ตอนที่ 9 : How to use .Net (Asp.net) Retrieve Entity from Table Storage - อ่านข้อมูลในตาราง |
ตอนที่ 9 : How to use .Net (Asp.net) Retrieve Entity from Table Storage - อ่านข้อมูลในตาราง หัวข้อนี้จะเป็นการเขียน .NET (ASP.Net) เพื่ออ่านข้อมูลจาก Table Storage ของ Windows Azure ด้วยการใช้ Library ของ Windows Azure ซึ่งจะสามารถเขียนเงื่อนไขการแสดงข้อมูลได้เหมือนกับคำสั่งของ SQL คือสามารถทำการ Filter ข้อมูลได้ตามต้องการ และข้อมูลที่ได้นั้นจะอยู่ในรูปแบบของ Array สามารถนำไปใช้งานอื่น ๆ ได้เช่น แสดงผลในหน้าจอของเว็บไซต์
ข้อมูล Table Storage ที่อยู่บน Windows Azure
CustomerEntity.cs เป็น Model Class สำหรับการเชื่อมต่อกับ Table Storage
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Microsoft.WindowsAzure.Storage.Table;
namespace myWebApp
{
public class CustomerEntity : TableEntity
{
public CustomerEntity(string pKey, string rKey)
{
this.PartitionKey = pKey;
this.RowKey = rKey;
}
public CustomerEntity() { }
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
}
Syntax การ อ่านข้อมูล
// Create the table client.
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
// Create the CloudTable object that represents the "people" table.
CloudTable table = tableClient.GetTableReference("people");
// Construct the query operation for all customer entities where PartitionKey="myCustomer".
TableQuery<CustomerEntity> query = new TableQuery<CustomerEntity>().Where(TableQuery.GenerateFilterCondition("PartitionKey", QueryComparisons.Equal, "myCustomer"));
// Print the fields for each customer.
foreach (CustomerEntity entity in table.ExecuteQuery(query))
{
Console.WriteLine("{0}, {1}\t{2}\t{3}", entity.PartitionKey, entity.RowKey,
entity.CustomerID, entity.Name);
}

Example เขียน .NET (ASP.Net) เพื่อแสดงข้อมูลจาก Table Storage ของ Windows Azure
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using Microsoft.WindowsAzure.Storage;
using Microsoft.WindowsAzure.Storage.Auth;
using Microsoft.WindowsAzure.Storage.Table;
namespace myWebApp
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
BindData();
}
}
protected void BindData()
{
// Create the connectionstring
String StorageConnectionString = "DefaultEndpointsProtocol=https;AccountName=[yourAccount];AccountKey=[yourKey]";
// Retrieve storage account from connection string.
CloudStorageAccount storageAccount = CloudStorageAccount.Parse(StorageConnectionString);
// Create the table client.
CloudTableClient tableClient = storageAccount.CreateCloudTableClient();
// Create the CloudTable object that represents the "customer" table.
CloudTable table = tableClient.GetTableReference("customer");
// Construct the query operation for all customer entities where PartitionKey="myCustomer".
TableQuery<CustomerEntity> query = new TableQuery<CustomerEntity>().Where(TableQuery.GenerateFilterCondition("PartitionKey", QueryComparisons.Equal, "myCustomer"));
// Create List Customer
List<CustomerEntity> ls = new List<CustomerEntity>();
// Print the fields for each customer.
foreach (CustomerEntity entity in table.ExecuteQuery(query))
{
// Create a new customer entity.
CustomerEntity cus = new CustomerEntity(entity.PartitionKey, entity.RowKey);
cus.CustomerID = entity.CustomerID;
cus.Name = entity.Name;
cus.Email = entity.Email;
cus.CountryCode = entity.CountryCode;
cus.Budget = entity.Budget;
cus.Used = entity.Used;
ls.Add(cus);
}
// Bind Data to GridView
this.myGridView.DataSource = ls;
this.myGridView.DataBind();
}
}
}
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="myWebApp.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>ThaiCreate.Com Azure Storage Tutorial</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView id="myGridView" runat="server" AutoGenerateColumns="False"
ShowFooter="True"
DataKeyNames="CustomerID"
ShowHeaderWhenEmpty ="true">
<Columns>
<asp:TemplateField HeaderText="CustomerID">
<ItemTemplate>
<asp:Label id="lblCustomerID" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCustomerID" size="5" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCustomerID" size="5" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label id="lblName" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditName" size="10" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddName" size="10" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Email">
<ItemTemplate>
<asp:Label id="lblEmail" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditEmail" size="20" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddEmail" size="20" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="CountryCode">
<ItemTemplate>
<asp:Label id="lblCountryCode" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCountryCode" size="2" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCountryCode" size="2" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Budget">
<ItemTemplate>
<asp:Label id="lblBudget" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditBudget" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddBudget" size="6" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Used">
<ItemTemplate>
<asp:Label id="lblUsed" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditUsed" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddUsed" size="6" runat="server"></asp:TextBox>
<asp:Button id="btnAdd" runat="server" Text="Add" CommandName="Add"></asp:Button>
</FooterTemplate>
</asp:TemplateField>
<asp:CommandField ShowEditButton="True" CancelText="Cancel" DeleteText="Delete" EditText="Edit" UpdateText="Update" HeaderText="Modify" />
<asp:CommandField ShowDeleteButton="True" HeaderText="Delete" />
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
Screenshot
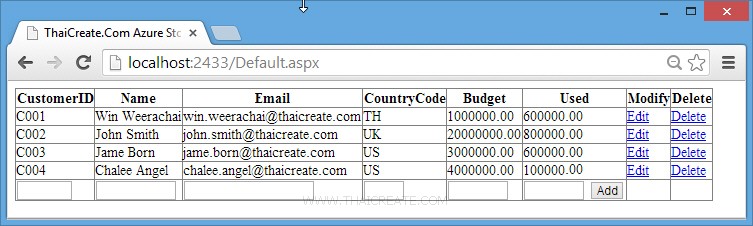
แสดงข้อมูลจาก Table Storage ในรูปแบบของ GridView
บทความที่เกี่ยวข้อง
บทความถัดไปที่แนะนำให้อ่าน
|