ตอนที่ 8 : How to use .Net (Asp.net) Add Entity to a Table Storage - บันทึกข้อมูลลงตาราง |
ตอนที่ 8 : How to use .Net (Asp.net) Add Entity to a Table Storage - บันทึกข้อมูลลงตาราง บทความที่สองของ Windows Azure Table Storage กับ .NET (ASP.Net) จะเป็นตัวอย่างเกี่ยวกับการ Insert ข้อมูลลงใน Table Storage ของ Windows Azure ซึ่งจะเหมือนกับการ Insert ข้อมูลแบบ SQL ปกติ เพียงแต่เราจะใช้การ Insert ผ่าน Library ด้วยการเขียนคำสั่งเพียงง่าย ๆ ก็สามารถที่จะ Insert ลงใน Table ได้ทันที
ตาราง Table ที่อยู่บน Windows Azure
ก่อนอื่นเราจะต้องสร้าง Class สำหรับเป็น Model ในการเชื่อมต่อระหว่า Table Storage กับ .NET ด้วยการสร้าง Class แล้ว Inherit ไปยัง TableEntity
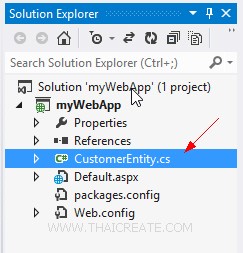
เราจะได้ไฟล์ Class ชื่อว่า CustomerEntity.cs
CustomerEntity.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Microsoft.WindowsAzure.Storage.Table;
namespace myWebApp
{
public class CustomerEntity : TableEntity
{
public CustomerEntity(string pKey, string rKey)
{
this.PartitionKey = pKey;
this.RowKey = rKey;
}
public CustomerEntity() { }
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
}
ใน Table นี้จะประกอบด้วยฟิวด์
- CustomerID
- Name
- Email
- CountryCode
- Budget
- Used
แต่เราจะเห็นว่าจะมีชื่อ partitionKey และ rowKey ตัวนี้เปรียบเสมือน Key ของ Table
Syntax การ Insert ข้อมูล
// Create a new customer entity.
CustomerEntity cus = new CustomerEntity("myCustomer", "C001");
cus.CustomerID = "C001";
cus.Name = "Win Weerachai";
cus.Email = "[email protected]";
cus.CountryCode = "TH";
cus.Budget = "1000000.00";
cus.Used = "600000.00";
จัดเก็บไว้ใน Table ชื่อว่า customer จะสังเกตุว่ามีการ Insert ข้อมูลที่เป็น PartitionKey และ RowKey ซึ่งตัวนี้เปรียบเหสมือน Key ของ Rows ควรเป็นค่าที่ไม่ซ้ำกัน ใช้ในการอ้างอิงถึง Key และ Index ทำให้สามารถอ่านข้อมูลได้อย่างรวดเร็ว

Example 1 เขียน .NET (ASP.Net) เพื่บันทึกข้อมูลลงใน Table Storage ของ Windows Azure
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using Microsoft.WindowsAzure.Storage;
using Microsoft.WindowsAzure.Storage.Auth;
using Microsoft.WindowsAzure.Storage.Table;
namespace myWebApp
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
// Create the connectionstring
String StorageConnectionString = "DefaultEndpointsProtocol=https;AccountName=[yourAccount];AccountKey=[yourKey]";
// Retrieve storage account from connection string.
CloudStorageAccount storageAccount = CloudStorageAccount.Parse(StorageConnectionString);
// Create the table client.
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
// Create the CloudTable object that represents the "customer" table.
CloudTable table = tableClient.GetTableReference("customer");
// Create a new customer entity.
CustomerEntity cus = new CustomerEntity("myCustomer", "C001");
cus.CustomerID = "C001";
cus.Name = "Win Weerachai";
cus.Email = "[email protected]";
cus.CountryCode = "TH";
cus.Budget = "1000000.00";
cus.Used = "600000.00";
// Create the TableOperation that inserts the customer entity.
TableOperation insertOperation = TableOperation.Insert(cus);
// Execute the insert operation.
table.Execute(insertOperation);
this.lblResult.Text = "Storage Table 'customer' has been inserted.";
}
}
}
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="myWebApp.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>ThaiCreate.Com Azure Storage Tutorial</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="lblResult" runat="server"></asp:Label>
</div>
</form>
</body>
</html>
Screenshot
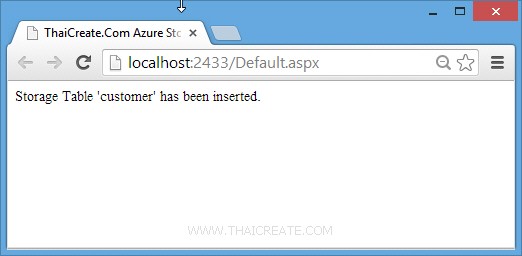
แสดงการ Insert ข้อมูล
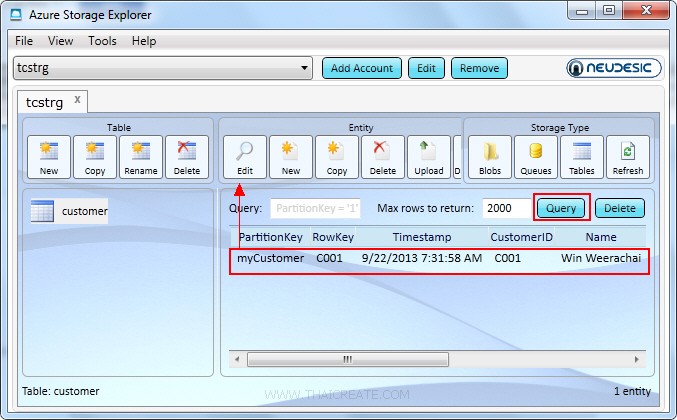
ดูผ่านโปรแกรม Azure Storage Explorer เลือก Edit
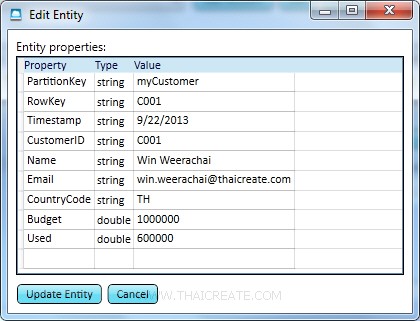
แสดงข้อมูลที่ถูก Insert ลงไปใน Table
Example 2 เขียน .NET (ASP.Net) สร้าง Form สำหรับ Insert ข้อมูลลงใน Azure Table Storage
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using Microsoft.WindowsAzure.Storage;
using Microsoft.WindowsAzure.Storage.Auth;
using Microsoft.WindowsAzure.Storage.Table;
namespace myWebApp
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
BindData();
}
}
protected void BindData()
{
// Create the connectionstring
String StorageConnectionString = "DefaultEndpointsProtocol=https;AccountName=[yourAccount];AccountKey=[yourKey]";
// Retrieve storage account from connection string.
CloudStorageAccount storageAccount = CloudStorageAccount.Parse(StorageConnectionString);
// Create the table client.
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
// Create the CloudTable object that represents the "customer" table.
CloudTable table = tableClient.GetTableReference("customer");
// Construct the query operation for all customer entities where PartitionKey="myCustomer".
TableQuery<CustomerEntity> query = new TableQuery<CustomerEntity>().Where(TableQuery.GenerateFilterCondition("PartitionKey", QueryComparisons.Equal, "myCustomer"));
// Create List Customer
List<CustomerEntity> ls = new List<CustomerEntity>();
// Print the fields for each customer.
foreach (CustomerEntity entity in table.ExecuteQuery(query))
{
// Create a new customer entity.
CustomerEntity cus = new CustomerEntity(entity.PartitionKey, entity.RowKey);
cus.CustomerID = entity.CustomerID;
cus.Name = entity.Name;
cus.Email = entity.Email;
cus.CountryCode = entity.CountryCode;
cus.Budget = entity.Budget;
cus.Used = entity.Used;
ls.Add(cus);
}
// Bind Data to GridView
this.myGridView.DataSource = ls;
this.myGridView.DataBind();
}
protected void myGridView_RowCommand(Object source, GridViewCommandEventArgs e)
{
if (e.CommandName == "Add")
{
//*** CustomerID ***//
TextBox txtCustomerID = (TextBox)myGridView.FooterRow.FindControl("txtAddCustomerID");
//*** Email ***//
TextBox txtName = (TextBox)myGridView.FooterRow.FindControl("txtAddName");
//*** Name ***//
TextBox txtEmail = (TextBox)myGridView.FooterRow.FindControl("txtAddEmail");
//*** CountryCode ***//
TextBox txtCountryCode = (TextBox)myGridView.FooterRow.FindControl("txtAddCountryCode");
//*** Budget ***//
TextBox txtBudget = (TextBox)myGridView.FooterRow.FindControl("txtAddBudget");
//*** Used ***//
TextBox txtUsed = (TextBox)myGridView.FooterRow.FindControl("txtAddUsed");
// Create the connectionstring
String StorageConnectionString = "DefaultEndpointsProtocol=https;AccountName=[yourAccount];AccountKey=[yourKey]";
// Retrieve storage account from connection string.
CloudStorageAccount storageAccount = CloudStorageAccount.Parse(StorageConnectionString);
// Create the table client.
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
// Create the CloudTable object that represents the "customer" table.
CloudTable table = tableClient.GetTableReference("customer");
// Create a new customer entity.
CustomerEntity cus = new CustomerEntity("myCustomer", txtCustomerID.Text);
cus.CustomerID = txtCustomerID.Text;
cus.Name = txtName.Text;
cus.Email = txtEmail.Text;
cus.CountryCode = txtCountryCode.Text;
cus.Budget = txtBudget.Text;
cus.Used = txtUsed.Text;
// Create the TableOperation that inserts the customer entity.
TableOperation insertOperation = TableOperation.Insert(cus);
// Execute the insert operation.
table.Execute(insertOperation);
BindData();
}
}
}
}
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="myWebApp.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>ThaiCreate.Com Azure Storage Tutorial</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView id="myGridView" runat="server" AutoGenerateColumns="False"
ShowFooter="True"
DataKeyNames="CustomerID"
ShowHeaderWhenEmpty ="true"
OnRowCommand="myGridView_RowCommand">
<Columns>
<asp:TemplateField HeaderText="CustomerID">
<ItemTemplate>
<asp:Label id="lblCustomerID" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCustomerID" size="5" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCustomerID" size="5" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label id="lblName" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditName" size="10" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddName" size="10" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Email">
<ItemTemplate>
<asp:Label id="lblEmail" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditEmail" size="20" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddEmail" size="20" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="CountryCode">
<ItemTemplate>
<asp:Label id="lblCountryCode" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCountryCode" size="2" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCountryCode" size="2" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Budget">
<ItemTemplate>
<asp:Label id="lblBudget" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditBudget" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddBudget" size="6" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Used">
<ItemTemplate>
<asp:Label id="lblUsed" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditUsed" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddUsed" size="6" runat="server"></asp:TextBox>
<asp:Button id="btnAdd" runat="server" Text="Add" CommandName="Add"></asp:Button>
</FooterTemplate>
</asp:TemplateField>
<asp:CommandField ShowEditButton="True" CancelText="Cancel" DeleteText="Delete" EditText="Edit" UpdateText="Update" HeaderText="Modify" />
<asp:CommandField ShowDeleteButton="True" HeaderText="Delete" />
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
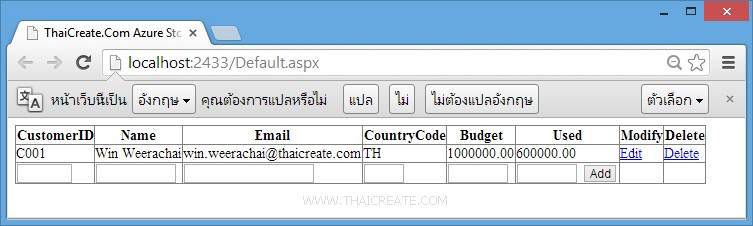
แสดง Form สำหรับ Insert ข้อมูล ซึ่งจะได้ผลลัพธ์เดียวกับตัวอย่างแรก
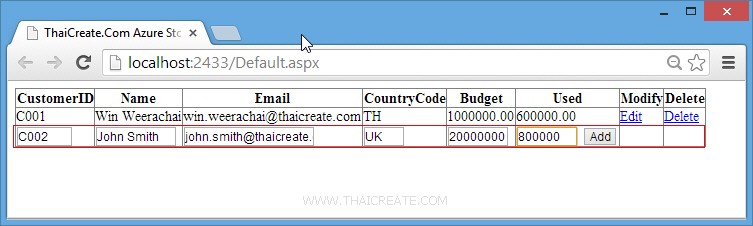
ทดสอบการ Input ข้อมูล
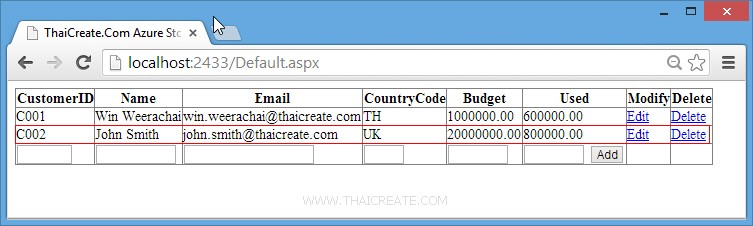
ข้อมูลถูกเพิ่มเรียบร้อย
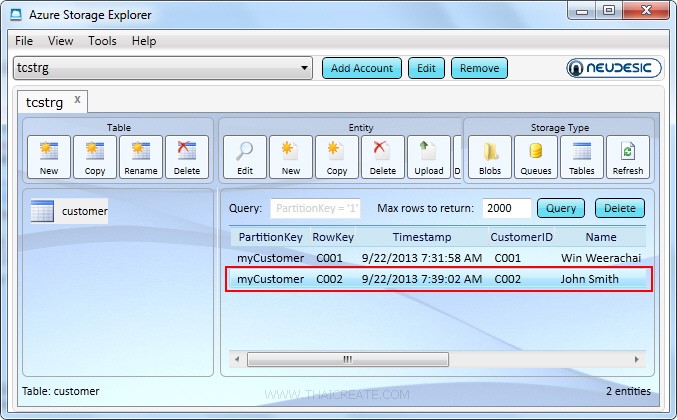
ดูผ่าน Azure Storage Explorer
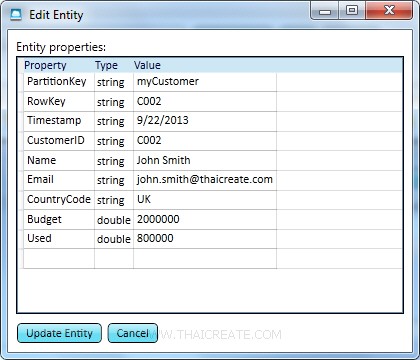
ดูผ่าน Azure Storage Explorer
บทความที่เกี่ยวข้อง
บทความถัดไปที่แนะนำให้อ่าน
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-12-29 20:07:14 /
2017-03-24 15:15:14 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|