ตอนที่ 7 : อ่านข้อมูล Mobile Services แบบมีเงื่อนไข Where และแสดงผล iOS / iPhone |
ตอนที่ 7 : อ่านข้อมูล Mobile Services แบบมีเงื่อนไข Where และแสดงผล iOS / iPhone บทความนี้จะเป็นการใช้ iOS / iPhone อ่านข้อมูลจาก Table ของ Mobile Services บน Windows Azure โดยจะยกตัวอย่างการเลือกข้อมูลแบบมีเงื่อนไข (Where Clause) เหมือนกับการ Where ใน Query ของ Table ทั่ว ๆ ไป และจะนำผลลัพธ์ที่ได้ไปแสดงบนในหน้าจอของ iOS ด้วยการใช้ TableView เพื่อแสดงรายการทั้งหมดที่ค้นพบ
รูปแบบการอ่านข้อมูลจาก Table แบบมีเงื่อนไข Where ของ Mobile Services ด้วย iOS
- (void)refreshDataOnSuccess:(QSCompletionBlock)completion
{
// Create a predicate that finds items where complete is false
NSPredicate * predicate = [NSPredicate predicateWithFormat:@"id == '1'"];
// Query the TodoItem table and update the items property with the results from the service
[self.table readWithPredicate:predicate completion:^(NSArray *results, NSInteger totalCount, NSError *error)
{
[self logErrorIfNotNil:error];
items = [results mutableCopy];
// Let the caller know that we finished
completion();
}];
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Start Service
self.memberService = [MyMemberService defaultService];
[self.memberService refreshDataOnSuccess:^
{
[myTable reloadData];
}];
}
รูปแบบการใช้ iOS อ่านข้อมูลจาก Mobile Services บน Windows Azure ด้วยใช้การ Where เงื่อนไข

ตอนนี้เรามี Mobile Services อยู่ 1 ตัว
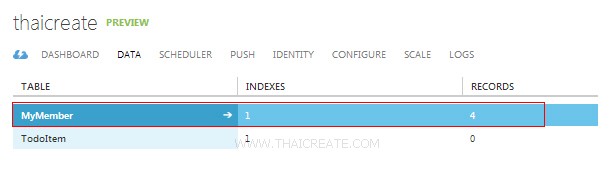
ชื่อตารางว่า MyMember
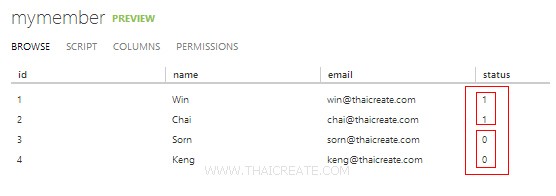
ข้อมูลในตาราง MyMember ประกอบด้วย Column ชื่อว่า id,name,email และ status

กลับมายังหน้า Project iOS บน Xcode
สำหรับพื้นฐานการสร้าง Project และการสร้าง Table รวมทั้งการติดต่อระหว่าง iOS กับ Mobile Services บน Windows Azure แนะนำให้อ่านบทความในตอนที่ 5
ตอนที่ 5: iOS / iPhone สร้างตาราง Table บน Mobile Services และการ Insert ข้อมูล
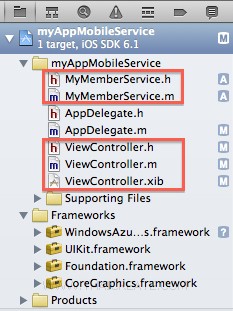
โครงสร้างไฟล์
ตัวอย่างการเขียน Query แบบ Where
Ex 1 : จะใช้ Where ด้วย Status = '1'
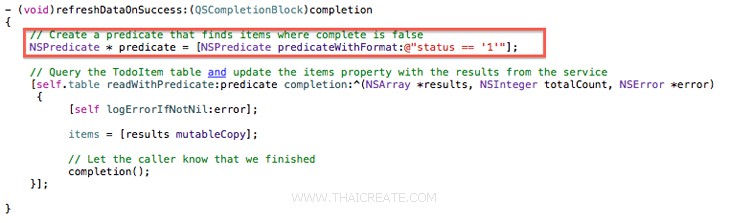
Screenshot
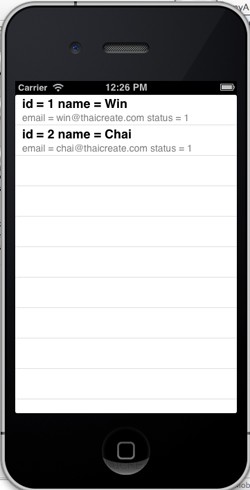
Ex 2 : จะใช้ Where ด้วย id = 1
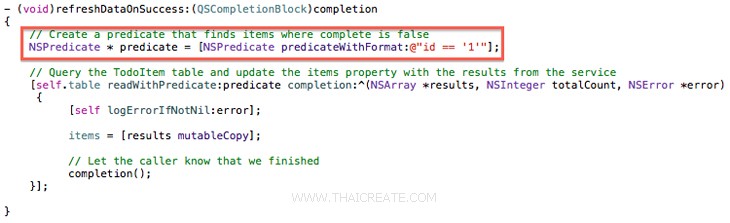
Screenshot
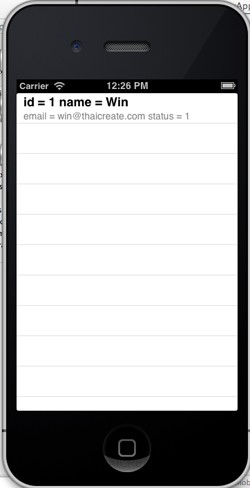
ในไฟล์ ViewController.h และ ViewController.m เขียน Code ดังนี้
ViewController.h
//
// ViewController.h
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UITableView *myTable;
}
@end
ViewController.m
//
// ViewController.m
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import "MyMemberService.h"
#import "ViewController.h"
@interface ViewController ()
// Private properties
@property (strong, nonatomic) MyMemberService *memberService;
@end
@implementation ViewController
@synthesize memberService;
- (void)viewDidLoad
{
[super viewDidLoad];
// Start Service
self.memberService = [MyMemberService defaultService];
[self.memberService refreshDataOnSuccess:^
{
[myTable reloadData];
}];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSDictionary *item = [self.memberService.items objectAtIndex:indexPath.row];
NSString *col1 =[NSString stringWithFormat:@"id = %@ name = %@",
[item objectForKey:@"id"],
[item objectForKey:@"name"]];
cell.textLabel.text = col1;
NSString *col2 =[NSString stringWithFormat:@"email = %@ status = %@",
[item objectForKey:@"email"],
[item objectForKey:@"status"]];
cell.detailTextLabel.text= col2;
return cell;
}
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Always a single section
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of items in the todoService items array
return [self.memberService.items count];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[myTable release];
[super dealloc];
}
@end
อันนี้ Code ในไฟล์ MyMemberService.h และ MyMemberService.m
MyMemberService.h
//
// MyMemberService.h
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import <Foundation/Foundation.h>
typedef void (^QSCompletionBlock) ();
typedef void (^QSBusyUpdateBlock) (BOOL busy);
@interface MyMemberService : NSObject
@property (nonatomic, strong) NSArray *items;
@property (nonatomic, strong) MSClient *client;
@property (nonatomic, copy) QSBusyUpdateBlock busyUpdate;
+ (MyMemberService *)defaultService;
- (void)refreshDataOnSuccess:(QSCompletionBlock)completion;
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response;
@end
MyMemberService.m
//
// MyMemberService.m
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import "MyMemberService.h"
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
@interface MyMemberService() <MSFilter>
@property (nonatomic, strong) MSTable *table;
@property (nonatomic) NSInteger busyCount;
@end
@implementation MyMemberService
@synthesize items;
+ (MyMemberService *)defaultService
{
// Create a singleton instance of MyMemberService
static MyMemberService* service;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
service = [[MyMemberService alloc] init];
});
return service;
}
-(MyMemberService *)init
{
self = [super init];
if (self)
{
// Initialize the Mobile Service client with your URL and key
MSClient *client = [MSClient clientWithApplicationURLString:@"https://thaicreate.azure-mobile.net/"
applicationKey:@"uJPAhkAkcTBuTyCNxaOSnDKFzkoYqB49"];
// Add a Mobile Service filter to enable the busy indicator
self.client = [client clientWithFilter:self];
// Create an MSTable instance to allow us to work with the TodoItem table
self.table = [_client tableWithName:@"MyMember"];
self.items = [[NSMutableArray alloc] init];
self.busyCount = 0;
}
return self;
}
- (void)refreshDataOnSuccess:(QSCompletionBlock)completion
{
// Create a predicate that finds items where complete is false
NSPredicate * predicate = [NSPredicate predicateWithFormat:@"id == '1'"];
// Query the TodoItem table and update the items property with the results from the service
[self.table readWithPredicate:predicate completion:^(NSArray *results, NSInteger totalCount, NSError *error)
{
[self logErrorIfNotNil:error];
items = [results mutableCopy];
// Let the caller know that we finished
completion();
}];
}
- (void)busy:(BOOL)busy
{
// assumes always executes on UI thread
if (busy)
{
if (self.busyCount == 0 && self.busyUpdate != nil)
{
self.busyUpdate(YES);
}
self.busyCount ++;
}
else
{
if (self.busyCount == 1 && self.busyUpdate != nil)
{
self.busyUpdate(FALSE);
}
self.busyCount--;
}
}
- (void)logErrorIfNotNil:(NSError *) error
{
if (error)
{
NSLog(@"ERROR %@", error);
}
}
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response
{
// A wrapped response block that decrements the busy counter
MSFilterResponseBlock wrappedResponse = ^(NSHTTPURLResponse *innerResponse, NSData *data, NSError *error)
{
[self busy:NO];
response(innerResponse, data, error);
};
// Increment the busy counter before sending the request
[self busy:YES];
next(request, wrappedResponse);
}
@end
Download Code ทั้งหมดได้จากท้ายของบทความ
บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|