ตอนที่ 10 : Validate และ Modify data in Mobile Services บน Windows Phone |
ตอนที่ 10 : Validate และ Modify data in Mobile Services บน Windows Phone การเขียน Script เป็นความสามารถของ Mobile Services ที่จะช่วยให้เราเขียนรูปแบบการทำงานและคำสั่งต่าง ๆ ได้ (คล้ายกับการเขียน Stored Procedure บน SQL Server) เช่น เราสามารถตรวจสอบความถูกต้องของข้อมูลก่อนการ Insert ลงใน Table และในกรณีที่ข้อมูลผิดพลาดก็สามารถที่จะแจ้งสถานะกลับไปยัง Windows Phone ได้ หรือจะเขียนพวกเหตุการณ์อื่น ๆ บน Script ก็ได้เช่นเดียวกัน เช่นการ Query ข้อมูลมาตรวจสอบก่อนการ Insert และการตรวจสอบข้อมูลอื่น ๆ ที่เกี่ยวข้อง
ในตัวอย่างนี้เราจะออกแบบ Mobile Services เพื่อรับ Input Data จาก Windows Phone โดยมีเงื่อนไขว่า Windows Phone จะส่งข้อมูลที่เป็น name และ email โดย name จะต้องมีความยาวไม่เกิน 5 ตัวอักษร ถ้าเกินนี้จะส่งค่า Error แจ้งกลับไปยัง Windows Phone

ในตอนนี้เรามี Mobile Services อยู่แล้ว 1 รายการ
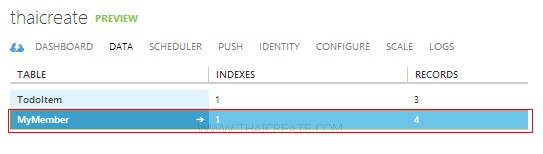
Table ตารางชื่อว่า MyMember ซึ่งอยู่รายการอยู่ 4 record
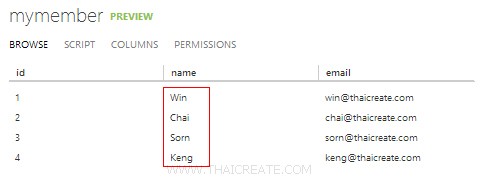
ในส่วนของ Script ที่เป็นคำสั่ง insert()
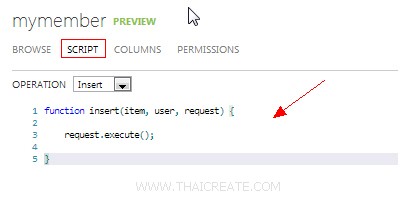
ให้เราเพิ่มเงื่อนไขนี้ลงไป โดยจะเป็นการตรวจสอบ name จะต้องไม่เกิน 5 ตัวอักษร
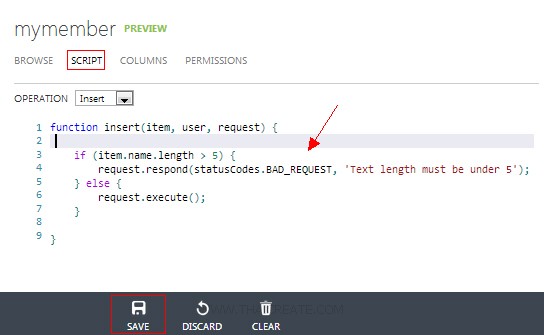
function insert(item, user, request) {
if (item.name.length > 5) {
request.respond(statusCodes.BAD_REQUEST, 'Text length must be under 5');
} else {
request.execute();
}
}
ถ้าเกิน 5 ตัวอักษรจะให้ return คำว่า "Text length must be under 5" กลับไป
ex.Message()
เป็นคำสั่ง สำหรับการอ่านค่า Error ที่ส่งมาจาก Script บน Windows Phone

กลับมายัง Project ของ Windows Phone บน Visual Studio
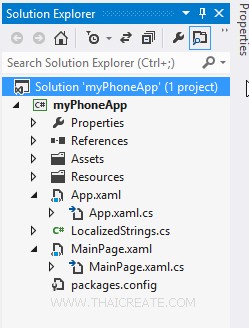
ในไฟล์ App.xaml.cs ให้ทำการสร้าง Url และ Key ที่ได้จาก Mobile Services
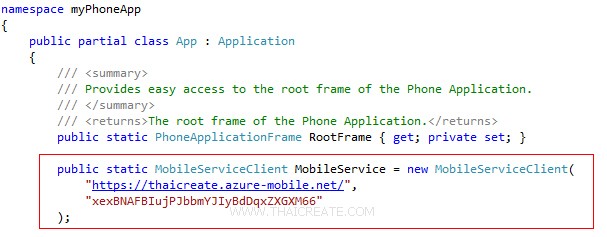
เพิ่ม Url และ Key ลงไปในไฟล์ App.xaml.cs จานั้นออกแบบ Layout และเขียน Code ดังนี้
MainPage.xaml
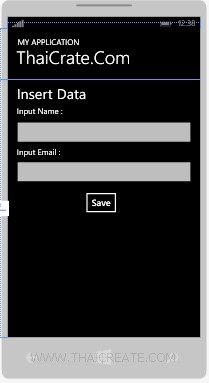
<phone:PhoneApplicationPage
x:Class="myPhoneApp.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:phone="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone"
xmlns:shell="clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
FontFamily="{StaticResource PhoneFontFamilyNormal}"
FontSize="{StaticResource PhoneFontSizeNormal}"
Foreground="{StaticResource PhoneForegroundBrush}"
SupportedOrientations="Portrait" Orientation="Portrait"
shell:SystemTray.IsVisible="True">
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock Text="MY APPLICATION" Style="{StaticResource PhoneTextNormalStyle}" Margin="12,0"/>
<TextBlock Text="ThaiCrate.Com" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}" FontSize="45"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<TextBlock HorizontalAlignment="Left" Margin="10,10,0,0" TextWrapping="Wrap" Text="Insert Data" VerticalAlignment="Top" FontSize="36"/>
<TextBlock HorizontalAlignment="Left" Margin="10,63,0,0" TextWrapping="Wrap" Text="Input Name :" VerticalAlignment="Top"/>
<TextBox x:Name="txtName" HorizontalAlignment="Left" Height="72" Margin="0,95,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="456"/>
<TextBlock HorizontalAlignment="Left" Margin="10,167,0,0" TextWrapping="Wrap" Text="Input Email :" VerticalAlignment="Top"/>
<TextBox x:Name="txtEmail" HorizontalAlignment="Left" Height="72" Margin="0,194,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="456"/>
<Button x:Name="btnSave" Content="Save" HorizontalAlignment="Left" Margin="172,271,0,0" VerticalAlignment="Top" Click="btnSave_Click"/>
</Grid>
</Grid>
</phone:PhoneApplicationPage>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Navigation;
using Microsoft.Phone.Controls;
using Microsoft.Phone.Shell;
using myPhoneApp.Resources;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace myPhoneApp
{
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
}
public partial class MainPage : PhoneApplicationPage
{
private MobileServiceCollection<MyMember, MyMember> items;
private IMobileServiceTable<MyMember> memberTable = App.MobileService.GetTable<MyMember>();
// Constructor
public MainPage()
{
InitializeComponent();
}
private async void btnSave_Click(object sender, RoutedEventArgs e)
{
try
{
items = await memberTable.ToCollectionAsync();
var insertItem = new MyMember { Name = txtName.Text
, Email = txtEmail.Text };
await memberTable.InsertAsync(insertItem);
items.Add(insertItem);
MessageBox.Show("Insert Data Successfully.");
}
catch (MobileServiceInvalidOperationException ex)
{
MessageBox.Show("Insert Data Failed! Error " + ex.Message);
}
}
}
}
Screenshot
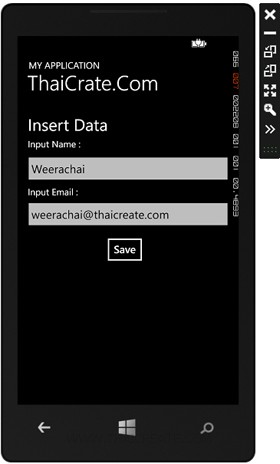
ทำการ Input ข้อมูล name ที่มี Length มากกว่า 5
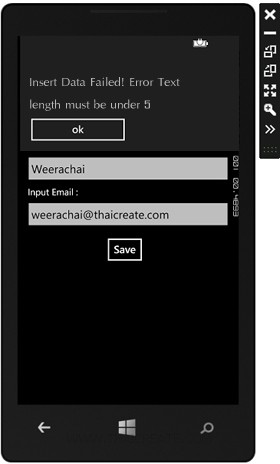
แสดง Error กลับมา
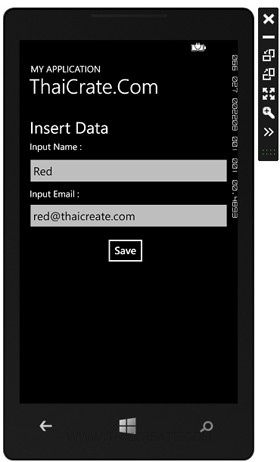
ลองเปลี่ยนเป็นข้อมูลที่ถูกต้อง
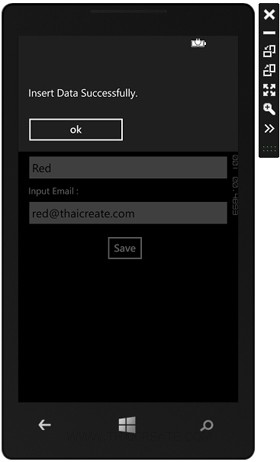
Insert ผ่าน
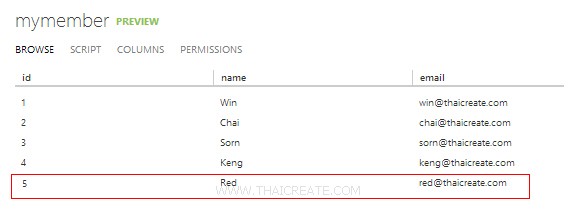
เมื่อกลับไปดูที่ Mobile Serveries บน Portal Management ข้อมูลก็จะถูก Insert เข้าเรียบร้อยแล้ว
บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|