ตอนที่ 11 : การเขียน Java Application ร่วมกับ SQL Database บน Windows Azure |
ตอนที่ 11 : การเขียน Java Application ร่วมกับ SQL Database บน Windows Azure ในการเขียนโปรแกรมด้วยภาษา Java Application ไม่ว่าจะเป็น App ที่ทำงานบน Web Browser (JSP) หรือ App ที่ทำงานบน Windows Desktop ทั่ว ๆ ไป ก็สามารถที่จะทำการเชื่อมต่อกับ SQL Database บน Windows Azure ได้เช่นเดียวกัน โดยใน SQL Azure / SQL Database จะรองรับการทำงานตั้งแต่ Java Developer Kit (JDK), v 1.6 เป็นต้นไป และใช้ Microsoft JDBC Driver 4.0 for SQL Server ในการที่จะเป็น Driver สำหรับการเชื่อมต่อระหว่าง Java กับ SQL Database
จากตัวอย่างก่อนหน้านี้เราได้ทำการสร้าง SQL Database บน Windows Azure และทำการเชื่อมต่อพร้อมกับสร้าง Table ขึ้นมา 1 รายการเรียบร้อยแล้ว
ตอนที่ 3 : เชื่อมต่อ SQL Database ผ่าน SQL Server Management Studio (SSMS)
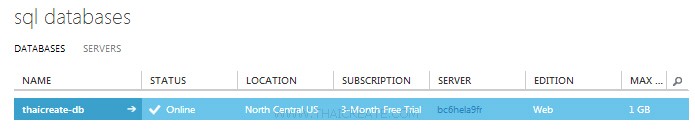
Service ของ SQL Database
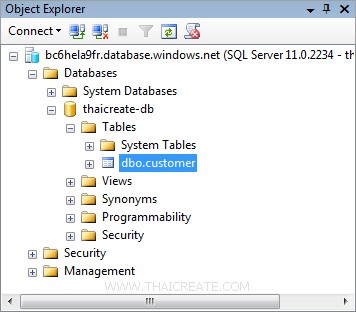
Table ชื่อว่า customer
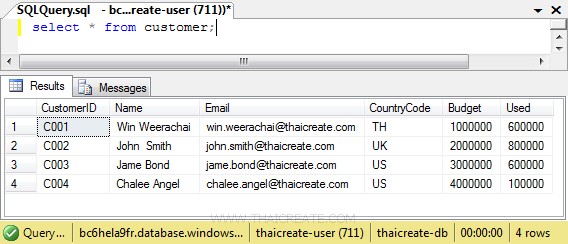
รายการข้อมูลบนตารางของ customer
SQL Database Info
Server Name : bc6hela9fr.database.windows.net
Server : bc6hela9fr
User : thaicreate-user
Password : password@123
Database Name : thaicreate-db
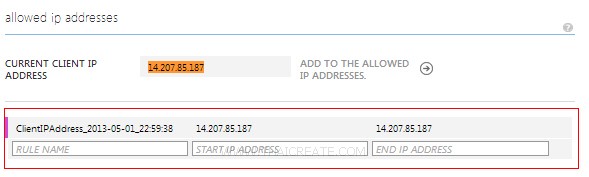
ในการที่จะเขียน .NET Application แบบ Client Server จะต้องมีการ Allow IP Address ของ Client ที่จะเชื่อมต่อไปยัง SQL Database ด้วย
Connection String : ตอนที่ 6 : SQL Azure รู้จักกับ Connection String สิทธิ์การใช้งาน SQL Database
ในตัวอย่างนีจะไม่ได้เจาะจงลงเข้าไปในรายละเอียดของการพัฒนา Java Application เพียงแต่จะยกตัวอย่างและรูปแบบการเรียกใช้ข้อมูลการติดต่อระหว่าง Java กับ SQL Database บน Windows Azure ซึ่งสามารถที่จะนำไปเป็นตัวอย่างในการพัฒนาโปรแกรมด้วย Java ได้เกือบทุกประเภทของ Application
Using the JDBC Driver สำหรับ Java และ SQL Database
JDBC SQL Database ConnectionString
jdbc:sqlserver://bc6hela9fr.database.windows.net:1433;database=thaicreate-db;user=thaicreate-user@bc6hela9fr;password={your_password_here};encrypt=true;hostNameInCertificate=*.database.windows.net;loginTimeout=30;
ให้ Import Library เข้าไปใน Class
import java.sql.*;
import com.microsoft.sqlserver.jdbc.*;
Connection String จริง ๆ ที่จะใช้
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
ตัวอย่างต่อไปนี้จะเป็นรูปแบบการเขียน Code เพื่อเชื่อมต่อไปยัง SQL Database ในรูปแบบต่าง ๆ
To create a table (การสร้างตาราง)
import java.sql.*;
import com.microsoft.sqlserver.jdbc.*;
public class HelloSQLAzure {
public static void main(String[] args)
{
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString =
"CREATE TABLE customer ( " +
"[CustomerID] [varchar](4) NOT NULL, " +
"[Name] [varchar](50) NULL, " +
"[Email] [varchar](50) NULL, " +
"[CountryCode] [varchar](2) NULL, " +
"[Budget] [float] NULL, " +
"[Used] [float] NULL, " +
" CONSTRAINT [PK_customer] PRIMARY KEY CLUSTERED " +
" ( " +
" [CustomerID] ASC " +
" ) " +
"); " +
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
statement.executeUpdate(sqlString);
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling
catch (ClassNotFoundException cnfe)
{
System.out.println("ClassNotFoundException " +
cnfe.getMessage());
}
catch (Exception e)
{
System.out.println("Exception " + e.getMessage());
e.printStackTrace();
}
finally
{
try
{
// Close resources.
if (null != connection) connection.close();
if (null != statement) statement.close();
if (null != resultSet) resultSet.close();
}
catch (SQLException sqlException)
{
// No additional action if close() statements fail.
}
}
}
}
To create an index on a table (การสร้างอินเดกให้กับตาราง)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString =
"CREATE CLUSTERED INDEX index1 " + "ON customer (Email)";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
statement.executeUpdate(sqlString);
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling and resource closing not shown...
To insert rows (การบันทึกรายการลงในตาราง)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString =
"SET IDENTITY_INSERT customer ON " +
"INSERT INTO customer " +
"(CustomerID, Name, Email, CountryCode, Budget, Used) " +
"VALUES('C005', 'Surachai Sirisart', '[email protected]'," +
" 'TH','2000000','0') ";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
statement.executeUpdate(sqlString);
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling and resource closing not shown...
To retrieve rows (การอ่านข้อมูลจากตาราง)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString = "SELECT * FROM customer";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
resultSet = statement.executeQuery(sqlString);
// Loop through the results
while (resultSet.next())
{
// Print out the row data
System.out.println(
"CustomerID : " + resultSet.getString("CustomerID") +
"Name : " + resultSet.getString("Name") +
"Email : " + resultSet.getString("Email") +
"CountryCode : " + resultSet.getString("CountryCode") +
"Budget : " + resultSet.getString("Budget") +
"Used : " + resultSet.getString("Used"));
}
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling and resource closing not shown...
To retrieve a count of rows (การนับจำนวนแถวข้อมูลของตาราง)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString = "SELECT COUNT (CustomerID) FROM customer";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
resultSet = statement.executeQuery(sqlString);
// Print out the returned number of rows.
while (resultSet.next())
{
System.out.println("There were " +
resultSet.getInt(1) +
" rows returned.");
}
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling and resource closing not shown...
To update rows (การแก้ไขอัพเดดข้อมูลในตาราง)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString =
"UPDATE customer " + "SET Name = 'Adisorn Bunsong' " + "WHERE CustomerID='C005'";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
statement.executeUpdate(sqlString);
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}// Exception handling and resource closing not shown...
To delete rows (แก้ไขข้อมูลในตาราง)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString =
"DELETE from customer " +
"WHERE CustomerID='C005' ";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
statement.executeUpdate(sqlString);
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling and resource closing not shown...
To check whether a table exists (ตรวจสอบตารางว่ามีอยู่หรือไม่)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString =
"IF EXISTS (SELECT 1 " +
"FROM sysobjects " +
"WHERE xtype='u' AND name='customer') " +
"SELECT 'customer table exists.'" +
"ELSE " +
"SELECT 'customer table does not exist.'";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
resultSet = statement.executeQuery(sqlString);
// Display the result.
while (resultSet.next())
{
System.out.println(resultSet.getString(1));
}
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling and resource closing not shown...
To drop an index (การลบอินเดก)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString =
"DROP INDEX index1 " +
"ON customer";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
statement.executeUpdate(sqlString);
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling and resource closing not shown...
To drop a table (การลบตาราง)
// Connection string for your SQL Database server.
// Change the values assigned to your_server,
// your_user@your_server,
// and your_password.
String connectionString =
"jdbc:sqlserver://bc6hela9fr.database.windows.net:1433" + ";" +
"database=thaicreate-db" + ";" +
"user=thaicreate-user@bc6hela9fr" + ";" +
"password=password@123";
// The types for the following variables are
// defined in the java.sql library.
Connection connection = null; // For making the connection
Statement statement = null; // For the SQL statement
ResultSet resultSet = null; // For the result set, if applicable
try
{
// Ensure the SQL Server driver class is available.
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
// Establish the connection.
connection = DriverManager.getConnection(connectionString);
// Define the SQL string.
String sqlString = "DROP TABLE customer";
// Use the connection to create the SQL statement.
statement = connection.createStatement();
// Execute the statement.
statement.executeUpdate(sqlString);
// Provide a message when processing is complete.
System.out.println("Processing complete.");
}
// Exception handling and resource closing not shown...
อ่านเพิ่มเติม
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2013-05-03 13:49:35 /
2017-03-24 10:58:36 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|