else {
request.execute();
}
}
ตรวจสอบการอ่านข้อมูล และการเพิ่มข้อมูล column ที่ query แล้ว
function read(query, user, request) {
request.execute({
success: function(results) {
results.forEach(function(r) {
r.scriptComment =
'this was added by a script after querying the database';
});
request.respond();
}
});
}

ตัวอย่างการ update และการสร้าง log
function update(item, user, request) {
request.execute({
error: function(err) {
// Do some custom logging, then call respond.
request.respond();
}
});
}
การเขียน query ก่อนการ insert()
function insert(item, user, request) {
var todoItemTable = tables.getTable('TodoItem');
// Check the supplied custom parameter to see if
// we should allow duplicate text items to be inserted.
if (request.parameters.duplicateText === 'false') {
// Find all existing items with the same text
// and that are not marked 'complete'.
todoItemTable.where({
text: item.text,
complete: false
}).read({
success: insertItemIfNotComplete
});
} else {
request.execute();
}
function insertItemIfNotComplete(existingItems) {
if (existingItems.length > 0) {
request.respond(statusCodes.CONFLICT,
"Duplicate items are not allowed.");
} else {
// Insert the item as normal.
request.execute();
}
}
}
อ่านเพิ่มเติมได้ที่นี่
Example ตัวอย่างการเขียน Script และ Query แบบง่าย ๆ

ตอนนี้เรามี Mobile Service อยู่ 1 รายการ
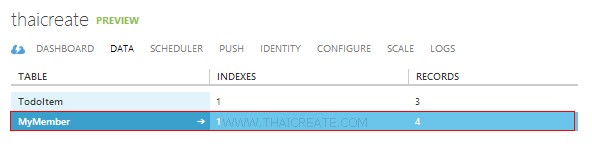
มีตารางชื่อว่า MyMember มีข้อมูลอยู่ 4 รายการ
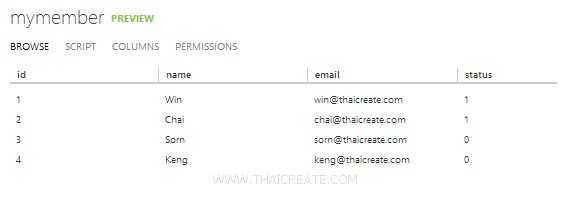
มีข้อมูลอยู่ 4 รายการ
กลับมายัง Project ของ iOS บน Xcode
สำหรับพื้นฐานการสร้าง Project และการสร้าง Table รวมทั้งการติดต่อระหว่าง iOS กับ Mobile Services บน Windows Azure แนะนำให้อ่านบทความในตอนที่ 5
ตอนที่ 5: iOS / iPhone สร้างตาราง Table บน Mobile Services และการ Insert ข้อมูล

โครงสร้างไฟล์
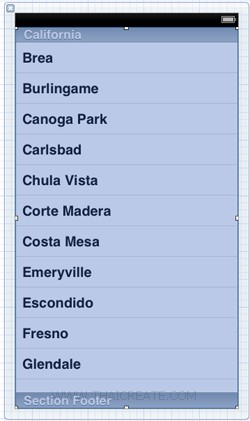
เราจะแสดงข้อมูลบน TableView
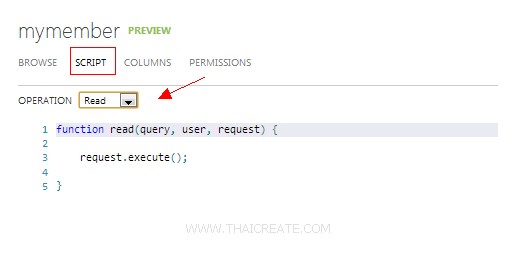
สังเกตุว่าตอนนี้จะใช้การอ่านข้อมูลจาก Table ทั้งหมด
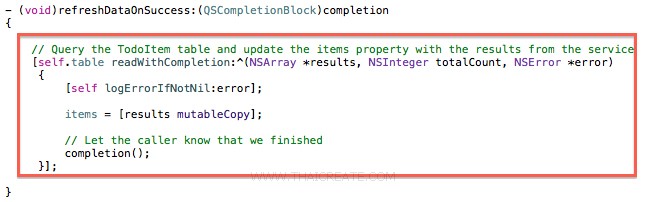
บน query ของ read() ก็ยังไม่ได้เขียนอะไรเพิ่ม
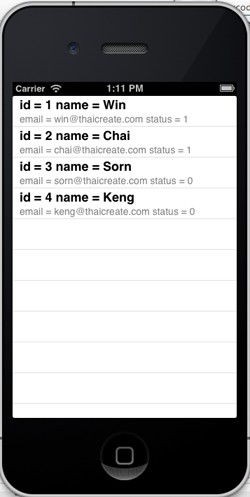
ผลลัพธ์ที่ได้
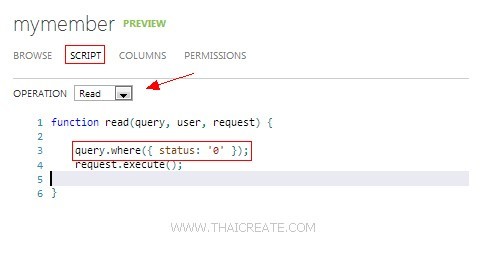
ลองแก้ไข Script บน Mobile Services ให้แก้คำสั่ง read() เป็นตามในรูปภาพ
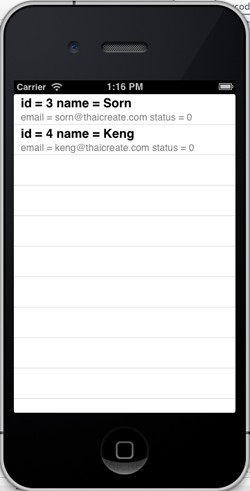
ผลลัพธ์ที่ได้เหมือนทำการแก้ไข Script บน Mobile Service ของ Windows Azure
สำหรับการเขียนและใช้งาน Scripts อยากให้ลองเข้าไปศึกษาในบทความนี้ครับ
ในไฟล์ ViewController.h และ ViewController.m เขียน Code ดังนี้
ViewController.h
//
// ViewController.h
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UITableView *myTable;
}
@end
ViewController.m
//
// ViewController.m
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import "MyMemberService.h"
#import "ViewController.h"
@interface ViewController ()
// Private properties
@property (strong, nonatomic) MyMemberService *memberService;
@end
@implementation ViewController
@synthesize memberService;
- (void)viewDidLoad
{
[super viewDidLoad];
// Start Service
self.memberService = [MyMemberService defaultService];
[self.memberService refreshDataOnSuccess:^
{
[myTable reloadData];
}];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSDictionary *item = [self.memberService.items objectAtIndex:indexPath.row];
NSString *col1 =[NSString stringWithFormat:@"id = %@ name = %@",
[item objectForKey:@"id"],
[item objectForKey:@"name"]];
cell.textLabel.text = col1;
NSString *col2 =[NSString stringWithFormat:@"email = %@ status = %@",
[item objectForKey:@"email"],
[item objectForKey:@"status"]];
cell.detailTextLabel.text= col2;
return cell;
}
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Always a single section
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of items in the todoService items array
return [self.memberService.items count];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[myTable release];
[super dealloc];
}
@end
อันนี้ Code ในไฟล์ MyMemberService.h และ MyMemberService.m
MyMemberService.h
//
// MyMemberService.h
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import <Foundation/Foundation.h>
typedef void (^QSCompletionBlock) ();
typedef void (^QSBusyUpdateBlock) (BOOL busy);
@interface MyMemberService : NSObject
@property (nonatomic, strong) NSArray *items;
@property (nonatomic, strong) MSClient *client;
@property (nonatomic, copy) QSBusyUpdateBlock busyUpdate;
+ (MyMemberService *)defaultService;
- (void)refreshDataOnSuccess:(QSCompletionBlock)completion;
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response;
@end
MyMemberService.m
//
// MyMemberService.m
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import "MyMemberService.h"
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
@interface MyMemberService() <MSFilter>
@property (nonatomic, strong) MSTable *table;
@property (nonatomic) NSInteger busyCount;
@end
@implementation MyMemberService
@synthesize items;
+ (MyMemberService *)defaultService
{
// Create a singleton instance of MyMemberService
static MyMemberService* service;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
service = [[MyMemberService alloc] init];
});
return service;
}
-(MyMemberService *)init
{
self = [super init];
if (self)
{
// Initialize the Mobile Service client with your URL and key
MSClient *client = [MSClient clientWithApplicationURLString:@"https://thaicreate.azure-mobile.net/"
applicationKey:@"uJPAhkAkcTBuTyCNxaOSnDKFzkoYqB49"];
// Add a Mobile Service filter to enable the busy indicator
self.client = [client clientWithFilter:self];
// Create an MSTable instance to allow us to work with the TodoItem table
self.table = [_client tableWithName:@"MyMember"];
self.items = [[NSMutableArray alloc] init];
self.busyCount = 0;
}
return self;
}
- (void)refreshDataOnSuccess:(QSCompletionBlock)completion
{
// Query the TodoItem table and update the items property with the results from the service
[self.table readWithCompletion:^(NSArray *results, NSInteger totalCount, NSError *error)
{
[self logErrorIfNotNil:error];
items = [results mutableCopy];
// Let the caller know that we finished
completion();
}];
}
- (void)busy:(BOOL)busy
{
// assumes always executes on UI thread
if (busy)
{
if (self.busyCount == 0 && self.busyUpdate != nil)
{
self.busyUpdate(YES);
}
self.busyCount ++;
}
else
{
if (self.busyCount == 1 && self.busyUpdate != nil)
{
self.busyUpdate(FALSE);
}
self.busyCount--;
}
}
- (void)logErrorIfNotNil:(NSError *) error
{
if (error)
{
NSLog(@"ERROR %@", error);
}
}
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response
{
// A wrapped response block that decrements the busy counter
MSFilterResponseBlock wrappedResponse = ^(NSHTTPURLResponse *innerResponse, NSData *data, NSError *error)
{
[self busy:NO];
response(innerResponse, data, error);
};
// Increment the busy counter before sending the request
[self busy:YES];
next(request, wrappedResponse);
}
@end
สามารถทำการดาวน์โหลด Code ทั้งหมดได้จากท้ายบทความ
บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|