ตอนที่ 10 : การทำ Validate และ Modify data in Mobile Services บน iOS / iPhone |
ตอนที่ 10 : การทำ Validate และ Modify data in Mobile Services บน iOS / iPhone การเขียน Script เป็นความสามารถของ Mobile Services ที่จะช่วยให้เราเขียนรูปแบบการทำงานและคำสั่งต่าง ๆ ได้ (คล้ายกับการเขียน Stored Procedure บน SQL Server) เช่น เราสามารถตรวจสอบความถูกต้องของข้อมูลก่อนการ Insert ลงใน Table และในกรณีที่ข้อมูลผิดพลาดก็สามารถที่จะแจ้งสถานะกลับไปยัง iOS ได้ หรือจะเขียนพวกเหตุการณ์อื่น ๆ บน Script ก็ได้เช่นเดียวกัน เช่นการ Query ข้อมูลมาตรวจสอบก่อนการ Insert และการตรวจสอบข้อมูลอื่น ๆ ที่เกี่ยวข้อง
ในตัวอย่างนี้เราจะออกแบบ Mobile Services เพื่อรับ Input Data จาก iOS โดยมีเงื่อนไขว่า iOS จะส่งข้อมูลที่เป็น name และ email โดย name จะต้องมีความยาวไม่เกิน 5 ตัวอักษร ถ้าเกินนี้จะส่งค่า Error แจ้งกลับไปยัง iOS

ในตอนนี้เรามี Mobile Services อยู่แล้ว 1 รายการ
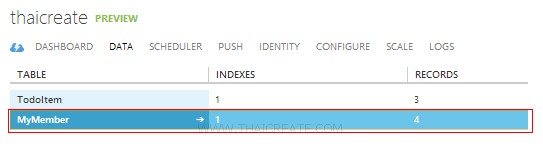
Table ตารางชื่อว่า MyMember ซึ่งอยู่รายการอยู่ 4 record
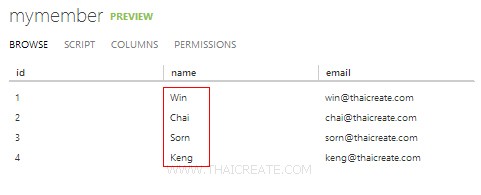
ในส่วนของ Script ที่เป็นคำสั่ง insert()
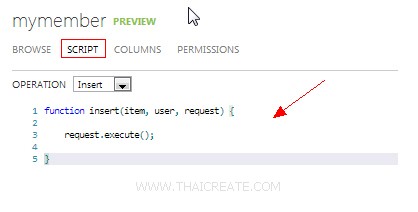
ให้เราเพิ่มเงื่อนไขนี้ลงไป โดยจะเป็นการตรวจสอบ name จะต้องไม่เกิน 5 ตัวอักษร
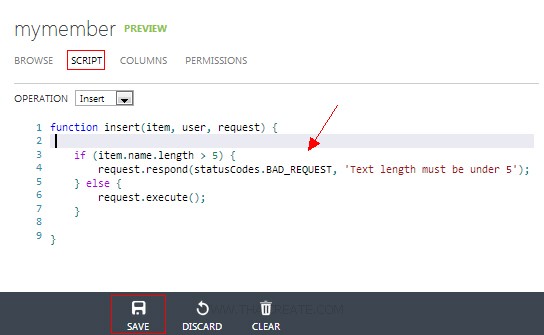
function insert(item, user, request) {
if (item.name.length > 5) {
request.respond(statusCodes.BAD_REQUEST, 'Text length must be under 5');
} else {
request.execute();
}
}
ถ้าเกิน 5 ตัวอักษรจะให้ return คำว่า "Text length must be under 5" กลับไป
if (error && error.code == MSErrorMessageErrorCode) {
NSLog(@"ERROR %@", error);
UIAlertView *av =
[[UIAlertView alloc]
initWithTitle:@"Request Failed"
message:error.localizedDescription
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil
];
[av show];
เป็นคำสั่ง สำหรับการอ่านค่า Error ที่ส่งมาจาก Script บน iOS

กลับมายัง Project ของ iOS บน Xcode
สำหรับพื้นฐานการสร้าง Project และการสร้าง Table รวมทั้งการติดต่อระหว่าง iOS กับ Mobile Services บน Windows Azure แนะนำให้อ่านบทความในตอนที่ 5
ตอนที่ 5: iOS / iPhone สร้างตาราง Table บน Mobile Services และการ Insert ข้อมูล
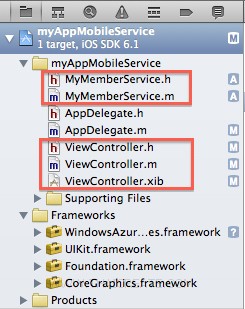
โครงสร้างไฟล์ของ Project บน Xcode
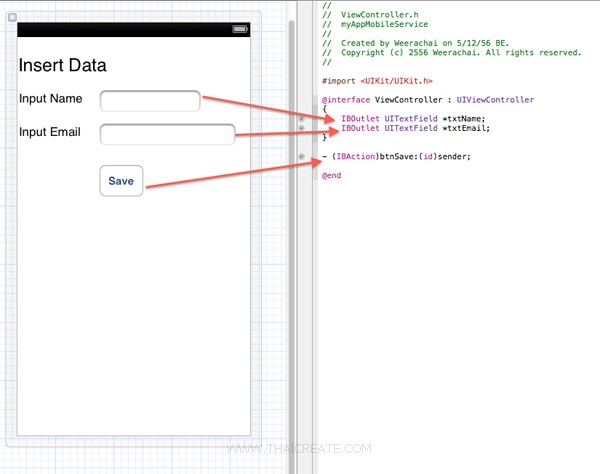
สร้าง Input ง่าย ๆ ขึ้นมา 2 ตัว ทำการเชื่อม IBOutlet ให้เรียบร้อย
Class สำรหับการ Insert ข้อมูลและการแสดง Error
-(void)addItem:(NSDictionary *)item completion:(QSCompletionBlock)completion
{
// Insert the item into the TodoItem table and add to the items array on completion
[self.table insert:item completion:^(NSDictionary *result, NSError *error)
{
[self logErrorIfNotNil:error];
BOOL goodRequest = !((error) && (error.code == MSErrorMessageErrorCode));
// detect text validation error from service.
if (goodRequest) // The service responded appropriately
{
NSUInteger index = [items count];
[(NSMutableArray *)items insertObject:result atIndex:index];
// Let the caller know that we finished
completion(0);
}
else{
// if there's an error that came from the service
// log it, and popup up the returned string.
if (error && error.code == MSErrorMessageErrorCode) {
NSLog(@"ERROR %@", error);
UIAlertView *av =
[[UIAlertView alloc]
initWithTitle:@"Request Failed"
message:error.localizedDescription
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil
];
[av show];
}
}
}];
}
เรียกใช้งาน Class และการ Insert ข้อมูล
- (IBAction)btnSave:(id)sender {
// Add Data Table Service
NSDictionary *item = @{ @"name": txtName.text, @"email": txtEmail.text };
[self.memberService addItem:item completion:^
{
UIAlertView *av =
[[UIAlertView alloc]
initWithTitle:@"Request Suscess"
message:@"Insert Data Successfully."
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil
];
[av show];
}];
}
Screenshot
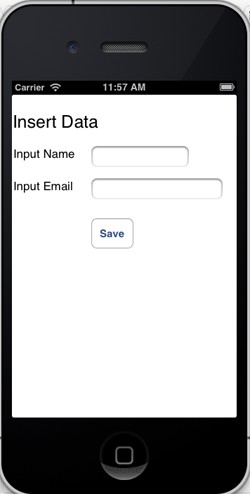
แสดงหน้าจอ App
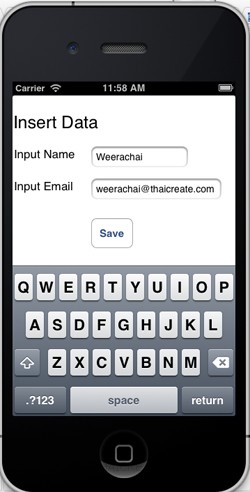
ทำการ Input ข้อมูล name ที่มี Length มากกว่า 5
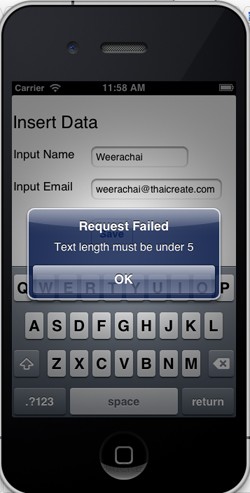
แสดง Error กลับมา
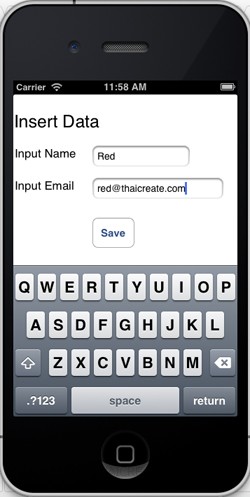
ลองเปลี่ยนเป็นข้อมูลที่ถูกต้อง
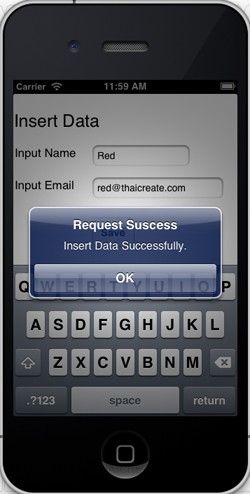
Insert ผ่าน
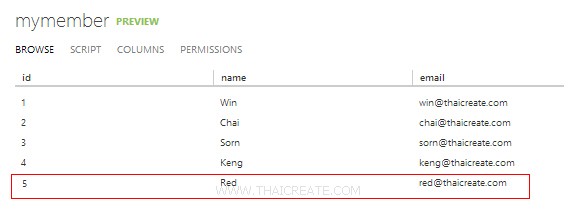
เมื่อกลับไปดูที่ Mobile Serveries บน Portal Management ข้อมูลก็จะถูก Insert เข้าเรียบร้อยแล้ว
ในไฟล์ ViewController.h และ ViewController.m เขียน Code ดังนี้
ViewController.h
//
// ViewController.h
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UITextField *txtName;
IBOutlet UITextField *txtEmail;
}
- (IBAction)btnSave:(id)sender;
@end
ViewController.m
//
// ViewController.m
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import "MyMemberService.h"
#import "ViewController.h"
@interface ViewController ()
// Private properties
@property (strong, nonatomic) MyMemberService *memberService;
@end
@implementation ViewController
@synthesize memberService;
- (void)viewDidLoad
{
[super viewDidLoad];
// Start Service
self.memberService = [MyMemberService defaultService];
}
- (IBAction)btnSave:(id)sender {
// Add Data Table Service
NSDictionary *item = @{ @"name": txtName.text, @"email": txtEmail.text };
[self.memberService addItem:item completion:^
{
UIAlertView *av =
[[UIAlertView alloc]
initWithTitle:@"Request Suscess"
message:@"Insert Data Successfully."
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil
];
[av show];
}];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[txtName release];
[txtEmail release];
[super dealloc];
}
@end
อันนี้ Code ในไฟล์ MyMemberService.h และ MyMemberService.m
MyMemberService.h
//
// MyMemberService.h
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import <Foundation/Foundation.h>
typedef void (^QSCompletionBlock) ();
typedef void (^QSBusyUpdateBlock) (BOOL busy);
@interface MyMemberService : NSObject
@property (nonatomic, strong) NSArray *items;
@property (nonatomic, strong) MSClient *client;
@property (nonatomic, copy) QSBusyUpdateBlock busyUpdate;
+ (MyMemberService *)defaultService;
- (void)addItem:(NSDictionary *)item
completion:(QSCompletionBlock)completion;
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response;
@end
MyMemberService.m
//
// MyMemberService.m
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import "MyMemberService.h"
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
@interface MyMemberService() <MSFilter>
@property (nonatomic, strong) MSTable *table;
@property (nonatomic) NSInteger busyCount;
@end
@implementation MyMemberService
@synthesize items;
+ (MyMemberService *)defaultService
{
// Create a singleton instance of MyMemberService
static MyMemberService* service;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
service = [[MyMemberService alloc] init];
});
return service;
}
-(MyMemberService *)init
{
self = [super init];
if (self)
{
// Initialize the Mobile Service client with your URL and key
MSClient *client = [MSClient clientWithApplicationURLString:@"https://thaicreate.azure-mobile.net/"
applicationKey:@"uJPAhkAkcTBuTyCNxaOSnDKFzkoYqB49"];
// Add a Mobile Service filter to enable the busy indicator
self.client = [client clientWithFilter:self];
// Create an MSTable instance to allow us to work with the TodoItem table
self.table = [_client tableWithName:@"MyMember"];
self.items = [[NSMutableArray alloc] init];
self.busyCount = 0;
}
return self;
}
-(void)addItem:(NSDictionary *)item completion:(QSCompletionBlock)completion
{
// Insert the item into the TodoItem table and add to the items array on completion
[self.table insert:item completion:^(NSDictionary *result, NSError *error)
{
[self logErrorIfNotNil:error];
BOOL goodRequest = !((error) && (error.code == MSErrorMessageErrorCode));
// detect text validation error from service.
if (goodRequest) // The service responded appropriately
{
NSUInteger index = [items count];
[(NSMutableArray *)items insertObject:result atIndex:index];
// Let the caller know that we finished
completion(0);
}
else{
// if there's an error that came from the service
// log it, and popup up the returned string.
if (error && error.code == MSErrorMessageErrorCode) {
NSLog(@"ERROR %@", error);
UIAlertView *av =
[[UIAlertView alloc]
initWithTitle:@"Request Failed"
message:error.localizedDescription
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil
];
[av show];
}
}
}];
}
- (void)busy:(BOOL)busy
{
// assumes always executes on UI thread
if (busy)
{
if (self.busyCount == 0 && self.busyUpdate != nil)
{
self.busyUpdate(YES);
}
self.busyCount ++;
}
else
{
if (self.busyCount == 1 && self.busyUpdate != nil)
{
self.busyUpdate(FALSE);
}
self.busyCount--;
}
}
- (void)logErrorIfNotNil:(NSError *) error
{
if (error)
{
NSLog(@"ERROR %@", error);
}
}
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response
{
// A wrapped response block that decrements the busy counter
MSFilterResponseBlock wrappedResponse = ^(NSHTTPURLResponse *innerResponse, NSData *data, NSError *error)
{
[self busy:NO];
response(innerResponse, data, error);
};
// Increment the busy counter before sending the request
[self busy:YES];
next(request, wrappedResponse);
}
@end

บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2013-06-15 19:51:29 /
2017-03-24 11:51:56 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|