ตอนที่ 11 : สร้าง Refine Mobile Services queries with paging บน Windows Phone |
ตอนที่ 11 : สร้าง Refine Mobile Services queries with paging บน Windows Phone การทำ Refine queries paging เป็นรูปแบบการเขียน Query เพื่อสร้างเงื่อนไขการแสดงข้อมูล Data จาก Mobile Services (คล้าย ๆ กับการเขียน Query เช่น Where , Top , Limit , Order By) โดยเราสามารถทำได้ทั้งระดับ Script ที่อยู่บน Mobile Services หรือจะเขียนเงื่อนไขภายใต้คำสั่ง Where() ที่อยู่บนคำสั่งของ Windows Phone ก็ได้เช่นเดียวกัน และรูปแบบการใช้งานคำสั่ง Script ก็สามารถเข้าใจได้ง่าย ๆ เช่น
private async void RefreshTodoItems()
{
// Define a filtered query that returns the top 3 items.
IMobileServiceTableQuery<TodoItem> query = todoTable
.Where(todoItem => todoItem.Complete == false)
.Take(3);
items = await query.ToCollectionAsync();
ListItems.ItemsSource = items;
}
จาก Code จะเหมือนกับ
SELECT TOP 3 * FROM table_name WHERE complete = 'false'
private async void RefreshTodoItems()
{
// Define a filtered query that skips the first 3 items and
// then returns the next 3 items.
IMobileServiceTableQuery<TodoItem> query = todoTable
.Where(todoItem => todoItem.Complete == false)
.Skip(3)
.Take(3);
items = await query.ToCollectionAsync();
ListItems.ItemsSource = items;
}
จากตัวอย่างจะเป็นการเลือก complete = false และ ไม่เอา 3 รายการแรก แต่เอาแค่ 3 รายการถัดไปที่พบ
Example ตัวอย่างการเขียน Refine queries แบบง่าย ๆ บน Windows Phone

ตอนนี้เรามี Mobile Services อยู่ 1 รายการ ที่ได้สร้างไว้ก่อนหน้านี้
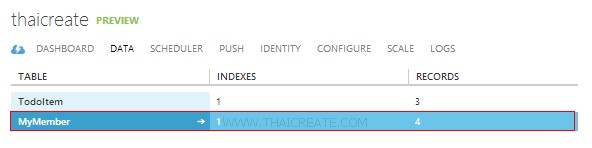
ชื่อตารางว่า MyMember มีข้อมุลอยู่ 4 รายการ
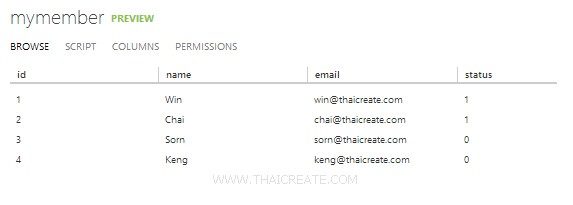
รายการข้อมูลในตาราง MyMember

กลับมายัง Project ของ Windows Phone บน Visual Studio
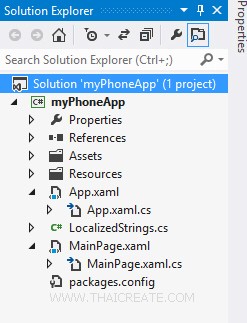
โครงสร้างไฟล์
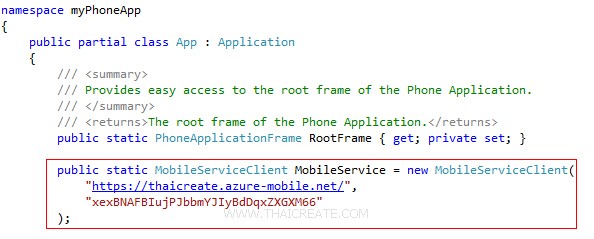
ในไฟล์ App.xaml.cs ให้ Copy Url และ key มาวางดังรูป จากนั้นออกแบบ Layout และเขียน Code ดังนี้
MainPage.xaml
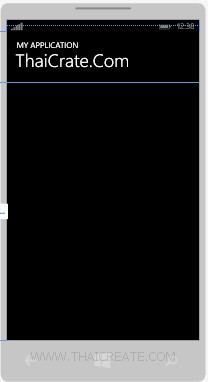
<phone:PhoneApplicationPage
x:Class="myPhoneApp.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:phone="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone"
xmlns:shell="clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
FontFamily="{StaticResource PhoneFontFamilyNormal}"
FontSize="{StaticResource PhoneFontSizeNormal}"
Foreground="{StaticResource PhoneForegroundBrush}"
SupportedOrientations="Portrait" Orientation="Portrait"
shell:SystemTray.IsVisible="True">
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock Text="MY APPLICATION" Style="{StaticResource PhoneTextNormalStyle}" Margin="12,0"/>
<TextBlock Text="ThaiCrate.Com" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}" FontSize="45"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<phone:LongListSelector Grid.Row="4" Grid.ColumnSpan="2" Name="myListItems">
<phone:LongListSelector.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="50">
<TextBlock Text="{Binding Id}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
</StackPanel>
<StackPanel Width="80">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
</StackPanel>
<StackPanel Width="250">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
</StackPanel>
<StackPanel Width="50">
<TextBlock Text="{Binding Status}" TextWrapping="Wrap" Margin="5,0,0,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</phone:LongListSelector.ItemTemplate>
</phone:LongListSelector>
</Grid>
</Grid>
</phone:PhoneApplicationPage>
MainPage.xaml,xml
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Navigation;
using Microsoft.Phone.Controls;
using Microsoft.Phone.Shell;
using myPhoneApp.Resources;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace myPhoneApp
{
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
[JsonProperty(PropertyName = "status")]
public string Status { get; set; }
}
public partial class MainPage : PhoneApplicationPage
{
private MobileServiceCollection<MyMember, MyMember> items;
private IMobileServiceTable<MyMember> memberTable = App.MobileService.GetTable<MyMember>();
// Constructor
public MainPage()
{
InitializeComponent();
}
private async void RefreshMemberItems()
{
try
{
items = await memberTable
.Where(memberItem => memberItem.Status == "0"
&& memberItem.Id == 4)
.OrderByDescending(memberItem => memberItem.Id)
.ToCollectionAsync();
}
catch (MobileServiceInvalidOperationException e)
{
MessageBox.Show(e.Message, "Error loading items", MessageBoxButton.OK);
}
myListItems.ItemsSource = items;
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
RefreshMemberItems();
}
}
}
Ex 1 : เขียน Query เพื่อเอา 3 ข้อมูลแรก
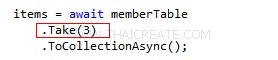
Screenshot
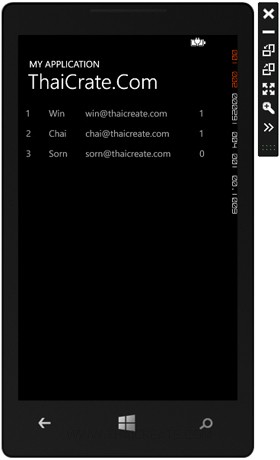
Ex 2 : เขียน Query ข้ามรายการแรก และเอาข้อมูลรายการถัดไป 2 ราบการ
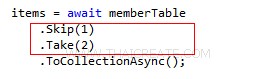
Screenshot
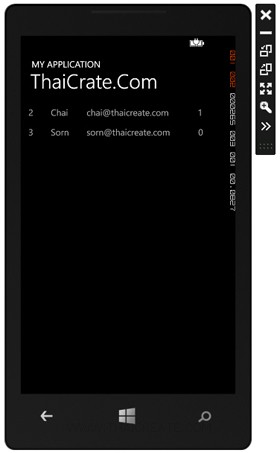
Ex 3 : เขียน Query เลือก Status = '0' และจัดเรียงด้วย Id แบบ Desc (มากไปหาน้อย)

Screenshot
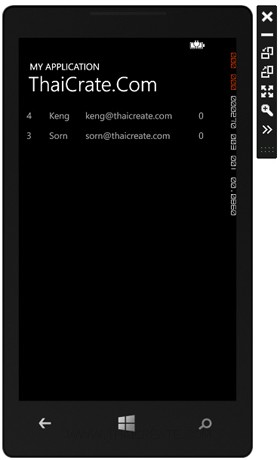
Ex 4 : เขียน Query เลือก Status = '0' และ Id = '4'
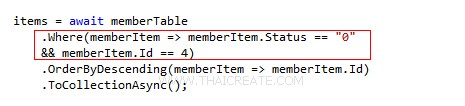
Screenshot
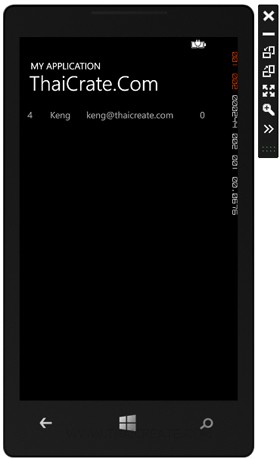
สำหรับการเขียนและใช้งาน Scripts อยากให้ลองเข้าไปศึกษาในบทความนี้ครับ
สามารถทำการดาวน์โหลด Code ทั้งหมดได้จากท้ายบทความ
บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|