ตอนที่ 8 : เขียน PHP เช่นการ Insert / Update / Delete ข้อมูลบน SQL Database |
ตอนที่ 8 : PHP ร่วมกับ SQL Azure เช่นการ Insert / Update / Delete ข้อมูลบน SQL Database ตัวอย่างนี้จะเป็นการใช้ PHP ติดต่อกับข้อมูล SQL Azure หรือ SQL Database บน Windows Azure และการกระทำกับข้อมูล เช่น การ Insert (เพิ่มข้อมูล) , Edit หรือ Update (แก้ไขข้อมูล) และ Delete (ลบข้อมูล) ที่อยู่ในตารางที่ถูกจัดเก็บไว้บน SQL Database โดนจะยกตัวอย่างการเขียน PHP กับ SQL Database ให้เข้าใจคร่าว ๆ 3 รุปแบบคือ Odbc , PDO และ sqlsrv
จากตัวอย่างกาอนหน้านี้เราได้ทำการสร้าง SQL Database บน Windows Azure และทำการเชื่อมต่อพร้อมกับสร้าง Table ขึ้นมา 1 รายการเรียบร้อยแล้ว
ตอนที่ 3 : เชื่อมต่อ SQL Database ผ่าน SQL Server Management Studio (SSMS)
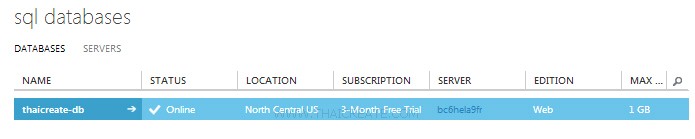
Service ของ SQL Database
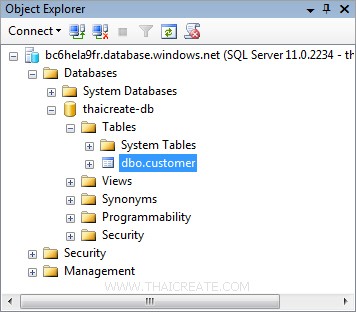
Table ชื่อว่า customer
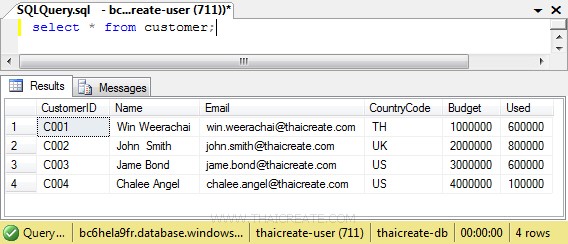
รายการข้อมูลบนตารางของ customer
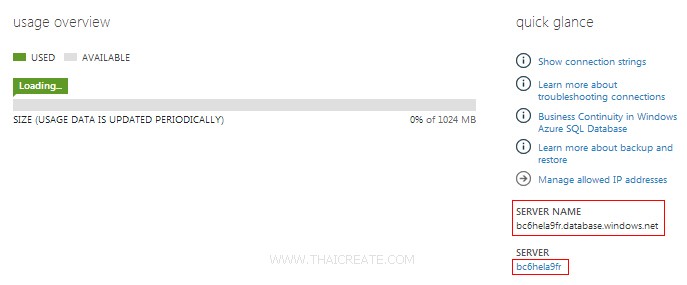
Dashboard และรายละเอียดของ Server
SQL Database Info
Server Name : bc6hela9fr.database.windows.net
Server : bc6hela9fr
User : thaicreate-user
Password : password@123
Database Name : thaicreate-db
Connection String : ตอนที่ 6 : SQL Azure รู้จักกับ Connection String สิทธิ์การใช้งาน SQL Database

สร้าง Service ของ Web Site เพื่อทดสอบ PHP กับ SQL Database
ตัวอย่างที่ 1 การใช้ PHP เชื่อมต่อกับ SQL Database ผ่าน Odbc เพื่อ Insert/Delete/Update ข้อมูล
รูปแบบ
//*** Add Condition ***//
if($_POST["hdnCmd"] == "Add")
{
$strSQL = "INSERT INTO customer ";
$strSQL .="(CustomerID,Name,Email,CountryCode,Budget,Used) ";
$strSQL .="VALUES ";
$strSQL .="('".$_POST["txtAddCustomerID"]."','".$_POST["txtAddName"]."' ";
$strSQL .=",'".$_POST["txtAddEmail"]."' ";
$strSQL .=",'".$_POST["txtAddCountryCode"]."','".$_POST["txtAddBudget"]."' ";
$strSQL .=",'".$_POST["txtAddUsed"]."') ";
$objQuery = odbc_exec($objConnect,$strSQL) or die(odbc_errormsg());
}
//*** Update Condition ***//
if($_POST["hdnCmd"] == "Update")
{
$strSQL = "UPDATE customer SET ";
$strSQL .="CustomerID = '".$_POST["txtEditCustomerID"]."' ";
$strSQL .=",Name = '".$_POST["txtEditName"]."' ";
$strSQL .=",Email = '".$_POST["txtEditEmail"]."' ";
$strSQL .=",CountryCode = '".$_POST["txtEditCountryCode"]."' ";
$strSQL .=",Budget = '".$_POST["txtEditBudget"]."' ";
$strSQL .=",Used = '".$_POST["txtEditUsed"]."' ";
$strSQL .="WHERE CustomerID = '".$_POST["hdnEditCustomerID"]."' ";
$objQuery = odbc_exec($objConnect,$strSQL) or die(odbc_errormsg());
}
//*** Delete Condition ***//
if($_GET["Action"] == "Del")
{
$strSQL = "DELETE FROM customer ";
$strSQL .="WHERE CustomerID = '".$_GET["CusID"]."' ";
$objQuery = odbc_exec($objConnect,$strSQL) or die(odbc_errormsg());
}
Sample : phpSqlDatabaseOdbc.php
<html>
<head>
<title>ThaiCreate.Com PHP & Windows Azure (SQL Azure)</title>
</head>
<body>
<?php
$server = "bc6hela9fr.database.windows.net,1433";
$database = "thaicreate-db";
$user = "thaicreate-user@bc6hela9fr";
$password = "password@123";
$objConnect = odbc_connect("Driver={SQL Server Native Client 10.0};Server=$server;Database=$database;", $user, $password) or die(odbc_errormsg());
//*** Add Condition ***//
if($_POST["hdnCmd"] == "Add")
{
$strSQL = "INSERT INTO customer ";
$strSQL .="(CustomerID,Name,Email,CountryCode,Budget,Used) ";
$strSQL .="VALUES ";
$strSQL .="('".$_POST["txtAddCustomerID"]."','".$_POST["txtAddName"]."' ";
$strSQL .=",'".$_POST["txtAddEmail"]."' ";
$strSQL .=",'".$_POST["txtAddCountryCode"]."','".$_POST["txtAddBudget"]."' ";
$strSQL .=",'".$_POST["txtAddUsed"]."') ";
$objQuery = odbc_exec($objConnect,$strSQL) or die(odbc_errormsg());
}
//*** Update Condition ***//
if($_POST["hdnCmd"] == "Update")
{
$strSQL = "UPDATE customer SET ";
$strSQL .="CustomerID = '".$_POST["txtEditCustomerID"]."' ";
$strSQL .=",Name = '".$_POST["txtEditName"]."' ";
$strSQL .=",Email = '".$_POST["txtEditEmail"]."' ";
$strSQL .=",CountryCode = '".$_POST["txtEditCountryCode"]."' ";
$strSQL .=",Budget = '".$_POST["txtEditBudget"]."' ";
$strSQL .=",Used = '".$_POST["txtEditUsed"]."' ";
$strSQL .="WHERE CustomerID = '".$_POST["hdnEditCustomerID"]."' ";
$objQuery = odbc_exec($objConnect,$strSQL) or die(odbc_errormsg());
}
//*** Delete Condition ***//
if($_GET["Action"] == "Del")
{
$strSQL = "DELETE FROM customer ";
$strSQL .="WHERE CustomerID = '".$_GET["CusID"]."' ";
$objQuery = odbc_exec($objConnect,$strSQL) or die(odbc_errormsg());
}
$strSQL = "SELECT * FROM customer";
$objQuery = odbc_exec($objConnect,$strSQL) or die(odbc_errormsg());
?>
<form name="frmMain" method="post" action="<?php echo $_SERVER["PHP_SELF"];?>">
<input type="hidden" name="hdnCmd" value="">
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
<th width="30"> <div align="center">Edit </div></th>
<th width="30"> <div align="center">Delete </div></th>
</tr>
<?php
while($objResult = odbc_fetch_array($objQuery))
{
?>
<?php
if($objResult["CustomerID"] == $_GET["CusID"] and $_GET["Action"] == "Edit")
{
?>
<tr>
<td><div align="center">
<input type="text" name="txtEditCustomerID" size="5" value="<?php echo $objResult["CustomerID"];?>">
<input type="hidden" name="hdnEditCustomerID" size="5" value="<?php echo $objResult["CustomerID"];?>">
</div></td>
<td><input type="text" name="txtEditName" size="20" value="<?php echo $objResult["Name"];?>"></td>
<td><input type="text" name="txtEditEmail" size="20" value="<?php echo $objResult["Email"];?>"></td>
<td><div align="center"><input type="text" name="txtEditCountryCode" size="2" value="<?php echo $objResult["CountryCode"];?>"></div></td>
<td align="right"><input type="text" name="txtEditBudget" size="5" value="<?php echo $objResult["Budget"];?>"></td>
<td align="right"><input type="text" name="txtEditUsed" size="5" value="<?php echo $objResult["Used"];?>"></td>
<td colspan="2" align="right"><div align="center">
<input name="btnAdd" type="button" id="btnUpdate" value="Update" OnClick="frmMain.hdnCmd.value='Update';frmMain.submit();">
<input name="btnAdd" type="button" id="btnCancel" value="Cancel" OnClick="window.location='<?php echo $_SERVER["PHP_SELF"];?>';">
</div></td>
</tr>
<?php
}
else
{
?>
<tr>
<td><div align="center"><?php echo $objResult["CustomerID"];?></div></td>
<td><?php echo $objResult["Name"];?></td>
<td><?php echo $objResult["Email"];?></td>
<td><div align="center"><?php echo $objResult["CountryCode"];?></div></td>
<td align="right"><?php echo $objResult["Budget"];?></td>
<td align="right"><?php echo $objResult["Used"];?></td>
<td align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?Action=Edit&CusID=<?php echo $objResult["CustomerID"];?>">Edit</a></td>
<td align="center"><a href="JavaScript:if(confirm('Confirm Delete?')==true){window.location='<?php echo $_SERVER["PHP_SELF"];?>?Action=Del&CusID=<?php echo $objResult["CustomerID"];?>';}">Delete</a></td>
</tr>
<?php
}
?>
<?php
}
?>
<tr>
<td><div align="center"><input type="text" name="txtAddCustomerID" size="5"></div></td>
<td><input type="text" name="txtAddName" size="20"></td>
<td><input type="text" name="txtAddEmail" size="20"></td>
<td><div align="center"><input type="text" name="txtAddCountryCode" size="2"></div></td>
<td align="right"><input type="text" name="txtAddBudget" size="5"></td>
<td align="right"><input type="text" name="txtAddUsed" size="5"></td>
<td colspan="2" align="right"><div align="center">
<input name="btnAdd" type="button" id="btnAdd" value="Add" OnClick="frmMain.hdnCmd.value='Add';frmMain.submit();">
</div></td>
</tr>
</table>
</form>
<?php
odbc_close($objConnect);
?>
</body>
</html>
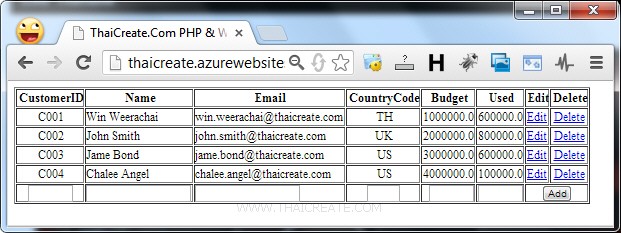

ผลลัพธ์ที่ได้
ตัวอย่างที่ 2 การใช้ PHP เชื่อมต่อกับ SQL Database ผ่าน PDO เพื่อ Insert/Delete/Update ข้อมูล
รูปแบบ
//*** Add Condition ***//
if($_POST["hdnCmd"] == "Add")
{
$strSQL = "INSERT INTO customer ";
$strSQL .="(CustomerID,Name,Email,CountryCode,Budget,Used) ";
$strSQL .="VALUES ";
$strSQL .="('".$_POST["txtAddCustomerID"]."','".$_POST["txtAddName"]."' ";
$strSQL .=",'".$_POST["txtAddEmail"]."' ";
$strSQL .=",'".$_POST["txtAddCountryCode"]."','".$_POST["txtAddBudget"]."' ";
$strSQL .=",'".$_POST["txtAddUsed"]."') ";
if($conn->exec($strSQL) !== false) echo 'Error!';
}
//*** Update Condition ***//
if($_POST["hdnCmd"] == "Update")
{
$strSQL = "UPDATE customer SET ";
$strSQL .="CustomerID = '".$_POST["txtEditCustomerID"]."' ";
$strSQL .=",Name = '".$_POST["txtEditName"]."' ";
$strSQL .=",Email = '".$_POST["txtEditEmail"]."' ";
$strSQL .=",CountryCode = '".$_POST["txtEditCountryCode"]."' ";
$strSQL .=",Budget = '".$_POST["txtEditBudget"]."' ";
$strSQL .=",Used = '".$_POST["txtEditUsed"]."' ";
$strSQL .="WHERE CustomerID = '".$_POST["hdnEditCustomerID"]."' ";
if($conn->exec($strSQL) !== false) echo 'Error!';
}
//*** Delete Condition ***//
if($_GET["Action"] == "Del")
{
$strSQL = "DELETE FROM customer ";
$strSQL .="WHERE CustomerID = '".$_GET["CusID"]."' ";
if($conn->exec($strSQL) !== false) echo 'Error!';
}
Sample : phpSqlDatabasePDO.php
<html>
<head>
<title>ThaiCreate.Com PHP & Windows Azure (SQL Azure)</title>
</head>
<body>
<?php
$serverName = "tcp:bc6hela9fr.database.windows.net,1433";
$userName = 'thaicreate-user@bc6hela9fr';
$userPassword = 'password@123';
$dbName = "thaicreate-db";
$conn = new PDO("sqlsrv:server=$serverName ; Database = $dbName", $userName, $userPassword);
//*** Add Condition ***//
if($_POST["hdnCmd"] == "Add")
{
$strSQL = "INSERT INTO customer ";
$strSQL .="(CustomerID,Name,Email,CountryCode,Budget,Used) ";
$strSQL .="VALUES ";
$strSQL .="('".$_POST["txtAddCustomerID"]."','".$_POST["txtAddName"]."' ";
$strSQL .=",'".$_POST["txtAddEmail"]."' ";
$strSQL .=",'".$_POST["txtAddCountryCode"]."','".$_POST["txtAddBudget"]."' ";
$strSQL .=",'".$_POST["txtAddUsed"]."') ";
if($conn->exec($strSQL) !== false) echo 'Error!';
}
//*** Update Condition ***//
if($_POST["hdnCmd"] == "Update")
{
$strSQL = "UPDATE customer SET ";
$strSQL .="CustomerID = '".$_POST["txtEditCustomerID"]."' ";
$strSQL .=",Name = '".$_POST["txtEditName"]."' ";
$strSQL .=",Email = '".$_POST["txtEditEmail"]."' ";
$strSQL .=",CountryCode = '".$_POST["txtEditCountryCode"]."' ";
$strSQL .=",Budget = '".$_POST["txtEditBudget"]."' ";
$strSQL .=",Used = '".$_POST["txtEditUsed"]."' ";
$strSQL .="WHERE CustomerID = '".$_POST["hdnEditCustomerID"]."' ";
if($conn->exec($strSQL) !== false) echo 'Error!';
}
//*** Delete Condition ***//
if($_GET["Action"] == "Del")
{
$strSQL = "DELETE FROM customer ";
$strSQL .="WHERE CustomerID = '".$_GET["CusID"]."' ";
if($conn->exec($strSQL) !== false) echo 'Error!';
}
/** List Record ***/
$strSQL = "SELECT * FROM customer";
$stmt = $conn->prepare($strSQL);
$stmt->execute();
?>
<form name="frmMain" method="post" action="<?php echo $_SERVER["PHP_SELF"];?>">
<input type="hidden" name="hdnCmd" value="">
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
<th width="30"> <div align="center">Edit </div></th>
<th width="30"> <div align="center">Delete </div></th>
</tr>
<?php
$arrValues = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($arrValues as $row){
?>
<?php
if($row["CustomerID"] == $_GET["CusID"] and $_GET["Action"] == "Edit")
{
?>
<tr>
<td><div align="center">
<input type="text" name="txtEditCustomerID" size="5" value="<?php echo $row["CustomerID"];?>">
<input type="hidden" name="hdnEditCustomerID" size="5" value="<?php echo $row["CustomerID"];?>">
</div></td>
<td><input type="text" name="txtEditName" size="20" value="<?php echo $row["Name"];?>"></td>
<td><input type="text" name="txtEditEmail" size="20" value="<?php echo $row["Email"];?>"></td>
<td><div align="center"><input type="text" name="txtEditCountryCode" size="2" value="<?php echo $row["CountryCode"];?>"></div></td>
<td align="right"><input type="text" name="txtEditBudget" size="5" value="<?php echo $row["Budget"];?>"></td>
<td align="right"><input type="text" name="txtEditUsed" size="5" value="<?php echo $row["Used"];?>"></td>
<td colspan="2" align="right"><div align="center">
<input name="btnAdd" type="button" id="btnUpdate" value="Update" OnClick="frmMain.hdnCmd.value='Update';frmMain.submit();">
<input name="btnAdd" type="button" id="btnCancel" value="Cancel" OnClick="window.location='<?php echo $_SERVER["PHP_SELF"];?>';">
</div></td>
</tr>
<?php
}
else
{
?>
<tr>
<td><div align="center"><?php echo $row["CustomerID"];?></div></td>
<td><?php echo $row["Name"];?></td>
<td><?php echo $row["Email"];?></td>
<td><div align="center"><?php echo $row["CountryCode"];?></div></td>
<td align="right"><?php echo $row["Budget"];?></td>
<td align="right"><?php echo $row["Used"];?></td>
<td align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?Action=Edit&CusID=<?php echo $row["CustomerID"];?>">Edit</a></td>
<td align="center"><a href="JavaScript:if(confirm('Confirm Delete?')==true){window.location='<?php echo $_SERVER["PHP_SELF"];?>?Action=Del&CusID=<?php echo $row["CustomerID"];?>';}">Delete</a></td>
</tr>
<?php
}
?>
<?php
}
?>
<tr>
<td><div align="center"><input type="text" name="txtAddCustomerID" size="5"></div></td>
<td><input type="text" name="txtAddName" size="20"></td>
<td><input type="text" name="txtAddEmail" size="20"></td>
<td><div align="center"><input type="text" name="txtAddCountryCode" size="2"></div></td>
<td align="right"><input type="text" name="txtAddBudget" size="5"></td>
<td align="right"><input type="text" name="txtAddUsed" size="5"></td>
<td colspan="2" align="right"><div align="center">
<input name="btnAdd" type="button" id="btnAdd" value="Add" OnClick="frmMain.hdnCmd.value='Add';frmMain.submit();">
</div></td>
</tr>
</table>
</form>
<?php
$conn = null;
?>
</body>
</html>
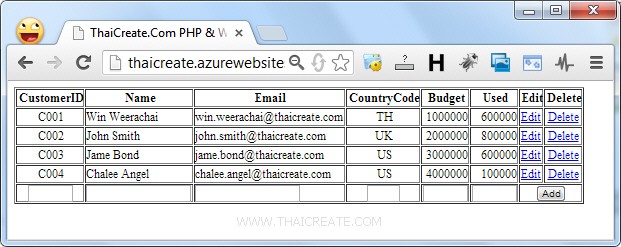
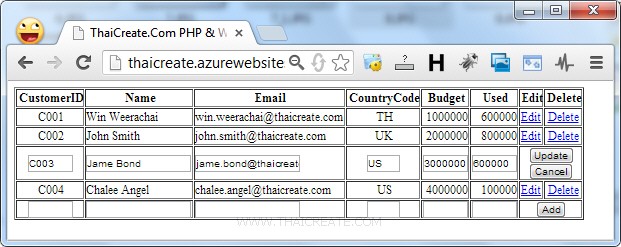
ผลลัพธ์

ตัวอย่างที่ 3 การใช้ PHP เชื่อมต่อกับ SQL Database ผ่าน PHP SQL Server (sqlsrv) เพื่อ Insert/Delete/Update ข้อมูล
รูปแบบ
//*** Add Condition ***//
if($_POST["hdnCmd"] == "Add")
{
$strSQL = "INSERT INTO customer ";
$strSQL .="(CustomerID,Name,Email,CountryCode,Budget,Used) ";
$strSQL .="VALUES ";
$strSQL .="('".$_POST["txtAddCustomerID"]."','".$_POST["txtAddName"]."' ";
$strSQL .=",'".$_POST["txtAddEmail"]."' ";
$strSQL .=",'".$_POST["txtAddCountryCode"]."','".$_POST["txtAddBudget"]."' ";
$strSQL .=",'".$_POST["txtAddUsed"]."') ";
$stmt = sqlsrv_query( $conn, $strSQL);
if( $stmt === false ) {
die( print_r( sqlsrv_errors(), true));
}
}
//*** Update Condition ***//
if($_POST["hdnCmd"] == "Update")
{
$strSQL = "UPDATE customer SET ";
$strSQL .="CustomerID = '".$_POST["txtEditCustomerID"]."' ";
$strSQL .=",Name = '".$_POST["txtEditName"]."' ";
$strSQL .=",Email = '".$_POST["txtEditEmail"]."' ";
$strSQL .=",CountryCode = '".$_POST["txtEditCountryCode"]."' ";
$strSQL .=",Budget = '".$_POST["txtEditBudget"]."' ";
$strSQL .=",Used = '".$_POST["txtEditUsed"]."' ";
$strSQL .="WHERE CustomerID = '".$_POST["hdnEditCustomerID"]."' ";
$stmt = sqlsrv_query( $conn, $strSQL);
if( $stmt === false ) {
die( print_r( sqlsrv_errors(), true));
}
}
//*** Delete Condition ***//
if($_GET["Action"] == "Del")
{
$strSQL = "DELETE FROM customer ";
$strSQL .="WHERE CustomerID = '".$_GET["CusID"]."' ";
$stmt = sqlsrv_query( $conn, $strSQL);
if( $stmt === false ) {
die( print_r( sqlsrv_errors(), true));
}
}
Sample : phpSqlDatabasesqlsrv.php
<html>
<head>
<title>ThaiCreate.Com PHP & Windows Azure (SQL Azure)</title>
</head>
<body>
<?php
$serverName = "tcp:bc6hela9fr.database.windows.net,1433";
$userName = 'thaicreate-user@bc6hela9fr';
$userPassword = 'password@123';
$dbName = "thaicreate-db";
$connectionInfo = array("Database"=>$dbName, "UID"=>$userName, "PWD"=>$userPassword, "MultipleActiveResultSets"=>true);
sqlsrv_configure('WarningsReturnAsErrors', 0);
$conn = sqlsrv_connect( $serverName, $connectionInfo);
//*** Add Condition ***//
if($_POST["hdnCmd"] == "Add")
{
$strSQL = "INSERT INTO customer ";
$strSQL .="(CustomerID,Name,Email,CountryCode,Budget,Used) ";
$strSQL .="VALUES ";
$strSQL .="('".$_POST["txtAddCustomerID"]."','".$_POST["txtAddName"]."' ";
$strSQL .=",'".$_POST["txtAddEmail"]."' ";
$strSQL .=",'".$_POST["txtAddCountryCode"]."','".$_POST["txtAddBudget"]."' ";
$strSQL .=",'".$_POST["txtAddUsed"]."') ";
$stmt = sqlsrv_query( $conn, $strSQL);
if( $stmt === false ) {
die( print_r( sqlsrv_errors(), true));
}
}
//*** Update Condition ***//
if($_POST["hdnCmd"] == "Update")
{
$strSQL = "UPDATE customer SET ";
$strSQL .="CustomerID = '".$_POST["txtEditCustomerID"]."' ";
$strSQL .=",Name = '".$_POST["txtEditName"]."' ";
$strSQL .=",Email = '".$_POST["txtEditEmail"]."' ";
$strSQL .=",CountryCode = '".$_POST["txtEditCountryCode"]."' ";
$strSQL .=",Budget = '".$_POST["txtEditBudget"]."' ";
$strSQL .=",Used = '".$_POST["txtEditUsed"]."' ";
$strSQL .="WHERE CustomerID = '".$_POST["hdnEditCustomerID"]."' ";
$stmt = sqlsrv_query( $conn, $strSQL);
if( $stmt === false ) {
die( print_r( sqlsrv_errors(), true));
}
}
//*** Delete Condition ***//
if($_GET["Action"] == "Del")
{
$strSQL = "DELETE FROM customer ";
$strSQL .="WHERE CustomerID = '".$_GET["CusID"]."' ";
$stmt = sqlsrv_query( $conn, $strSQL);
if( $stmt === false ) {
die( print_r( sqlsrv_errors(), true));
}
}
$strSQL = "SELECT * FROM customer";
$stmt = sqlsrv_query($conn, $strSQL);
?>
<form name="frmMain" method="post" action="<?php echo $_SERVER["PHP_SELF"];?>">
<input type="hidden" name="hdnCmd" value="">
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="98"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="59"> <div align="center">Budget </div></th>
<th width="71"> <div align="center">Used </div></th>
<th width="30"> <div align="center">Edit </div></th>
<th width="30"> <div align="center">Delete </div></th>
</tr>
<?php
while($row = sqlsrv_fetch_array($stmt, SQLSRV_FETCH_ASSOC))
{
?>
<?php
if($row["CustomerID"] == $_GET["CusID"] and $_GET["Action"] == "Edit")
{
?>
<tr>
<td><div align="center">
<input type="text" name="txtEditCustomerID" size="5" value="<?php echo $row["CustomerID"];?>">
<input type="hidden" name="hdnEditCustomerID" size="5" value="<?php echo $row["CustomerID"];?>">
</div></td>
<td><input type="text" name="txtEditName" size="20" value="<?php echo $row["Name"];?>"></td>
<td><input type="text" name="txtEditEmail" size="20" value="<?php echo $row["Email"];?>"></td>
<td><div align="center"><input type="text" name="txtEditCountryCode" size="2" value="<?php echo $row["CountryCode"];?>"></div></td>
<td align="right"><input type="text" name="txtEditBudget" size="5" value="<?php echo $row["Budget"];?>"></td>
<td align="right"><input type="text" name="txtEditUsed" size="5" value="<?php echo $row["Used"];?>"></td>
<td colspan="2" align="right"><div align="center">
<input name="btnAdd" type="button" id="btnUpdate" value="Update" OnClick="frmMain.hdnCmd.value='Update';frmMain.submit();">
<input name="btnAdd" type="button" id="btnCancel" value="Cancel" OnClick="window.location='<?php echo $_SERVER["PHP_SELF"];?>';">
</div></td>
</tr>
<?php
}
else
{
?>
<tr>
<td><div align="center"><?php echo $row["CustomerID"];?></div></td>
<td><?php echo $row["Name"];?></td>
<td><?php echo $row["Email"];?></td>
<td><div align="center"><?php echo $row["CountryCode"];?></div></td>
<td align="right"><?php echo $row["Budget"];?></td>
<td align="right"><?php echo $row["Used"];?></td>
<td align="center"><a href="<?php echo $_SERVER["PHP_SELF"];?>?Action=Edit&CusID=<?php echo $row["CustomerID"];?>">Edit</a></td>
<td align="center"><a href="JavaScript:if(confirm('Confirm Delete?')==true){window.location='<?php echo $_SERVER["PHP_SELF"];?>?Action=Del&CusID=<?php echo $row["CustomerID"];?>';}">Delete</a></td>
</tr>
<?php
}
?>
<?php
}
?>
<tr>
<td><div align="center"><input type="text" name="txtAddCustomerID" size="5"></div></td>
<td><input type="text" name="txtAddName" size="20"></td>
<td><input type="text" name="txtAddEmail" size="20"></td>
<td><div align="center"><input type="text" name="txtAddCountryCode" size="2"></div></td>
<td align="right"><input type="text" name="txtAddBudget" size="5"></td>
<td align="right"><input type="text" name="txtAddUsed" size="5"></td>
<td colspan="2" align="right"><div align="center">
<input name="btnAdd" type="button" id="btnAdd" value="Add" OnClick="frmMain.hdnCmd.value='Add';frmMain.submit();">
</div></td>
</tr>
</table>
</form>
<?php
sqlsrv_close($conn);
?>
</body>
</html>
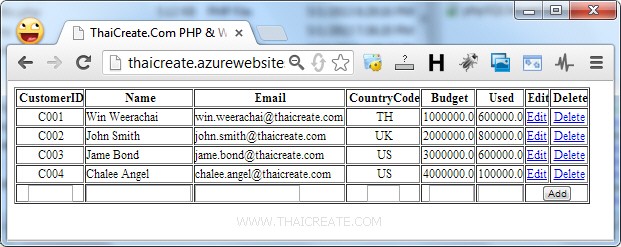
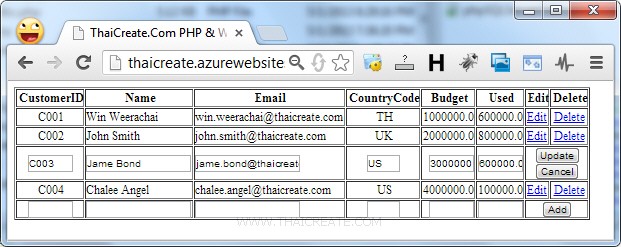
ผลลัพธ์
อ่านเพิ่มเติม
|