ตอนที่ 5 : How to use .Net (Asp.net) List the Blobs การแสดงรายการไฟล์จาก Blob |
ตอนที่ 5 : How to use .Net (Asp.net) List the Blobs การแสดงรายการไฟล์จาก Blob บทความนี้จะเป็นหัวข้อเกี่ยวกับการใช้ .NET (ASP.Net) เพื่ออ่านค่าจาก Blob Storage ของ Windows Azure และหลังจากที่ได้รายการ Blob ที่ถูกส่งมาจาก Storage และอยู่ในรูปแบบของ Array เราจะเอาค่าที่ได้มาแสดงรายการในหน้า Web และในกรณีที่ Blob เป็น Image เราก็สามารถที่จะแสดงภาพเหล่านั้นได้ทันที
ข้อมูลที่อยู่ใน Blob ถูกจัดเก็บไว้ใน Container ชื่อว่า pictures
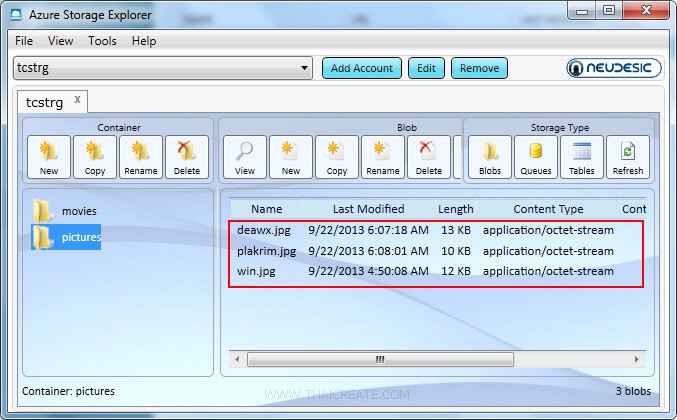
Syntax การอ่านรายการไฟล์ใน Blob
// Create the blob client.
CloudBlobClient blobClient = storageAccount.CreateCloudBlobClient();
// Retrieve reference to a previously created container.
CloudBlobContainer container = blobClient.GetContainerReference("photos");
// Loop over items within the container and output the length and URI.
foreach (IListBlobItem item in container.ListBlobs(null, false))
{
if (item.GetType() == typeof(CloudBlockBlob))
{
CloudBlockBlob blob = (CloudBlockBlob)item;
Console.WriteLine("Block blob of length {0}: {1}", blob.Properties.Length, blob.Uri);
}
else if (item.GetType() == typeof(CloudPageBlob))
{
CloudPageBlob pageBlob = (CloudPageBlob)item;
Console.WriteLine("Page blob of length {0}: {1}", pageBlob.Properties.Length, pageBlob.Uri);
}
else if (item.GetType() == typeof(CloudBlobDirectory))
{
CloudBlobDirectory directory = (CloudBlobDirectory)item;
Console.WriteLine("Directory: {0}", directory.Uri);
}
}
กรณีที่ดูผ่าน Azure Storage Explorer
Example 1 การใช้ .NET (ASP.Net) เพื่ออ่านรายการไฟล์ที่อยู่ใน Blob
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using Microsoft.WindowsAzure.Storage;
using Microsoft.WindowsAzure.Storage.Auth;
using Microsoft.WindowsAzure.Storage.Blob;
using System.IO;
namespace myWebApp
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
// Create the connectionstring
String StorageConnectionString = "DefaultEndpointsProtocol=https;AccountName=[yourAccount];AccountKey=[yourKey]";
// Retrieve storage account from connection string.
CloudStorageAccount storageAccount = CloudStorageAccount.Parse(StorageConnectionString);
// Create the blob client.
CloudBlobClient blobClient = storageAccount.CreateCloudBlobClient();
// Retrieve reference to a previously created container.
CloudBlobContainer container = blobClient.GetContainerReference("pictures");
// Loop over items within the container and output the length and URI.
foreach (IListBlobItem item in container.ListBlobs(null, false))
{
if (item.GetType() == typeof(CloudBlockBlob))
{
CloudBlockBlob blob = (CloudBlockBlob)item;
Response.Write(blob.Properties.Length + " - " + blob.Uri + "<br>");
}
}
}
}
}
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="myWebApp.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>ThaiCreate.Com Azure Storage Tutorial</title>
</head>
<body>
<form id="form1" runat="server">
<div>
</div>
</form>
</body>
</html>
Screenshot
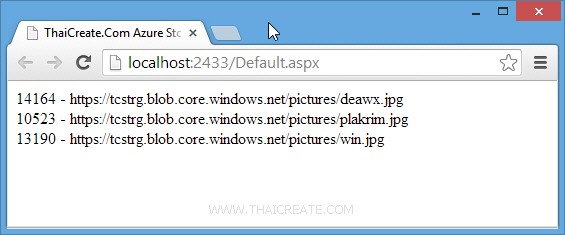
Example 2 การใช้ .NET (ASP.Net) เพื่ออ่านรายการไฟล์ที่อยู่ใน Blob โดยแสดงข้อมูลในรูปแบบของ GridView
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using Microsoft.WindowsAzure.Storage;
using Microsoft.WindowsAzure.Storage.Auth;
using Microsoft.WindowsAzure.Storage.Blob;
using System.IO;
namespace myWebApp
{
public partial class Default : System.Web.UI.Page
{
public class Member
{
public string URL { set; get; }
public string Name { set; get; }
}
protected void Page_Load(object sender, EventArgs e)
{
// Create the connectionstring
String StorageConnectionString = "DefaultEndpointsProtocol=https;AccountName=[yourAccount];AccountKey=[yourKey]";
// Retrieve storage account from connection string.
CloudStorageAccount storageAccount = CloudStorageAccount.Parse(StorageConnectionString);
// Create the blob client.
CloudBlobClient blobClient = storageAccount.CreateCloudBlobClient();
// Retrieve reference to a previously created container.
CloudBlobContainer container = blobClient.GetContainerReference("pictures");
// Create List
List<Member> ls = new List<Member>();
// Loop over items within the container and output the length and URI.
foreach (IListBlobItem item in container.ListBlobs(null, false))
{
if (item.GetType() == typeof(CloudBlockBlob))
{
CloudBlockBlob blob = (CloudBlockBlob)item;
ls.Add(new Member { URL = blob.Uri.ToString() , Name = blob.Name });
}
}
// Create data to GridView
this.myGridView.DataSource = ls;
this.myGridView.DataBind();
}
protected void myGridView_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
//*** URL ***//
Label lblURL = (Label)(e.Row.FindControl("lblURL"));
if (lblURL != null)
{
lblURL.Text = DataBinder.Eval(e.Row.DataItem,"URL").ToString();
}
//*** Name ***//
Label lblName = (Label)(e.Row.FindControl("lblName"));
if (lblName != null)
{
lblName.Text = DataBinder.Eval(e.Row.DataItem, "NAME").ToString();
}
//*** View ***//
Image imgView = (Image)(e.Row.FindControl("imgView"));
if (imgView != null)
{
imgView.ImageUrl = DataBinder.Eval(e.Row.DataItem, "URL").ToString();
}
}
}
}
}
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="myWebApp.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>ThaiCreate.Com Azure Storage Tutorial</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="myGridView" runat="server"
AutoGenerateColumns="False"
OnRowDataBound="myGridView_RowDataBound" Width="600px">
<Columns>
<asp:TemplateField HeaderText="URL">
<ItemTemplate>
<asp:Label ID="lblURL" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label ID="lblName" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="View">
<ItemTemplate>
<asp:Image ID="imgView" runat="server" />
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" />
</asp:TemplateField>
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
Screenshot
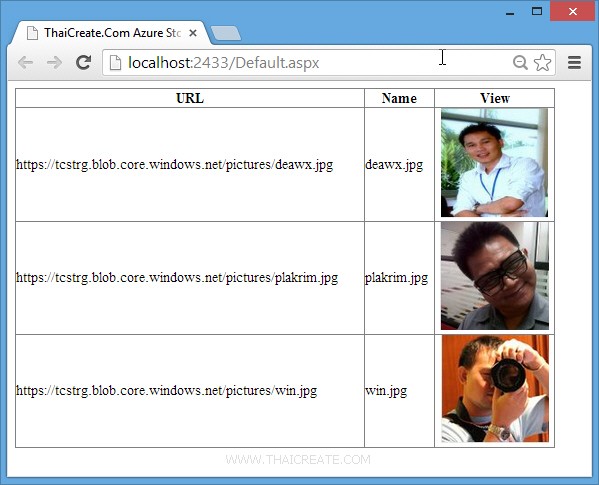
บทความที่เกี่ยวข้อง
บทความถัดไปที่แนะนำให้อ่าน
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-12-29 20:06:30 /
2017-03-24 15:12:57 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|